Serial communication refers to a communication method that transmits data bit by bit between peripherals and computers through data signal lines, ground lines, control lines, etc. This communication method uses fewer data lines and can save communication costs in long-distance communication, but its transmission speed is lower than parallel transmission. The serial port is a very common device communication protocol on computers. The pyserial module encapsulates Python's access to the serial port and provides a unified interface for use on multiple platforms.
1. Environment configuration
My python environment is version 3.7.8, and the download path is as follows:
1.python 3.7.8
You can directly enter the official website to download and install: Download Python | Python.org
2.serial library installation
Install using pip interface
pip install serial
pip install pyserial
For a detailed description of the pip interface, please see: [Python] pip, a detailed explanation of the Python package management tool! _Python package management tool pip_==PP's blog-CSDN blog
2. Use of basic functions of serial library
1. Serial port initialization function serial.Serial():
ser = serial.Serial('COM3',115200,timeout=5)
Let’s talk about the functions of the three parameters in the above function:
Parameter 1: com3 is the port number for serial port reading and writing
Parameter 2: 115200 is the baud rate of the serial port
Parameter 3: timeout is the timeout setting of the serial port
The above three parameters are commonly used parameters. We will mainly talk about them here. Other parameters are less used and will not be introduced here. If you are interested, you can search in the official documents or other blog areas.
2.write function serial port writes data
Write = ser.write(b'bsp\n')
Send data to the serial port
b: This parameter represents the bytes type. If you send a string directly, an error will be reported.
\n: The meaning of line break
bsp: the content to be sent
Note: You must add b in front, which means writing data in bytes like a serial port.
3.read() function serial port reads data
ser.read() ####从端口读字节数据,默认1个字节
ser.read_all() ####从端口接收全部数据
ser.readline() ###读一行数据
ser.readlines() ###读多行数据
The serial port reading data operations are introduced here in a unified manner, as follows:
(1) ser.read(): Read byte data from the port, default is 1 byte
(2) ser.read_all(): Receive all data from the port
(3) ser.readline(): Read a line of data
(4) ser.readlines(): Read multiple lines of data
After introducing the use of several basic functions, let's learn more about the use of the serial library through practical examples.
3. Example demonstration
Go directly to the code:
import serial
import time
if __name__ == '__main__':
ser = serial.Serial('COM3',115200,timeout=5) ##连接串口,打开
time.sleep(0.5)
Write = ser.write(b'Hello\n') ##发送数据
Read = ser.read() ###接收1个字节数据
print(Read)
Write = ser.write(b'Hello\n') ##发送数据
Read = ser.readline() ##接收一行数据
print(Read)
Write = ser.write(b'Hello\n') ##发送数据
Read = ser.read_all() ###接收所有数据
print(Read)
Write = ser.write(b'Hello\n') ##发送数据
Read = ser.readlines() ###读多行数据
print(Read)
ser.close() ###关闭串口连接
Output:
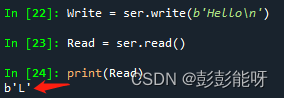
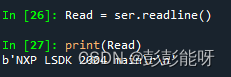
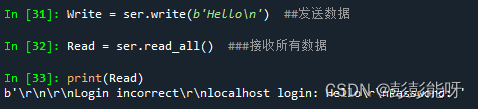

Here, the "Hello" character is also sent to the serial port and read using ser.readlines() as shown in the figure above.
In addition to the use of the above functions, there are also the following functions and functions:
ser.isOpen() |
Check whether the port is open |
ser.open() |
open port |
flush() |
Wait for all data to be written out |
flushInput() |
Discard all data in receive buffer |
flushOutput() |
Terminate the current write operation and discard the data in the send buffer |
4. Summary
The basic serial port read and write operations are as shown above. If you want to learn more about the use of the serial library, you can also read my other article:
[python] Make a serial port tool (Part 1)! _Python writing serial port tool_==PP's blog-CSDN blog
@Neng