1. Android side: Open directly through URL Scheme.
2. iOS side (2 types): (1) Open using URL Scheme. (2) Open using Universal link.
3. Precautions for using the Universal link method: (1) Native app developers need to configure universal link to the front end; (2) Only devices with ios9 and above (including ios9) support universal link; (3) Operation and maintenance needs to be done Personnel configures the h5 page path specified by the universal link (when accessing 404 (open WeChat or Qiwei, the app is not installed on the phone), it will be directed to the h5 landing page).
4. In WeChat or Qiwei WeChat: URL Scheme and Universal link are not supported to open the APP. You need to use the WeChat guide page to prompt how to open it in the browser.
5. schemaUrl: Let the android and ios developers of the native app configure it and give it the front-end URL.
1. Create a new tool function file utils.js as follows:
// android终端
export const isAndroid = u.indexOf('Android') > -1 || u.indexOf('Adr') > -1
// ios终端
export const isIos = !!u.match(/\(i[^;]+;( U;)? CPU.+Mac OS X/)
// 微信
export function isWeixin() {
return navigator.userAgent.toLowerCase().indexOf('micromessenger') !== -1
}
/**
* 获取IOS的版本号
*/
const getIosVersion = () => {
const ua = navigator.userAgent.toLowerCase();
const version = ua.match(/cpu iphone os (.*?) like mac os/);
const finalVersionStr = version && version[1].replace(/_/g,".")
return finalVersionStr
}
/**
* 判断IOS设备版本号,是否大于ios9
*/
const isGreatOrSameIos9 = () => {
const currentVersion = getIosVersion()
const versionList = currentVersion.split('.')
return Number(versionList[0]) >= 9
}
/**
* 打开IOS的商品详情的universal link
*/
const openIosGoodDetailUniversalLink = (paramsStr) => {
let ele = document.createElement('a')
ele.style.display = 'none'
ele.href = 'https://www.xxxx.com.cn/open/xxxx?action_type=1024&' + paramsStr // ios的universal link
document.body.appendChild(ele)
ele.click()
}
/**
* h5打开手机上的app
* @param { Object } that 页面实例
* @param { String } propName 控制微信浏览器(微信、企业微信)引导页显示的变量
* @param { String } paramsStr 打开APP内某个页面的业务传参
* @Note 1、ios的univeral link在微信或企业微信上,即使安装了app,也不会直接跳到 APP, 而是显示h5页面。
* @Note 2、ios的itunes下载链接:在微信和企业微信中都无法显示页面, 加载不了。
*/
export const handleH5InvokeApp = (that, propName = 'showWeiXinGuide', paramsStr) => {
// 微信 或 企微中: 需要在浏览器中打开
try {
if(isWeixin()) {
that[propName] = true
} else if (isAndroid || isIos) {
const schemaUrl = 'xxxx://action?action_type=1024&' + paramsStr
const downloadMarketUrl = isAndroid ? 'https://www.xxx.com/xxx' : 'https://itunes.apple.com/us/app/xxx/xxx?l=zh&ls=1&mt=x'
let iframeEle = null
let timer = setTimeout(function(){
document.hasFocus() && (window.location.href = downloadMarketUrl)
}, 3000)
if (isIos) {
// ios9以上(包含ios9): 使用univeral link方式
if (isGreatOrSameIos9()) {
clearTimeout(timer)
openIosGoodDetailUniversalLink(paramsStr)
return
} else {
iframeEle = document.createElement('iframe')
iframeEle.src = schemaUrl
iframeEle.style.display = 'none'
document.body.appendChild(iframeEle)
}
// ios 中无法触发 visibilitychange 事件,所以需要 pagehide 来做兼容
window.addEventListener('pagehide', function(event) {
clearTimeout(timer)
})
} else {
window.location.href = schemaUrl
}
// 各种浏览器兼容
let hidden, visibilityChange;
if (typeof document.hidden !== "undefined") {
hidden = "hidden";
visibilityChange = "visibilitychange";
} else if (typeof document.mozHidden !== "undefined") {
hidden = "mozHidden";
visibilityChange = "mozvisibilitychange";
} else if (typeof document.msHidden !== "undefined") {
hidden = "msHidden";
visibilityChange = "msvisibilitychange";
} else if (typeof document.webkitHidden !== "undefined") {
hidden = "webkitHidden";
visibilityChange = "webkitvisibilitychange";
}
// 安卓: 可以直接使用 visibilitychange 来判断标签页展示、还是隐藏
document.addEventListener(visibilityChange, function() {
if(document.hidden) {
clearTimeout(timer)
}
}, false);
} else {
alert('只能在手机端浏览器打开') // 部分手机端浏览器可设置为Web打开
}
} catch (e) {
console.log('h5唤起app失败', e)
}
}
2. The guide page component open-in-browser-guide.vue in WeChat or enterprise micro environment (using uniapp-h5 syntax) :
<template>
<view class="guide-container">
<u-mask :show="show" @click="handleClose">
<view class="main">
<view class="content">
<text>步骤一、点击右上角的三个点</text>
<text>步骤二、选择在浏览器中打开</text>
<u-image width="166rpx" height="257rpx" :src="src" class="image-wrap"></u-image>
</view>
</view>
</u-mask>
</view>
</template>
<script>
export default {
props: {
show: {
type: Boolean,
default: false
}
},
data() {
return {
// 箭头图片(图片资源在博客最下方)
src: require('../../static/imgs/guide-arrow.png'),
};
},
methods: {
handleClose() {
this.$emit('update:show', false)
}
}
}
</script>
<style lang="scss" scoped>
.guide-container {
.main {
display: flex;
justify-content: center;
height: 100%;
.content {
display: flex;
flex-direction: column;
align-items:center;
justify-content: center;
width: 80%;
font-size: 32rpx;
line-height: 2;
margin: -80rpx auto 0;
color: #fff;
}
.image-wrap {
border-style: none;
position: absolute;
transform: rotateZ(246deg);
top: 20%;
right: 100rpx;
}
}
}
</style>
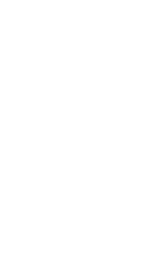
guide-arrow.png
Reference links:
Its URL Schema: https://www.jianshu.com/p/5bf51eb5322a?utm_campaign=maleskine&utm_content=note&utm_medium=seo_notes&utm_source=recommendation
How to implement H5 to evoke APP (such as awakening Zhihu): https://blog.csdn.net/qq_41960279/article/details/124817190