1. The role of QFrame
The QFrame class inherits from the QWidget class and is inherited by QAbstractScrollArea, QLabel, QLCDNumber, QSplitter, QStackedWidget, and QToolBox. As the base class of many basic controls, QFrame provides many member methods to subclasses to implement the design of the frame style of the subclass. Frame styles primarily consist of the frame shape and a shadow style used to visually separate the frame from surrounding widgets. Right now
- QFrame provides member functions for designing frame styles for subclasses to help subclasses design unique styles: either raised borders, sunken borders, raised content, or sunken content, etc.
- QFrame can also be used directly to create simple placeholder frames that contain no content.
2. QFrame member type document
2.1 enum QFrame::Shadow
This enumeration type defines the type of shadow used to provide a 3D effect to frames.
QFrame::Plain | 0x0010 | Borders or content appear to be as tall as surrounding controls |
QFrame::Raised | 0x0020 | Borders or content appear to be raised compared to surrounding controls |
QFrame::Sunken | 0x0030 | Borders or content appear sunken compared to surrounding controls |
2.2 enum QFrame::Shape
This enum type defines the shape of available frames.
QFrame::NoFrame | 0 | Don't draw anything |
QFrame::Box | 0x0001 | Draw a border to wrap the content and highlight the shadow effect of the border |
QFrame::Panel | 0x0002 | Draw a content plane to highlight the shadow effect of the content |
QFrame::StyledPanel | 0x0006 | Draws a rectangular panel whose appearance depends on the current GUI style. It can be raised or sunken. |
QFrame::HLine | 0x0004 | Draw a horizontal line to separate and highlight the shadow effect of the line |
QFrame::VLine | 0x0005 | Draw a vertical line to separate and highlight the shadow effect of the line |
QFrame::WinPanel | 0x0003 | Draws a rectangular panel that can be moved up or down like in Windows 2000. Specifying this shape sets the line width to 2 pixels. WinPanel is provided for compatibility. |
For GUI style independence, we recommend using StyledPanel instead.
2.3 enum QFrame::StyleMask
This enumeration defines two constants that can be used to extract the two components of frameStyle().
QFrame::Shadow_Mask | 0x00f0 | The shadow part of the control's framStyle() return value |
QFrame::Shape_Mask | 0x000f | The shape part of the control's framStyle() return value |
2.4 Style combinations that can be achieved using QFrame
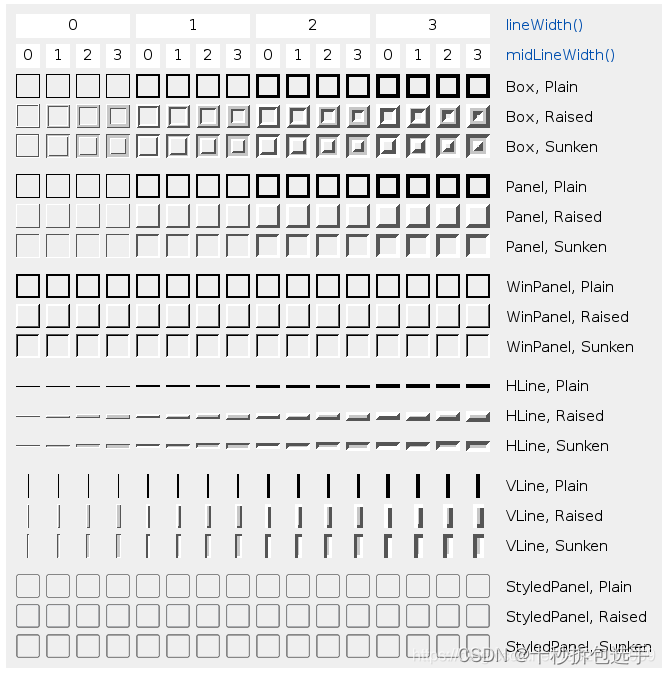
3. Commonly used member functions of QFrame
3.1 Introduction to member function prototypes
member function prototype | describe |
QFrame(QWidget *parent = nullptr, Qt::WindowFlags f = Qt::WindowFlags()) | Constructor |
virtual ~QFrame() | destructor |
QRect frameRect() const | Get the frame size of QFrame |
void setFrameRect(const QRect &) | Set the frame size of QFrame |
QFrame::Shadow frameShadow() const | Get the shadow style, |
void setFrameShadow(QFrame::Shadow) | Set shadow style, |
QFrame::Shape frameShape() const | Get the shape of the frame |
void setFrameShape(QFrame::Shape) | Set the shape of the frame |
int frameStyle() const | Get the frame's shape and shadow style |
void setFrameStyle(int style) | Set the frame's shape and shadow style |
int lineWidth() const | Get the width of the frame line |
void setLineWidth(int) | Set the width of the frame lines |
int midLineWidth() const | Get the width of the center line of the frame |
void setMidLineWidth(int) | Set the width of the center line of the frame |
3.2 Simple example
Use QLabel, a subclass of QFrame, to complete the design of a simple login interface. By changing the QLabel frame style, it is more consistent with the style of QLineEdit, QPushButton and other controls.
//.h文件
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private:
QLabel *useLabel;
QLabel *pwLabel;
QLabel *useEdit;
QLabel *pwEdit;
QLabel *checkBtn;
QLabel *cancelBtn;
};
//.c文件
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
this->resize(400,550);
useLabel = new QLabel(this);
useLabel->setText("用户名:");
useLabel->move(70,150);
useLabel->resize(50,30);
//设置"用户名"标签的框架样式为 无边框+无阴影
useLabel->setFrameShape(QFrame::NoFrame);
useLabel->setFrameShadow(QFrame::Plain);
useEdit = new QLabel(this);
useEdit->move(150,150);
useEdit->resize(200,30);
//设置"用户名"输入框的框架样式为 边框+突起
useEdit->setFrameStyle(QFrame::Box|QFrame::Raised);
useEdit->setLineWidth(2);
pwLabel = new QLabel(this);
pwLabel->setText("密码:");
pwLabel->move(70,210);
pwLabel->resize(50,30);
//设置"密码"标签的框架样式为 无边框+无阴影
pwLabel->setFrameStyle(QFrame::NoFrame|QFrame::Plain);
pwEdit = new QLabel(this);
pwEdit->move(150,210);
pwEdit->resize(200,30);
//设置"用户名"输入框的框架样式为 内容+凹陷
pwEdit->setFrameStyle(QFrame::Panel|QFrame::Sunken);
pwEdit->setLineWidth(2);
checkBtn = new QLabel(this);
checkBtn->setText("确认");
checkBtn->setAlignment(Qt::AlignCenter);
checkBtn->move(100,290);
checkBtn->resize(80,30);
//设置"确认"按钮的框架样式为 内容+突起
checkBtn->setFrameStyle(QFrame::Panel|QFrame::Raised);
checkBtn->setLineWidth(3);
cancelBtn = new QLabel(this);
cancelBtn->setText("取消");
cancelBtn->setAlignment(Qt::AlignCenter);
cancelBtn->move(240,290);
cancelBtn->resize(80,30);
//设置"取消"按钮的框架样式为 内容+突起
cancelBtn->setFrameStyle(QFrame::Panel|QFrame::Raised);
cancelBtn->setLineWidth(3);
}
Rendering: