Article directory
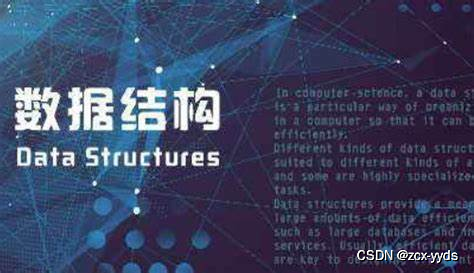
1. Direct insertion sort
1. Insertion sort idea
Direct insertion sort is to insert the records to be sorted into an already sorted ordered sequence according to their key values, until all records are inserted and a new ordered sequence is obtained.
The idea of insertion sort is just like when we usually play poker and sort cards, insert each card one by one into an ordered sequence of cards, and eventually all the cards will be in order.
2. Program code
//插入排序
void InsertSort(int* a, int n)
{
for (int i = 0; i < n-1; i++)
{
//end可看作从左至右有序的最后一个数的下标
int end = i;
int tmp = a[end + 1];
while (end >= 0)
{
if (tmp < a[end])
{
a[end + 1] = a[end];
}
else
{
break;
}
end--;
}
//此时tmp的值大于或等于下标为end的值,所以插入在它的后面
a[end+1] = tmp;
}
}
Code analysis:
When inserting the i (i>=1) element, the previous a[0], a[1],...,a[i-1] have been sorted. At this time, use the sorting code of a[i] and Compare the sorting code order of a[i-1], a[i-2],..., find the insertion position, insert a[i], and move the elements at the original position backward.
Direct insertion sort is a stable sorting algorithm. The closer the element set is to order, the higher the time efficiency of the direct insertion sort algorithm. Its time complexity is O(n 2) and its space complexity is O (1).
3. Test
//打印数组
void PrintArray(int* a, int n)
{
for (int i = 0; i < n; i++)
{
printf("%d ", a[i]);
}
printf("\n");
}
//测试直接插入排序
void TestInsertSort()
{
//任意建立一组无序的数
int a[] = {
9,1,2,5,7,4,8,6,3,5,1,2,3,5,1,8,3 };
InsertSort(a, sizeof(a) / sizeof(int));
PrintArray(a, sizeof(a) / sizeof(int));
}
int main()
{
printf("直接插入排序:\n");
TestInsertSort();
return 0;
}
2. Hill sorting
1. What is Hill sorting?
Hill sorting method is also called shrinking increment method. The basic idea of Hill sorting is: first select an integer gap, divide all records in the sequence to be sorted into gap groups, put all records with a distance of gap into the same group, and sort the records in each group . Then reduce the gap, you can take half of it, and repeat the above grouping and sorting work. When the gap reaches 1, the array is in order.
When gap=1, it is equivalent to direct insertion sort.. Therefore, Hill sorting can be split into two parts: presorting and direct insertion sorting:
- Pre-sorting: When the gap is greater than 1, pre-sorting can make large numbers go to the back of the sequence faster, and small numbers to the front faster. The larger the gap, the faster the jump and the less ordered it is. The smaller the gap, the slower the jump. , the closer to order
- Direct insertion sort: The gap continues to decrease. When the gap is 1, it is equivalent to direct insertion sort. After the last direct insertion sort, the sequence will be in order.
2. Hill sorting diagram
Use the Hill sorting algorithm to sort the sequence in the figure below:
There are a total of 8 numbers in this sequence. We selected the initial gap value as 8/2=4, and the numbers separated by 4 are a group, as shown below. The same group of numbers has the same color.
After sorting each group, the gap is divided by 2 to become 2
After each group is sorted, the gap is divided by 2 and becomes 1. This is equivalent to direct insertion sorting.
3. Program code
//希尔排序
void ShellSort(int* a, int n)
{
//gap进入循环后会先除2
int gap = n ;
while (gap > 1)
{
gap /= 2;
for (int i = 0; i < n - gap; i++)
{
int end = i;
int tmp = a[end + gap];
while (end >= 0)
{
if (tmp < a[end])
{
a[end + gap] = a[end];
}
else
{
break;
}
end -= gap;
}
a[end + gap] = tmp;
}
}
}
Hill sorting is unstable. It is an optimization of direct insertion sorting. Because there are more than one ways to take the gap value, the time complexity of Hill sorting is difficult to calculate.
4. Test
//打印数组
void PrintArray(int* a, int n)
{
for (int i = 0; i < n; i++)
{
printf("%d ", a[i]);
}
printf("\n");
}
//测试希尔排序
void TestShellSort()
{
//任意建立一组无序的数
int a[] = {
9,1,2,5,7,4,8,6,3,5,1,2,3,5,1,8,3 };
ShellSort(a, sizeof(a) / sizeof(int));
PrintArray(a, sizeof(a) / sizeof(int));
}
int main()
{
printf("希尔排序:\n");
TestShellSort();
return 0;
}