Article directory
1. Title
Design a function to add two numbers. +
or other arithmetic operators may not be used.
Example:
Input: a = 1, b = 1
Output: 2
hint:
a
,b
may be negative or 0- The result will not overflow as a 32-bit integer
Click here to jump to the question.
2. C# problem solution
Perform binary addition of a and b, ai and bi represent the value of the i-th bit of a and b (0 or 1), and ci represents the carry of the i-th bit (0 or 1). Use ans to represent the calculation result. In the initial case, all bits of ans are 0.
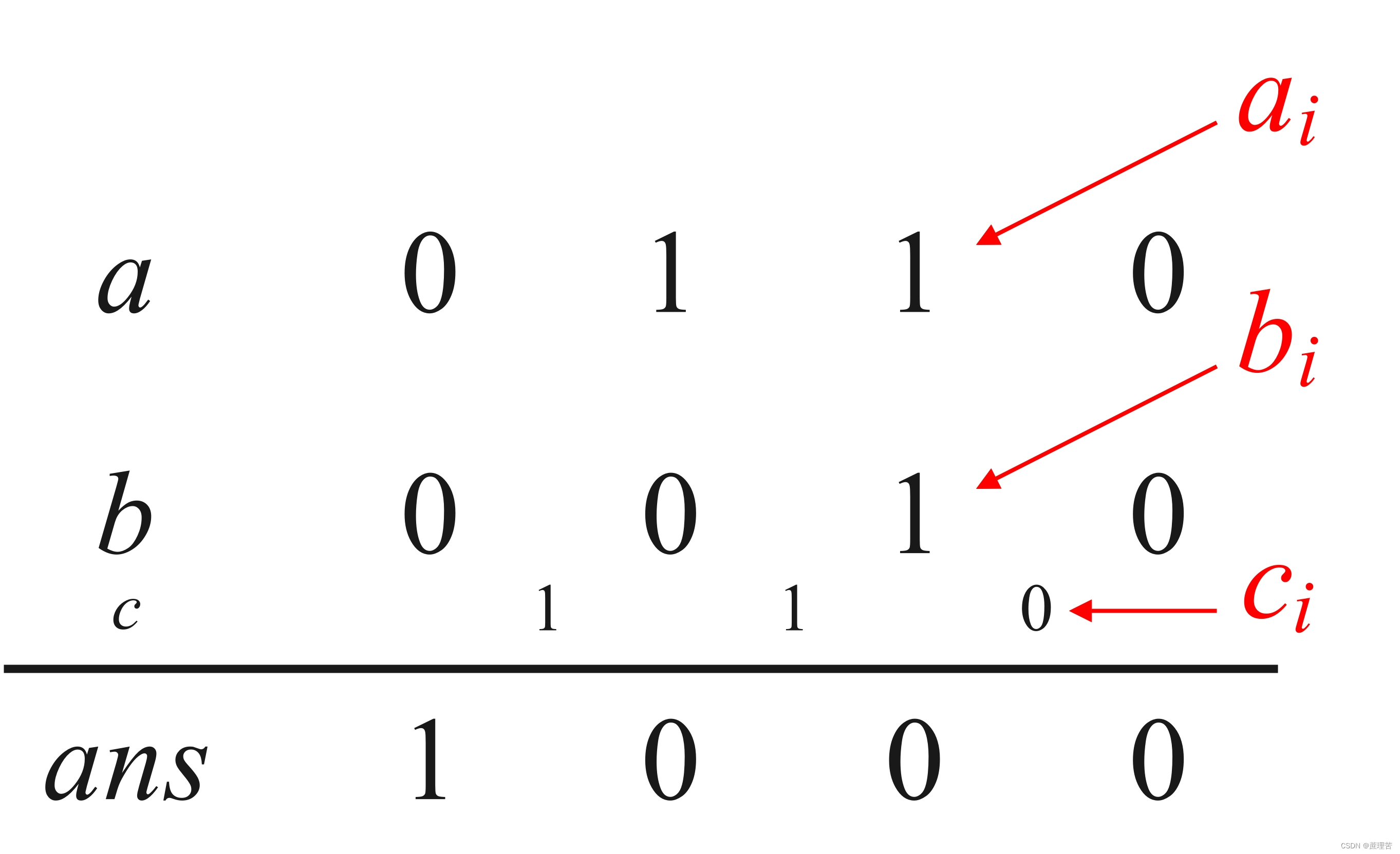
- ci = 0
- ai = bi: ai and bi are either 0 or 1, so the result of the bit after addition is 0, and ans does not process it.
- ai = bi = 0, then the bit is carried to 0 after calculation;
- ai = bi = 1, then the bit is carried by 1 after calculation.
Therefore ci = ai.
- ai != bi: One of ai and bi is 0, and the other is 1. There will be no carry after adding. Considering ci = 0, ci is not processed, and the position of ans is 1.
- ai = bi: ai and bi are either 0 or 1, so the result of the bit after addition is 0, and ans does not process it.
- ci = 1
- ai = bi: ai is either 0 or 1, so the bit result after addition is 1, set ans to 1.
- ai = bi = 0, then the bit is carried to 0 after calculation;
- ai = bi = 1, then the bit is carried by 1 after calculation.
Therefore ci = ai.
- ai != bi: One of ai and bi is 0, and the other is 1, so the bit results after addition are both 0, and a carry is generated. Therefore ans is not processed and ci becomes 1. Considering that the premise of this branch is that ci is already 1, this situation can be skipped.
- ai = bi: ai is either 0 or 1, so the bit result after addition is 1, set ans to 1.
public class Solution {
public int Add(int a, int b) {
int ai, bi, ci = 0, i = 1, ans = 0;
do {
ai = (a & i) == 0 ? 0 : 1; // 获取 a 的每一位
bi = (b & i) == 0 ? 0 : 1; // 获取 b 的每一位
if (ci == 0) {
// 进位为 0
if (ai == bi) ci = ai;
else ans |= i;
}
else if (ai == bi) {
// 进位为 1
ci = ai;
ans |= i;
}
i <<= 1; // 左移 i
} while (i != 0);
return ans;
}
}
- Time: 20 ms, beats 77.78% of users using C#
- Memory: 25.95 MB, beats 94.44% of users using C#