1. Mission Overview
Use UnitTest and Selenium to write automated use cases to test the integer single addition function [such as 1+2=3] inonline adder
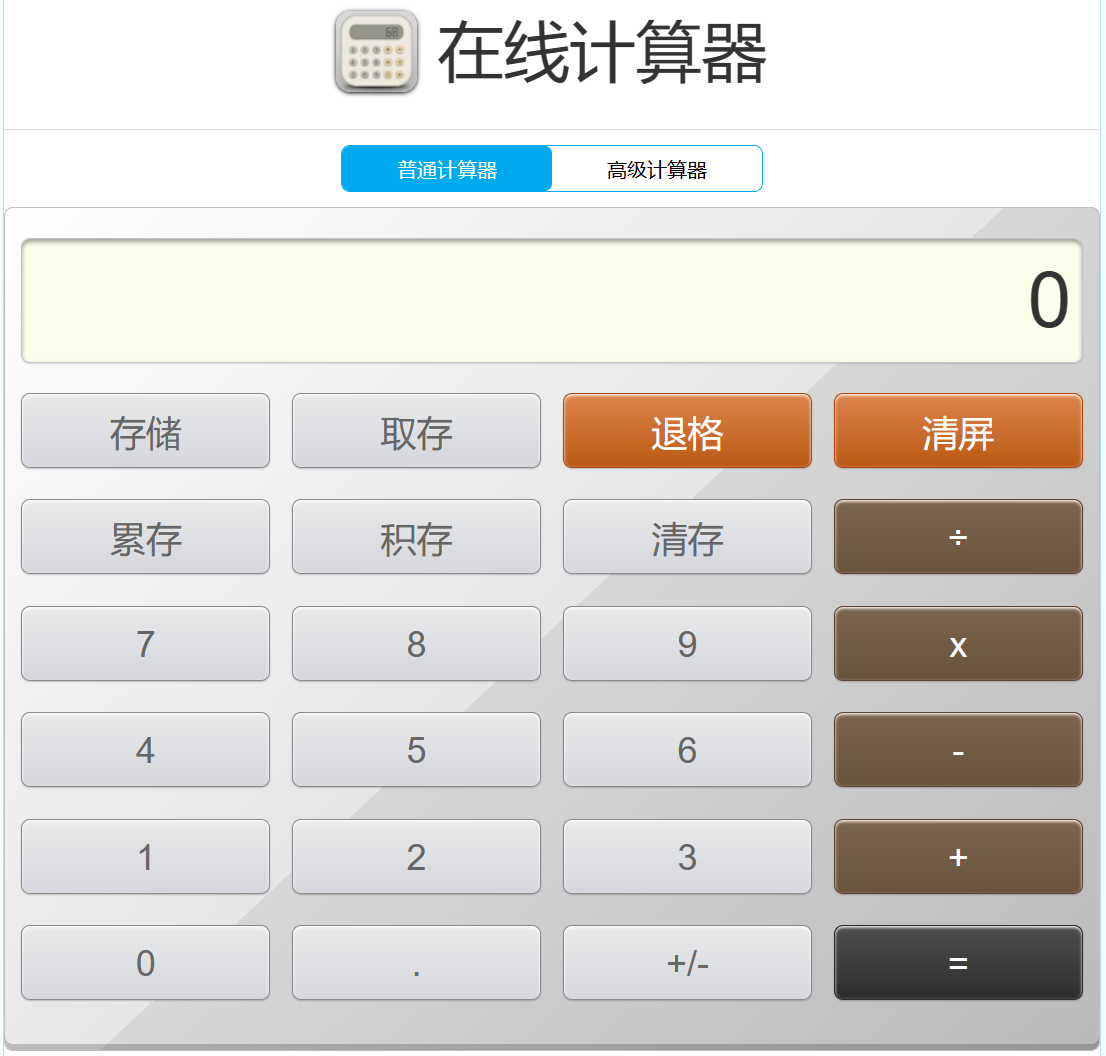
Manual operation process (test whether 1+2 equals 3):
- OpenOnline Adder
- Click the button
1
, then click the button+
, then click the button2
, then click the button again=
- Observe whether the calculation result of the computer frame is equal to
3
. If an error occurs, keep the screenshot, otherwise the test passes - Close browser
Automated test writing process:
-
Use selenium to instantiate the browser, openonline adder and maximize the window
-
Use CSS selectors to target the button
清屏
and click [This is for insurance]Use CSS selector to target button
1
, clickUse CSS selector to target button
+
, clickUse CSS selector to target button
2
, clickUse CSS selector to target button
=
, click -
Use the CSS selector to locate the result box, obtain the value of this component
value
and compare it with the expected result3
. If an error occurs during the process, screenshots are retained. -
Close browser
2.PO mode
Page Objece, a layered mechanism, allows different layers to do different types of things, making the code structure clear and increasing reusability.
- Two layers: object logic layer + business data layer
- Three layers: object library layer + logic layer + business data layer [used in this case]
- Four layers: object library layer + logic layer + business layer + data layer
base layer
Encapsulate the most basic methods, such as click, get value, browser instantiation and other operations, and achieve reuse by being inherited.
page layer
Define some configuration data, such as the online calculator URL, the positioning method of each button, etc., and obtain some basic methods by inheriting the Base class, and further improve the processing logic on this basis
scripts layer
Write a test class to improve the business processing process. The script calls the page and sets an assertion [that is, whether the result is correct]. When an error occurs, take a screenshot and store it in the image layer.
3. Source code details
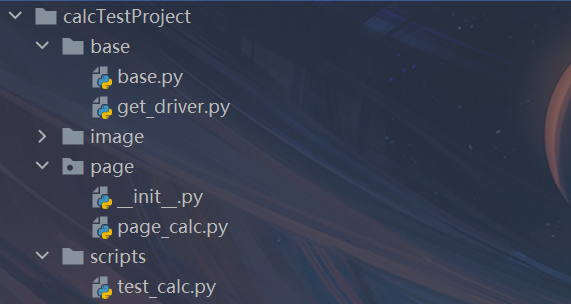
base layer
base.py
import time
from selenium.webdriver.support.wait import WebDriverWait
class Base:
# 初始化方法
def __init__(self,driver):
self.driver = driver
# 查找元素
def base_find_element(self,loc,timeout=30,poll_frequency=0.5):
"""
:param loc: 元素的定位信息,格式为元组
:param timeout: 显式等待最长时间
:param poll_frequency: 显式等待请求间隔
:return: 返回查找到的对象
"""
# 显式等待特定组件
return WebDriverWait(self.driver,
timeout=timeout,
poll_frequency=poll_frequency).until(lambda x:x.find_element(*loc))
# 点击
def base_click(self,loc):
self.base_find_element(loc).click()
# 获取组件value属性值
def base_get_value(self,loc):
return self.base_find_element(loc).get_attribute('value')
# 截图
def base_get_img(self):
self.driver.get_screenshot_as_file(f'../image/{
time.strftime("%Y_%m_%d %H_%M_%S")}.png')
get_driver.py
from selenium import webdriver
from calcTestProject import page
class GetDriver:
driver = None
# 获取driver
@classmethod
def get_driver(cls):
if cls.driver is None:
option = webdriver.ChromeOptions()
# 指定chrome存储路径的二进制形式
option.binary_location = page.binary_location
# 实例化浏览器
cls.driver = webdriver.Chrome(options=option)
# 最大化窗口
cls.driver.maximize_window()
# 打开浏览器
cls.driver.get(page.url)
return cls.driver
# 推出driver
@classmethod
def quit_driver(cls):
if cls.driver:
cls.driver.quit()
# 置空
cls.driver=None
page layer
__init__.py
from selenium.webdriver.common.by import By
""""以下为服务器域名配置数据"""
url = "http://zaixianjisuanqi.bmcx.com"
# 谷歌浏览器位置
binary_location='D:\Chrome\Google\Chrome\Application\chrome.exe'
"""以下为计算器配置数据"""
# 加号
clac_add = (By.CSS_SELECTOR,'#simpleAdd')
# 等号
clac_eq = (By.CSS_SELECTOR,'#simpleEqual')
# 获取结果
clac_result = (By.CSS_SELECTOR,'#resultIpt')
# 清屏
clac_clear = (By.CSS_SELECTOR,'#simpleClearAllBtn')
page_calc.py
from calcTestProject.base.base import Base
from selenium.webdriver.common.by import By
from calcTestProject import page
class PageCalc(Base):
# 点击数字方法
def page_click_num(self,num):
for n in str(num):
# 按照计算需求拼接数字按键的loc
loc = (By.CSS_SELECTOR,f'#simple{
n}')
self.base_click(loc)
# 点击加号
def page_click_add(self):
self.base_click(page.clac_add)
# 点击等号
def page_click_eq(self):
self.base_click(page.clac_eq)
# 获取结果方法
def page_get_value(self):
self.base_get_value(page.clac_result)
# 点击清屏
def page_click_clear(self):
self.base_click(page.clac_clear)
# 截图
def page_get_image(self):
self.base_get_img()
# 组装加法业务方法
def page_add_calc(self,a,b):
# 清屏
self.page_click_clear()
# 点击第一个数
self.page_click_num(a)
# 点击加号
self.page_click_add()
# 点击第二个数
self.page_click_num(b)
# 点击等号
self.page_click_eq()
# 返回计算结果
return self.page_get_value()
script layer
test_calc.py
import unittest
from parameterized import parameterized
from calcTestProject.page.page_calc import PageCalc
from calcTestProject.base.get_driver import GetDriver
class TestCalc(unittest.TestCase):
# 类前置操作
@classmethod
def setUpClass(cls):
# 获取driver对象
cls.driver = GetDriver().get_driver()
cls.calc = PageCalc(cls.driver)
# 下拉条,防止广告遮挡
js = "window.scrollTo(0,256)"
cls.driver.execute_script(js)
# 类后置操作
@classmethod
def tearDownClass(cls):
GetDriver().quit_driver()
# 测试加法方法(1+2是否等于3)
@parameterized.expand(((1,2,3),))
def test_add_calc(self,a,b,expect):
# 调用计算业务方法
self.calc.page_add_calc(a,b)
try:
# 断言【判断测试结果】
self.assertEqual(self.calc.page_get_value(),str(expect))
except:
# 截图
self.calc.page_get_image()