1. Inheritance (extends)
1.1 The concept of inheritance
Inheritance is a basic feature of object-oriented, which allows subclasses to inherit the characteristics and behaviors of parent classes to improve code reuse and maintainability. The following picture vividly demonstrates the relationship between inheritance and classes:
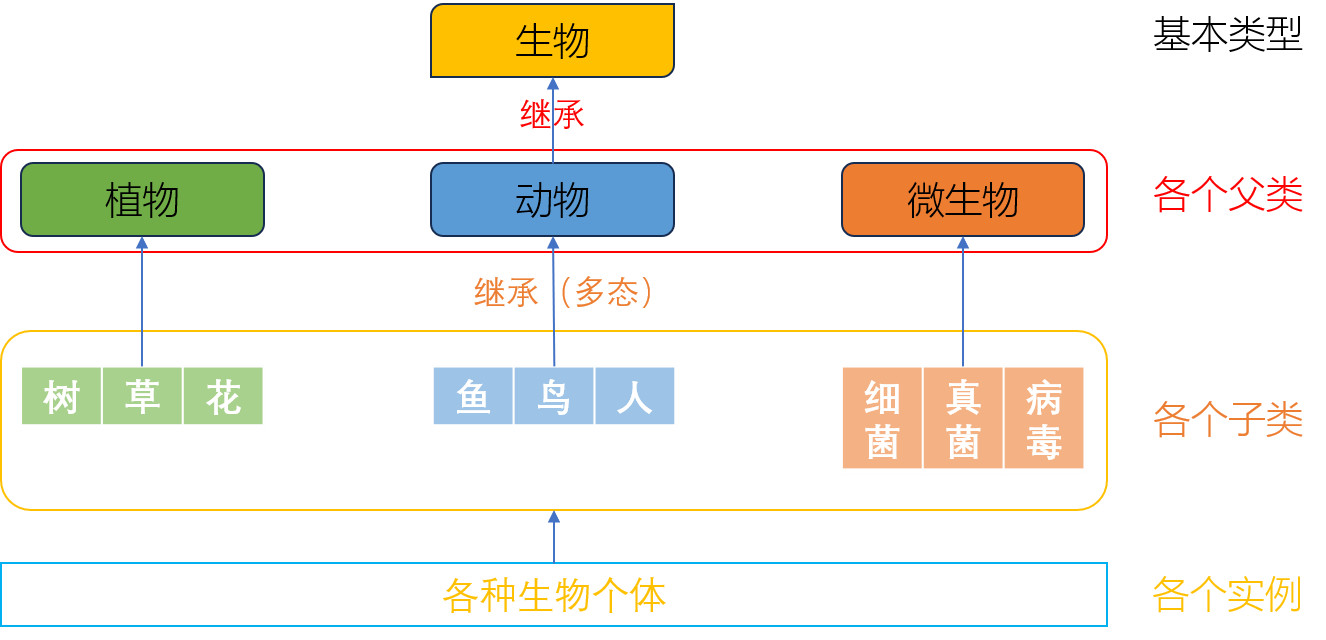
In the picture above, "animals", "herbivores", "carnivores" and various specific animal types are equivalent to classes in Java, and the "father-son" relationship between them is inheritance. Each specific The actual individuals of animal types are objects, or instances of classes.
Inheritance in Java is declared through the extends keyword:
class Father {}
class Son extends Father {}
1.2 Inheritance model
Generally speaking, object-oriented programming languages should have four inheritance patterns, but there are only three inheritance patterns in Java: single inheritance, multiple inheritance, and polymorphism. Some object-oriented languages also have an inheritance pattern of multiple inheritance, such as Python. The following picture vividly demonstrates the differences between the four inheritance modes.
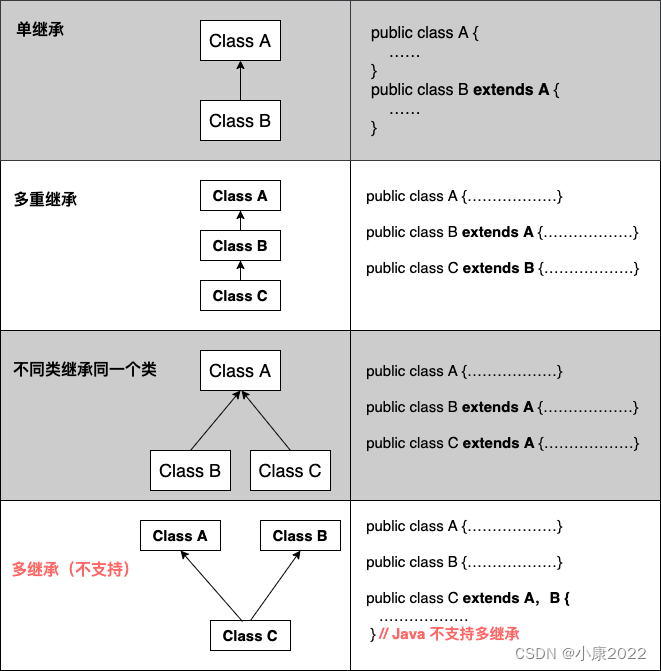
In the above figure, "different classes inherit the same class" refers to polymorphism.
1.3 this keyword
The this keyword literally means this. In a class, it represents the instance object of this class itself (not the class itself). This is set to facilitate calling some properties and methods of the object. Regarding the origin of the name this, actually this is borrowed from C++. The this keyword in C++ object-oriented and the this keyword in Java have almost the same meaning. Other object-oriented programming languages have similar ones, but not all of them are keywords. For example, in Python, a special parameter with a conventional name of self has a similar function.
We can access the class itself via the this keyword:
class Son {
Integer age = 6;
void printAge (){
System.out.println(this.age); // Output: 6
}
}
1.4 super keyword
Since there is this to represent itself, there is a keyword that represents its parent class, which is super. The super keyword and this are slightly different in scope definition. this represents an instance, while super represents a class and is the parent class of the class, but it does not refer to a specific parent class, but all its parent classes. A kind of combination.
For example, GrandSon -> Son -> Father, then for GrandSon, this represents the instance object of GrandSon, and super represents a combination of Son and Father. You can think of super as a special class that contains properties and methods of both Son and Father.
Regarding the origin of the super keyword name, in fact, the more standard term for parent class is superclass, but the "father-child" relationship makes it easier to explain the inheritance relationship, so we generally refer to superclasses as parent classes, but in fact, super It is the meaning of super, which means a super class, that is, the collection of all parent classes of subclasses. In other programming languages, super is almost always used to express it.
We can access the parent class through the super keyword:
class Father {
Integer age = 66;
}
class Son extends Father {
Integer age = 6;
void printAge (){
System.out.println(this.age); // Output: 6
System.out.println(super.age); // Output: 66
}
}
1.5 final keyword
The final keyword has been detailed in other articles and will not be repeated here. For details, please see:Keywords final and static in Java-CSDN Blog
1.6 Polymorphism
Polymorphism is the ability of the same behavior to have multiple different manifestations or forms. To put it simply, it is "multiple forms". When a method has multiple different implementations, or when an abstract class/interface has multiple different implementation classes, it is polymorphism. To give an example close to daily life, a pen (abstract type), we do not specify its specific type, can be used to write (abstract method), we do not specify its specific writing effect, then there are many things that belong to this The classification of pens, such as different colors of black pens, red pens, blue pens, etc., achieve different color effects and have multiple forms, then this is polymorphic. What I mentioned above is discussed from the perspective of method, but it can also be considered from the perspective of type. For example, brushes, pencils, pens, etc. are all pens, but they are not exactly the same.
There are many benefits to using polymorphism:
- Eliminate coupling relationships between types;
- replaceability;
- Scalability;
- flexibility;
For an object-oriented program to have a good structure, polymorphism is almost essential. So, how is polymorphism implemented in Java?
There are generally three ways to implement polymorphism in Java:
- Rewriting:By rewriting methods in subclasses to achieve the same method name but different functional effects, polymorphism is achieved;
- Interface:An interface with multiple different implementations is polymorphic;
- Abstract classes and abstract methods:Similar to interfaces, an abstract class or abstract method can have multiple different implementations after inheritance by multiple different subclasses, which can also be implemented Polymorphism;
2. Implementations
Implementation is different from inheritance. Inheritance is relative to classes, but implementation is relative to interfaces. There are one more ways of implementation than inheritance, that is, implementation can be "multi-implemented." All object-oriented languages have the concept of inheritance, but not all object-oriented languages have the concept of implementation, because only some languages have real interfaces, such as Java and C#.
Implementations are declared via the implements keyword:
interface Father1 {}
interface Father2 {}
class Son implements Father1, Father2 {}
Interfaces can be implemented by classes, but interfaces themselves cannot be implemented by interfaces. In other words, a class can either inherit a class or implement an interface, but an interface can only inherit an interface. The specific situation is as follows:
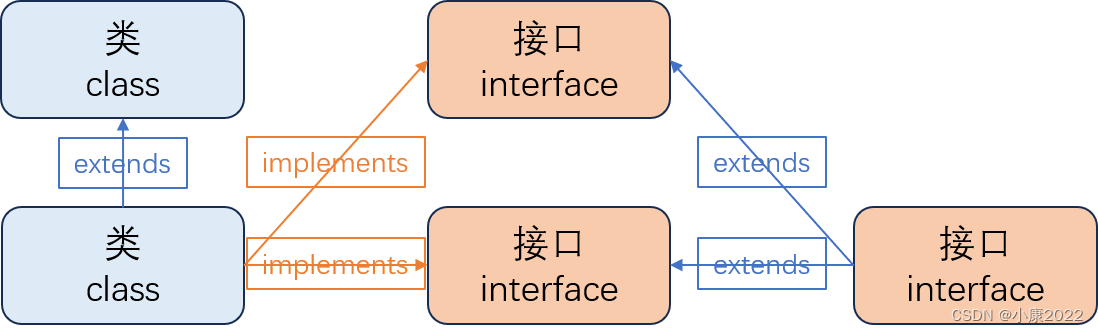
In the picture above, multiple lines indicate that "multiple inheritance" is possible, but it is not true multiple inheritance. See the following examples for details:
interface interface1 {}
interface interface2 {}
interface interface3 extends interface1, interface2 {} // 多“继承”
class class1 {}
class class2 extends class1 {} // 继承
class class3 implements interface1, interface2 {} // 多实现