1. Introduction - Before learning arrays, when we save a number, we will define a variable:
int a;//save an integer
double b;//save a decimal
char c;//Save a character
......
What should I do if I need to save N integers, decimals, and characters? It's obviously not feasible to save them one by one. At this time, it's time to use the array.
2.one number pair
2.1 Grammar format
Type specifier array name [integer expression];
Type specifier: Specifies the type of array element, any legal type in C language can be used.
Array name: Like variables, arrays also have a name, which conforms to the regulations of C language identifiers.
Integer expression: specifies the number of elements in the array ( The C language standard before stipulates that there cannot be variables in this expression, and the later standard
Variables are allowed to appear in this expression, but cannot be initialized)
int n = 10;
int a[n]; //Yes
int a[n] = {1,2,3....}; //Not possible
For example:
int a[10];//An array is defined with 10 elements, each element is of type int, so 4*10 bytes of memory are allocated
double b[5];//An array is defined with 5 elements, each element is of double type, and 5*8 bytes of memory are allocated here.
......
2.2 The storage order of one-dimensional array elements in memory is to store them in continuous memory address space.
2.3 How to represent an element in an array: subscript method
Array name [subscript]//Subscript: integer range [0,n-1] (n represents the number of array elements)
See picture
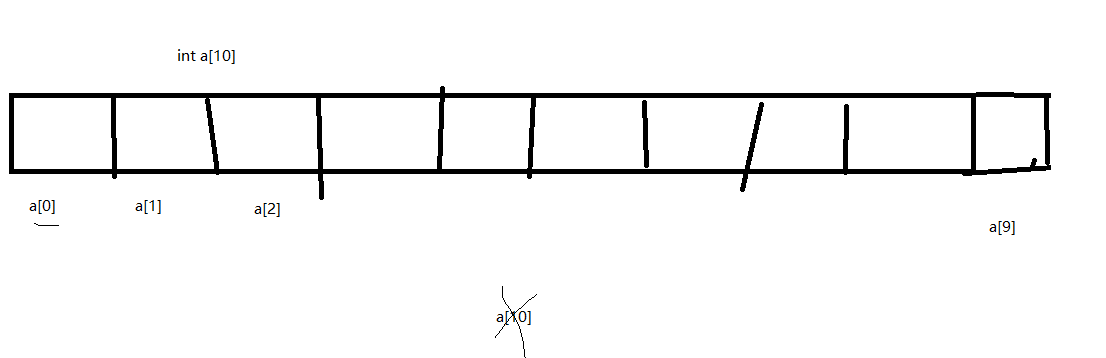
practise:
Define an array int a[10];
Accordingly, enter 10 numbers from the keyboard to assign values to each element of array a.
#include<stdio.h>
int main()
{
int a[10];
int i;
for(i=0;i<10;i++)//验证数组元素存储是连续并且按顺序的
{
printf("%p ",&a[i]);//这里打印输出的是地址(%p)
}
for(i=0;i<10;i++)//依次输入10个整数给数组元素赋值
{
scanf("%d",&a[i]);
}
for(i=0;i<10;i++)//输出数组元素
{
printf("%d ",a[i]);
}
printf("\n");
return 0;
}
2.4 Initialization of one-dimensional array (give initial values to the array elements when defining the array). Use {} to initialize the array.
(1) All array elements are initialized
int a[5] = {10,6,23,100,68};//An array a is defined, with 5 elements, and the values are initialized to 10,6,23,100,68 respectively.
int a[5];
a = {1,2,3,4,5};//Wrong
(2) You can initialize only the first part of the elements
The following elements take default values (the default value of int type is 0, the default value of double type is 0.0, the default value of char type is '\0'..)
int a[10] = {1,2,3,4,5};
a[0] ~ a[4] are initialized to 1,2,3,4,5 respectively, while a[5] ~ a[9] all adopt the default value 0
(3) If all elements are initialized, the number of array elements can be omitted when defining the array.
like:
int a[] = {1,2,3,4,5,6};
You can omit the number of elements. The compiler will infer the number of elements based on the number of values in {}, and 6 will be automatically added in [ ].
(4) Initialization of array of char type
char c[5] = {'a','b','c','d', 39;e'};Because there is no '\0' as the end mark, it does not have a specified length, which will be explained in detail later.
char c[6] = {'a','b','c','d','e' ;}; //c[5] saves '\0'
There is also a concise and special way of writing
char c[6] = "abcde"; //The effect of this initialization is exactly the same as the above effect. What c[5] saves is '\0'
practise:
Define an array with element type int, initialize it, and then find the sum, minimum and maximum value of all elements of the array
The idea of "fighting in the arena" is to first assume that a certain element is the target element, and then compare it with the following elements in turn (fighting in the arena)
int main()
{
int a[10] = {1,2,3,4,5,6,10,21,100,50};
int sum = 0;
int i;
int min = a[0];
int max = a[0];
for(i=0;i<10;i++)
sum += a[i];
for(i=1;i<10;i++)
{
if(a[i] < min)
{
min = a[i];
}
if(a[i] > max)
{
max = a[i];
}
}
printf("数组元素之和:%d\n",sum);
printf("数组最小值为:%d,最大值为:%d\n",min,max);
return 0;
}
practise:
Calculate the sum of the first 15 terms of the Fibonacci sequence, 1 1 2 3 5 8...: The number starting from the third term is the sum of the first two terms.
First find the value of each of the first 15 items, and then add them in a loop.
int a[15] = {1,1};
int i;
for(i=2;i<15;i++)//从第三项开始,等于前两项之和
{
a[i] = a[i-1] + a[i-2];//依次计算 a[2]~a[14]的值
}
int sum = 0;
for(i=0;i<15;i++)
{
sum+=a[i];
}
printf("前15项之和为%d\n",sum);
practise:
Given a one-dimensional array (element type is Int), determine whether the array elements are increasing
If incrementing prints YES otherwise prints NO
int a[10] = {3,4,5....};
递增
a[0] < a[1] < a[2] < a[3] < a[4] < a[5] < a[6] < a[7] < a[8] < a[9]
int flag = 0;
for(i=0;i<9;i++)//注意:防止数组下标越界
{
if(a[i]>=a[i+1])
{
printf("NO\n");
flag = 1;
break;//只要 a[i]>=a[i+1]成立一次,就说明不是递增,打印NO,并且break
}
}
if(flag == 0) //if(i==9)
printf("YES\n");
practise:
Given a one-dimensional array in ascending order, find whether a certain element exists. If it exists, print YES. If it does not exist, print NO.
int a[10] = {5,10,14,20,25,30,37,50,100,200};
int x;
scanf("%d",&x);
int i;
for(i=0;i<10;i++)
{
if(a[i] == x)
{
printf("YES\n");
break;
}
}
if(i==10)
{
printf("NO\n");
}
If there are 1,000,000 elements in the above array, the worst case scenario requires searching 1,000,000 times, which is not efficient. Optimization algorithm:
"Binary Search Method/Half Search Method" (The prerequisite for using this method is ordering)
Idea: Compare with the middle element every time. If it is larger than the middle element (the first half of the data is excluded), it is smaller than the middle element (the last half of the data is excluded), if they are equal, they are found.
#include<stdio.h>
int main()
{
int a[10000];
int i;
for(i=0;i<10000;i++)
{
a[i] = i*2;
}
int x;
scanf("%d",&x);//输入要查找的元素
int begin = 0;
int end = 10000-1;
int mid = (end - begin)/2 + begin; //也可以写为:(end+begin)/2,前面那个方便理解
int count = 0;
while(begin<=end)// begin>end说明范围不存在了,结束循环
{
count ++;
if(x == a[mid])//和中间元素做比较
{
printf("YES,下标为%d\n",mid);
break;
}
else if(x > a[mid])//排除了 mid及前面的所有元素 [mid+1, end]
{
begin = mid+1;
mid = (end - begin)/2+ begin;
}
else//排除了 mid及后面的所有元素 [begin, mid-1]
{
end = mid-1;
mid = (end - begin)/2+ begin;
}
}
if(begin>end)
{
printf("NO\n");
}
printf("一共比较了%d次\n",count);
return 0;
}
There are many other algorithms for sorting, which will be explained in detail in the next chapter.
3,Two-dimensional array: similar to a mathematical matrix, with rows and columns
Definition syntax:
Type specifier array name[row size][column size];
Row size, column size: integer expression (variables could not be written before)
Number of elements: row size * column size
Type specifier: the type of each element
for example:
int a[3][4];
There are 12 elements in 3 rows and 4 columns, each element is of type int
Memory distribution:
Store continuously in order
How to represent an element in an array: subscript method
a[i][j](0<=i<N 0<=j<M where N is the row size and M is the column size)
A two-digit array with three rows and four columns can be represented as follows:
int a[3][4];
a[0][0] a[0][1] a[0][2] a[0][3]
a[1][0] a[1][1] a[1][2] a[1][3]
a[2][0] a[2][1] a[2][2] a[2][3]
practise:
Define a two-dimensional array, the element type is int, and assign values to each element by inputting from the keyboard.
Reprint verification
int a[3][3];
int i,j;
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
scanf("%d",&a[i][j]);
}
}
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
printf("%d ",a[i][j]);
}
printf("\n");
}
Two-dimensional array initialization
use{}
int a[3][4] = {1,2,3,4,5,6,7,8,9,10,11,12};
int a[3][4] = { {1,2,3,4},{5,6,7,8},{9,10,11,12}};
int a[3][4] = {1,2,3,4,5,6,7,8,9,10};//a[2][2] = 0 a[2][3] = 0
int a[3][4] = { {1,2,3},{5,6},{9,10,11}};//a[0][3]=0 a[1][2]=0 a[1][3]=0 a[2][3]=0
//In some cases, the row size can be omitted and automatically derived based on the number of subsequent initial values. Column size can never be omitted
int a[][4] = {1,2,3,4,5,6,7,8,9,10};
practise:
Define and initialize a two-dimensional array with element type int. Find the number of "mountain top elements"
"Mountaintop element": larger than the surrounding elements (up, down, left, right)
10 20 80
9 90 70
30 10 8
80, 90, and 30 are all mountain top elements
#include<stdio.h>
int main()
{
int a[3][3] = {
10, 20 ,80 ,
9 , 90 ,70 ,
30, 10 ,8
};
int count = 0;
int i,j;
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
if((i==0 || a[i][j] > a[i-1][j]) //利用惰性运算
&& (i==2 || a[i][j] > a[i+1][j])
&& (j==0 || a[i][j] > a[i][j-1])
&& (j==2 || a[i][j] > a[i][j+1])
)
{
count++;
}
}
}
printf("count=%d\n",count);
return 0;
}
practise:
Print the first 10 lines of Yang Hui's triangle
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 1
.........
#include<stdio.h>
int main()
{
int a[15][15] = {0};
int i,j;
for(i=0;i<15;i++)
{
a[i][0] = 1;//第一列所有元素都为1
a[i][i] = 1;//斜对角所有元素都为1
}
for(i=2;i<15;i++)//行
{
for(j=1;j<i;j++)
{
a[i][j] = a[i-1][j] + a[i-1][j-1];
}
}
for(i=0;i<15;i++)
{
for(j=0;j<=i;j++)
{
printf("%4d ",a[i][j]);
}
printf("\n");
}
return 0;
}
5,Character number combination
The array element type is char, and the basic syntax also satisfies all the syntax of arrays mentioned earlier.
for example:
char c[5] = {'a','b','c','d','e'};
Access element subscript method: c[0] c[1] ...c[4]
There are some differences from other types of arrays. like:
Initialization can be done like this:
char c[6] = "abcde";//The last element is automatically complemented by the system '\0'
In C language, a character array can be used to represent a string. String: a set of characters ending with the '\0' character.
String length: the number of characters before '\0' (excluding '\0' itself, which is the terminator of the string)
char c[6] = "abcde"; //Array c has 6 elements, and the length of string c is 5
char c[5] = {'a','b','c','d','e' ;};//Array c has 5 elements, and the length of string c is uncertain.
char c[] = "abcde";//Array c has 6 elements, followed by a '\0', and the length of string c is 5
char c[] = {'a','b','c','d','e' };//Array c has 5 elements, and the length of string c is uncertain
char c[6] = {'a','b','c','d','e' ;};//Array c has 6 elements, c[5] defaults to '\0', and the length of string c is 5
char c[5]={“abcde”}; “abcde” occupies 6 bytes, which exceeds the length of c
When you want to use a character array as a string, you must specify its length. Traversing character arrays has one more method than traversing arrays of other types when specifying their length.
int a[10] = {1,2,3,4,5,6,7,8,9,10};
for(i=0;i<10;i++)
{
printf("%d ",a[i]);
}
char c[6] = "abcde";
for(i=0;i<5;i++)//You can use loop statements to traverse array elements like other arrays
{
printf("%c",c[i]);
}
You can also use %s to traverse it directly, such as:
printf("%s",c);//When using %s to print, you must specify its length, otherwise problems will occur
//Because when %s prints, it starts from the first element and ends when it encounters '\0'; it does not care whether it crosses the boundary or not.
practise:
Use a character array to save a string, and then change the lowercase letters in the string to uppercase, and the uppercase letters to lowercase
char c[] = "abc12XYz";
int i;
for(i=0;i<strlen(c);i++)
{
if(c[i]>='a' && c[i]<='z')
c[i] -= 32;
else if(c[i]>='A' && c[i]<='Z')
c[i] += 32;
}
-------------------
int i=0;
while(c[i] != '\0')
{
if(c[i]>='a' && c[i]<='z')
c[i] -= 32;
else if(c[i]>='A' && c[i]<='Z')
c[i] += 32;
i++;
}