int main()
{
int a[5] = { 1, 2, 3, 4, 5 };
int *ptr = (int *)(&a + 1);
printf( "%d,%d", *(a + 1), *(ptr - 1));
return 0;
}
We first need to know here(&a + 1) What is - &a is to take out the address of the entire array. Originally, ptr pointed to the address of 1, and then gave it +1 It means skipping this array, that is
(a+1) where a represents the address of the first element, +1 represents the address of the second element, so *(*() is the second element, which is 2; 1+ a
*(ptr-1) is the address pointing to 5, and then dereferenced it is 5; so the answer to this question is 2, 5;
2
int main()
{
int a[4] = { 1, 2, 3, 4 };
int *ptr1 = (int *)(&a + 1);
int *ptr2 = (int *)((int)a + 1);
printf( "%x,%x", ptr1[-1], *ptr2);
return 0;
}

#include <stdio.h>
int main()
{
int a[3][2] = { (0, 1), (2, 3), (4, 5) };
int *p;
p = a[0];
printf( "%d", p[0]);
return 0;
}
a is a two-dimensional array of three rows and two column pairs. Pay attention to the initialization of the two-dimensional array here, int a] < /span>) };, among them is Separated by ",", not "{}", so he only initialized 3 elements, namely 1, 3, 5, and the rest are all 0, that is, this two-dimensional array should be5, 4), (3, 2), (1 , 0 { (=2][3[
p = a[0 ];a[0]indication is &a[0][0], p[0]indication*(p+0)indication*pindication1;
4
int main()
{
int a[5][5];
int(*p)[4];
p = a;
printf( "%p,%d\n", &p[4][2] - &a[4][2], &p[4][2] - &a[4][2]);
return 0;
}
a is of type int(*)[5], p is of type int(*)[4], as shown in the figure
FFFFFFFC和-4
5
int main()
{
int aa[2][5] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int *ptr1 = (int *)(&aa + 1);
int *ptr2 = (int *)(*(aa + 1));
printf( "%d,%d", *(ptr1 - 1), *(ptr2 - 1));
return 0;
}
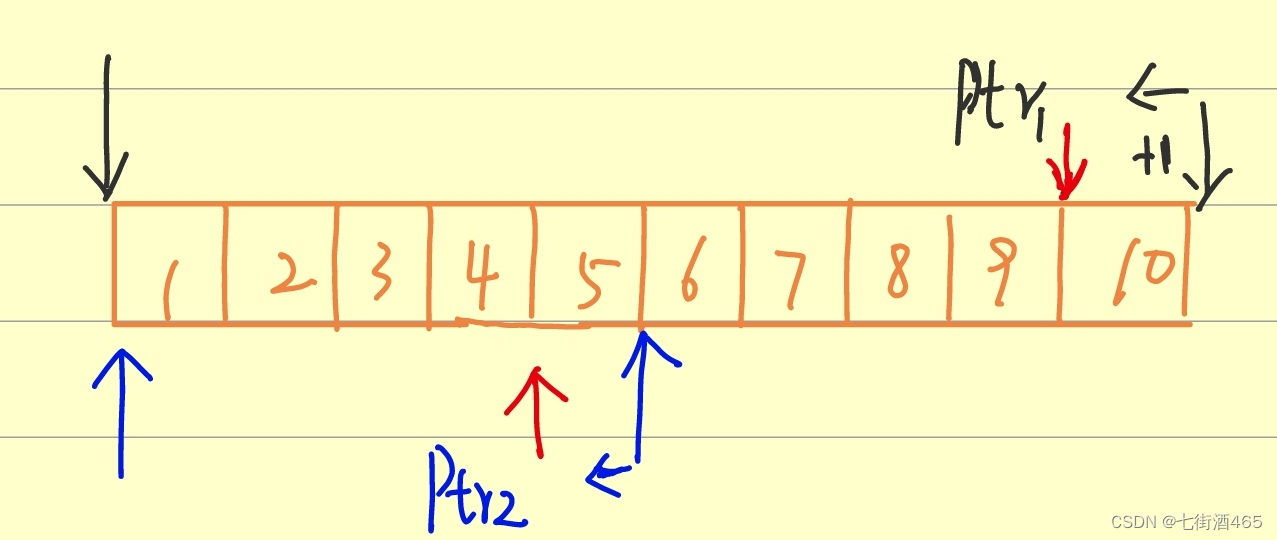