Knowledge point one: while loop
1) while (test condition)
cyclical behavior
#include <stdio.h>
int main()
{
int i = 1, sum = 0;
while(i <= 100)
{
sum = i + sum;
i++;
}
printf("%d %d\n", i, sum);
return 0;
}
Explanation: int i = 1, sum = 0; declare variables i and sum. and initialized to 1 and 0.
The test condition of the while statement is i <= 100. As long as i is in the range from 1 to 100 and the expression is true, the loop can be entered.
Within the loop, i + sum is calculated, the result is assigned to sum, and i will increase by 1 each time.
When i is 1, the expression 1 <= 100 is true and the loop can be entered.
The value of sum is 1 + 0. i becomes 2.
When i is 2, the expression 2 <= 100 is true and the loop can be entered.
The value of sum is 2 + (1 + 0). i becomes 3.
When i is 3, the expression 3 <= 100 is true and the loop can be entered.
The value of sum is 3 + (2 + 1 + 0). i becomes 4.
Until the value of i is 101, the expression 101 <= 100 is false, the test condition is not established, and the loop stops.
Knowledge point 2: True and false in C language
1) During the expression evaluation process: true will be represented by 1, false will be represented by 0;
2) So what if we deliberately change the test condition result to a value other than 1 or 0?
#include <stdio.h>
int main()
{
while(2)
{
printf("Hello");
}
return 0;
}
# while treats 2 as true and loops an infinite number of times.
3) In C language: non-zero means true;
Tip: If your program is stuck in an infinite loop, or you want to interrupt the execution of the program, you can press the key combination Ctrl + C to stop the program;
Knowledge point 3: 3 conditions for correct limited number of loops
#include <stdio.h>
int main()
{
int i = 1, sum = 0;
while(i <= 100)
{
sum = i + sum;
i++;
}
printf("%d %d\n", i, sum);
return 0;
}
1) In the above summation code, we first set the counter i to 1;
2) Then, i is compared with a finite value as the loop condition;
3) And, the counter will change every time it loops;
4) These 3 conditions make the loop run a specific number of times and exit at the right time:
a) At the beginning, set the initial value for the counter~~int i=1;
b) The counter is compared with a finite value as the loop condition ~~i<=100;
c) Update counter ~~i++;
5) If the initial value of the counter is not set correctly, it will cycle the wrong number of times. If the loop condition is set incorrectly, the loop will not start or end. The loop cannot be ended without updating the counter.
Knowledge point 4: do while loop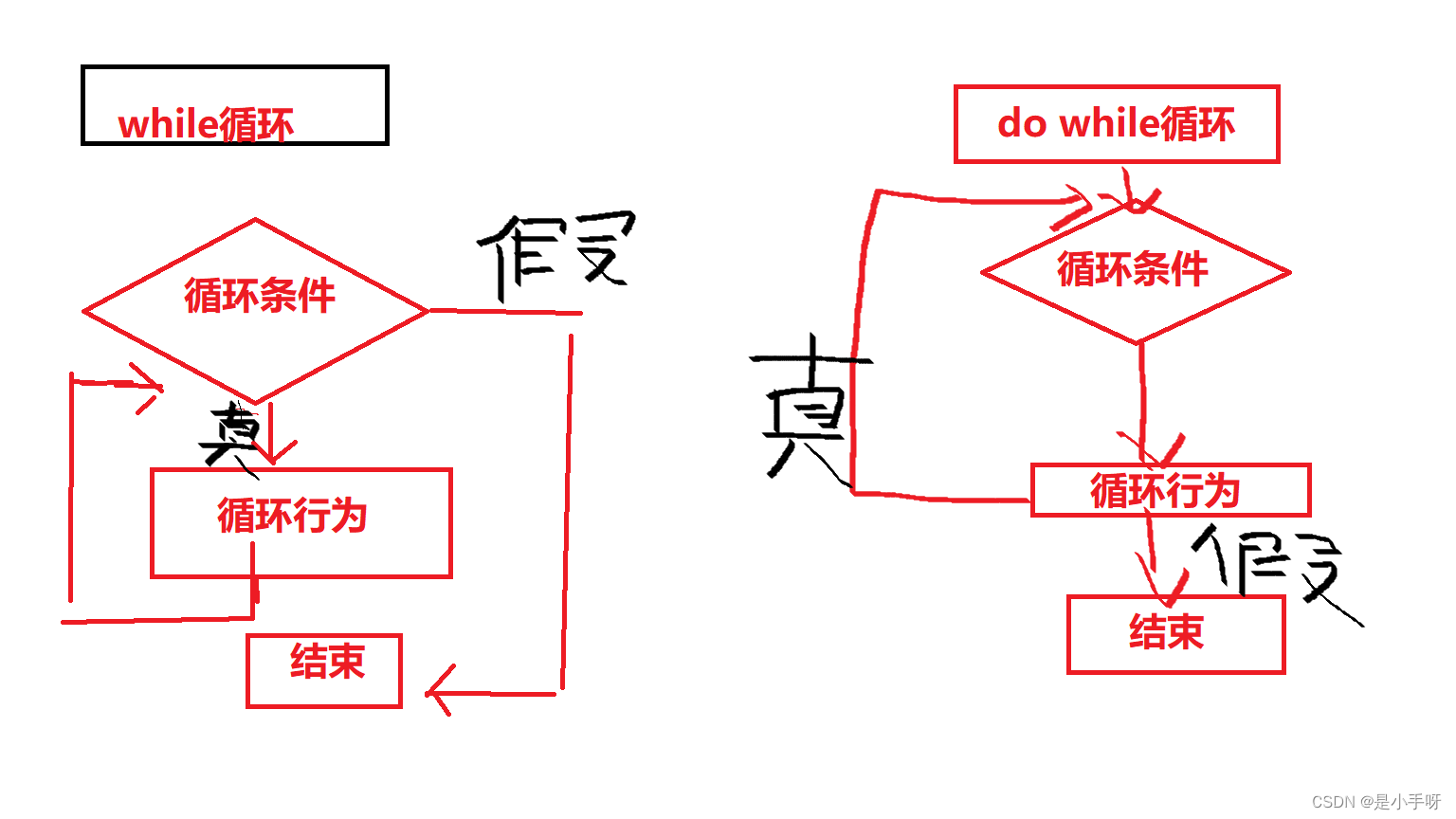
1) The while loop will check whether the loop condition is met when the loop enters. If it is not satisfied, it will not be executed even once;
2) do while will first execute the loop behavior once and then check the loop condition. The loop condition will be executed at least once;
3) The paradigm of do while:
do
循环行为
while(循环条件); // 注意do while有分号结尾
do{
循环行为1
循环行为2
循环行为3
...
}while(循环条件); // 注意do while有分号结尾
#Please note that do while needs to end with a semicolon;
Knowledge point five: for loop
1) The for keyword provides another compact way of writing loops;
#include <stdio.h>
int main()
{
int i, sum = 0;
for(i = 1; i <= 100; i++)
{
sum = i + sum;
}
printf("%d %d\n", i, sum);
return 0;
}
2) The structure of the for loop is as follows: for (counter setting initial value; loop condition; counter update)
cyclical behavior
3) Like while, for only has an effect on the statement following it. If you want to loop through multiple statements, use curly braces to combine them into a matching statement;
for (计数器设置初始值; 循环条件; 计数器更新)
{
循环行为1
循环行为2
循环行为3
...
}
a) The counter setting initial value in the for loop is only executed once at the beginning;
b) After running all loop behaviors once, a counter update will be performed;
c) If the loop condition is met, the loop will be entered, otherwise the loop will end;
4) If i is only used as a counter, and after the loop ends, the value of i is not concerned. i can be declared when initializing the counter;
#include <stdio.h>
int main()
{
int sum = 0;
for(int i = 1; i <= 100; i++)
{
sum = i + sum;
}
printf("%d\n", sum);
return 0;
}
Note: i is only valid within the loop body, and will become invalid after the loop ends.
5) It is worth noting that the three parts within the brackets of the for loop can be written in other places;
a) The counter is initialized to empty;
#include <stdio.h>
int main()
{
int i = 0; // 在这初始化计数器
for(; i <= 100; i = i + 2)
{
printf("%d ", i);
}
return 0;
}
b) The counter is updated to empty;
#include <stdio.h>
int main()
{
for(int i = 0; i <= 100; )
{
printf("%d ", i);
i = i + 2; // 在这更新计数器
}
return 0;
}
c) The loop condition is empty, which will lead to an infinite loop;
#include <stdio.h>
int main()
{
for(int i = 0; ; i = i + 2)
{
printf("%d ", i);
}
return 0;
}
Note: If you just want to write an infinite loop, you can leave out all three conditions. Or write an expression that is always true. The following 3 are all infinite loops. But the while loop cannot omit the loop condition.
for(;;)
循环行为
for(;100;)
循环行为
while(100)
循环行为