Step 1: Install sortablejs depends on npm install sortablejs --save
npm install sortablejs --save
Step 2: Basic table code
<el-table
ref="manufacturing"
row-key="keys"
:data="form.manufacturing"
tooltip-effect="dark"
border
style="width: 50%"
:row-class-name="tableRowClassName"
:header-cell-style="{
background: '#f9fafc',
padding: '5px 0',
color: '#333',
fontSize: '14px',
height: '50px',
}"
:cell-style="{
padding: '5px 0',
fontSize: '14px',
}"
>
<el-table-column type="selection" width="55" align="center">
</el-table-column>
<el-table-column
type="index"
width="50"
label="序号"
align="center"
>
</el-table-column>
<el-table-column prop="name" label="工序名称" align="center">
<template slot-scope="scope">
<div style="display: flex">
<el-select
placeholder="请选择"
v-model="form.manufacturing[scope.$index].name"
filterable
remote
value-key
:remote-method="manufacturListing"
style="width: 80%"
size="small"
@focus="manufacturListingNull"
clearable
>
<el-option
v-for="item in manufacturingList"
:key="item.id"
:label="item.name"
:value="item.name"
>
</el-option>
</el-select>
<div class="edit-list">
<span
@click="(erpFundamentalAdding = true), (sendType = '3')"
>新增</span
>
</div>
</div>
</template>
</el-table-column>
<el-table-column
type="index"
width="170"
label="操作"
align="center"
>
<template slot-scope="scope">
<div style="display: flex">
<el-button
type="danger"
@click="handleDelete(scope.$index, scope.row, 4)"
>删除</el-button
>
<el-button
type="primary"
@click="addSupplier(4)"
v-if="scope.$index + 1 == form.manufacturing.length"
>添加</el-button
>
</div>
</template>
</el-table-column>
</el-table>
Rendering:

The most important of which is
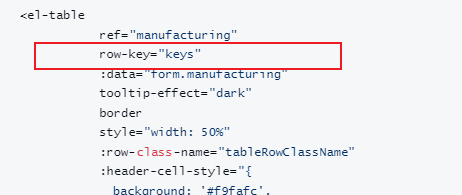
row-key='id' must be added, otherwise there will be rebound or disordered sorting. This id must be unique, which means that the id of each row is unique and will not be repeated. If there is no id, it can be used Math.round() random numbers instead to reduce repetition rate,
Step 3: Introduce the downloaded dependencies

import Sortable from "sortablejs";
data(){
form:{
manufacturing: [
{
keys: Math.random().toFixed(4),
name: "",
id: "",
},
],
}
}
Step 4: Find the table dom element (referring to the parent container of the element to be dragged)
rowDrop() {
const tbody = this.$refs.manufacturing.$el.querySelector(
".el-table__body-wrapper > table > tbody"
);
const _this = this;
Sortable.create(tbody, {
onEnd({ newIndex, oldIndex }) {
const currRow = _this.form.manufacturing.splice(oldIndex, 1)[0];
_this.form.manufacturing.splice(newIndex, 0, currRow);
},
});
}
Step 5: Trigger this event after the mount completes its life cycle
mounted() {
this.$nextTick(() => {
this.rowDrop();
});
},
After completing the above five steps, you can basically achieve it. If it doesn't work, please leave a message.