Leading AI research lab OpenAI has created a powerful API that allows developers to take advantage of cutting-edge AI capabilities and generate language models using GPT3 and more. In this tutorial, we'll show you how to use the OpenAI API with Axios and JavaScript, giving you a glimpse into the future of AI-powered web development.
Axios is a popular JavaScript library for making HTTP requests and integrating with APIs, making it the perfect tool for accessing the OpenAI API. What to do if your laptop has no soundWhether you are an experienced developer or just starting out, this guide will walk you through how to use the OpenAI API with Axios and JavaScript work together so you can start creating innovative AI-powered applications today.
You can find more information about the Axios library at:https://axios-http.com/: a>
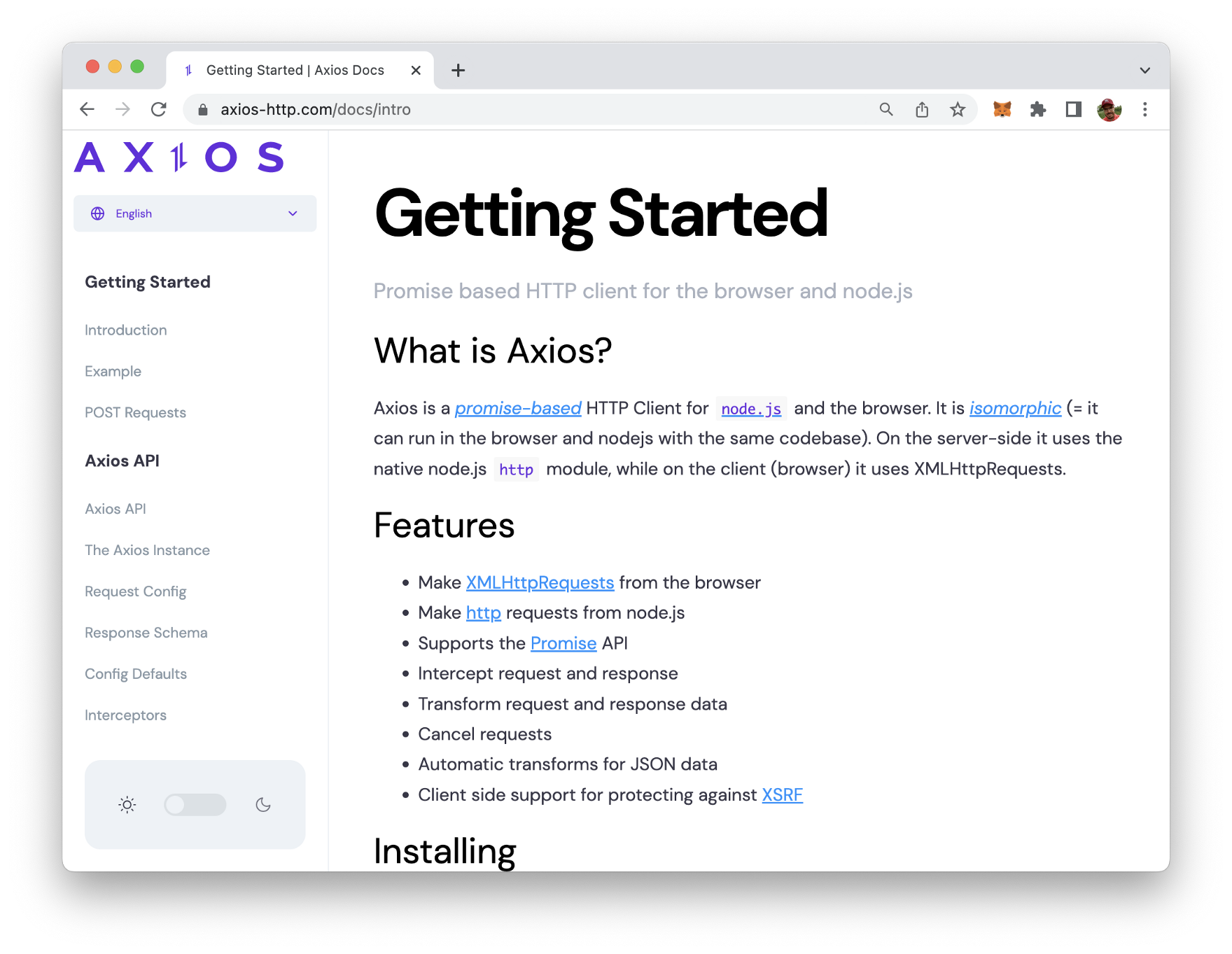
Retrieve OpenAI API key
To obtain an API key for the OpenAI API, you need to register one on the OpenAI website (https://openai.com/) Free OpenAI account:
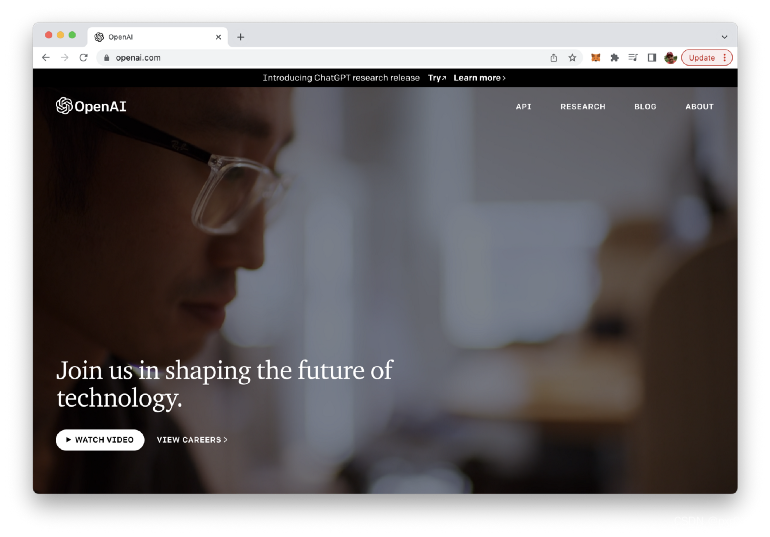
To register for an account, click the "Register" button in the upper right corner of the home page and follow the prompts to create a new account. After you create your account, you can obtain your API key by visiting the API section of your account settings.
In the "API" section, you'll find a button labeled "Generate API Key." Click this button to generate a new API key, which you can then use to make requests to the OpenAI API from your web application. Please keep your API key safe and do not share it with anyone else.
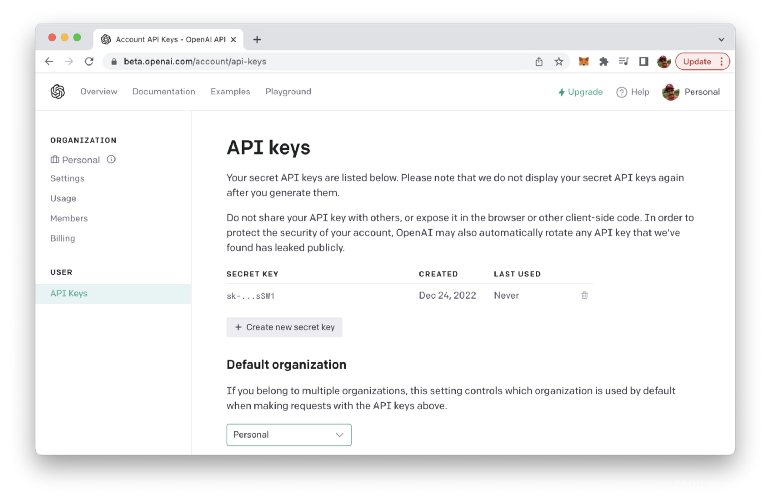
Setup items
Let's set up the JavaScript project by first creating a new project folder:
$ mkdir openai-axios
$ cd openai-axios
In the new project folder, create a new initial package.json file using the Node.js Package Manager (NPM). This is a prerequisite so that we can install more dependencies using NPM:
$ npm init -y
When you run this command, you should be able to see what is written to package.json on the console:
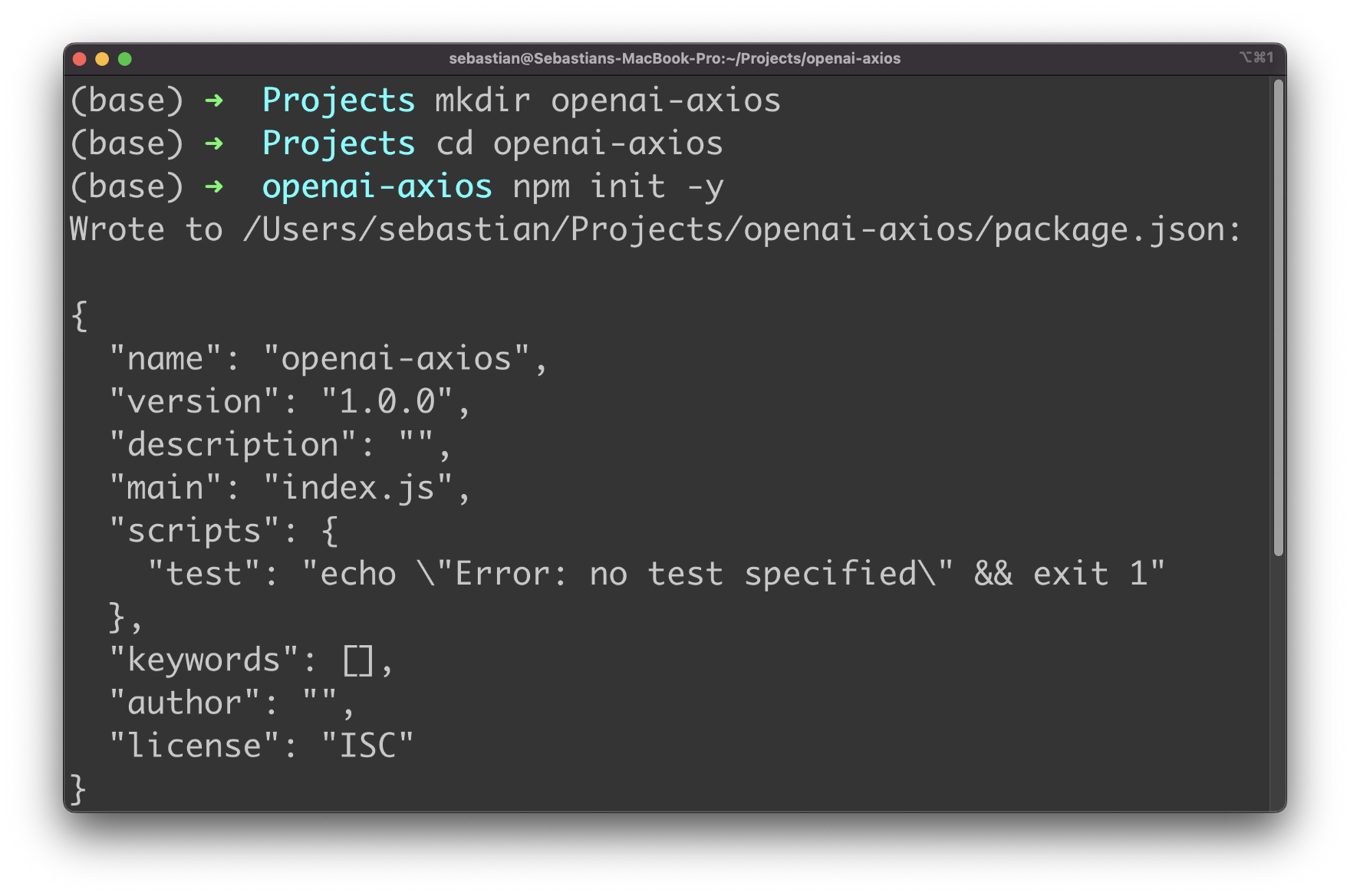
Next, use NPM to install the packages dotenv and axios.
$ npm install dotenv axios
Dotenv is a zero-dependency module that loads environment variables from .env files into process.env. We will use an environment variable to store the API key we retrieve from the OpenAI dashboard.
Next we need to create two files in the project folder:
$ touch index.js .env
Access the OpenAI API using Axios
First let's create an environment variable in .env:
OPENAI_API_KEY=[INSERT YOUR OPENAI API KEY HERE]
Next switch to the file index.js and start with the following lines of code:
const axios = require("axios");
This require statement is required to include the Axios library. Another require statement is needed to activate the dotenv library and make environment variables accessible through process.env.
const apiKey = process.env.OPENAI_API_KEY;
Next we create an Axios client instance using the axios.create method. At the same time, we set an authorization header containing the OpenAI API key:
const client = axios.create({
headers: {
Authorization: "Bearer " + apiKey,
},
});
Let's use the Axios client object to send an HTTP POST request to one of the OpenAI API endpoints:
const params = {
prompt: "How are you?",
model: "text-davinci-003",
max_tokens: 10,
temperature: 0,
};
client
.post("https://api.openai.com/v1/completions", params)
.then((result) => {
console.log(result.data.choices[0].text);
})
.catch((err) => {
console.log(err);
});
This code uses the Axios library to send an HTTP POST request to the OpenAI API. Make a request to the endpoint "https://api.openai.com/v1/completions". This endpoint of the OpenAI API allows developers to generate text completion for a given prompt.
The params object is a JavaScript object that contains the parameters of the API request, including the prompt to complete, the model to use, the maximum number of tokens to generate, and the temperature to use when generating.
The request is made using the .post() method of the client object. The .post() method takes two parameters: the URL endpoint and the parameter object.
The response to the request is handled using the Promise .then() method, which is called when the request is successful. The result object returned by the API is logged to the console along with the text of the first selection in the response data's selection array.
If an error occurs, the Promise .catch() method will be called. It logs error messages to the console, allowing developers to diagnose any issues with API requests.
The following listing contains the complete source code for index.js:
const axios = require("axios");
require("dotenv").config();
const apiKey = process.env.OPENAI_API_KEY;
const client = axios.create({
headers: {
Authorization: "Bearer " + apiKey,
},
});
const params = {
prompt: "How are you?",
model: "text-davinci-003",
max_tokens: 10,
temperature: 0,
};
client
.post("https://api.openai.com/v1/completions", params)
.then((result) => {
console.log(result.data.choices[0].text);
})
.catch((err) => {
console.log(err);
});
Let's run the application using the node command:
$ node index.js
As output, you should be able to see the response generated and returned by OpenAI:
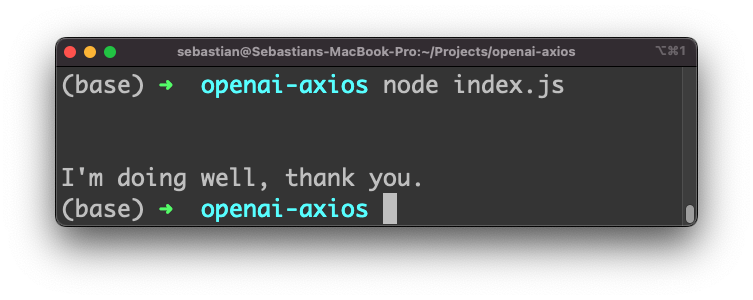
in conclusion
This tutorial provides a comprehensive guide on how to get started using the OpenAI API and Axios in JavaScript, from setting up your environment to making your first API call. We hope this guide helps show you how to harness the power of AI and drive innovation in your projects.