Snake War
If you want to improve your programming skills, you need to type more code. Writing a small game yourself is a very good way to practice. Next, let’s complete a classic game together
贪吃蛇大作战
, experience in practice向对象的优点
Game flow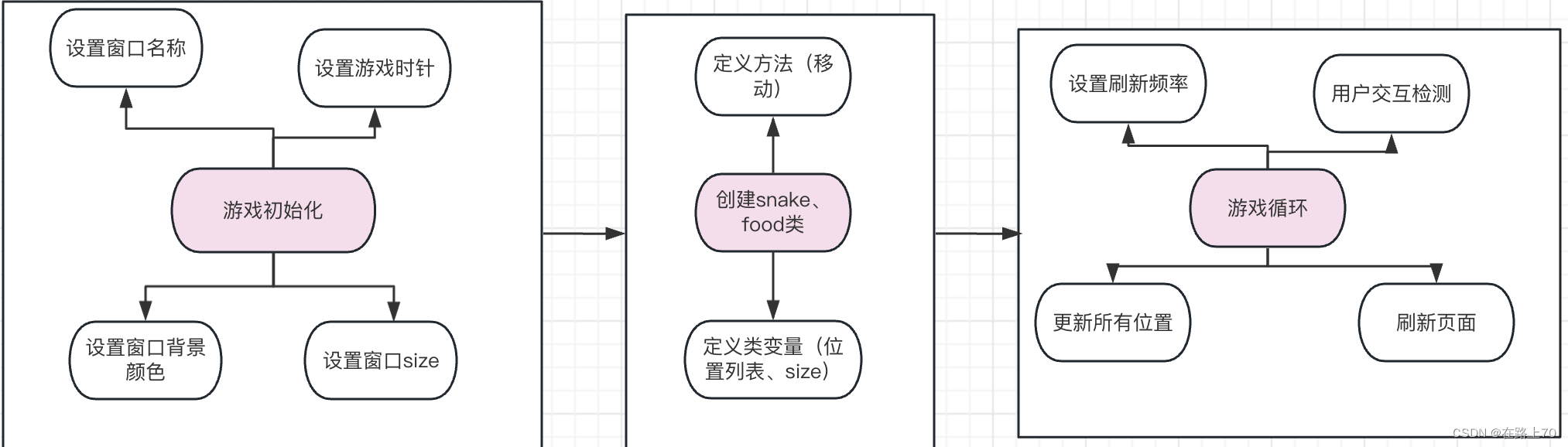
1.Initialize the game window
# 初始化 Pygame
pygame.init()
# 设置游戏界面大小、背景颜色和游戏标题
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption('贪吃蛇')
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
snake_size = 20
# 定义一个计时器来控制蛇的移动速度
clock = pygame.time.Clock()
2. Define related classes
Define snake class
class snake(object):
# 初始化蛇的初始位置、大小和速度
def __init__(self):
self.snake_x = 100
self.snake_y = 100
self.snake_size = 20
self.snake_speed = 5
# 定义蛇的移动方向
snake_direction = 'right'
# 定义一个列表来保存蛇的身体坐标
snake_body = []
def move_(self, snake_direction):
if snake_direction == 'up':
self.snake_y -= self.snake_speed
elif snake_direction == 'down':
self.snake_y += self.snake_speed
elif snake_direction == 'left':
self.snake_x -= self.snake_speed
elif snake_direction == 'right':
self.snake_x += self.snake_speed
self.snake_direction=snake_direction
self.snake_body.insert(0, [self.snake_x, self.snake_y])
Every time you move a step, add the new head coordinates to snake_body[0], and there will be an update body operation later.
Define food class
# 定义食物的大小
food_size = 20
#定义食物类
class food(object):
def __init__(self,food_x,food_y):
self.food_x = food_x
self.food_y = food_y
#检测食物是否被吃
def ate(self, snake):
snake_x = snake.snake_x
snake_y = snake.snake_y
food_x = self.food_x
food_y = self.food_y
if (snake_x == food_x and snake_y == food_y) or (snake_x == food_x and abs(snake_y - food_y) < snake_size) or (
snake_y == food_y and abs(snake_x - food_x) < snake_size):
self.food_x = random.randrange(0, screen_width - snake_size, 10)
self.food_y = random.randrange(0, screen_height - snake_size, 10)
snake.snake_body.append([snake_x, snake_y])
3 situations when a snake eats food
- The snake head coordinates coincide with the food coordinates (snake_x == food_x and snake_y == food_y)
- The snake touches the food from the left and right sides (snake_x == food_x and abs(snake_y - food_y) < snake_size)
- The snake touches the food from the upper and lower sides snake_y == food_y and abs(snake_x - food_x) < snake_size)
3. The main part of the game
1. Define a draw function
# 定义一个函数来绘制蛇和食物
def draw(snake, food):
screen.fill(BLACK)
for pos in snake.snake_body:
pygame.draw.rect(screen, GREEN, [pos[0], pos[1], snake_size, snake_size])
print(food.food_x, food.food_y)
pygame.draw.rect(screen, RED, [food.food_x, food.food_y, food_size, food_size])
pygame.display.update()
At the beginning of the function, screen.fill(BLACK) must be performed to clear the entire window, because pygame.display.update() updates based on the original and will retain traces of snakes and food.
2.main() function
# 主循环
def main(snake,food):
while True:
snake_direction = snake.snake_direction
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 退出游戏
pygame.quit()
quit()
# 处理按键事件
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake_direction = 'up'
elif event.key == pygame.K_DOWN:
snake_direction = 'down'
elif event.key == pygame.K_LEFT:
snake_direction = 'left'
elif event.key == pygame.K_RIGHT:
snake_direction = 'right'
# 移动蛇的头部
snake.move_( snake_direction)
# 判断是否吃到食物
food.ate(snake)
# 更新蛇的身体坐标
if len(snake.snake_body) > 1:
snake.snake_body.pop()
# 判断游戏是否结束
snake_x = snake.snake_x
snake_y = snake.snake_y
food_x = food.food_x
food_y = food.food_y
snake_body = snake.snake_body
if snake_x < 0 or snake_x > screen_width - snake_size or snake_y < 0 or snake_y > screen_height - snake_size or [
snake_x, snake_y] in snake_body[1:]:
# 游戏结束,显示分数并等待退出
font = pygame.font.Font(None, 36)
text = font.render('Score: ' + str(len(snake_body)), True, WHITE)
screen.blit(text, ((screen_width - text.get_width()) / 2, (screen_height - text.get_height()) / 2))
pygame.display.update()
pygame.time.wait(2000)
pygame.quit()
quit()
# 绘制蛇和食物
draw(snake, food)
# 控制蛇的移动速度
clock.tick(20)
Two situations when the game ends
1. Snake hits the wall (snake_x < 0 or snake_x > screen_width - snake_size or snake_y < 0 or snake_y > screen_height - snake_size) < /span>
2. Snake eats itself ([ snake_x, snake_y] in snake_body[1:])
4. Results display
到这里游戏以及圆满完成了,来看看运行效果
Complete code
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏界面大小、背景颜色和游戏标题
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption('贪吃蛇')
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
snake_size = 20
# 定义一个计时器来控制蛇的移动速度
clock = pygame.time.Clock()
class snake(object):
# 初始化蛇的初始位置、大小和速度
def __init__(self):
self.snake_x = 100
self.snake_y = 100
self.snake_size = 20
self.snake_speed = 5
# 定义蛇的移动方向
snake_direction = 'right'
# 定义一个列表来保存蛇的身体坐标
snake_body = []
def move_(self, snake_direction):
if snake_direction == 'up':
self.snake_y -= self.snake_speed
elif snake_direction == 'down':
self.snake_y += self.snake_speed
elif snake_direction == 'left':
self.snake_x -= self.snake_speed
elif snake_direction == 'right':
self.snake_x += self.snake_speed
self.snake_direction=snake_direction
self.snake_body.insert(0, [self.snake_x, self.snake_y])
# 定义食物的大小
food_size = 20
#定义食物类
class food(object):
def __init__(self,food_x,food_y):
self.food_x = food_x
self.food_y = food_y
def ate(self, snake):
snake_x = snake.snake_x
snake_y = snake.snake_y
food_x = self.food_x
food_y = self.food_y
if (snake_x == food_x and snake_y == food_y) or (snake_x == food_x and abs(snake_y - food_y) < snake_size) or (
snake_y == food_y and abs(snake_x - food_x) < snake_size):
self.food_x = random.randrange(0, screen_width - snake_size, 10)
self.food_y = random.randrange(0, screen_height - snake_size, 10)
snake.snake_body.append([snake_x, snake_y])
# 定义一个函数来绘制蛇和食物
def draw(snake, food):
screen.fill(BLACK)
for pos in snake.snake_body:
pygame.draw.rect(screen, GREEN, [pos[0], pos[1], snake_size, snake_size])
print(food.food_x, food.food_y)
pygame.draw.rect(screen, RED, [food.food_x, food.food_y, food_size, food_size])
pygame.display.update()
# 主循环
def main(snake,food):
while True:
snake_direction = snake.snake_direction
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 退出游戏
pygame.quit()
quit()
# 处理按键事件
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake_direction = 'up'
elif event.key == pygame.K_DOWN:
snake_direction = 'down'
elif event.key == pygame.K_LEFT:
snake_direction = 'left'
elif event.key == pygame.K_RIGHT:
snake_direction = 'right'
# 移动蛇的头部
snake.move_( snake_direction)
print(1)
# 判断是否吃到食物
food.ate(snake)
print(2)
# 更新蛇的身体坐标
if len(snake.snake_body) > 1:
snake.snake_body.pop()
# 判断游戏是否结束
snake_x = snake.snake_x
snake_y = snake.snake_y
food_x = food.food_x
food_y = food.food_y
snake_body = snake.snake_body
if snake_x < 0 or snake_x > screen_width - snake_size or snake_y < 0 or snake_y > screen_height - snake_size or [
snake_x, snake_y] in snake_body[1:]:
# 游戏结束,显示分数并等待退出
font = pygame.font.Font(None, 36)
text = font.render('Score: ' + str(len(snake_body)), True, WHITE)
screen.blit(text, ((screen_width - text.get_width()) / 2, (screen_height - text.get_height()) / 2))
pygame.display.update()
pygame.time.wait(2000)
pygame.quit()
quit()
# 绘制蛇和食物
print(3)
draw(snake, food)
# 控制蛇的移动速度
clock.tick(20)
if __name__ == "__main__":
main( snake(),food(random.randrange(0, screen_width - snake_size, 20),random.randrange(0, screen_width - snake_size, 20)))
感谢你的观看,如果对你有帮助的话,请点个赞哦,最后,祝我们一起迈向更高的目标,共同追求卓越与进步!