Hibernate practical operation of MySQL database 2024.1.8
- Prerequisite environment (Java+MySQL+Navicat+VS Code)
- 1. Introduction to Hibernate
- 2. Create tables in MySQL database
- 3. Java operates MySQL instance (Hibernate)
- 4. Summary
Prerequisite environment (Java+MySQL+Navicat+VS Code)
![]() |
![]() |
![]() |
![]() |
1. Introduction to Hibernate
Hibernate is an open source project released under the LGPL (Lesser GNU Public License) license. It can directly provide relevant support, and the underlying driver can switch databases at will, which is fast and concise. Separate the business layer from the specific database and only Hibernate
develop it to complete the persistence of data and objects. If you are interested and want to learn more, it is recommended to refer to its official documentation and guided example learning, such as QuickStart and Chinese documentation .
![]() |
![]() |
![]() |
![]() |
1.1 Understanding HQL
HQL is Hibernate Query Language
the abbreviation of HQL. It can provide richer , more flexible and more powerful query capabilities, HQL
is closer to SQL
statement query syntax, provides rich support for dynamic query parameter binding, provides SQL
query methods similar to standard statements, and also provides More object-oriented encapsulation . Especially in 实体查询、更新、删除
aspects, some common usage examples are as follows:
from User user where user age=20;
from User user where user age between 20 and 30;
from User user where user age in(20,30);
from User user where user name is null;
from User user where user name like '%zx%';
from User user where (user age%2)=1;
from User user where user age=20 and user name like '%zx%';
In addition, you can refer to the community documentation for specific grammar learning .
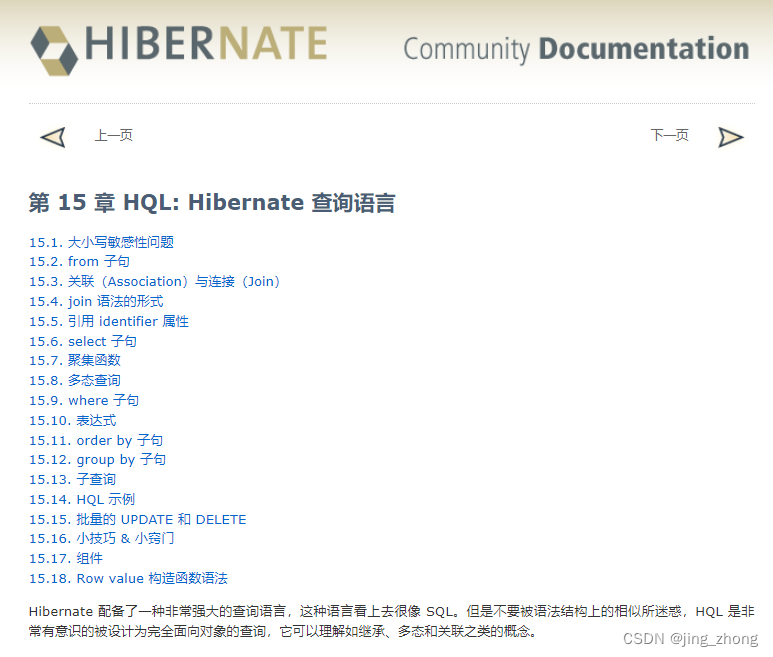
2. Create tables in MySQL database
In order to facilitate the subsequent use Java
of code to operate MySQL
the database, you first need to design the data table structure, set the number, type, length, alias, and English name of the fields in the table, write the SQL script and execute it in the database, and then view it in Navicat
or MySQL Workbench
.
2.1 Writing SQL scripts
Field name | Field Type | Field alias |
---|---|---|
id | text | Uniquely identifies |
name | text | name |
date | date | date |
income | decimal | income |
The contents of the script file in the above table create_Test.sql
are as follows:
CREATE database if NOT EXISTS `my_db` default character set utf8mb4 collate utf8mb4_unicode_ci;
use `my_db`;
SET NAMES utf8mb4;
CREATE TABLE if NOT EXISTS `test` (
`id` CHAR(50) NOT NULL,
`name` VARCHAR(50),
`date` timestamp(6),
`income` float4,
PRIMARY KEY (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
2.2 MySQL execution script
The first step is to open MySQL Workbench
or Navicat
create a new MySQL
database connection and enter the correctusernameandpasswordAfter testing the connection, you can see the database after the connection is successful;
in the second step , click New Query in the menu, and then copy SQL
the statement in the edit box. Of course, you can also open an existing SQL
file, and then click the Execute button to view The database and data table have been successfully created.
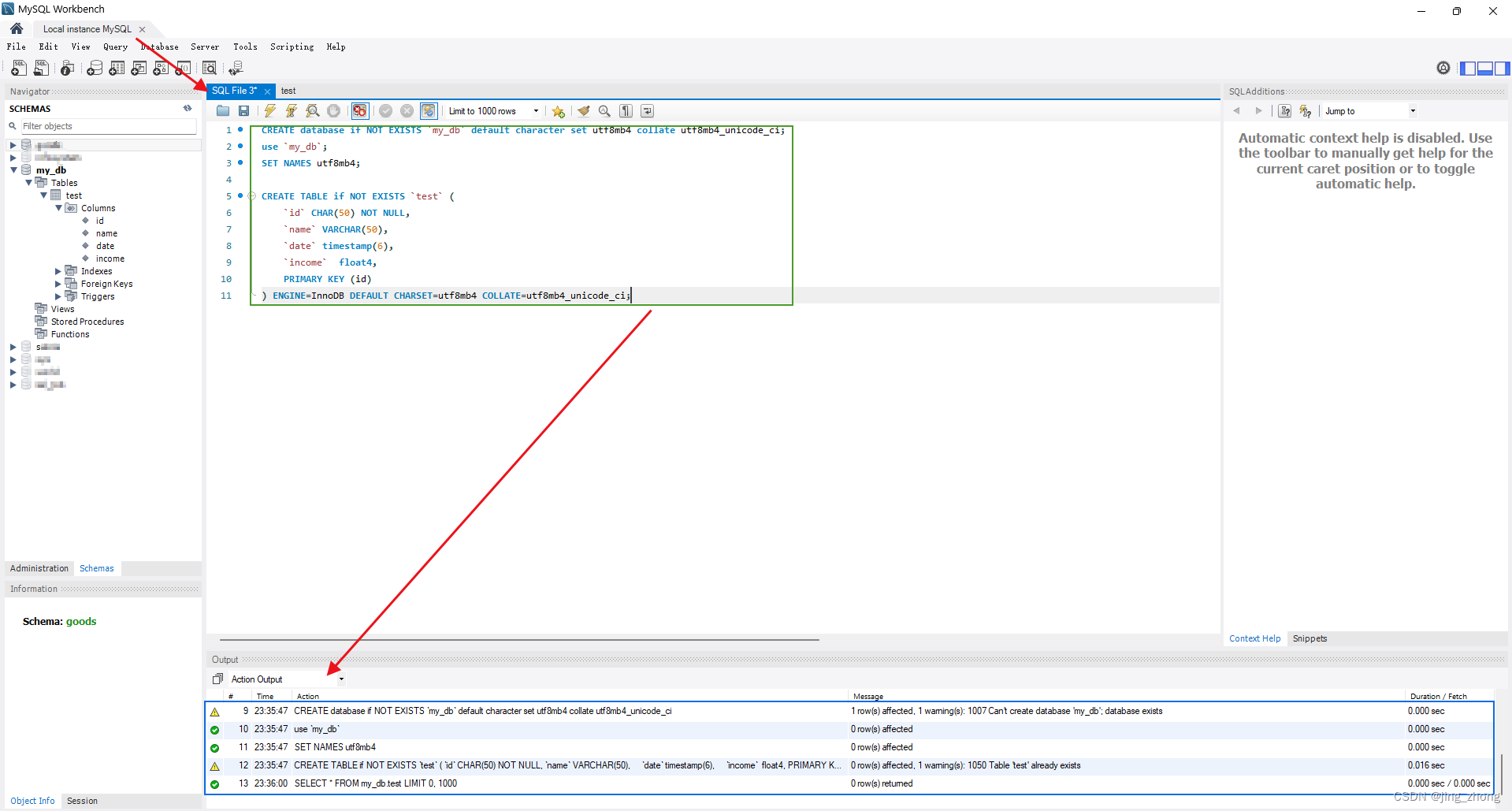
![]() |
![]() |
3. Java operates MySQL instance (Hibernate)
The following will take a complete Java
project as an example to introduce how to use pairs to perform operations Hibernate
such as adding MySQL
, deleting, modifying, and checking. The whole process may be a bit cumbersome, but it is relatively basic and can be done step by step. It is divided into: (1) Prepare three-party dependency jar
packages; (2) Understand the database connection configuration information; (3) Set up the jdbc database connection in the MySQL
configuration file ; (4) Write classes to implement database operations; (5) Test execution to see the effect.XML
MySQL
Java
3.1 Prepare dependent third-party jar packages
Java
The names of the third-party jar packages that the project code depends on are as follows, which can be downloaded from the Maven official warehouse . (Note: If you use Maven , you can also introduce it in the pom file)
antlr-2.7.7.jar
byte-buddy-1.11.22.jar
classmate-1.5.1.jar
dom4j-2.1.1.jar
hibernate-commons-annotations-5.0.4.Final.jar
hibernate-core-5.3.14.Final.jar
javax.persistence-api-2.2.jar
jboss-logging-3.3.3.Final.jar
jta-1.1.jar
mysql-connector-java-8.0.18.jar
![]() |
![]() |
3.2 Understand the MySQL database connection configuration information and configure XML files
Name database | IP address | port | username | password |
---|---|---|---|---|
my_db | localhost | 3306 | root | 123456 |
3.2.1 Create a new hibernate.cfg.xml file
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- 指定方言 -->
<property name="dialect">
org.hibernate.dialect.MySQLDialect
</property>
<!-- 链接数据库url -->
<property name="connection.url">
jdbc:mysql://localhost:3306/my_db?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8
</property>
<!-- 连接数据库的用户名 -->
<property name="connection.username">
root
</property>
<!-- 数据库的密码 -->
<property name="connection.password">
123456
</property>
<!-- 数据库驱动 -->
<property name="connection.driver_class">
com.mysql.jdbc.Driver
</property>
<!-- 显示sql语句 -->
<property name="show_sql">
true
</property>
<!-- 格式化sql语句 -->
<property name="format_sql">true</property>
<!-- 映射文件配置 -->
<mapping resource="com/Row.hbm.xml" />
</session-factory>
</hibernate-configuration>
3.2.2 Create a new Row.hbm.xml file
src
The files under the project Row.hbm.xml
are mapping configurations to the tables in hibernate
the database . At the same time, it should be noted that they are placed in the same directory ( package ) as the corresponding data table classes . Therefore, when writing string statements, you need to query them instead of actually When executed, it will be mapped to the data table! ! !my_db
test
HQL
Row
test
Row
test
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.Row" table="test">
<id name="id" column="id">
<!-- <generator class="org.hibernate.id.UUIDGenerator"/> -->
<generator class="uuid"/>
</id>
<property name="name" column="name"/>
<property name="date" column="date"/>
<property name="income" column="income"/>
</class>
</hibernate-mapping>
3.3 Write Java classes to implement database operations (Row+App)
The class is written Row.java
as test
an abstraction of each row of records in the data table, and the App
class is written to call the main function to connect to the database and perform corresponding operation instances.
3.3.1 Row.java
package com;
import java.util.Date;
import javax.persistence.*;
import org.hibernate.annotations.GenericGenerator;
@Entity
@Table
public class Row {
//设置主键
@Id //配置uuid,原本jpa是不支持uuid的,但借用hibernate的方法能够实现。
@GeneratedValue(generator = "idGenerator")
@GenericGenerator(name = "idGenerator", strategy = "org.hibernate.id.UUIDGenerator") // uuid
@Column(name = "id", unique = true, nullable = false, length = 32)
private String id;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
private Date date;
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
private Float income;
public Float getIncome() {
return income;
}
public void setIncome(Float income) {
this.income = income;
}
}
3.3.2 App.java (including main function)
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.hibernate.Session;
// import org.hibernate.id.UUIDHexGenerator;
import java.util.List;
import java.util.Date;
import com.Row;
public class App {
// 一、插入数据(新增)
public static void insertData(String name, int count)
{
Configuration config = new Configuration().configure();// 创建Configuration对象并加载hibernate.cfg.xml配置文件
SessionFactory sessionFactory = config.buildSessionFactory();// 获取SessionFactory
Session session = sessionFactory.openSession();// 从sessionFactory 获取 Session
Transaction transaction = session.beginTransaction();// 事务开启
for(int i=0;i<count;i++)
{
Row row = new Row(); //uuid已经自动生成,无须手动添加
row.setName(name);
Date date = new Date();
row.setDate(date);
Float income = new Float((i+1) * count + 0.5);
row.setIncome(income);
Object res = session.save(row); // 将对象(Row)保存到表test中
System.out.println("id:" + res);
}
transaction.commit(); // 事务提交
session.close(); // 关闭session & sessionFactory
sessionFactory.close();
}
// 二、根据条件删除数据库(删除)
public static void deleteData(String condition)
{
Configuration config = new Configuration().configure();// 创建Configuration对象并加载hibernate.cfg.xml配置文件
SessionFactory sessionFactory = config.buildSessionFactory();// 获取SessionFactory
Session session = sessionFactory.openSession();// 从sessionFactory 获取 Session
try
{
Transaction transaction = session.beginTransaction();
String hql = "delete from Row where " + condition;
int rowsAffected = session.createQuery(hql).executeUpdate();
System.out.println(rowsAffected + " rows affected.");
transaction.commit();
}
catch(Exception e){
System.out.println("出错: " + e.getMessage());
e.printStackTrace();
}
finally{
if (session != null)
session.close(); // 关闭session & sessionFactory
if (sessionFactory != null)
sessionFactory.close();
}
}
// 三、根据条件更新数据库(修改)
public static void updateData(String condition)
{
Configuration config = new Configuration().configure();// 创建Configuration对象并加载hibernate.cfg.xml配置文件
SessionFactory sessionFactory = config.buildSessionFactory();// 获取SessionFactory
Session session = sessionFactory.openSession();// 从sessionFactory 获取 Session
try
{
Transaction transaction = session.beginTransaction();
String hql = "update Row " + condition;
int rowsAffected = session.createQuery(hql).executeUpdate();
System.out.print(rowsAffected + " rows affected.");
transaction.commit();
}
catch(Exception e){
System.out.println("出错: " + e.getMessage());
e.printStackTrace();
}
finally{
if (session != null)
session.close(); // 关闭session & sessionFactory
if (sessionFactory != null)
sessionFactory.close();
}
}
// 四、根据条件查询数据库(查询)
public static void queryAllData(String condition){
Configuration config = new Configuration().configure();// 创建Configuration对象并加载hibernate.cfg.xml配置文件
SessionFactory sessionFactory = config.buildSessionFactory();// 获取SessionFactory
Session session = sessionFactory.openSession();// 从sessionFactory 获取 Session
try
{
String hql = "from Row where " + condition;
List<Row> results = session.createQuery(hql).list();
if(results.size() == 0){
System.out.println("找不到记录!");
}
else{
for(Row row: results){
System.out.println("编号:" + row.getId() + ", 名称:" + row.getName() + ", 日期:" + row.getDate() + ", 收入:" + row.getIncome());
}
}
}
catch(Exception e){
System.out.println("出错: " + e.getMessage());
e.printStackTrace();
}
finally{
if (session != null)
session.close(); // 关闭session & sessionFactory
if (sessionFactory != null)
sessionFactory.close();
}
}
public static void main(String[] args) throws Exception {
insertData("张三", 10);
// queryAllData("1=1"); // "1=1" "id = '4028896c8cdd6d55018cdd6d56420002'"
// updateData("set name = '12233330000' WHERE 1=1");
// deleteData("1=1"); // name = 'jing_zhong'
}
}
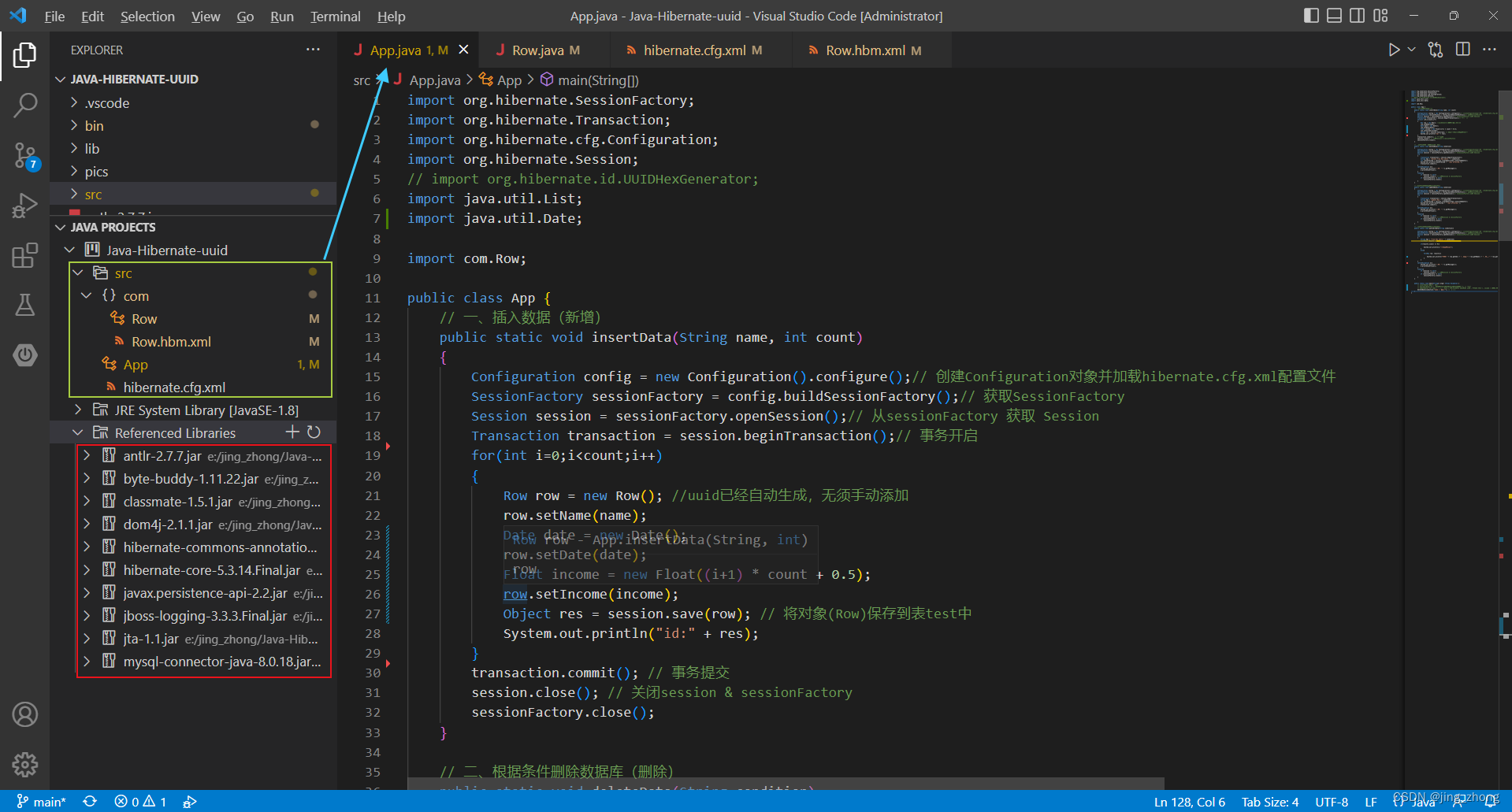
3.4 Test execution and use Navicat to view database results
Four commonly usedAdd, delete, modify and checkOperate to perform test execution. VSCode
The console and Navicat
database results are shown in the figure below.
3.4.1 New
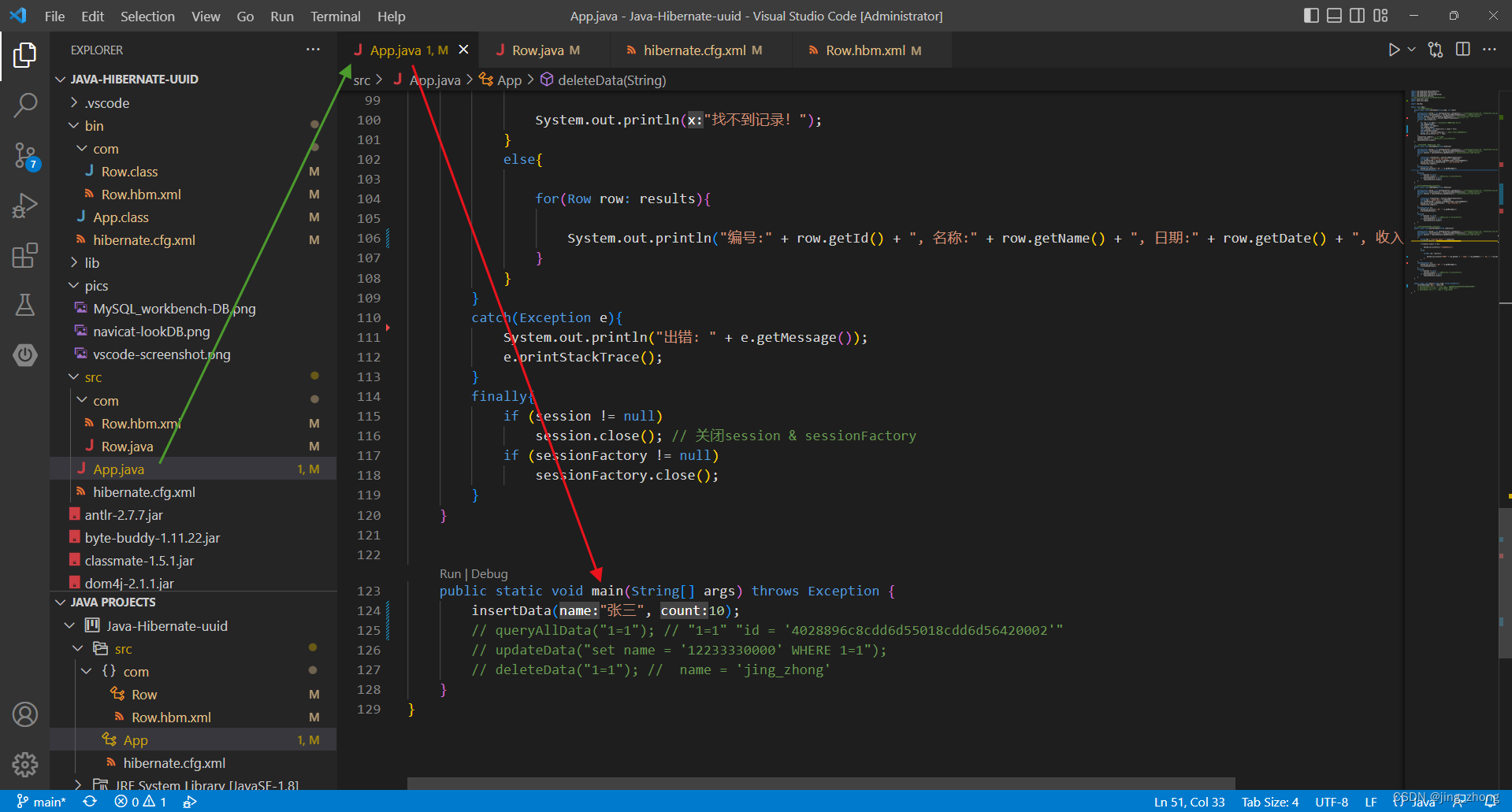
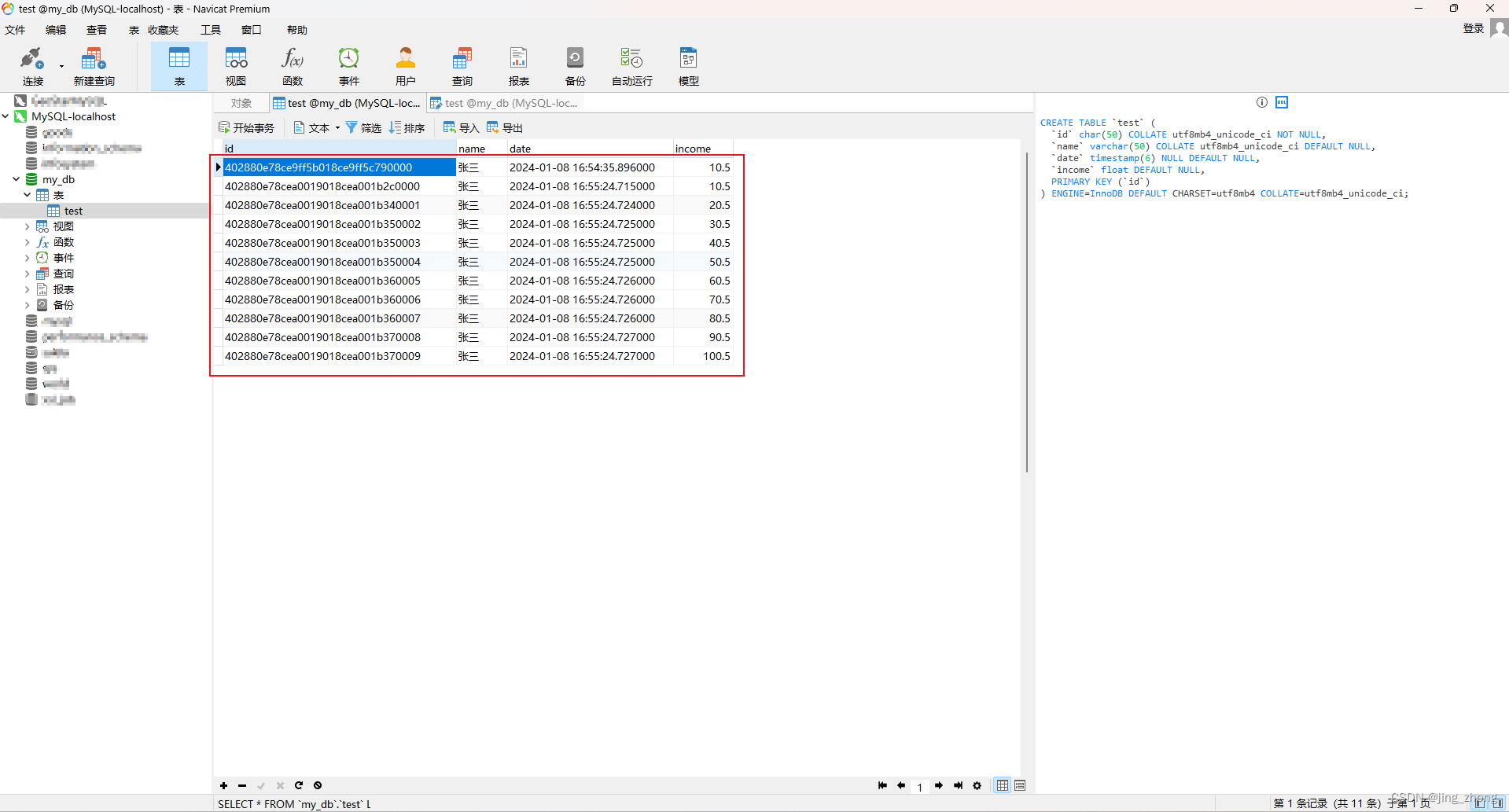
3.4.2 Modification
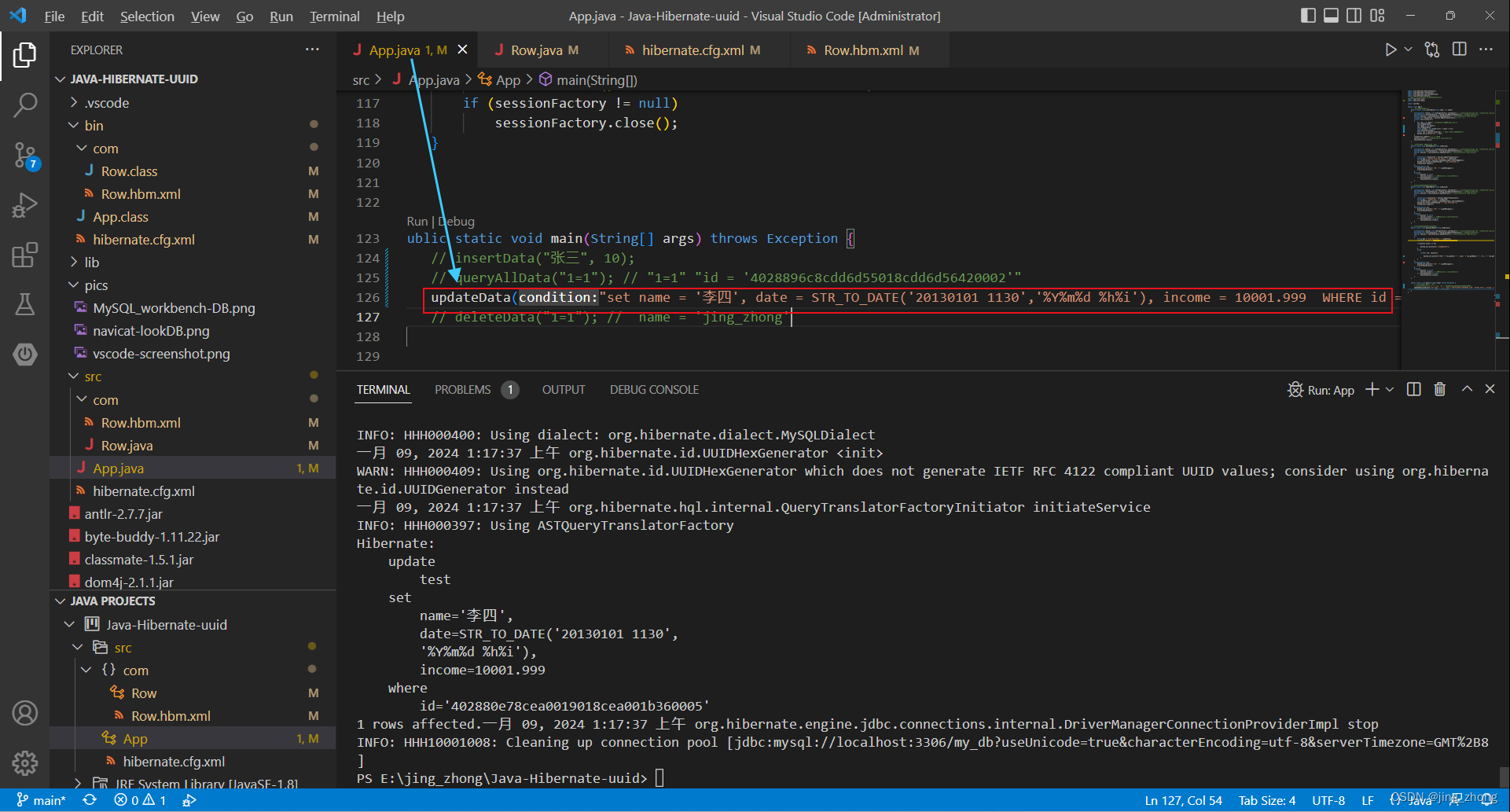
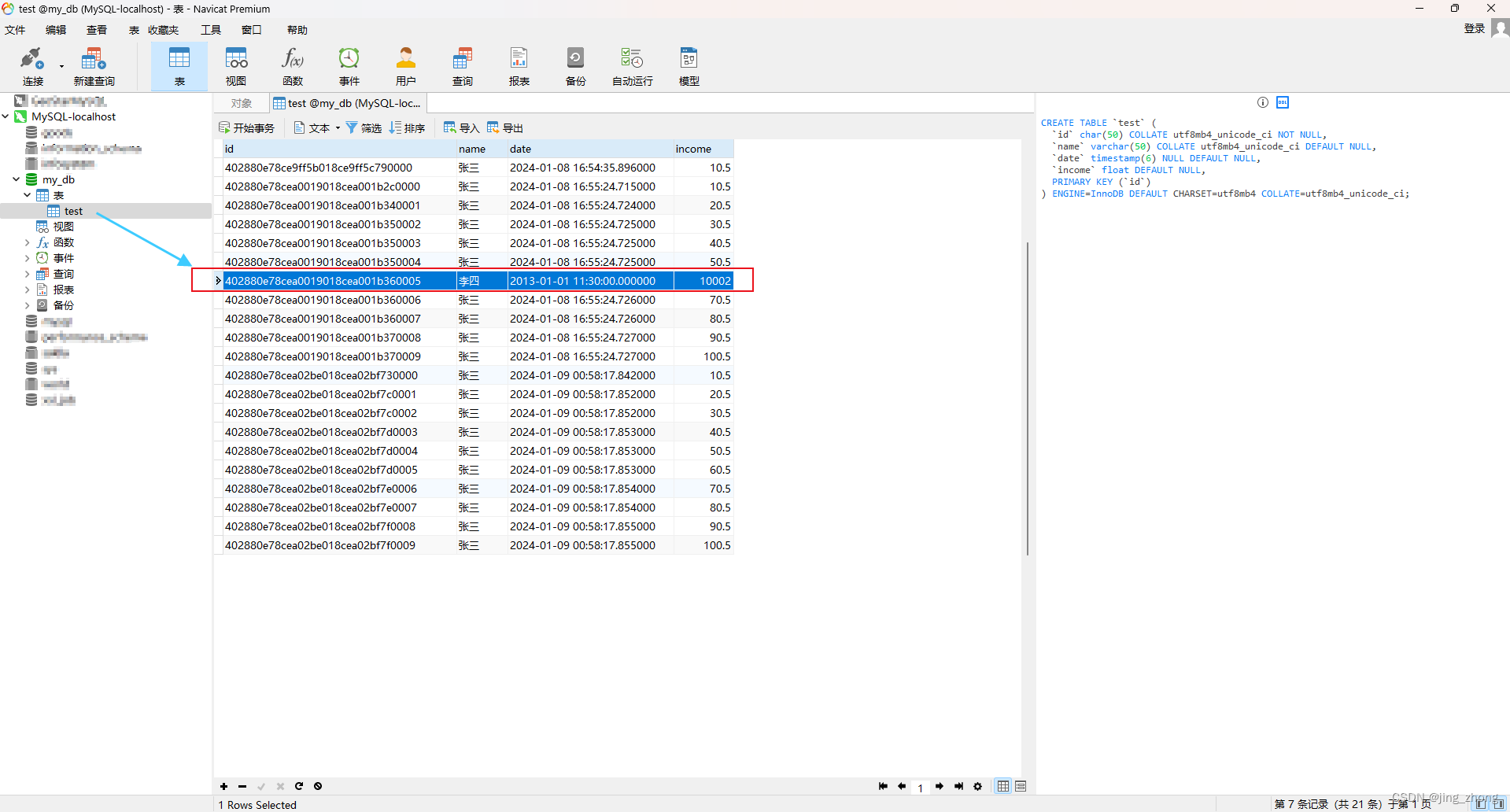
3.4.3 Query
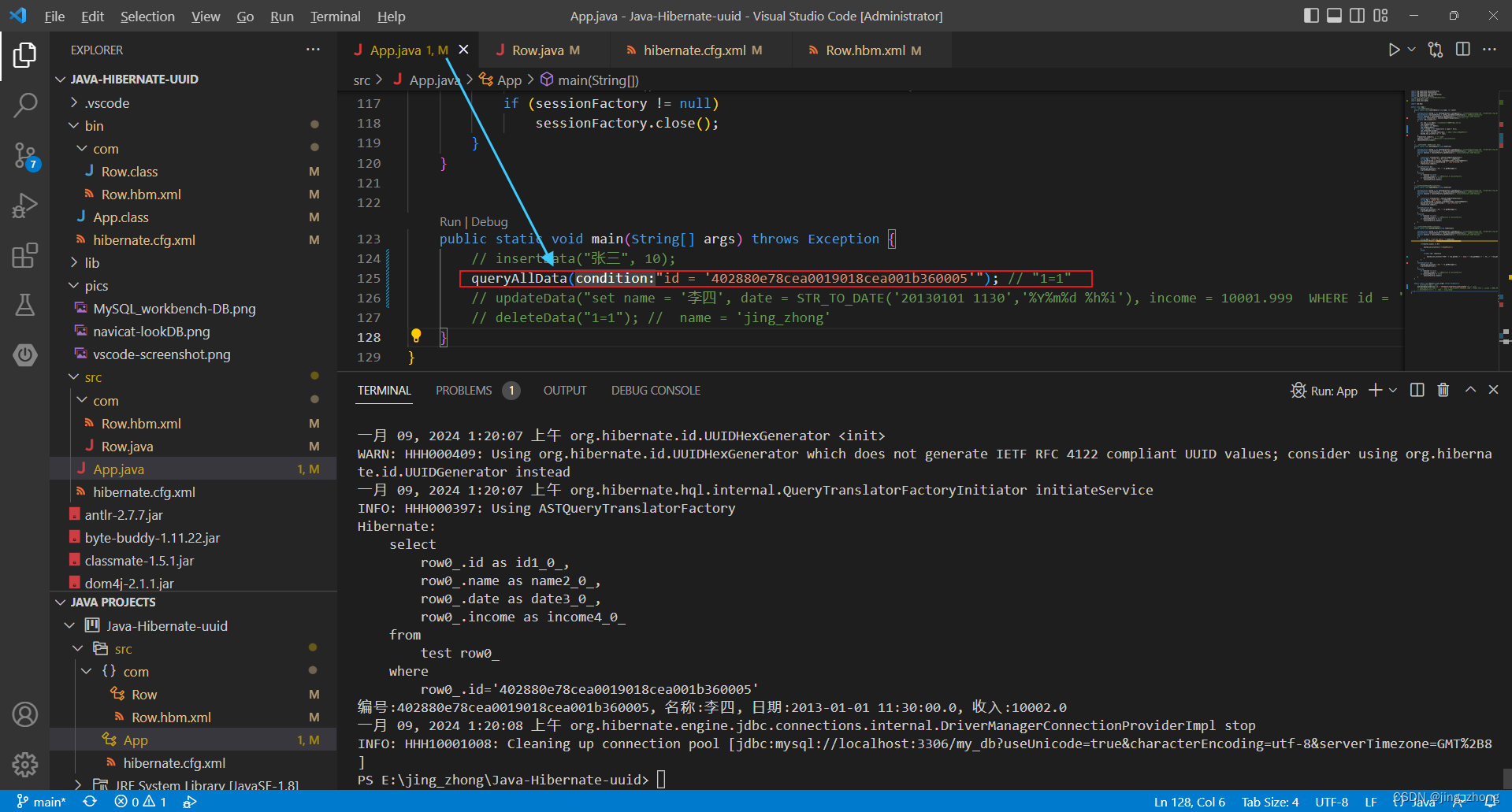
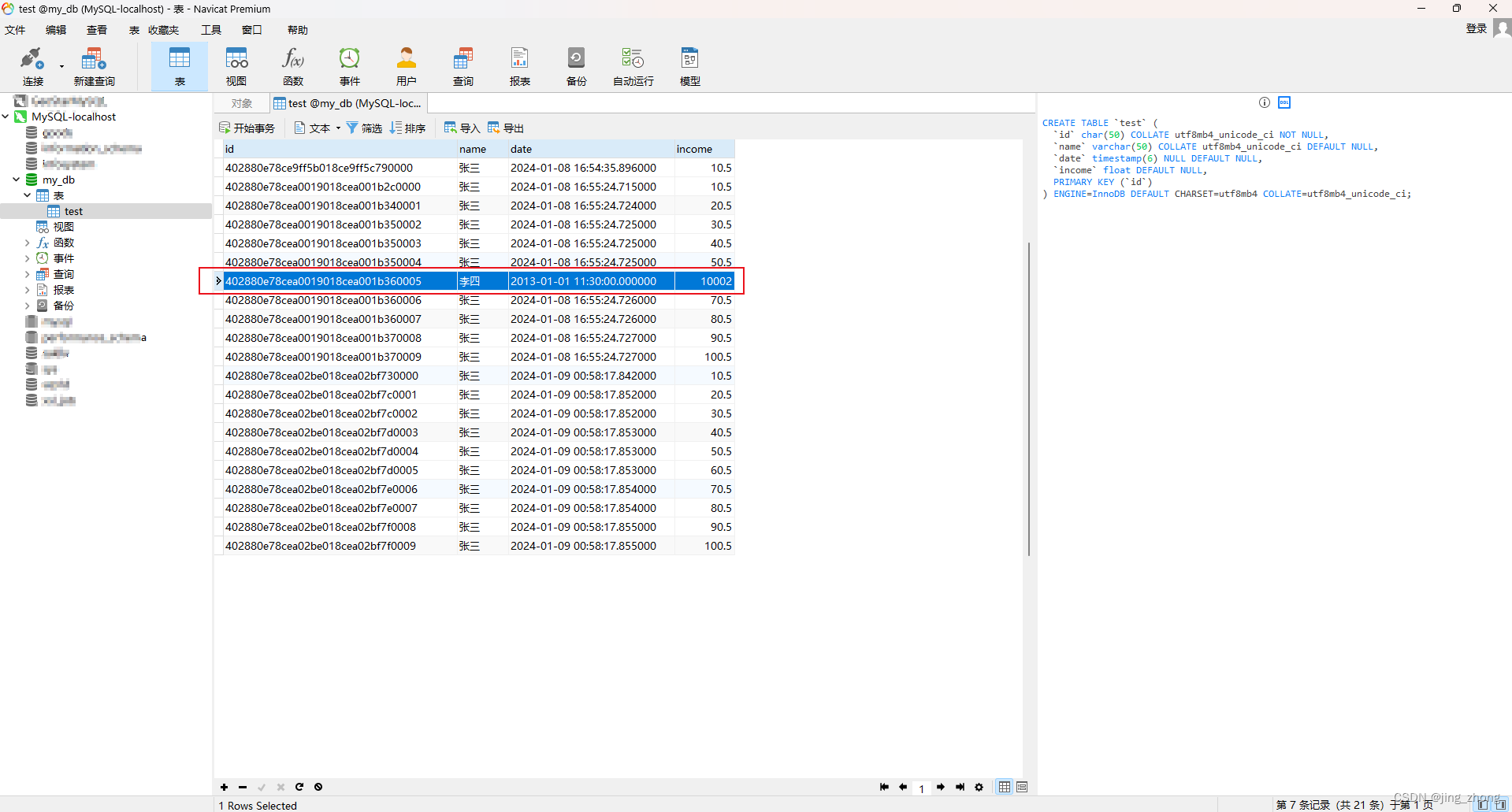
3.4.4 Delete
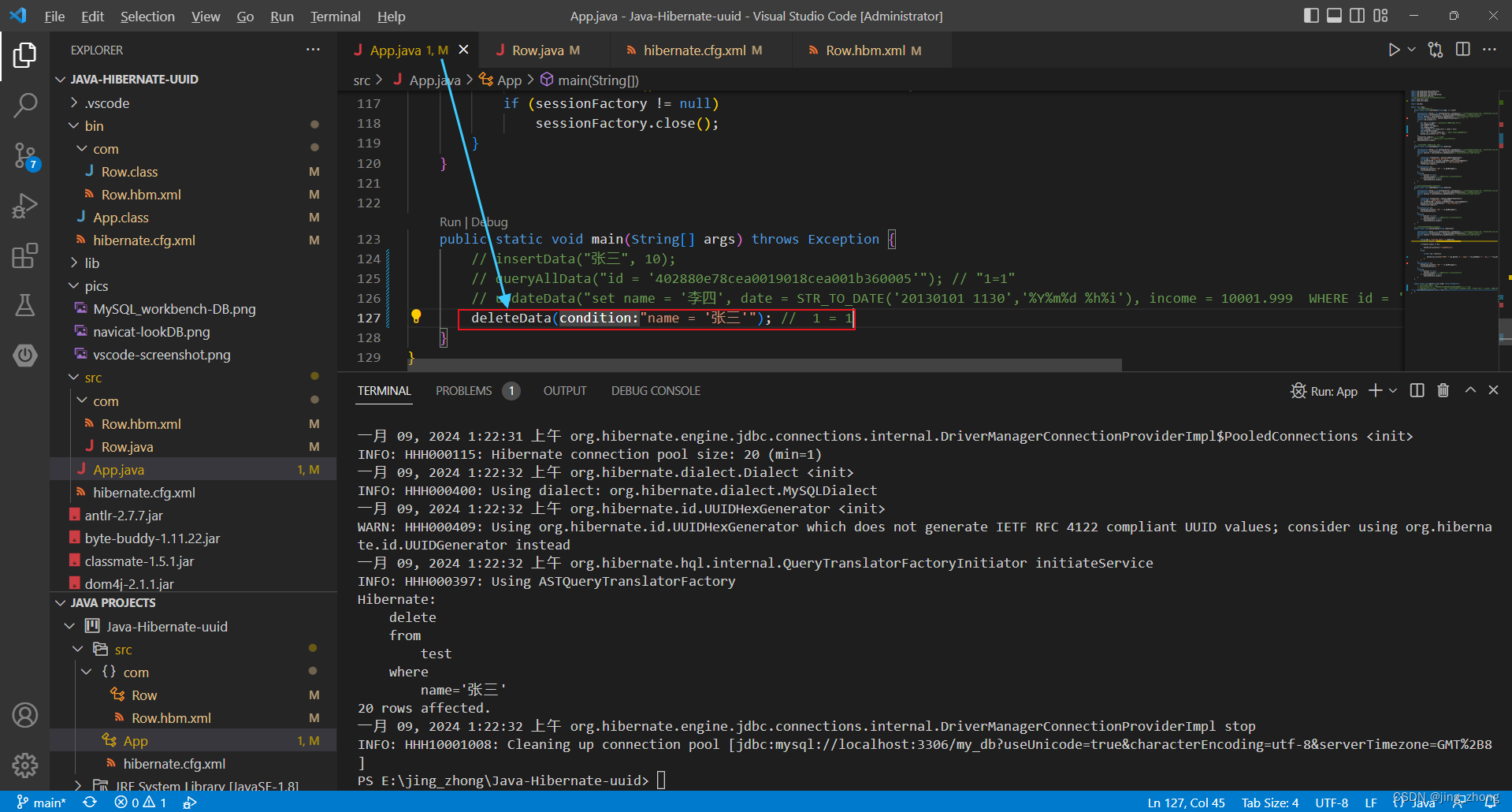
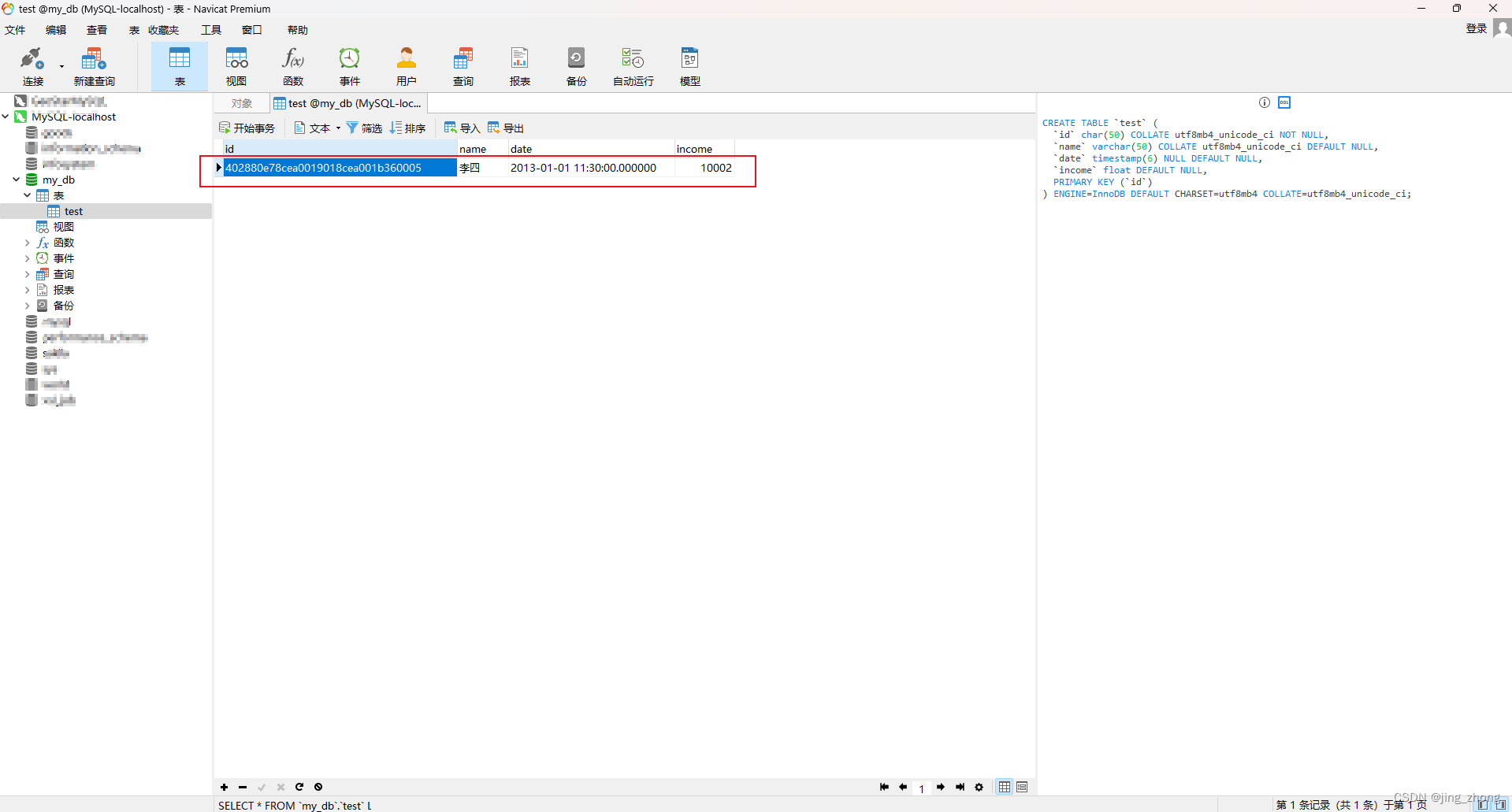
4. Summary
MySQL
As an extremely popular relational database , database has always been praised by developers/engineers for its simplicity and fast advantages . Although there are various databases popular on the market, whether they are open source or commercial databases ,fromdesign conceptandQuery execution speed and efficiencyThere will be more or less differences on the version, and of course 软件、数据库、连接驱动
the versions will be updated, iterated and upgraded accordingly .
In daily study and work, using the database proficiently and learning to use scripting languages such as SQL
or HQL
to interact with the database in a friendly manner is not only a kind of fun, but also the only choice in addition to file resource management and storage. Everyone will benefit a lot and improve efficiency. Everyone is welcome to communicate and discuss new technologies or problems encountered. Thousands of developers work together to solve technical problems, answer questions and help technology upgrades, jointly create a good ecosystem, and embrace Green Future finally hopes that all friends can learn and achieve success .Apply what you learnoh!