The main discussion is how integers and floating point types are stored in memory.
1. Integer and floating point types
integer family
char
unsigned char
signed char
short
unsigned short [int]
signed short [int]
int
unsigned int
signed int
long
unsigned long [int]
signed long [int]
long long
unsigned long long
signed long long
floating point family
float
double
This blog is completed under the vs2019 compiler, which is a little-endian storage method.
Second, first introduce the big and small endian storage methods
Introduction to big and small endian
What is big endian and little endian:
Big-endian (storage) mode means that the low-order bits of data are stored in the high address of the memory, and the high-order bits of the data are stored in the low address of the memory.
middle;
little endian (storage) mode means that the low bits of the data are stored in the low address of the memory, and the high bits of the data
,
are saved In the highlands of memory
address.
Take 0x11223344 in hexadecimal as an example:
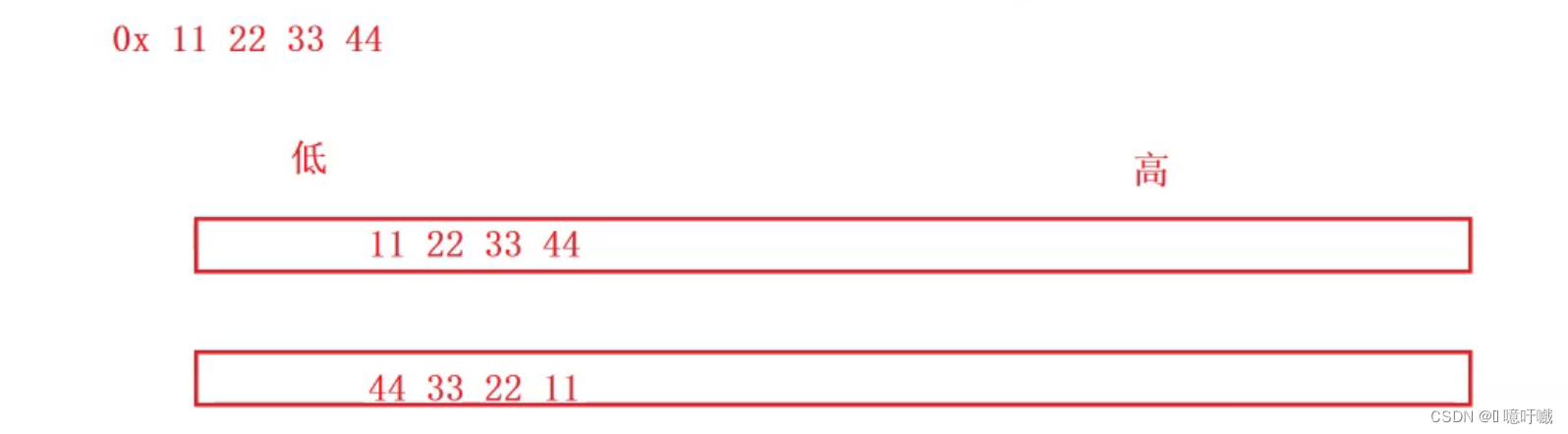
Why are there big endian and little endian:
Why are there big and small endian modes? This is because in computer systems, we measure bytes, and each address unit
corresponds to a byte, and a byte is
8 bits
. But in
C
language except
8 bit
< In addition to a i=7>char
, there is also16 bitshort
type,
32 bit
long type (required (See the specific compiler). In addition, for processors with more than 8 bits, such as 16 a> bits or 32
bit processor, since the register width is larger than one byte, there must be a problem of how to arrange multiple bytes. because
This leads to big-endian storage mode and little-endian storage mode.
Example: One piece
16bit
type
short
type
0x11 为 ,nan 0x1122 の值为 x , 0x0010 , existing central location
x
is the high byte,
0x22
is the low byte. For big-endian mode, put
0x11
in the low address, that is,
0x0010
,
0x22
is placed high
address, that is,
0x0011
. Little endian mode is just the opposite. Our commonly used
X86
structure is little endian mode, while
KEIL C51
is big-endian mode. Many
ARM
and
DSP
are in little-endian mode. Some
ARM
processors can also be selected by hardware to be in big-endian mode
Or little endian mode.
You can determine the big and small endianness through the following code
#include <stdio.h>
int main()
{
int i = 1;
if (*(char*)&i == 1)
{
printf("小端\n");
}
else
{
printf("大端\n");
}
return 0;
}
3. Storage of integers in memory
Original code, inverse code, complement code
There are three ways of expressing integers in computers
2
in base code, namely original code, complement code and complement code.
Three types of display methods are uniform
code position
sum
number position
Two parts, code position is used
0
display
“
correct
”
, for
1
display
“
default
”
, the number is
The original, inverse, and complement codes of positive numbers are the same.
There are three ways to represent negative integers in different ways.
Original code
The original code can be obtained by directly translating the value into binary in the form of positive and negative numbers.
reverse code
The one's complement code can be obtained by keeping the sign bit of the original code unchanged and inverting the other bits bit by bit.
complement
rebuttal
+1
obtainable result.
At the same time, the original code can be obtained by inverting -1 and then inverting, or by inverting and then +1.
For shaping: the data stored in the memory actually stores the complement code.
why?
In computer systems, numerical values are always represented and stored using two's complement codes. The reason is that using two's complement codes, the sign bit and the numerical domain can be unified
is processed in one process; at the same time, addition and subtraction can also be processed in a unified manner (CPU
has only adders
) In addition, the operation process of converting complement code and original code is the same, and no additional hardware circuit is required.
4. Signed and unsigned integers
At the same time, for integer types, there are unsigned and signed types. For char, the range of unsigned char is 0~255, and the range of signed char is -128~127.
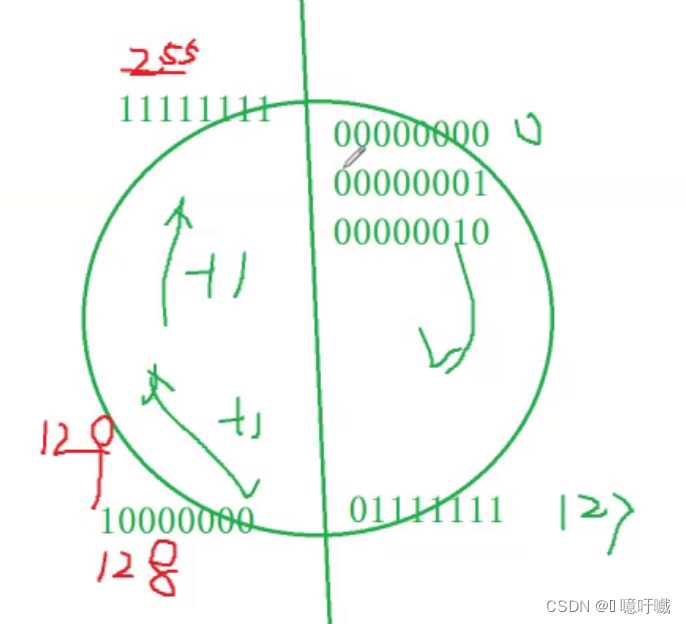
Five, Floating point number storage rules
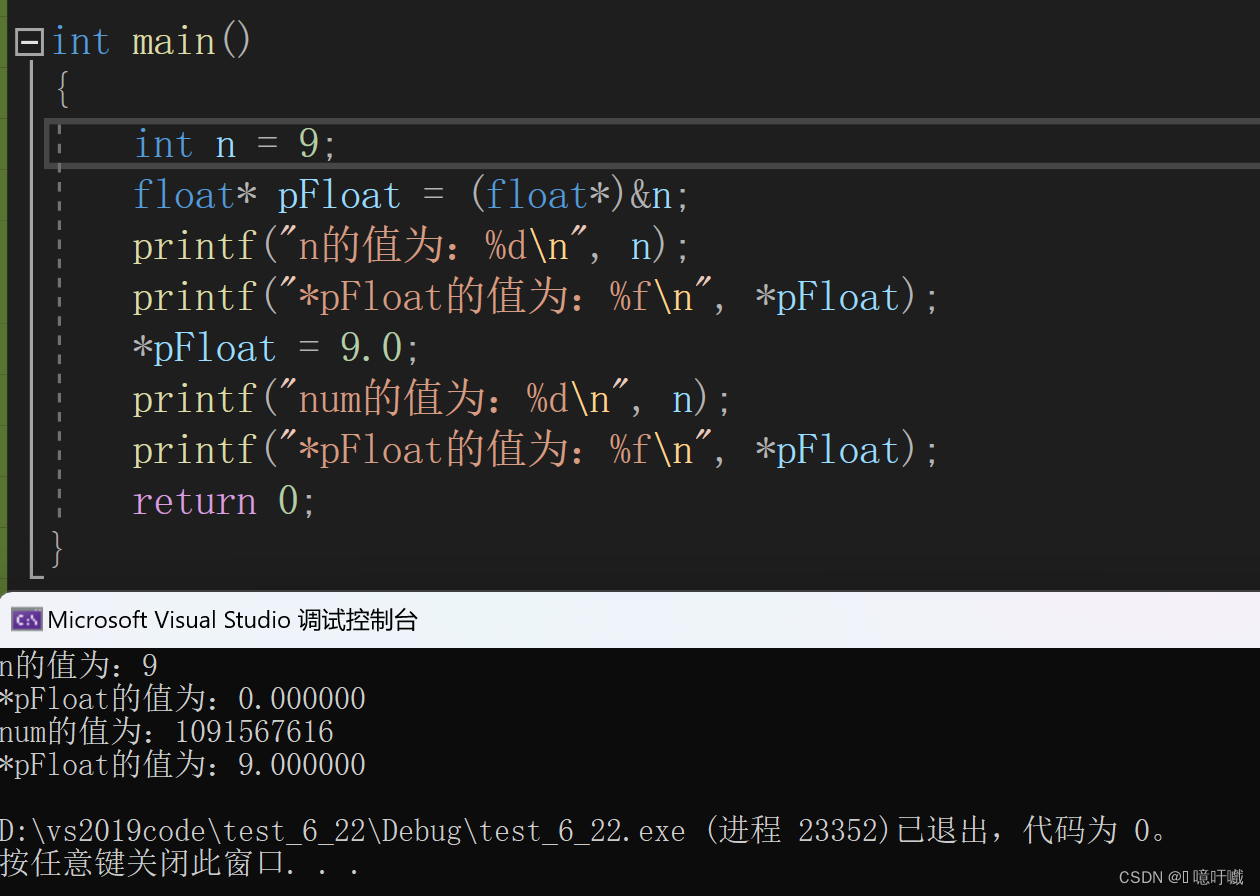
From this code, it can be seen that the reading methods and storage rules of integer and floating-point types are different. So, what is the storage method of floating-point types?
According to international standards
IEEE
(Institute of Electrical and Electronics Engineers)
754
, any binary floating point number
V
can be expressed in the following form:
(-1)^S * M * 2^E
(-1)^S
Display code position, current
S=0
,
Number of charges. positive number; 8>V
V
M
Display effective digits, Daiyu etc.
1
, Xiaoyu
2
.
2^E
represents the exponent bit.
for example:
Decadal system
5.0
, Copying two system system
101.0
, Equivalent to
1.01×2^2
.
な么、按涉上面
V
のqual form,可以输入
S=0
,
M=1.01
,
E=2
.
IEEE 754
Description:
对于
32
Positive floating point number, highest
1
Position code position
S
, adhesive
8
position index< /span>
Effective number with rank23,剩下
E
.
对于
64
Number of floating points, highest
1
Position code S, adhesive
11
Position index
E
,剩下的
52
ranking effective numeral
M
.
IEEE 754
effective digit
M
sum index
E
,There are some special considerations.
As mentioned before,
1≤M<2
, that is to say,
M
represents the decimal part. xxxxxx, where 1.xxxxxx
can be written in the form of
IEEE 754
stipulates that when saving
M
inside the computer, the first digit of this number will be defaulted It is always
1
, so it can be discarded and only the following xxxxxx part is saved. For example, when saving
1.01
, only 01
is saved. When reading, the first < /span>
1 significant figure. Add it. The purpose of this is to save
1
As for the index
E
, the situation is more complicated.
first,
E
each unsigned int (
unsigned int
)
This means that if
E
is
8
bits , its value range is
0~255
; if
E
is
11
bits, its value range is
0~2047
. However, we know that E
in scientific notation can appear as a negative number, so IEEE 754
stipulates that when stored in memory
must be added to an intermediate number, for 8 digits E, the middle number is 127; for11bitE, the middle number is 1023.
E
Insufficiency
0
Or insufficiency
1 < /span>
At this time, the floating point number is represented by the following rules, that is, the calculated value of the exponent
E
minus
127
(or
1023
), get the true value, and then
Significant digits
M
preceded by the first digit
1
.
E
entire
0
At this time, floating point numerical index
E
etc.
1-127
(Someone
1-1023
) Immediately true,
Significant digits
M
No more first digits
1
, but is restored to the decimal value of
0.xxxxxx
. This is done to represent
±0
, and to be close to
A very small number of 0
.
E
entire
1
At this time, the effective number
M
Total
0
Display ;
In this way, the problem just now will be easily solved.
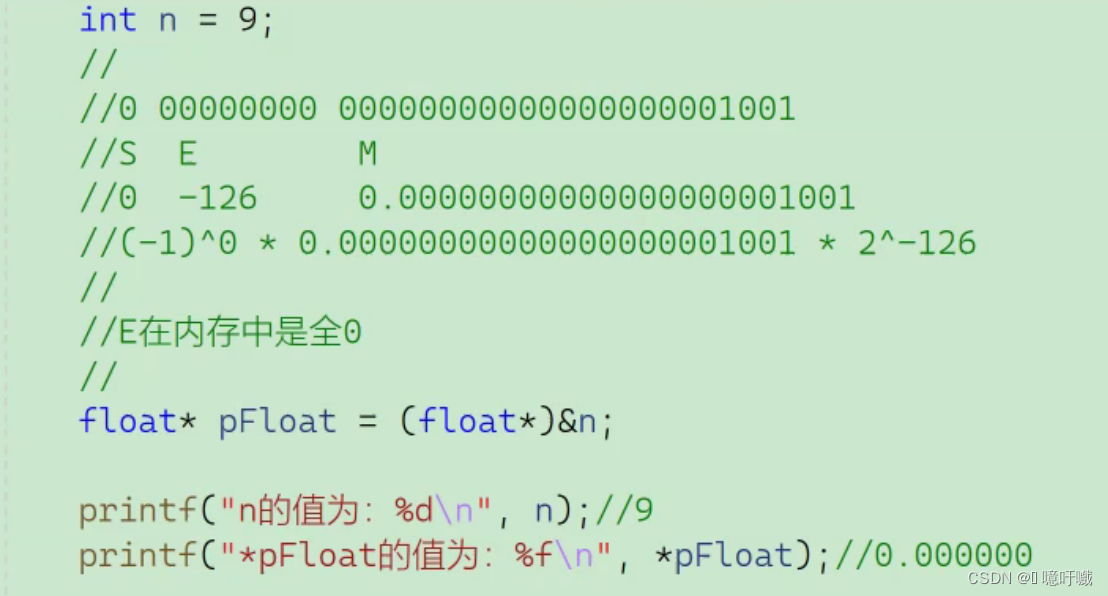