Process Basics (1)
A process refers to a running program, as shown in the figure below, which is the smallest unit of resource allocation. You can view running processes through commands such as "ps" or "top". Threads are the smallest scheduling unit of the system. A process can have multiple threads. , threads in the same process can share the same resources of this process.
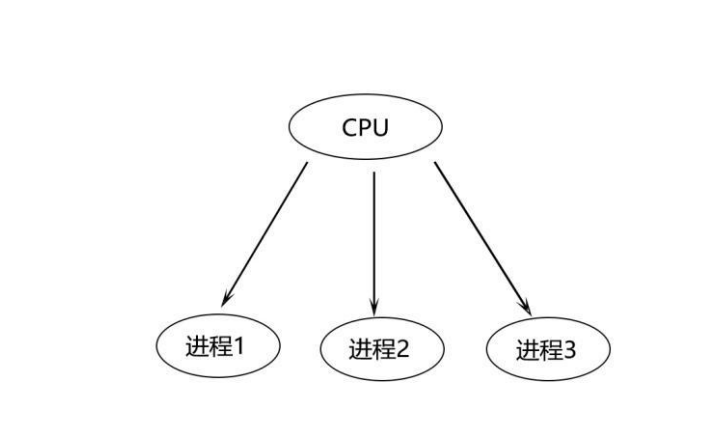
Each process has a unique identifier, the process ID, or pid for short. What are the methods of inter-process communication?
Pipe communication: named pipes, unnamed pipes.
Signal communication: signal sending, signal receiving, signal processing.
IPC communication: shared memory, message queue, semaphore.
Socket communication.
The three basic states and transitions of the process:
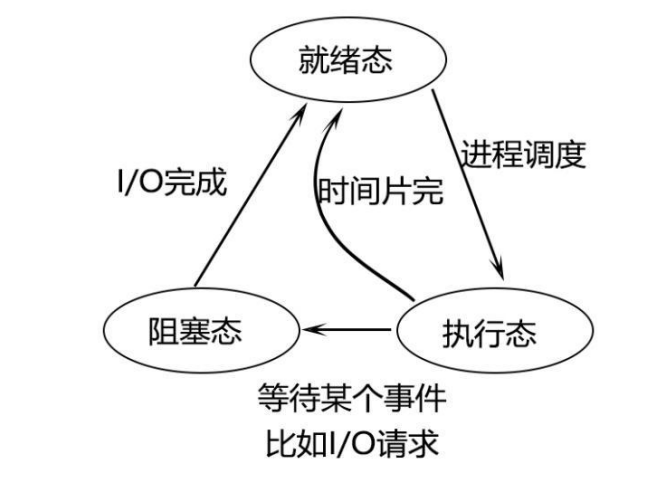
Process creation
All processes are created by other processes (except the idle process with pid number 0). The init process with pid number 1 is the first process that runs after the system starts. It is the parent process of all processes. The init process will initialize part of System services, create other processes.
The process that creates a new process is called the parent process, and the new process is called the child process. The parent process and the child process have the same code segment and data segment, and have independent address spaces. Using copy-on-write technology, that is, the new process created will not immediately copy the resource space of the parent process, but will only copy the resources when they are modified. In addition, the signals and file locks suspended by the parent process will not be inherited by the child process.
After the child process ends, its parent process must reclaim its resources, otherwise it will become a zombie process.
If the parent process ends first, the child process will be adopted by the init process and is called an orphan process.
Commonly used functions for creating processes are defined as follows:
head File |
#include <sys/types.h> #include <unistd.h> |
function |
pid_t getpid(void); |
return value |
PID number |
Function |
Get the PID of this process |
head File |
#include <sys/types.h> #include <unistd.h> |
function |
pid_t getppid(void); |
return value |
PID number |
Function |
Get parent process PID |
head File |
#include <unistd.h> |
function |
pid_t fork(void); |
return value |
If the call is successful, the parent process returns the child process number, the child process returns 0, and if the call fails, it returns -1. |
Function |
System call to create a process |
// 在父进程中创建子进程
#include <stdio.h>
#include <unistd.h>
int main(void)
{
pid_t pid;
pid = fork();
if (pid < 0)
{
printf("fork is error \n");
return -1;
}
//父进程
if (pid > 0)
{
printf("This is parent,parent pid is %d\n", getpid());
}
//子进程
if (pid == 0)
{
printf("This is child,child pid is %d,parent pid is %d\n", getpid(), getppid());
}
return 0;
}
The running results are as follows:
