Table of contents
Packaging and resource loading framework directory
text
The hot update process is not fixed, and the state machine written by each person is also different, but there are certainly some necessary steps, such as downloading the list, comparing versions, downloading the AB package, and marking the download completion. Each of my next articles is an important step in downloading the AB package, and most likely cannot be omitted.
Check if sandbox path exists
public static string MakePersistentLoadPath(string path)
{
#if UNITY_EDITOR
// 注意:为了方便调试查看,编辑器下把存储目录放到项目里
string projectPath = Path.GetDirectoryName(Application.dataPath).Replace("\\","/");
projectPath = GetRegularPath(projectPath);
return StringFormat.Format("{0}/Sandbox/{1}", projectPath, path);
#else
return StringFormat.Format("{0}/Sandbox/{1}", Application.persistentDataPath, path);
#endif
}
Check whether the download temporary directory exists
Please note that when downloading the hot update, the AB package is first downloaded to the temporary directory, and then copied to the sandbox directory.
public static string MakeDownloadTempPath(string path)
{
#if UNITY_EDITOR
string projectPath = Path.GetDirectoryName(Application.dataPath).Replace("\\", "/");
projectPath = GetRegularPath(projectPath);
return StringFormat.Format("{0}/Sandbox_Temp/{1}", projectPath, path);
#else
return StringFormat.Format("{0}/Sandbox_Temp/{1}", Application.persistentDataPath, path);
#endif
}
After confirming that the paths exist, start downloading
As introduced before, the generated AB package list looks like this. This file is generated into a binary bytes file and thrown to the server for downloading.
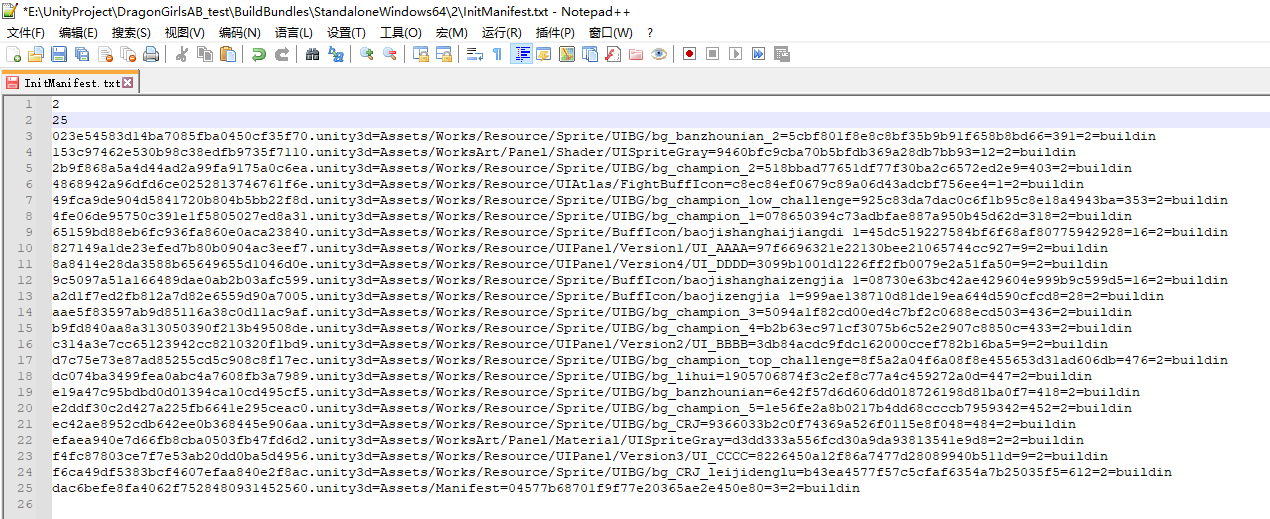
The first line is the SVN version number
The second line is the number of AB packages
Starting from the third line is the resource package information, separated by = sign to separate the valid data, respectively
MD5.unity3d = Resource path = HashId of resource path = Package body KB size = SVN version number = Start hot update mode
Each row of data encapsulates a PatchElement class, the code is below
Our project encapsulates UnityWebRequest and calls it WebDataRequest. If you don’t want to encapsulate it, just use UnityWebRequest. The WebDataRequest code is at the back.
private IEnumerator DownLoad()
{
// 解析APP里的补丁清单
string filePath = AssetPathHelper.MakeStreamingLoadPath(PatchDefine.InitManifestFileName);
string url = AssetPathHelper.ConvertToWWWPath(filePath);
using (WebDataRequest downloader = new WebDataRequest(url))
{
yield return downloader.DownLoad();
if (downloader.States == EWebRequestStates.Success)
{
PatchHelper.Log(ELogLevel.Log, "Parse app patch manifest.");
ParseAppPatchManifest(downloader.GetData());
}
else
{
throw new System.Exception($"Fatal error : Failed download file : {url}");
}
}
}
// 解析补丁清单文件相关接口
public void ParseAppPatchManifest(byte[] data)
{
if (AppPatchManifest != null)
throw new Exception("Should never get here.");
AppPatchManifest = new PatchManifest(true);
AppPatchManifest.Parse(data);
}
The PatchManifest class is a class that specializes in parsing AB package lists. You can tell by looking at the code that the ultimate purpose of the Parse method is to parse each line of data in the list into PatchElement and then add it to the dictionary and save it to be called when downloading.
/// <summary>
/// 补丁清单文件
/// </summary>
public class PatchManifest
{
private bool _isParse = false;
/// <summary>
/// 资源版本号
/// </summary>
public int DllVersion { private set; get; }
public int ResVersion { private set; get; }
private bool IsInit = false;
/// <summary>
/// 所有打包文件列表
/// </summary>
public readonly Dictionary<string, PatchElement> Elements = new Dictionary<string, PatchElement>();
public PatchManifest(bool isInit = false)
{
IsInit = isInit;
}
/// <summary>
/// 解析数据
/// </summary>
public void Parse(byte[] data)
{
using (var ms = new MemoryStream(data))
{
using(var br = new BinaryReader(ms))
{
Parse(br);
}
}
}
public void ParseFile(string filePath)
{
using (var fs = File.OpenRead(filePath))
{
using (var br = new BinaryReader(fs))
{
Parse(br);
}
}
}
/// <summary>
/// 解析数据
/// </summary>
public void Parse(BinaryReader br)
{
if (br == null)
throw new Exception("Fatal error : Param is null.");
if (_isParse)
throw new Exception("Fatal error : Package is already parse.");
_isParse = true;
// 读取版本号
DllVersion = br.ReadInt32();
ResVersion = br.ReadInt32();
GameVersion.PatchResDesc = ResVersion + " dllVer:" + DllVersion;
int fileCount = br.ReadInt32();
// 读取所有Bundle的数据
for(var i = 0; i < fileCount; i++)
{
var ele = PatchElement.Deserialize(br, IsInit);
if (Elements.ContainsKey(ele.Name))
throw new Exception($"Fatal error : has same pack file : {ele.Name}");
Elements.Add(ele.Name, ele);
}
}
}
PatchElement is an encapsulation of each row of data in the AB package list. The Parse of PatchManifest will create an Elements dictionary in a loop to save this data.
public class PatchElement
{
/// <summary>
/// 文件名称
/// </summary>
public string Name { private set; get; }
/// <summary>
/// 文件MD5
/// </summary>
public string MD5 { private set; get; }
/// <summary>
/// 文件版本
/// </summary>
public int Version { private set; get; }
/// <summary>
/// 文件大小
/// </summary>
public long SizeKB { private set; get; }
/// <summary>
/// 构建类型
/// buildin 在安装包中
/// ingame 游戏中下载
/// </summary>
public string Tag { private set; get; }
/// <summary>
/// 是否是安装包内的Patch
/// </summary>
public bool IsInit { private set; get; }
/// <summary>
/// 下载文件的保存路径
/// </summary>
public string SavePath;
/// <summary>
/// 每次更新都会先下载到Sandbox_Temp目录,防止下到一半重启导致逻辑不一致报错
/// temp目录下的文件在重新进入更新流程时先校验md5看是否要跳过下载
/// </summary>
public bool SkipDownload { get; set; }
public PatchElement(string name, string md5, int version, long sizeKB, string tag, bool isInit = false)
{
Name = name;
MD5 = md5;
Version = version;
SizeKB = sizeKB;
Tag = tag;
IsInit = isInit;
SkipDownload = false;
}
public void Serialize(BinaryWriter bw)
{
bw.Write(Name);
bw.Write(MD5);
bw.Write(SizeKB);
bw.Write(Version);
if (IsInit)
bw.Write(Tag);
}
public static PatchElement Deserialize(BinaryReader br, bool isInit = false)
{
var name = br.ReadString();
var md5 = br.ReadString();
var sizeKb = br.ReadInt64();
var version = br.ReadInt32();
var tag = EBundlePos.buildin.ToString();
if (isInit)
tag = br.ReadString();
return new PatchElement(name, md5, version, sizeKb, tag, isInit);
}
}
The following is the download data package, which is essentially UnityWebRequest.
public class WebDataRequest : WebRequestBase, IDisposable
{
public WebDataRequest(string url) : base(url)
{
}
public override IEnumerator DownLoad()
{
// Check fatal
if (States != EWebRequestStates.None)
throw new Exception($"{nameof(WebDataRequest)} is downloading yet : {URL}");
States = EWebRequestStates.Loading;
// 下载文件
CacheRequest = new UnityWebRequest(URL, UnityWebRequest.kHttpVerbGET);
DownloadHandlerBuffer handler = new DownloadHandlerBuffer();
CacheRequest.downloadHandler = handler;
CacheRequest.disposeDownloadHandlerOnDispose = true;
CacheRequest.timeout = Timeout;
yield return CacheRequest.SendWebRequest();
// Check error
if (CacheRequest.isNetworkError || CacheRequest.isHttpError)
{
MotionLog.LogWarning($"Failed to download web data : {URL} Error : {CacheRequest.error}");
States = EWebRequestStates.Fail;
}
else
{
States = EWebRequestStates.Success;
}
}
public byte[] GetData()
{
if (States == EWebRequestStates.Success)
return CacheRequest.downloadHandler.data;
else
return null;
}
public string GetText()
{
if (States == EWebRequestStates.Success)
return CacheRequest.downloadHandler.text;
else
return null;
}
}