Recently
, an old man asked a question, saying, what if the java jar file is executed in Android? This is actually a very simple question. Can’t we just write an app and put it in it? But people say that there is no App and you can run it directly using the command line. When explaining this requirement, I was confused. I didn't have a good idea to think about this problem. Fortunately, after checking the information, I found that you can actually use the command line to execute the jar package command in Android.
First, let’s sort out the knowledge we need:
- The Android virtual machine, whether it is Dalvik or Art, cannot directly run Java jar files. It requires an intermediate product dex, which I won’t go into details here;
- How to generate dex manually requires the use of dx/d8 tools;
- Commonly used compilation commands for java, such as javac / java cp
- Commonly used adb/linux commands, such as adb shell / adb push/ rm -rf and other commands.
1. java project
First, let's take a look at our java helloworld project, which is a pure java project:
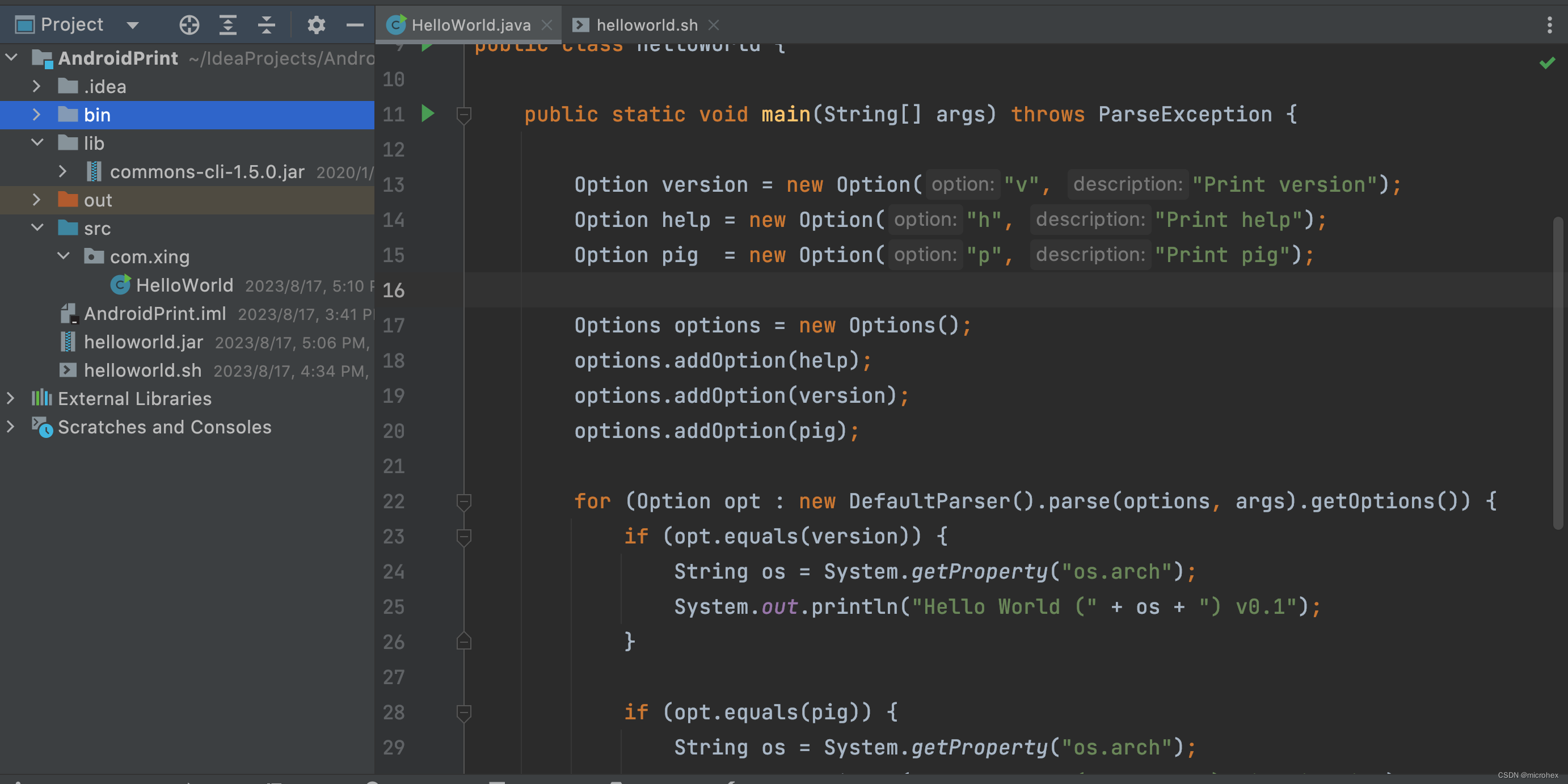
After we have written our HelloWorld, we can manually compile the java file into a class file through the command line. The command line is as follows:
javac -source 1.8 -target 1.8 -d bin -cp lib/commons-cli-1.5.0.jar src/com/xing/HelloWorld.java
One more thing to say here:
- -source 1.8 -target 1.8 specifies that the Java version of the compiled source code and the generated target class file is 1.8
- -d is the directory where class files are generated;
- The lib/commons-cli-1.5.0.jar followed by -cp is the jar package that my HelloWorld.java depends on.
So you need to bring it when compiling here.
If you need to use the java 11 version, the compilation command is:
javac -source 11 -target 11 -d bin -cp lib/commons-cli-1.5.0.jar src/com/xing/HelloWorld.java
2. Compile into dex file
After generating the class file, we need to compile the class file into a dex file. There are two ways: dex and d8. d8 is a compilation method with better update effect than dex, which can be found under the android compilation tool folder build-tools. Please indicate the following two ways of writing:
1. dx method:
./Android/sdk/build-tools/28.0.2/dx --output=helloworld.jar --dex ./bin lib/commons-cli-1.5.0.jar
--output=helloworld.jar
: Specify the name of the output dex file as helloworld.jar--dex
: Specify to perform dex conversion operation./bin
: The directory where the Java class file to be converted is located (just generated)lib/commons-cli-1.5.0.jar
: Additional jar library files, including additional classes that may be needed
2. d8 method:
./Android/sdk/build-tools/33.0.1/d8 --release lib/commons-cli-1.5.0.jar bin/Helloworld.class --output helloworld.jar
--release
:Enable release mode optimization conversionlib/commons-cli-1.5.0.jar
:Additional jar library filesbin/Helloworld.class
:Java class file to be converted--output helloworld.jar
:Output dex file
3. Upload files
Since we need to operate in the Android environment, we first write a helloworld.sh execution command. The advantage of this command is that we don’t have to type many commands all the time and can directly execute the .sh file. The file defines the environment in which the jar runs, and then the most important command is "exec app_process" to execute the jar command in the Andorid environment.
base=/data/local/tmp/helloworld
export CLASSPATH=$base/helloworld.jar
export ANDROID_DATA=$base
mkdir -p $base/dalvik-cache
exec app_process $base com.xing.HelloWorld "$@"
I won’t talk about other commands here, but mainly talk about the usage of app_process:
app_process
:Executable file used to start an Android application process, located in the /system/bin directory$base
:Jar package path, needs to be replaced with the actual Jar package file nameHelloWorld
: This is the main class in the Jar package and needs to be modified to the actual main class name."$@"
: Indicates that the parameters passed to the Java program$@
will be expanded into all parameters and passed in.
After defining the helloworld.sh file, the main file directory should be as follows:
|-bin
| |____com/xing/HelloWorld.class
|
|-lib
| |____commons-cli-1.5.0.jar
|
|-src
| |____com/xing/HelloWorld.java
|
|-helloworld.jar
|-helloworld.sh
Then we need to upload the target file to android. The basic command is as follows:
-
Create temporary folder helloworld
adb shell mkdir -p /data/local/tmp/helloworld
-
Push helloworld.jar into the temporary helloworld folder:
adb push helloworld.jar /data/local/tmp/helloworld
-
Push helloworld.sh into the temporary helloworld folder:
adb push helloworld.sh /data/local/tmp/helloworld
-
Elevate privileges to helloworld.sh so that it has executable permissions
adb shell chmod 777 /data/local/tmp/helloworld/helloworld.sh
-
Execute the code directly:
adb shell /data/local/tmp/helloworld/helloworld.sh -p
The -p here is what I added customly. If everything runs normally, you should be able to see the results of the operation:

Of course, if you have any questions, you can discuss it with me. You can add me v:javainstalling and remark [Jar].