Article directory
- 1. Servlet basics
- 2. Introduction to Servlet Development
- 3. ServletConfig and ServletContext
-
- (1) ServletConfig interface
-
- 1. ServletConfig interface
- 2. Case demonstration ServletConfig method call
- 2. Case demonstration of obtaining the container initialization parameters of a web application
- 3. Implement multiple Servlet objects to share data
- 4. Case demonstrates multiple Servlet objects sharing data
- 5. Read resource files under the web application
- 6. Case demonstration of reading resource files under Web application
- 4. HttpServletResponse object
- 5. HttpServletResponse application
- 6. HttpServletRequest object
1. Servlet basics
- Goal: Master the concepts, features and interfaces of Servlet
(1) Servlet overview
1. What is Servlet?
- Servlet is a Java application running on the Web server side, which is written in Java language. The difference from Java programs is that the Servlet object mainly encapsulates the processing of HTTP requests, and its operation requires the support of the Servlet container. In terms of Java Web applications, Servlet applications occupy a very important position, and it is also very powerful in processing Web requests.
2, Servlet container
- Servlet is provided by the Servlet container, which refers to the server that provides the Servlet function (this lecture notes refers to Tomcat). The Servlet container dynamically loads Servlets onto the server. Servlets related to the HTTP protocol interact with clients using HTTP requests and HTTP responses. Therefore, the Servlet container supports requests and responses for all HTTP protocols.
3. Servlet application architecture
- The Servlet request will first be received by the HTTP server (such as Apache). The HTTP server is only responsible for parsing the static HTML page. The Servlet request is forwarded to the Servlet container. The Servlet container will call the corresponding Servlet according to the mapping relationship in the web.xml file. , the Servlet returns the processed results to the Servlet container, and transmits the response to the client through the HTTP server.
(2) Characteristics of Servlet
- Servlet is written in Java language. It not only has the advantages of Java language, but also encapsulates Web-related applications. At the same time, the Servlet container also provides related extensions to applications, which are excellent in terms of functions, performance, security, etc. .
1. Powerful function
- Servlet is written in Java language. It can call objects and methods in Java API. In addition, Servlet object encapsulates Web applications, provides Servlet's programming interface for Web applications, and can also handle HTTP requests accordingly, such as processing Submit data, session tracking, read and set HTTP header information, etc. Since Servlet not only has the API provided by Java, but also can call the Servlet API programming interface encapsulated by Servlet, it is very powerful in terms of business functions.
2. Portable
- The Java language is cross-platform. The so-called cross-platform means that the running of the program does not depend on the operating system platform. It can run on multiple system platforms, such as the currently commonly used operating systems Windows, Linux and UNIX.
3. High performance
- The Servlet object is initialized when the Servlet container starts. When the Servlet object is requested for the first time, the Servlet container instantiates the Servlet object. At this time, the Servlet object resides in memory. If there are multiple requests, the Servlet will not be instantiated again, and other requests will still be processed by the Servlet object instantiated for the first time. Each request is a thread, not a process.
4. High safety
- Servlet uses Java's security framework, and the Servlet container can also provide additional security functions for Servlet, and its security is very high.
5. Expandable
- Java language is an object-oriented programming language. Servlet is written in Java language, so it has the advantages of object-oriented. In business logic processing, actual business needs can be expanded through features such as encapsulation and inheritance.
(3) Servlet interface
1. Servlet interface
- For the development of Servlet technology, SUN provides a series of interfaces and classes, the most important of which is the javax.servlet.Servlet interface. Servlet is a class that implements the Servlet interface. It is created and called by the Web container to receive and respond to user requests.
2. Servlet interface methods
method declaration | Function description |
---|---|
void init(ServletConfig config) | After the Servlet is instantiated, the Servlet container calls this method to complete the initialization work. |
ServletConfig getServletConfig() | Used to obtain the configuration information of the Servlet object and return the ServletConfig object of the Servlet |
String getServletInfo() | Returns a string containing information about the Servlet, such as author, version, and copyright information. |
void service(ServletRequest request,ServletResponse response) | Responsible for responding to user requests. This method is called when the container receives a request from the client to access the Servlet object. The container will construct a ServletRequest object representing the client's request information and a ServletResponse object used to respond to the client and pass them to the service() method as parameters. In the service() method, you can obtain client-related information and request information through the ServletRequest object. After processing the request, call the method of the ServletResponse object to set the response information. |
void destroy() | Responsible for releasing the resources occupied by the Servlet object. When the server is shut down or the Servlet object is removed, the Servlet object will be destroyed and the container will call this method |
3. Life cycle methods in Servlet interface
- Among the five methods in the Servlet interface, three of them, init(), service(), and destroy(), can represent the life cycle of the Servlet, and they will be called at a specific moment. It should be noted that the Servlet container refers to the Web server.
4. Implementation class of Servlet interface
- For the Servlet interface, SUN provides two default interface implementation classes: GenericServlet and HttpServlet. GenericServlet is an abstract class that provides partial implementation of the Servlet interface. It does not implement HTTP request processing. HttpServlet is a subclass of GenericServlet. It inherits all methods of GenericServlet and provides specific operation methods for POST, GET and other types of HTTP requests.
5. Common methods and functions of the HttpServlet class
method declaration | Function description |
---|---|
protected void doGet(HttpServletRequest req, HttpServletResponse resp) | Method for handling HTTP requests of type GET |
protected void doPost(HttpServletRequest req, HttpServletResponse resp) | Method for handling HTTP requests of type POST |
protected void doPut(HttpServletRequest req, HttpServletResponse resp) | Method for handling HTTP requests of type PUT |
2. Introduction to Servlet Development
(1) Implement the first Servlet program
- Goal: Master how to use IDEA tools to develop Servlet programs
1. Use IDEA to complete Servlet development
- In actual development, IDEA (or Eclipse, etc.) tools are usually used to complete the development of Servlets. We use IDEA to complete the development of Servlets, because IDEA not only automatically compiles Servlets, but also automatically creates web.xml file information and completes the Servlet virtual path. of mapping.
(1) Create a new Web project
-
Select the "Create New Project" option on the IDEA homepage to enter the new project interface.
-
In the New Project interface, select the "Java" option in the left column, and then check the "Web Application" option. After selecting, click the "Next" button to enter the interface for filling in project information.
-
In the New Project interface, the "Project name" option is used to refer to the name of the project, and the "Project localtion" option is used to specify the root directory of the Web project. Set the root directory of the project to
D:\web_work\chapter04
, and useWebDemo
as the name of the Web project. After the settings are completed, click the "Finish" button to enter the development interface.
-
Modify Artifact name -
WebDemo
-
Edit Tomcat server configuration
-
Switch to the [Server] tab
-
Start the server and see the effect
(2) Create Servlet class
-
Create a new net.xyx.servlet package
-
Create
ServletDemo01
class in net.xyxi.servlet package -
At this point the IDEA tool will automatically generate Servlet code
-
In order to better demonstrate the running effect of Servlet, next add some code to the doGet() and doPost() methods of ServletDemo01. Set the urlPatterns attribute value in the @WebServlet annotation: urlPatterns = "/demo01"
package net.xyx.servlet;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:Servlet演示类
* 作者:xyx
* 日期:2023年03月12日
*/
@WebServlet(name = "ServletDemo01", urlPatterns = "/demo01")
public class ServletDemo01 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取字符输出流
PrintWriter out = response.getWriter();
// 输出信息
out.print("<h1>Hello Servlet World~</h1>");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- In fact, Servlet is essentially an HTML page embedded in a Java program.
(3) Start Servlet
-
Start the server and display the home page
-
Access the url address "localhost:8080/WebDemo/demo01" of the ServletDemo01 class on the page
-
Correspondence diagram
-
If you want to display a red message and display it in the center, you need to use the style
2. Class exercise - Create a Web project and use Servlet to display personal information
-
Create a Java Enterprise project
-
Set project name and save location
-
Click the [Finish] button
-
View the names of Artifacts
-
Change the name to ShowInfo
-
Configure tomcat server
-
Delete ShowInfo in Deployment and add it again
-
Switch to the [Server] tab and view the URL
-
Start the tomcat server and check the effect
-
Modify the home page file to display personal information in table form
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>个人信息</title>
</head>
<body>
<table style="text-align: center" border="1" align="center" cellpadding="5">
<tr>
<td>学号</td>
<td>姓名</td>
<td>性别</td>
<td>年龄</td>
<td>专业</td>
<td>班级</td>
<td>手机</td>
</tr>
<tr>
<td>20210201</td>
<td>陈燕文</td>
<td>女</td>
<td>18</td>
<td>软件技术专业</td>
<td>2021软件2班</td>
<td>15890456780</td>
</tr>
</table>
</body>
</html>
-
Start the server and view the results
-
Create the net.huawei.servlet package and create the InfoServlet class in the package
package net.xyx.servlet;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:显示个人信息
* 作者:xyx
* 日期:2023年03月31日
*/
@WebServlet(name = "InfoServlet", urlPatterns = "/info")
public class InfoServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取字符输出流
PrintWriter out = response.getWriter();
// 往客户端输出信息
out.print("<!DOCTYPE html>");
out.print("<html>");
out.print("<head>");
out.print("<meta charset='UTF-8'");
out.print("<title>个人信息</title>");
out.print("</head>");
out.print("<body style='text-align: center'>");
out.print("<table border='1' align='center' cellpadding='5'>");
out.print("<tr>");
out.print("<td>学号</td>");
out.print("<td>姓名</td>");
out.print("<td>性别</td>");
out.print("<td>年龄</td>");
out.print("<td>专业</td>");
out.print("<td>班级</td>");
out.print("<td>手机</td>");
out.print("</tr>");
out.print("<tr>");
out.print("<td>20210201</td>");
out.print("<td>陈燕文</td>");
out.print("<td>女</td>");
out.print("<td>18</td>");
out.print("<td>软件技术专业</td>");
out.print("<td>2021软件2班</td>");
out.print("<td>15890456780</td>");
out.print("</tr>");
out.print("</table>");
out.print("</body>");
out.print("</html>");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
Start the tomcat server and visit http://localhost:8080/ShowInfo/info
-
Chinese garbled characters appear on the page, and the character encoding needs to be set (you need to check the default character encoding of the Chrome browser)
-
Set the response object character encoding to UTF-8, consistent with the character encoding currently used by the browser
-
Restart the tomcat server and visit http://localhost:8080/ShowInfo/info
-
Regardless of the character encoding currently used by the browser, set the response object content type to require the browser to use the specified character encoding.
-
Restart the tomcat server and visit http://localhost:8080/ShowInfo/info
-
Check the character encoding currently used by the browser. It has been changed to GBK.
(2) Configuration of Servlet
- Objective: Master the two ways to complete Servlet configuration: through the web application configuration file web.xml, and through the @WebServlet annotation to complete Servlet configuration
- If you want the Servlet to run correctly in the server and process the request information, you must perform appropriate configuration. There are two main ways to configure the Servlet, namely through the configuration file web.xml of the Web application and using the @WebServlet annotation. way to complete.
1. Configure Servlet using web.xml
- In the web.xml file, registration is done through tags, and several sub-elements are included under the tags.
attribute name | Type Description |
---|---|
<servlet-name> |
String specifies the name of the Servlet, which is generally the same as the Servlet class name and must be unique. |
<servlet-class> |
String specifies the location of the Servlet class, including package name and class name |
<description> |
String specifies the description information of the Servlet |
<display-name> |
String specifies the display name of the Servlet |
-
Map the Servlet to the URL address, use tags for mapping, use sub-tags to specify the name of the Servlet to be mapped, the name must be the same as the one previously registered under the tag; use sub-tags to map URL addresses, you must add "/" before the address, otherwise access Less than.
-
After registering the Servlet in the deployment description file web.xml, you can comment out the annotations on the InfoServlet class.
-
Restart the tomcat server and access
http://localhost:8080/ShowInfo/info
2. @WebServlet annotation attributes
- The @WebServlet annotation is used to replace other tags in the web.xml file. This annotation will be processed by the container during project deployment, and the container will deploy the corresponding class as a Servlet based on the specific attribute configuration. For this purpose, the @WebServlet annotation provides some properties.
Property declaration | Function description |
---|---|
String name | Specifies the name attribute of the Servlet, which is equivalent to. If not specified explicitly, the |
The value of Servlet is the fully qualified name of the class. | |
String[] value | This attribute is equivalent to the urlPatterns attribute. The urlPatterns and value attributes cannot be used at the same time. |
String[] urlPatterns | Specifies a set of Servlet URL matching patterns. Equivalent to label. |
int loadOnStartup | Specifies the loading order of Servlets, which is equivalent to labels. |
WebInitParam[] | Specify a set of Servlet initialization parameters, equivalent to tags. |
boolean asyncSupported | Declares whether the Servlet supports asynchronous operation mode, equivalent to the tag. |
String description | The description information of the Servlet is equivalent to the label. |
String displayName ervlet | The display name of , usually used with tools, is equivalent to the label. |
@WebServlet | Annotations can be annotated on any class that inherits the HttpServlet class and are class-level annotations. Next, use the @WebServlet annotation to annotate the InfoServlet class. |
-
Comment out the registration tag for InfoServlet in the web.xml file
-
Restart the tomcat server and visit http://localhost:8080/ShowInfo/info
-
Use the @WebServlet annotation to mark the InfoServlet class as a Servlet. The name attribute value in the @WebServlet annotation is used to specify the name attribute of the servlet. Equivalently, if the name attribute of @WebServlet is not set, its default value is the full class name of the Servlet. The urlPatterns attribute value is used to specify a set of servlet URL matching patterns, which is equivalent to a label. If you need to set multiple properties in the @WebServlet annotation, separate them with commas. The @WebServlet annotation can greatly simplify the Servlet configuration steps and reduce the development difficulty for developers.
3. Configure multiple URLs for a Servlet
-
It’s like a person has multiple external contact methods
-
Start the server and access
http://localhost:8080/ShowInfo/info
-
access
http://localhost:8080/ShowInfo/message
(3) Servlet life cycle
- Goal: Master the three life cycles of Servlet, initialization phase, running phase and destruction phase
1. Servle life cycle - initialization phase
- When the client sends an HTTP request to the Servlet container to access the Servlet, the Servlet container will first parse the request and check whether the Servlet object already exists in the memory. If so, use the Servlet object directly; if not, create a Servlet instance object, and then Complete the initialization of the Servlet by calling the init() method. It should be noted that during the entire life cycle of the Servlet, its init() method is only called once.
2. Servlet life cycle - running phase
- This is the most important stage in the Servlet life cycle. At this stage, the Servlet container will create a ServletRequest object representing the HTTP request and a ServletResponse object representing the HTTP response for the client request, and then pass them as parameters to the Servlet's service() method. . The service() method obtains the client request information from the ServletRequest object and processes the request, and generates the response result through the ServletResponse object. During the entire life cycle of the Servlet, for each access request of the Servlet, the Servlet container will call the service() method of the Servlet once and create new ServletRequest and ServletResponse objects. In other words, the service() method will be used throughout the entire life of the Servlet. It will be called multiple times during the cycle.
3. Servlet life cycle - destruction phase
- When the server is shut down or the web application is removed from the container, the Servlet is destroyed along with the web application. Before destroying the Servlet, the Servlet container will call the Servlet's destroy() method to allow the Servlet object to release the resources it occupies. In the entire life cycle of the Servlet, the destroy() method is only called once. It should be noted that once the Servlet object is created, it will reside in the memory and wait for client access. The Servlet object will not be destroyed until the server is shut down or the web application is removed from the container.
4. Case demonstration Servlet life cycle
- Create the ServletDemo02 class in the WebDemo project, write the init() method and destroy() method in the ServletDemo02 class, and override the service() method to use a case to demonstrate the execution effect of the Servlet life cycle method.
package net.xyx.servlet;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:演示Servlet生命周期
* 作者:xyx
* 日期:2023年03月12日
*/
@WebServlet(name = "ServletDemo02", value = "/demo02")
public class ServletDemo02 extends HttpServlet {
@Override
public void init() throws ServletException {
System.out.println("init()方法被调用……");
}
protected void service(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("service()方法被调用……");
response.setContentType("text/html; charset=utf-8");
PrintWriter out = response.getWriter();
out.print("<h1>演示Servlet生命周期~</h1>");
}
@Override
public void destroy() {
System.out.println("destroy()方法被调用……");
}
}
-
Start the tomcat server and access
http://localhost:8080/WebDemo/demo02
-
Refresh the browser twice, visit ServletDemo02 twice, and view the print results of the tomcat console.
-
The init() method is only executed on the first access, and the service() method is executed on each access.
-
If you want to remove ServletDemo02, you can stop the WebDemo project in IDEA. At this time, the Servlet container will call the destroy() method of ServletDemo02 and print out the "destroy() method was called..." message on the IDEA console.
-
For example: we can only be born once (init() method) and die once (destroy() method), and we can change jobs many times in the middle to provide different services to the society (service() method).
3. ServletConfig and ServletContext
(1) ServletConfig interface
- Goal: Master how to use ServletConfig to obtain configuration information
1. ServletConfig interface
- During the running of the Servlet, some configuration information is often needed, such as the encoding used by the file, etc. This information can be configured in the properties of the @WebServlet annotation. When Tomcat initializes a Servlet, it encapsulates the Servlet's configuration information into a ServletConfig object, and passes the ServletConfig object to the Servlet by calling the init(ServletConfig config) method. ServletConfig defines a series of methods for obtaining configuration information.
Method description | Function description |
---|---|
String getInitParameter(String name) | Returns the corresponding initialization parameter value based on the initialization parameter name. |
Enumeration getInitParameterNames() | Returns an Enumeration object containing all initialization parameter names |
ServletContext getServletContext() | Returns a ServletContext object representing the current web application |
String getServletName() | Returns the name of the Servlet |
2. Case demonstration ServletConfig method call
- Taking the getInitParameter() method as an example to explain the calling of the ServletConfig method
- Create the ServletDemo03 class in the net.huawei.servlet package of the WebDemo project
package net.xyx.servlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebInitParam;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Enumeration;
/**
* 功能:演示ServletConfig方法的调用
* 作者:xyx
* 日期:2023年03月31日
*/
@WebServlet(name = "ServletDemo03", urlPatterns = "/demo03",
initParams = {@WebInitParam(name="encoding", value = "utf-8"),
@WebInitParam(name="text-color", value = "red"),
@WebInitParam(name="font-size", value= "25")})
public class ServletDemo03 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应对象内容类型
response.setContentType("text/html; charset=utf-8");
// 获取打印输出流
PrintWriter out = response.getWriter();
// 获取Servlet配置对象
ServletConfig config = getServletConfig();
// 获取初始化参数名枚举对象
Enumeration<String> initParams = config.getInitParameterNames();
// 遍历初始化参数名枚举对象,输出参数名及其值
while (initParams.hasMoreElements()) {
String initParam = initParams.nextElement();
out.print(initParam + " : " + config.getInitParameter(initParam) + "<br />");
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
Start the tomcat server and visit http://localhost:8080/WebDemo/demo03
(二)ServletContext接口
目标:掌握ServletContext接口的使用方法
1、获取Web应用程序的初始化参数
当Servlet容器启动时,会为每个Web应用创建一个唯一的ServletContext对象代表当前Web应用。ServletContext对象不仅封装了当前Web应用的所有信息,而且实现了多个Servlet之间数据的共享。
在web.xml文件中,可以配置Servlet的初始化信息,还可以配置整个Web应用的初始化信息。Web应用初始化参数的配置方式具体如下所示。
参数名
参数值
参数名
参数值
1
2
3
4
5
6
7
8
<context-param>
元素位于根元素<web-app>
中,它的子元素<param-name>
和<param-value>
分别用来指定参数的名字和参数值。可以通过调用ServletContext接口中定义- getInitParameterNames()和getInitParameter(String name)方法,分别获取参数名和参数值。
2、案例演示获取Web应用的容器初始化参数
- 在WebDemo项目的web.xml文件中,配置容器初始化参数信息和Servlet信息
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<context-param>
<param-name>college</param-name>
<param-value>泸州职业技术学院</param-value>
</context-param>
<context-param>
<param-name>address</param-name>
<param-value>泸州市龙马潭区长桥路2号</param-value>
</context-param>
<context-param>
<param-name>secretary</param-name>
<param-value>何杰</param-value>
</context-param>
<context-param>
<param-name>president</param-name>
<param-value>谢鸿全</param-value>
</context-param>
</web-app>
- 在net.huawei.servlet包里创建
ServletDemo04
类
package net.xyx.servlet;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Enumeration;
/**
* 功能:获取Web应用的容器初始化参数
* 作者:xyx
* 日期:2023年03月31日
*/
@WebServlet(name = "ServletDemo04", urlPatterns = "/demo04")
public class ServletDemo04 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应对象内容类型
response.setContentType("text/html; charset=utf-8");
// 获取打印输出流
PrintWriter out = response.getWriter();
// 获取Servlet容器对象
ServletContext context = getServletContext();
// 获取容器的初始化参数名枚举对象
Enumeration<String> paramNames = context.getInitParameterNames();
// 通过循环遍历显示全部参数名与参数值
while (paramNames.hasMoreElements()) {
// 获取参数名
String name = paramNames.nextElement();
// 按参数名获取参数值
String value = context.getInitParameter(name);
// 输出参数名和参数值
out.print(name + " : " + value + "<br />");
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- 启动tomcat服务器,访问
http://localhost:8080/WebDemo/demo04
3、实现多个Servlet对象共享数据
- 由于一个Web应用中的所有Servlet共享同一个ServletContext对象,所以ServletContext对象的域属性可以被该Web应用中的所有Servlet访问。ServletContext接口中定义了用于增加、删除、设置ServletContext域属性的四个方法。
方法说明 | 功能描述 |
---|---|
Enumeration getAttributeNames() | 返回一个Enumeration对象,该对象包含了所有存放在ServletContext中的所有域属性名 |
Object getAttibute(String name) | 根据参数指定的属性名返回一个与之匹配的域属性值 |
void removeAttribute(String name) | 根据参数指定的域属性名,从ServletContext中删除匹配的域属性 |
void setAttribute(String name,Object obj) | 设置ServletContext的域属性,其中name是域属性名,obj是域属性值 |
4、案例演示多个Servlet对象共享数据
- 在net.xyx.servlet包里创建
ServletDemo05
类
package net.xyx.servlet;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:写入域属性
* 作者:xyx
* 日期:2023年03月31日
*/
@WebServlet(name = "ServletDemo05", urlPatterns = "/demo05")
public class ServletDemo05 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应对象内容类型
response.setContentType("text/html; charset=utf-8");
// 获取Servlet容器对象
ServletContext context = getServletContext();
// 写入域属性
context.setAttribute("id", "20210201");
context.setAttribute("name", "陈雅雯");
context.setAttribute("gender", "女");
context.setAttribute("age", "18");
context.setAttribute("major", "软件技术专业");
context.setAttribute("class", "2021软件2班");
context.setAttribute("telephone", "15890903456");
// 获取打印输出流
PrintWriter out = response.getWriter();
// 输出提示信息
out.print("<h3>成功地写入了域属性~</h3>");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- 在net.xyx.servlet包里创建
ServletDemo06
类
package net.xyxx.servlet;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Enumeration;
/**
* 功能:读取域属性
* 作者:xyx
* 日期:2023年03月31日
*/
@WebServlet(name = "ServletDemo06", urlPatterns = "/demo06")
public class ServletDemo06 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应对象内容类型
response.setContentType("text/html; charset=utf-8");
// 获取打印输出流
PrintWriter out = response.getWriter();
// 获取Servlet容器对象
ServletContext context = getServletContext();
// 获取全部域属性名枚举对象
Enumeration<String> attributeNames = context.getAttributeNames();
// 通过循环显示域属性名与域属性值
while (attributeNames.hasMoreElements()) {
// 获取域属性名
String name = attributeNames.nextElement();
// 获取域属性值
Object value = context.getAttribute(name);
// 输出域属性名与域属性值
out.print(name + " : " + value + "<br />");
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
启动tomcat服务器,先访问
http://localhost:8080/WebDemo/demo05
-
再访问
http://localhost:8080/WebDemo/demo06
-
有很多域属性不是我们写入的,如果我们只想显示我们写入的域属性,那么我们就要修改一下ServletDemo06的代码
-
重启服务器,访问
http://localhost:8080/WebDemo/demo05
-
再访问
http://localhost:8080/WebDemo/demo06
5、读取Web应用下的资源文件
- ServletContext接口定义了一些读取Web资源的方法,这些方法是依靠Servlet容器来实现的。
- Servlet容器根据资源文件相对于Web应用的路径,返回关联资源文件的IO流、资源文件在文件系统的绝对路径等。
方法说明 | 功能描述 |
---|---|
Set getResourcePaths(String path) | 返回一个Set集合,集合中包含资源目录中子目录和文件的路径名称。参数path必须以正斜线(/)开始,指定匹配资源的部分路径 |
String getRealPath(String path) | 返回资源文件在服务器文件系统上的真实路径(文件的绝对路径)。参数path代表资源文件的虚拟路径,它应该以正斜线(/)开始,“/”表示当前Web应用的根目录,如果Servlet容器不能将虚拟路径转换为文件系统的真实路径,则返回null |
URL getResource(String path) | 返回映射到某个资源文件的URL对象。参数path必须以正斜线(/)开始,“/”表示当前Web应用的根目录 |
InputStream getResourceAsStream(String path) | 返回映射到某个资源文件的InputStream输入流对象。参数path传递规则和getResource()方法完全一致 |
6、案例演示读取Web应用下的资源文件
- 在net.xyx.servlet包里创建属性文件 -
college.properties
name = 泸州职业技术学院
address = 泸州市龙马潭区长桥路2号
secretary = 何杰
president = 谢鸿全
- 在net.xyxservlet包里创建
ServletDemo07
类
package net.xyx.servlet;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.Properties;
/**
* 功能:读取资源文件
* 作者:xyx
* 日期:2023年03月13日
*/
@WebServlet(name = "ServletDemo07", urlPatterns = "/demo07")
public class ServletDemo07 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置内容类型
response.setContentType("text/html; charset=utf-8");
// 获取Servlet容器对象
ServletContext context = getServletContext();
// 获取打印输出流
PrintWriter out = response.getWriter();
// 读取资源文件,得到字节输入流
InputStream in = context.getResourceAsStream(
"/WEB-INF/classes/net/huawei/servlet/college.properties");
// 创建属性对象(Map接口的实现类)
Properties pros = new Properties();
// 属性对象加载资源文件的资源文件输入流
pros.load(in);
// 往客户端输出属性值
out.println("name = " + pros.getProperty("name") + "<br />");
out.println("address = " + pros.getProperty("address") + "<br />");
out.println("secretary = " + pros.getProperty("secretary") + "<br />");
out.println("president = " + pros.getProperty("president") + "<br />");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
启动tomcat服务器,访问
http://localhost:8080/WebDemo/demo07
-
属性文件的路径问题
-
读取属性文件得到的字节流编码是ISO-8859-1,需要做一个转码处理
-
重启tomcat服务器,先访问
http://localhost:8080/WebDemo/demo07
-
在Web项目开发中开发者可能需要获取资源的绝对路径。通过调用getRealPath(String path)方法获取资源文件的绝对路径。
-
在net.xyx.servlet包里创建ServletDemo08类
package net.xyx.servlet;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:获取资源的绝对路径
* 作者:xyx
* 日期:2023年03月13日
*/
@WebServlet(name = "ServletDemo08", urlPatterns = "/demo08")
public class ServletDemo08 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取Servlet容器对象
ServletContext context = getServletContext();
// 获取打印输出流
PrintWriter out = response.getWriter();
// 创建资源路径字符串
String path = "/WEB-INF/classes/net/huawei/servlet/college.properties";
// 获取资源绝对路径
String realPath = context.getRealPath(path);
// 输出资源绝对路径
out.println("college.properties: " + realPath);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- 重启tomcat服务器,访问
http://localhost:8080/WebDemo/demo08
四、HttpServletResponse对象
(一)发送状态码相关的方法
- 目标:掌握HttpServletResponse接口定义的3个发送状态码的方法
当Servlet向客户端回送响应消息时,需要在响应消息中设置状态码,状态码代表着客户端请求服务器的结果。为此,HttpServletResponse接口定义了3个发送状态码的方法。
1、HttpServletResponse接口—setStatus(int status)方法
- setStatus(int status)方法用于设置HTTP响应消息的状态码,并生成响应状态行。由于响应状态行中的状态描述信息直接与状态码相关,而HTTP版本由服务器确定,所以,只要通过setStatus(int status)方法设置了状态码,即可实现状态行的发送。例如,正常情况下,Web服务器会默认产生一个状态码为200的状态行。
2、HttpServletResponse接口—sendError(int sc)方法
- sendError(int sc)方法用于发送表示错误信息的状态码,例如,404状态码表示找不到客户端请求的资源。
3、HttpServletResponse接口—sendError(int code,String message)方法
- sendError(int code, String message)方法除了设置状态码,还会向客户端发出一条错误信息。服务器默认会创建一个HTML格式的错误服务页面作为响应结果,其中包含参数message指定的文本信息,这个HTML页面的内容类型为“text/html”,保留cookies和其他未修改的响应头信息。如果一个对应于传入的错误码的错误页面已经在web.xml中声明,那么这个声明的错误页面会将优先建议的message参数服务于客户端。
(二)发送响应头相关的方法
- 目标:掌握HttpServletResponse接口设置HTTP响应头字段的方法
方法说明 | 功能描述 |
---|---|
void addHeader(String name, String value) | 用来设置HTTP协议的响应头字段,其中,参数name用于指定响应头字段的名称,参数value用于指定响应头字段的值。addHeader()方法可以增加同名的响应头字段 |
void setHeader(String name, String value) | 用来设置HTTP协议的响应头字段,其中,参数name用于指定响应头字段的名称,参数value用于指定响应头字段的值。setHeader()方法则会覆盖同名的头字段 |
void addIntHeader(String name, int value) | 专门用于设置包含整数值的响应头。避免了调用addHeader()方法时,需要将int类型的设置值转换为String类型的麻烦 |
void setIntHeader(String name, int value) | 专门用于设置包含整数值的响应头。避免了调用setHeader()方法时,需要将int类型的设置值转换为String类型的麻烦 |
void setContentLength(int len) | 该方法用于设置响应消息的实体内容的大小,单位为字节。对于HTTP协议来说,这个方法就是设置Content-Length响应头字段的值 |
void setContentType(String type) | 该方法用于设置Servlet输出内容的MIME类型,对于HTTP协议来说,就是设置Content-Type响应头字段的值。例如,如果发送到客户端的内容是jpeg格式的图像数据,就需要将响应头字段的类型设置为“image/jpeg”。需要注意的是,如果响应的内容为文本,setContentType()方法的还可以设置字符编码,如:text/html;charset=UTF-8 |
void setLocale(Locale loc) | 该方法用于设置响应消息的本地化信息。对HTTP来说,就是设置Content-Language响应头字段和Content-Type头字段中的字符集编码部分。需要注意的是,如果HTTP消息没有设置Content-Type头字段,setLocale()方法设置的字符集编码不会出现在HTTP消息的响应头中,如果调用setCharacterEncoding()或setContentType()方法指定了响应内容的字符集编码,setLocale()方法将不再具有指定字符集编码的功能 |
void setCharacterEncoding(String charset) | 该方法用于设置输出内容使用的字符编码,对HTTP 协议来说,就是设置Content-Type头字段中的字符集编码部分。如果没有设置Content-Type头字段,setCharacterEncoding方法设置的字符集编码不会出现在HTTP消息的响应头中。setCharacterEncoding()方法比setContentType()和setLocale()方法的优先权高,setCharacterEncoding()方法的设置结果将覆盖setContentType()和setLocale()方法所设置的字符码表 |
- 需要注意的是,addHeader()、setHeader()、addIntHeader()、setIntHeader()方法都是用于设置各种头字段的,而setContetType()、setLoacale()和setCharacterEncoding()方法用于设置字符编码,这些设置字符编码的方法可以有效解决中文字符乱码问题。
(三)发送响应消息体相关的方法
- 目标:掌握发送响应消息体相关的方法getOutputStream()和getWriter()
- 由于在HTTP响应消息中,大量的数据都是通过响应消息体传递的,所以,ServletResponse遵循IO流传递大量数据的设计理念。在发送响应消息体时,定义了两个与输出流相关的方法。
1、getOutputStream()方法
- getOutputStream()方法所获取的字节输出流对象为ServletOutputStream类型。由于ServletOutputStream是OutputStream的子类,它可以直接输出字节数组中的二进制数据。所以,要想输出二进制格式的响应正文,就需要调用getOutputStream()方法。
2、getWriter()方法
getWriter()方法所获取的字符输出流对象为PrintWriter类型。由于PrintWriter类型的对象可以直接输出字符文本内容,所以,要想输出内容为字符文本的网页文档,需要调用getWriter()方法。
3、案例演示发送响应消息体
-
创建net.xyx.response包
-
在net.xyx.response包里创建PrintServlet01类
package net.huawei.response;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.OutputStream;
/**
* 功能:演示响应体输出字节流
* 作者:xyx
* 日期:2023年04月07日
*/
@WebServlet(name = "PrintServlet01", urlPatterns = "/print01")
public class PrintServlet01 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 定义字符串数据
String data = "欢迎访问泸州职业技术学院~";
// 获取字节输出流对象
OutputStream out = response.getOutputStream();
// 往客户端输出信息
out.write(data.getBytes());
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
重启tomcat服务器,访问
http://localhost:8080/WebDemo/print01
-
在net.xyx.response包里创建
PrintServlet02
类
package net.xyx.response;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:演示响应体打印字符流
* 作者:xyx
* 日期:2023年04月07日
*/
@WebServlet(name = "PrintServlet02", urlPatterns = "/print02")
public class PrintServlet02 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 定义字符串数据
String data = "欢迎访问泸州职业技术学院~";
// 获取打印字符流
PrintWriter out = response.getWriter();
// 往客户端输出信息
out.println(data);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
重启tomcat服务器,访问http://localhost:8080/WebDemo/print02
-
为了解决页面中文乱码问题,要修改代码
-
重启tomcat服务器,访问http://localhost:8080/WebDemo/print02
五、HttpServletResponse应用
(一)实现请求重定向
- 目标:掌握HttpServletResponse接口的sendRedirect()方法,实现请求重定向
在某些情况下,针对客户端的请求,一个Servlet类可能无法完成全部工作。这时,可以使用请求重定向来完成。所谓请求重定向,指的是Web服务器接收到客户端的请求后,可能由于某些条件限制,不能访问当前请求URL所指向的Web资源,而是指定了一个新的资源路径,让客户端重新发送请求。
1、HttpServletResponse接口—sendRedirect()方法
- 为了实现请求重定向,HttpServletResponse接口定义了一个sendRedirect()方法,该方法用于生成302响应码和Location响应头,从而通知客户端重新访问Location响应头中指定的URL。
sendRedirect()方法的完整声明:public void sendRedirect(java.lang.String location) throws java.io.IOException - 需要注意的是,参数location可以使用相对URL,Web服务器会自动将相对URL翻译成绝对URL,再生成Location头字段。
- sendRedirect()方法的工作原理
2、案例演示实现请求重定向
- 创建登录页面 -
login.html
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>用户登录</title>
</head>
<body>
<form action="login" method="post">
<fieldset>
<legend>用户登录</legend>
<table cellpadding="2" align="center">
<tr>
<td align="right">用户名:</td>
<td>
<input type="text" name="username"/>
</td>
</tr>
<tr>
<td align="right">密码:</td>
<td>
<input type="password" name="password"/>
</td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="submit" value="登录"/>
<input type="reset" value="重置"/>
</td>
</tr>
</table>
</fieldset>
</form>
</body>
</html>
- 创建欢迎页面 -
welcome.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>登录成功</title>
</head>
<body>
<h3 style="text-align: center">欢迎你,登录成功~</h3>
</body>
</html>
- 在net.xyx.response包里创建LoginServlet类,处理用户登录请求
package net.xyx.response;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* 功能:登录处理程序
* 作者:xyx
* 日期:2023年04月07日
*/
@WebServlet(name = "LoginServlet", urlPatterns = "/login")
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 获取登录表单提交的用户名和密码
String username = request.getParameter("username");
String password = request.getParameter("password");
// 判断是否登录成功,决定重定向到不同页面
if ("howard".equals(username) && "903213".equals(password)) {
// 重定向到欢迎页面
response.sendRedirect("/WebDemo/welcome.html");
} else {
// 重定向到登录页面
response.sendRedirect("/WebDemo/login.html");
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
重启tomcat服务器,访问
http://localhost:8080/WebDemo/login.html
-
在login.html页面填写用户名“howard”,密码“903213”
-
单击登录按钮,跳转到欢迎页面
-
录屏操作演示
(二)动手实践:解决中文输出乱码问题
- 目标:掌握如何解决中文输出乱码问题
1、中文乱码问题
- 由于计算机中的数据都是以二进制形式存储的,所以,当传输文本时,就会发生字符和字节之间的转换。字符与字节之间的转换是通过查码表完成的,将字符转换成字节的过程称为编码,将字节转换成字符的过程称为解码,如果编码和解码使用的码表不一致,就会导致乱码问题。
2、案例演示解决乱码问题
在net.xyx.response包里创建ChineseServlet
类
package net.xyx.response;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:演示解决中文乱码问题
* 作者:xyx
* 日期:2023年03月13日
*/
@WebServlet(name = "ChineseServlet", urlPatterns = "/chinese")
public class ChineseServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 创建数据字符串
String data = "欢迎访问泸州职业技术学院~";
// 获取打印字符输出流
PrintWriter out = response.getWriter();
// 在页面输出信息
out.println(data);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}```
- 重启tomcat服务器,访问`http://localhost:8080/WebDemo/chinese`
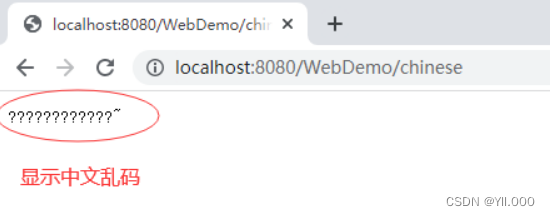
- 浏览器显示的内容都是“???~”,说明发生了乱码问题。此处产生乱码的原因是response对象的字符输出流在编码时,采用的是ISO-8859-1的字符码表,该码表并不兼容中文,会将“欢迎访问泸州职业技术学院”编码为“63 63 63 63 63 63 63 63 63 63 63 63”(在ISO-8859-1的码表中查不到的字符就会显示63)。当浏览器对接收到的数据进行解码时,会采用默认的码表GB2312,将“63 ”解码为“?”,因此,浏览器将“欢迎访问泸州职业技术学院”十二个字符显示成了“???”。
- 解决页面乱码问题
- `HttpServletResponse`接口提供了一个`setCharacterEncoding()`方法,该方法用于设置字符的编码方式,接下来对`ChineseServlet`类进行修改,在代码String data = "欢迎访问泸州职业技术学院~";前增加一行代码,设置字符编码使用的码表为utf-8。
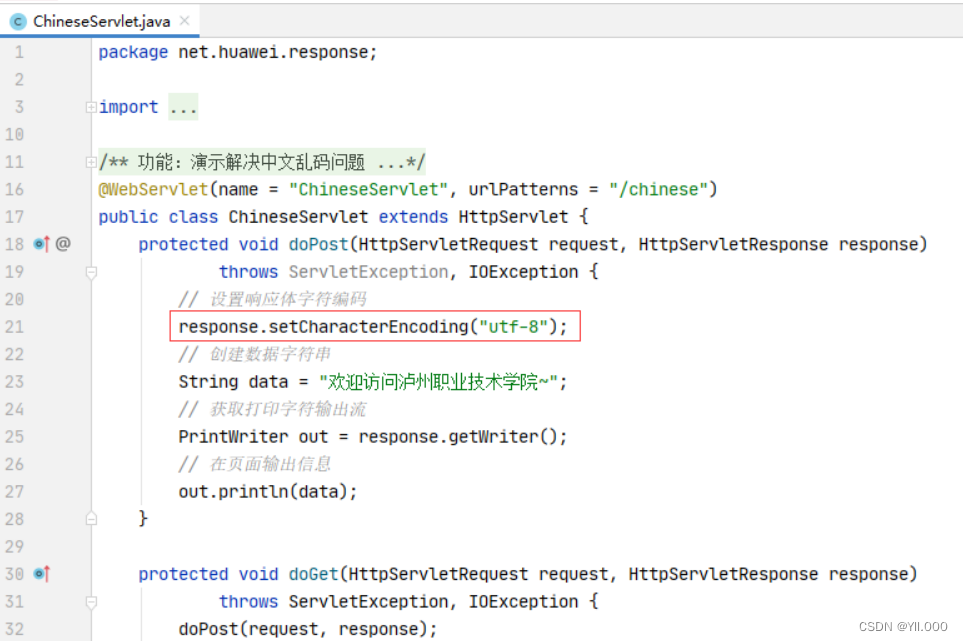
- 重启tomcat服务器,访问`http://localhost:8080/WebDemo/chinese`
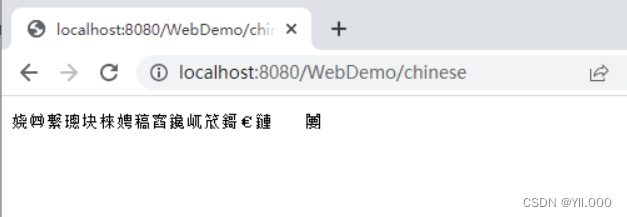
- 浏览器中显示的乱码虽然不是`“????????????~`”,但也不是需要输出的“欢迎访问泸州职业技术学院~”,这是由于浏览器解码错误导致的。`response`对象的字符输出流设置的编码方式为UTF-8,而浏览器使用的解码方式是GBK。
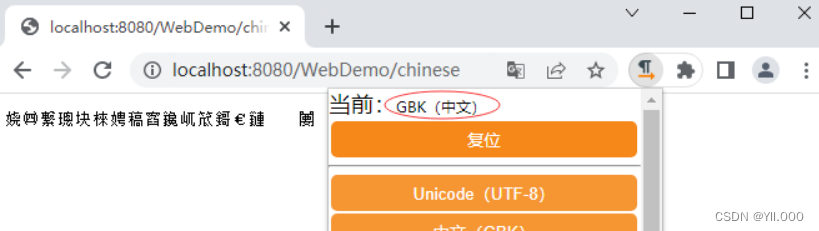
- 将`response`对象的字符输出流设置的编码方式改为GBK
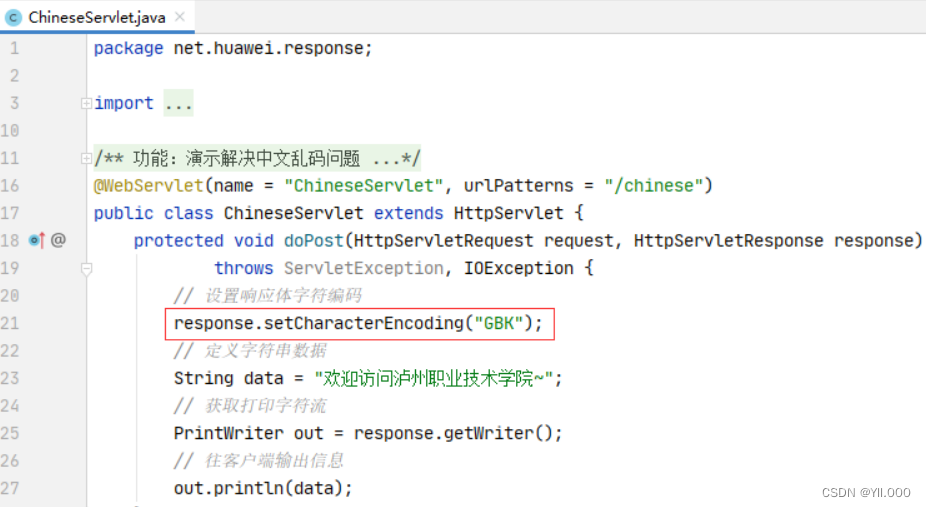
- 重启tomcat服务器,访问`http://localhost:8080/WebDemo/chinese`
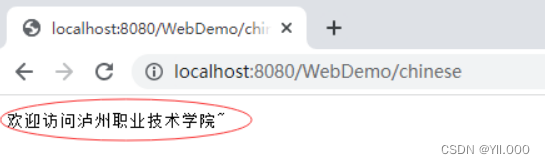
- 一种更好的方法来解决页面中文乱码问题,直接要求浏览器按照某种字符编码来显示中文
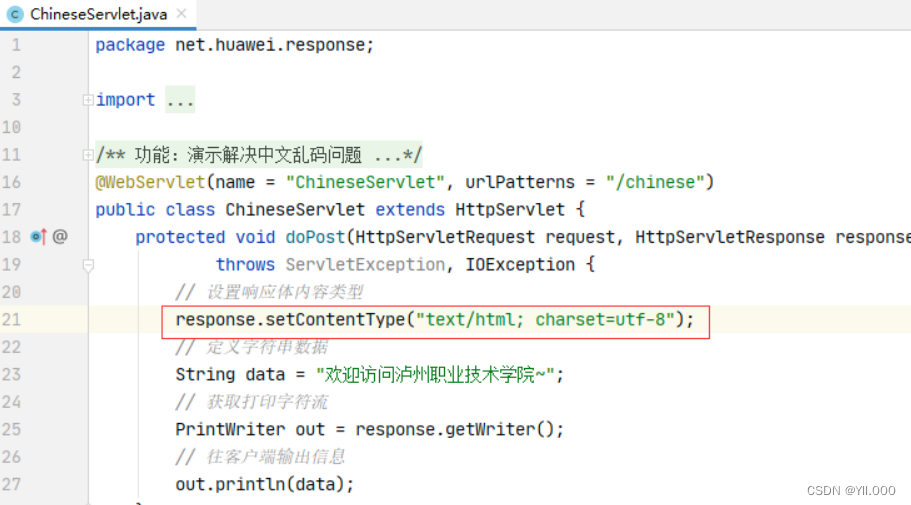
`response.setContentType("text/html;charset=utf-8")`;让浏览器采用utf-8字符编码
- 重启tomcat服务器,访问`http://localhost:8080/WebDemo/chinese`
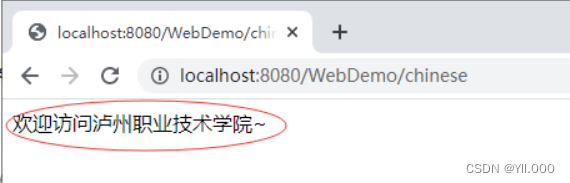
- 当然也可以换种方式来解决
```xml
// 设置HttpServletResponse使用utf-8编码
response.setCharacterEncoding("utf-8");
// 通知浏览器使用utf-8解码
response.setHeader("Content-Type", "text/html;charset=utf-8");
六、HttpServletRequest对象
(一)获取请求行信息的相关方法
- 目标:掌握使用
HttpServletRequest
接口中的方法获取请求行
1、相关方法
方法声明 | 功能描述 |
---|---|
String getMethod( ) | 该方法用于获取HTTP请求消息中的请求方式(如GET、POST等) |
String getRequestURI( ) | 该方法用于获取请求行中资源名称部分,即位于URL的主机和端口之后、参数部分之前的数据 |
String getQueryString( ) | 该方法用于获取请求行中的参数部分,也就是资源路径后面问号(?)以后的所有内容 |
String getProtocol( ) | 该方法用于获取请求行中的协议名和版本,例如HTTP/1.0或HTTP/1.1 |
String getContextPath( ) | 该方法用于获取请求URL中属于Web应用程序的路径,这个路径以“/”开头,表示相对于整个Web站点的根目录,路径结尾不含“/”。如果请求URL属于Web站点的根目录,那么返回结果为空字符串(“”) |
String getServletPath( ) | 该方法用于获取Servlet的名称或Servlet所映射的路径 |
String getRemoteAddr( ) | 该方法用于获取请求客户端的IP地址,其格式类似于“192.168.0.3” |
String getRemoteHost( ) | 该方法用于获取请求客户端的完整主机名,其格式类似于“pc1.itcast.cn”。需要注意的是,如果无法解析出客户机的完整主机名,该方法将会返回客户端的IP地址 |
int getRemotePort() | 该方法用于获取请求客户端网络连接的端口号 |
String getLocalAddr() | 该方法用于获取Web服务器上接收当前请求网络连接的IP地址 |
String getLocalName() | 该方法用于获取Web服务器上接收当前网络连接IP所对应的主机名 |
int getLocalPort() | 该方法用于获取Web服务器上接收当前网络连接的端口号 |
String getServerName() | 该方法用于获取当前请求所指向的主机名,即HTTP请求消息中Host头字段所对应的主机名部分 |
int getServerPort() | 该方法用于获取当前请求所连接的服务器端口号,即如果HTTP请求消息中Host头字段所对应的端口号部分 |
String getScheme() | 该方法用于获取请求的协议名,例如http、https或ftp |
StringBuffer getRequestURL() | 该方法用于获取客户端发出请求时的完整URL,包括协议、服务器名、端口号、资源路径等信息,但不包括后面的查询参数部分。注意,getRequestURL()方法返回的结果是StringBuffer类型,而不是String类型,这样更便于对结果进行修改 |
2、案例演示
创建net.xyx.request包,在包里创建RequestLineServlet
类
package net.xyx.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:输出请求行的相关信息
* 作者:xyx
* 日期:2023年03月13日
*/
@WebServlet(name = "RequestLineServlet", urlPatterns = "/request")
public class RequestLineServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html;charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取并输出请求行的相关信息
out.println("getMethod: " + request.getMethod() + "<br />");
out.println("getRequestURI: " + request.getRequestURI() + "<br />");
out.println("getQueryString: " + request.getQueryString() + "<br />");
out.println("getProtocol: " + request.getProtocol() + "<br />");
out.println("getContextPath: " + request.getContextPath() + "<br />");
out.println("getPathInfo: " + request.getPathInfo() + "<br />");
out.println("getPathTranslated: " + request.getPathTranslated() + "<br />");
out.println("getServletPath: " + request.getServletPath() + "<br />");
out.println("getRemoteAddr: " + request.getRemoteAddr() + "<br />");
out.println("getRemoteHost: " + request.getRemoteHost() + "<br />");
out.println("getRemotePort: " + request.getRemotePort() + "<br />");
out.println("getLocalAddr: " + request.getLocalAddr() + "<br />");
out.println("getLocalName: " + request.getLocalName() + "<br />");
out.println("getLocalPort: " + request.getLocalPort() + "<br />");
out.println("getServerName: " + request.getServerName() + "<br />");
out.println("getServerPort: " + request.getServerPort() + "<br />");
out.println("getScheme: " + request.getScheme() + "<br />");
out.println("getRequestURL: " + request.getRequestURL() + "<br />");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
重启tomcat服务器,访问
http://localhost:8080/WebDemo/request
-
getContextPath: /WebDemo + getServletPath: /request = getRequestURI: /WebDemo/request
-
getScheme: http + “:” + getServerName: localhost + “:” + getServerPort: 8080 + getRequestURI: /WebDemo/request = getRequestURL: - - -http://localhost:8080/WebDemo/request
-
URI: Uniform Resource Identifier 统一资源标识符
-
URL: Uniform Resource Locator 统一资源定位器
演示请求字符串
package net.xyx.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:输出请求行的相关信息
* 作者:xyx
* 日期:2023年04月14日
*/
@WebServlet(name = "RequestLineServlet", urlPatterns = "/request")
public class RequestLineServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取并输出请求行的相关信息
out.println("getMethod: " + request.getMethod() + "<br />");
out.println("getRequestURI: " + request.getRequestURI() + "<br />");
out.println("getQueryString: " + request.getQueryString() + "<br />");
out.println("getProtocol: " + request.getProtocol() + "<br />");
out.println("getContextPath: " + request.getContextPath() + "<br />");
out.println("getPathInfo: " + request.getPathInfo() + "<br />");
out.println("getPathTranslated: " + request.getPathTranslated() + "<br />");
out.println("getServletPath: " + request.getServletPath() + "<br />");
out.println("getRemoteAddr: " + request.getRemoteAddr() + "<br />");
out.println("getRemoteHost: " + request.getRemoteHost() + "<br />");
out.println("getRemotePort: " + request.getRemotePort() + "<br />");
out.println("getLocalAddr: " + request.getLocalAddr() + "<br />");
out.println("getLocalName: " + request.getLocalName() + "<br />");
out.println("getLocalPort: " + request.getLocalPort() + "<br />");
out.println("getServerName: " + request.getServerName() + "<br />");
out.println("getServerPort: " + request.getServerPort() + "<br />");
out.println("getScheme: " + request.getScheme() + "<br />");
out.println("getRequestURL: " + request.getRequestURL() + "<br />");
// 在控制台输出请求字符串的信息
if (request.getQueryString() != null) {
String strQuery = request.getQueryString();
String[] queries = strQuery.split("&");
for (String query : queries) {
String[] fields = query.split("=");
System.out.println(fields[0] + " : " + fields[1]);
}
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- 重启tomcat服务器,访问
http://localhost:8080/WebDemo/request?username=howard&password=903213
还可以将查询字符串继续按=
进行拆分
(二)获取请求头的相关方法
- 目标:掌握使用
HttpServletRequest
接口获取HTTP请求头字段的方法
1、相关方法
方法声明 | 功能描述 |
---|---|
String getHeader(String name) | 该方法用于获取一个指定头字段的值,如果请求消息中没有包含指定的头字段,getHeader()方法返回null;如果请求消息中包含有多个指定名称的头字段,getHeader()方法返回其中第一个头字段的值 |
Enumeration getHeaders(String name) | 该方法返回一个Enumeration集合对象,该集合对象由请求消息中出现的某个指定名称的所有头字段值组成。在多数情况下,一个头字段名在请求消息中只出现一次,但有时候可能会出现多次 |
Enumeration getHeaderNames() | 该方法用于获取一个包含所有请求头字段的Enumeration对象 |
int getIntHeader(String name) | 该方法用于获取指定名称的头字段,并且将其值转为int类型。需要注意的是,如果指定名称的头字段不存在,返回值为-1;如果获取到的头字段的值不能转为int类型,将发生NumberFormatException异常 |
long getDateHeader(String name) | 该方法用于获取指定头字段的值,并将其按GMT时间格式转换成一个代表日期/时间的长整数,这个长整数是自1970年1月1日0点0分0秒算起的以毫秒为单位的时间值 |
String getContentType() 该方法用于获取Content-Type头字段的值,结果为String类型 | |
int getContentLength() | |
String getCharacterEncoding() | 该方法用于返回请求消息的实体部分的字符集编码,通常是从Content-Type头字段中进行提取,结果为String类型 |
2、案例演示
- 在net.xyx.request包里创建
RequestHeadersServlet
类
package net.xyx.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Enumeration;
/**
* 功能:演示获取请求头的信息
* 作者:xyx
* 日期:2023年04月14日
*/
@WebServlet(name = "RequestHeaderServlet", urlPatterns = "/header")
public class RequestHeaderServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取请求头名枚举对象
Enumeration<String> headerNames = request.getHeaderNames();
// 遍历所有请求头,并通过getHeader()方法获取一个指定名称的头字段
while (headerNames.hasMoreElements()) {
// 获取头字段名称
String headerName = headerNames.nextElement();
// 输出头字段名称及其值
out.println(headerName + " : " + request.getHeader(headerName) + "<br />");
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
重启tomcat服务器,访问
http://localhost:8080/WebDemo/header
-
按F12键,通过开发者工具查看请求头信息
(三)请求转发
- 目标:掌握使用HttpServletRequest接口将请求转发
1、getRequestDispatcher()方法
- Servlet之间可以相互跳转,利用Servlet的跳转可以很容易地把一项任务按模块分开,例如,使用一个Servlet实现用户登录,然后跳转到另外一个Servlet实现用户资料修改。Servlet的跳转要通过
RequestDispatcher
接口的实例对象实现。HttpServletRequest
接口提供了getRequestDispatcher()
方法用于获取RequestDispatcher
对象,getRequestDispatcher()
方法的具体格式:RequestDispatcher getRequestDispatcher(String path)
getRequestDispatcher()
The method returns anRequestDispatcher
object that encapsulates the resource specified by a path. Among them, the parameter path must start with "/" and is used to represent the root directory of the current web application. It should be noted that the contents in the WEB-INF directory are also visible toRequestDispatcher
objects. Therefore, the resource passed to thegetRequestDispatcher(String path)
method can be a file in the WEB-INF directory.
2. forward() method
- After obtains
RequestDispatcher
the object, if the current Web resource does not want to process the request, theRequestDispatcher
interface provides a forward() method, which can forward the current The request is passed to other Web resources to process the information and respond to the client. This method is called request forwarding. The specific format of the forward() method:forward(ServletRequest request,ServletResponse response)
forward()
The method is used to pass a request from oneServlet
to another web resource. InServlet
, you can perform preliminary processing on the request, and then pass the request to other resources for response by calling the forward() method. It should be noted that this method must be called before the response is submitted to the client, otherwise an exception will be thrown. When the browser accesses Servlet1, the request can be forwarded to other Web resources through the method. After other Web resources process the request, the response result is directly returned to browser.IllegalStateException
forward()
3. Case demonstration request forwarding
CreateRequestForwardServlet
class in net.xyx.request package, save the data in request
object, and then forward it to another Servlet
to handle
package net.xyx.request;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* 功能:演示请求转发
* 作者:xyx
* 日期:2023年03月13日
*/
@WebServlet(name = "RequestForwardServlet", urlPatterns = "/forward")
public class RequestForwardServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html;charset=utf-8");
// 设置请求对象属性
request.setAttribute("message", "欢迎访问泸州职业技术学院~");
// 获取请求派发器对象(参数是请求转发的Servlet的url)
RequestDispatcher dispatcher = request.getRequestDispatcher("/result");
// 请求转发给url为`/result`的Servlet
dispatcher.forward(request, response);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
CreateResultServlet
class in net.xyx.request package, used to obtain RequestForwardServlet
stored in request
Data in the object and output
package net.xyx.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:处理转发的请求
* 作者:xyx
* 日期:2023年03月13日
*/
@WebServlet(name = "ResultServlet", urlPatterns = "/result")
public class ResultServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html;charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取请求转发保存在request对象里的数据
String message = (String) request.getAttribute("message");
// 输出获取的信息
if (message != null) {
out.println("转发来的消息:" + message);
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
Correspondence diagram
Restart the tomcat server and visit http://localhost:8080/WebDemo/forward
Note: The request path of RequestForwardServlet is still displayed in the address bar, but the browser displays the content to be output in ResultServlet. This is because request forwarding is a behavior that occurs within the server. From RequestForwardServlet to ResultServlet, it is a request. In a request, the request attribute can be used for data sharing (same request forwarding).
(4) Obtain request parameters
Objective: Master the use of HttpServletRequest interface to obtain request parameters
1. Related methods
Method declaration function description
String getParameter(String name) This method is used to obtain the parameter value of a specified name. If the request message does not contain a parameter with the specified name, the getParameter() method returns null. ; If the parameter with the specified name exists but no value is set, an empty string is returned; if the request message contains multiple parameters with the specified name, the getParameter() method returns the first parameter value that appears
String[] getParameterValues(String name) This method is used to return an array of String type. There can be multiple parameters with the same name in the HTTP request message (usually generated by a form containing multiple field elements with the same name). ), if you want to obtain all parameter values corresponding to the same parameter name in the HTTP request message, you should use the getParameterValues() method
Enumeration getParameterNames() This method is used to return a request message containing Enumeration object of all parameter names in the request message. On this basis, all parameters in the request message can be traversed.
Map getParameterMap() This method is used to get all parameter names and values in the request message. Load into a Map object and return
2. Case demonstration
Create a registration page - register.html
username: | |
password: | |
gender: | male female |
interest: | Watch movies, code, play games |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
- Create the
RquestParamsServlet
class in the net.xyx.request package
package net.xyx.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:获取请求参数
* 作者:XYX
* 日期:2023年04月14日
*/
@WebServlet(name = "RequestParamsServlet", urlPatterns = "/register")
public class RequestParamsServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取注册表单提交的数据
String username = request.getParameter("username");
String password = request.getParameter("password");
String gender = request.getParameter("gender");
String[] interests = request.getParameterValues("interest");
// 输出获取的表单数据
out.println("姓名:" + username + "<br />");
out.println("密码:" + password + "<br />");
out.println("性别:" + gender + "<br />");
out.print("兴趣:");
for (int i = 0; i < interests.length; i++) {
out.println(interests[i]);
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
Correspondence diagram
Restart the tomcat server, visithttp://localhost:8080/WebDemo/register.html
, and fill in the form data
Click the [Register] button
(5) Solve the problem of Chinese garbled request parameters
-
Goal: Master how to solve Chinese garbled request parameters
-
In the
HttpServletRequest
interface, asetCharacterEncoding()
method is provided, which is used to set the decoding method of therequest
object -
Modify
ResquestParamsServlet
class, add a line of code, and set the character encoding of the request object
-
Restart the tomcat server, visit
http://localhost:8080/WebDemo/register.html
, and fill in the form data
-
Click the [Register] button and there will be no Chinese garbled characters on the page.
(6) Passing data through the Request object
- Goal: Master the use of ServletRequest interface to operate attributes
1. Methods for operating attributes of the Request object
(1) setAttribute() method
setAttribute()
The method is used to associate an object with aname
and store it into anServletRequest
object. Its complete declaration:public void setAttribute(String name,Object o)
;
setAttribute()
The first parameter of the parameter list of the method receives a name of type String, and the second parameter receives an object o of type Object. It should be noted that if the attribute with the specified name already exists in theServletRequest
object, thesetAttribute()
method will first delete the original attribute and then add the new attribute. If the attribute value object passed to thesetAttribute()
method is null, the attribute with the specified name is deleted. The effect is the same as theremoveAttribute()
method.
(2) getAttribute() method
getAttribute()
The method is used to return the attribute object with the specified name from theServletRequest
object. Its complete declaration:public Object getAttribute(String name)
;
(3) removeAttribute() method
removeAttribute()
The method is used to delete the attribute with the specified name from theServletRequest
object. Its complete declaration:public void removeAttribute(String name)
;
(4) getAttributeNames() method
getAttributeNames()
The method is used to return an object containing all the attribute names in theServletRequest
object. On this basis, you can < a i=3>All attributes in the object are traversed. Its complete statement: ; It should be noted that only data belonging to the same request can pass data through the object.Enumeration
ServletRequest
public Enumeration getAttributeNames()
ServletRequest
2. Case demonstration of transferring data through Request object
CreateRquestServlet01
class in net.xyx.request package
package net.xyx.request;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* 功能:设置请求对象属性,请求转发
* 作者:xyx
* 日期:2023年04月14日
*/
@WebServlet(name = "RequestServlet01", urlPatterns = "/request01")
public class RequestServlet01 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 设置请求对象属性
request.setAttribute("id", "20210201");
request.setAttribute("name", "陈燕文");
request.setAttribute("gender", "女");
request.setAttribute("age", "18");
request.setAttribute("major", "软件技术专业");
request.setAttribute("class", "2021级软件2班");
request.setAttribute("phone", "15890903456");
// 获取请求派发器对象(参数是请求转发的Servlet的url)
RequestDispatcher dispatcher = request.getRequestDispatcher("/request02");
// 请求转发给url为`/request02`的Servlet
dispatcher.forward(request, response);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- inside
net.xyx.request
packing village buildingRquestServlet02
class
package net.xyx.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Enumeration;
/**
* 功能:获取请求转发的数据
* 作者:xyx
* 日期:2023年04月14日
*/
@WebServlet(name = "RequestServlet02", urlPatterns = "/request02")
public class RequestServlet02 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取请求对象属性名枚举对象
Enumeration<String> attributes = request.getAttributeNames();
// 获取请求转发保存在request对象里的数据并输出
while (attributes.hasMoreElements()) {
// 获取属性名
String attribute = attributes.nextElement();
// 输出属性名及其值
out.println(attribute + " : " + request.getAttribute(attribute) + "<br />");
}
// 删除请求对象的属性
while (attributes.hasMoreElements()) {
// 获取属性名
String attribute = attributes.nextElement();
// 删除属性
request.removeAttribute(attribute);
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
-
Start the server and access
http://localhost:8080/WebDemo/request01
-
Modify RequestServlet02 to only display student information
package net.huawei.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
/**
* 功能:获取请求转发的数据
* 作者:xyx
* 日期:2023年04月14日
*/
@WebServlet(name = "RequestServlet02", urlPatterns = "/request02")
public class RequestServlet02 extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 设置响应体内容类型
response.setContentType("text/html; charset=utf-8");
// 获取字符输出流
PrintWriter out = response.getWriter();
// 获取请求转发保存在request对象里的数据并输出
out.println("学号:" + request.getAttribute("id") + "<br />");
out.println("姓名:" + request.getAttribute("name") + "<br />");
out.println("性别:" + request.getAttribute("gender") + "<br />");
out.println("年龄:" + request.getAttribute("age") + "<br />");
out.println("专业:" + request.getAttribute("major") + "<br />");
out.println("班级:" + request.getAttribute("class") + "<br />");
out.println("手机:" + request.getAttribute("phone") + "<br />");
// 删除请求对象的属性
request.removeAttribute("id");
request.removeAttribute("name");
request.removeAttribute("gender");
request.removeAttribute("age");
request.removeAttribute("major");
request.removeAttribute("class");
request.removeAttribute("phone");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
}
- Start the server and access
http://localhost:8080/WebDemo/request01