Before talking about the prototype, let’s talk about how to copy the structure. We know that recursion and JSON can be used to deep copy objects, but what if we only copy the structure?
You can use the factory pattern, or it can also be called a factory function. A simple understanding is to put some raw materials into the function to process all the same products. The factory function is written similar to the constructor function, as follows:
function createUser(name, age) {
return {
name, age, getName() {
return this.name
}
}
}
let user1 = createUser('Peter', 28)
let user2 = createUser('Andy', 18)
user1.name // Peter
The disadvantage of the factory function is that although user1 and use2 use the same function, they exist independently. Such creation will waste space.
console.log(user1.getName == user2.getName) // false
So we can use prototypes. Prototypes allow objects to share parts. No matter how many users are created, they all refer to the same address, which greatly saves storage space.
function createUser(name, age) {
this.name = name
this.age = age
}
createUser.prototype.getName = function () {
console.log(this.name)
}
let user1 = new createUser('Peter', 28)
let user2 = new createUser('Andy', 18)
user1.name // Peter
console.log(user1.getName == user2.getName) // true
prototype
The prototype attribute of the constructor
Each function has a prototype attribute, which points to an Object empty object by default (called: prototype object)
There is an attribute constructor in the prototype object , which points to the function object
The role of prototype
Used to add some methods or attributes to the prototype attributes. Each instance object created by new can be shared with the methods and attributes on the prototype .
The __proto__ attribute of the instance object
Each instance object has a __proto__ attribute, which points to the prototype attribute in the function (that is, called: object prototype)
Explicit and implicit prototypes
Function => Constructor shows prototype prototype
Object => Instance object implicit prototype __proto__
The prototype in the constructor is equivalent to __proto__ in the instance object
prototype chain
Every time a function is created, the parser will add a prototype attribute to the function to point to the prototype object according to specific rules. The prototype object will get a constructor property by default, pointing back to the constructor associated with it.
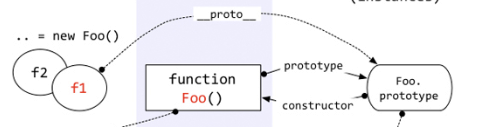
The displayed prototype and the implicit prototype point to the same empty object. This empty object is also an instance object, which is derived from the Object instance. The implicit prototype of the empty object points to the displayed prototype of Object, but Object is special. Its implicit prototype is null
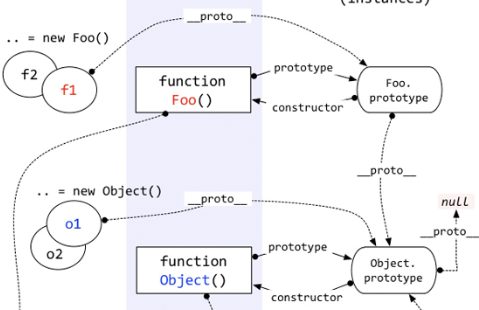
But Object is instantiated from function, so the implicit prototype of Object points to the explicit prototype of the function, and the implicit prototype of the explicit prototype of the function points to the explicit prototype of the object.
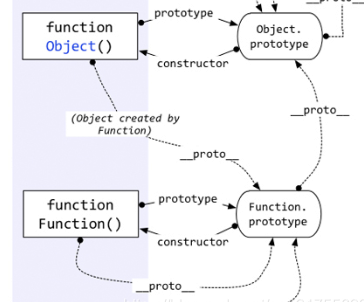
The constructor is also derived from a Function instance, so the implicit prototype of the constructor points to the explicit prototype of the function
The structure of the entire prototype chain is as follows:
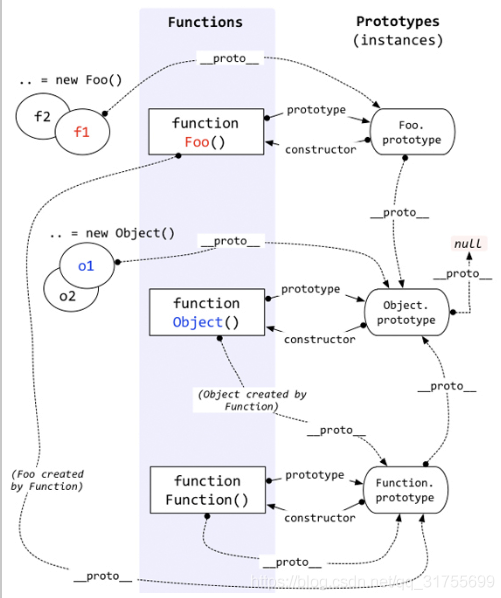
Prototype chain search rules
When accessing a property through an object, the search will start based on the name of the property, which will follow the following search order:
1. The search starts from the instance object itself. If an attribute name is found on this instance, the value corresponding to the name will be returned and the search will not continue.
2. If this attribute is not found, it will be searched in the prototype object along the __proto__ of the instance object. If the attribute is found in the prototype object, the corresponding value will be returned and the search will not continue.
3. If it is not found yet, continue to search along the __proto__ of the prototype object. The __proto__ of the prototype object of Object is null. If it is not found in the prototype of the Object object, it is the end and undefined is returned directly.
Summarize
prototype
It is used to share the parts of the object that need to be shared and save storage space.
每创建好一个构造函数,解析器就会自动添加一个prototype属性执行一个空对象(原型对象),原型对象也会添加一个constructor属性指回该构造函数
用new创建出来的对象称为实例对象,在创建的时候也会自动添加一个__proto__属性指向空对象 构造函数的.prototype == 实例对象的.__proto__
原型链
原型链查找规则:先在自身上找,没有就沿着 __proto__ 去原型对象中查找,都找不到就返回 undefined