Preface
This article is a summary of the necessary knowledge points for Java programmer interviews. It explains in detail the principles of JVM, multi-threading, data structures and algorithms, distributed cache, design patterns, etc. I hope readers can gain a better understanding of the basic principles of Java by reading this book. A deeper, more comprehensive understanding.
The interviewer usually has a comprehensive understanding of the interviewer's knowledge structure in just two hours. If the interviewer is sloppy in answering questions and cannot get to the essence of the question, it will be difficult to fully express himself, which will ultimately affect the interview result. In response to this situation, this article strives to be concise when explaining knowledge points without being muddled. It introduces in detail the core knowledge points that Java programmers are often asked during interviews.
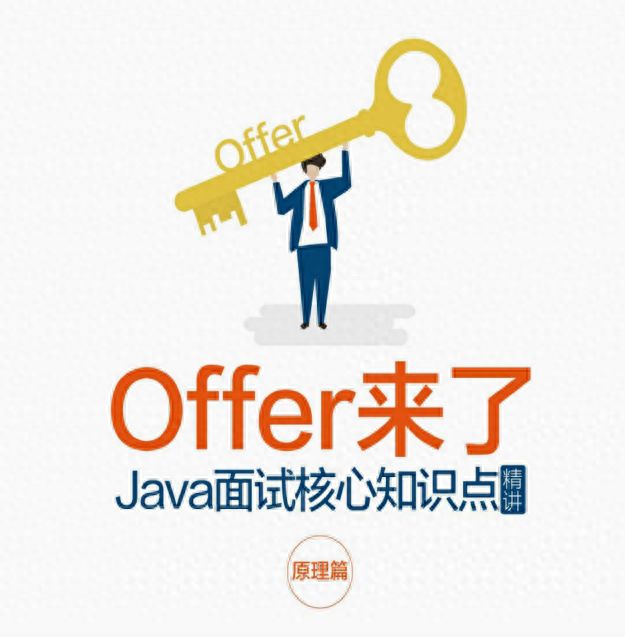
This article will be introduced in three parts: table of contents, main content, and suggestions for readers to read this article. I hope you will like it, and I hope it will help you learn!
Table of contents

main content
This article has 9 chapters in total, and the contents of each chapter are as follows.
Chapter 1 explains the principles of JVM, including JVM operating mechanism, JVM memory model, common garbage collection algorithms and JVM class loading mechanism.
1.1 JVM operating mechanism
1.2 Multi-threading
1.3 JVM memory area
1.4 JVM runtime memory
1.5 Garbage collection and algorithms
1.6 4 reference types in Java
1.7 Generational collection algorithm and partition collection algorithm
1.8 Garbage Collector
1.9 Java network programming model
1.10 JVM class loading mechanism
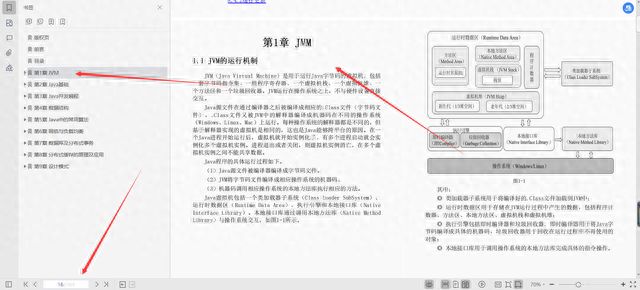
Chapter 2, this chapter will provide a detailed introduction to commonly used basic Java knowledge, including Java collections, exception classification and processing, reflection mechanism, annotations, internal classes, generics, and serialization.
2.1 Collection
2.2 Exception classification and processing
2.3 Reflection mechanism
2.4 Notes
2.5Inner classes
2.6 Generics
2.7 Serialization
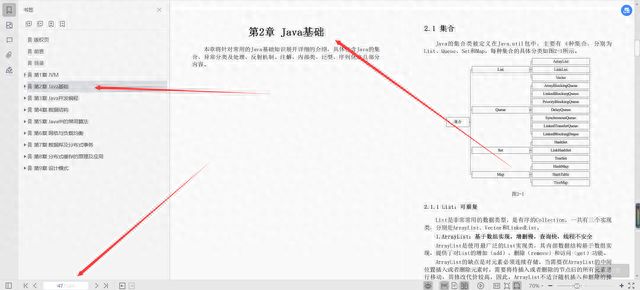
Chapter 3 Compared with traditional single thread, multi-threading can make better use of multiple CPU resources of the server based on the multi-core configuration of the operating system, making the program run more efficiently. Java provides support for multi-threading to concurrently execute multiple threads within a process. Each thread performs different tasks in parallel to meet the requirements for writing efficient programs.
3.1 How to create Java threads
3.2 How the thread pool works
3.3 5 commonly used thread pools
3.4 Thread life cycle
3.5 Basic methods of threads
3.6 Locks in Java
3.7 Thread context switching
3.8 Java blocking queue
3.9 Java Concurrency Keywords
3.10 How multiple threads share data
3.11 ConcurrentHashMap concurrency
3.12 Thread Scheduling in Java
3.13 Process Scheduling Algorithm
3.14What is CAS
3.15 ABA problem
3.16What is AQS
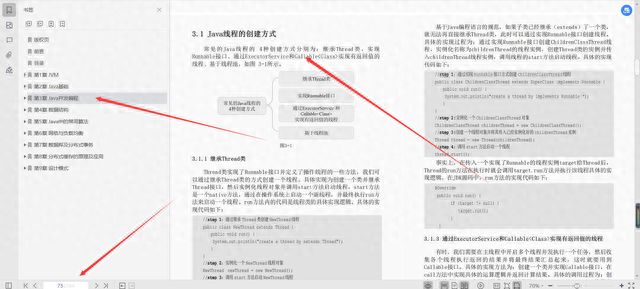
Chapter 4 Data structure refers to the way data is stored and organized. Some people think that "program = data structure + algorithm". Therefore, a good data structure is crucial to the running of the program, especially in complex systems. Designing excellent data structures can improve the flexibility and performance of the system. In the process of program design and development, it is inevitable to use various data structures. For example, sometimes you need to define your own data structure according to the characteristics of the product, so data structures are crucial to program design. This chapter will introduce in detail commonly used data structures, including stacks, queues, linked lists, binary trees, red-black trees, hash tables and bitmaps.
4.1 Stack and its Java implementation
4.2 Queue and its Java implementation
4.3 Linked list
4.4 Hash table
4.5 Binary sorting tree
4.6 Red-black tree
4.7 Figure
4.8 bitmap
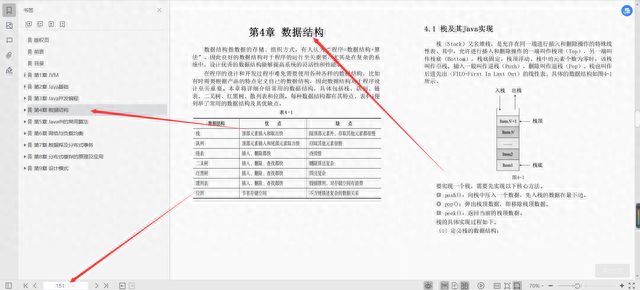
Chapter 5 In the computer world, "data structure + algorithm = program", therefore algorithms play a vital role in program development. Although we rarely design algorithms ourselves during development, we cannot do without algorithms at work. Whether it is the algorithm provided by the development package or the algorithm we design ourselves, algorithms are everywhere in the program.
Commonly used algorithms include search algorithms and sorting algorithms. Search algorithms include linear search algorithm, depth-first search algorithm, breadth-first search algorithm and binary search algorithm. Here we focus on the most commonly used and fastest binary search algorithm.
Sorting algorithms are very common algorithms and are applicable to everything from database design to sorting lists. Commonly used sorting algorithms include bubble sort, insertion sort, quick sort, Hill sort, merge sort, bucket sort, heap sort and radix sort. This chapter will introduce these algorithms in detail.
In addition, some algorithms that are essential in applications will also be introduced, such as pruning algorithm, backtracking algorithm, shortest path algorithm, maximum subarray algorithm and longest common factor algorithm.
5.1 Binary search algorithm
5.2 Bubble sort algorithm
5.3 Insertion sort algorithm
5.4 Quick sort algorithm
5.5 Hill sorting algorithm
5.6 Merge sort algorithm
5.7 Bucket sort algorithm
5.8 Radix Sort Algorithm
5.9 Other algorithms
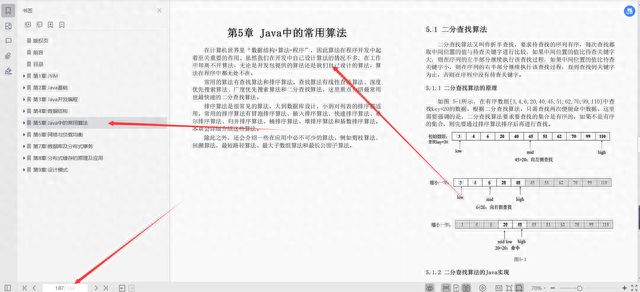
Chapter 6 In the computer field, the network is a virtual platform for information transmission, reception, and sharing. It connects information from various points, surfaces, and entities to realize the sharing of these resources. In large-scale distributed systems, the network plays a vital role. This chapter briefly introduces the commonly used 7-layer network architecture, as well as the principles of TCP/IP, HTTP and CDN. This is what we must understand to build a distributed system. , only by understanding these principles can we design a good system and perform more targeted system architecture tuning.
Load balancing is built on the existing network structure, providing a cheap, effective, and transparent method to expand the bandwidth of network devices and servers, increase throughput, enhance network data processing capabilities, and improve network flexibility and availability. Commonly used load balancing in projects include four-layer load balancing and seven-layer load balancing.
This chapter explains the principles of network and load balancing, covering TCP/IP, HTTP, common load balancing algorithms and LVS principles.
6.1 Network
6.2 Load balancing
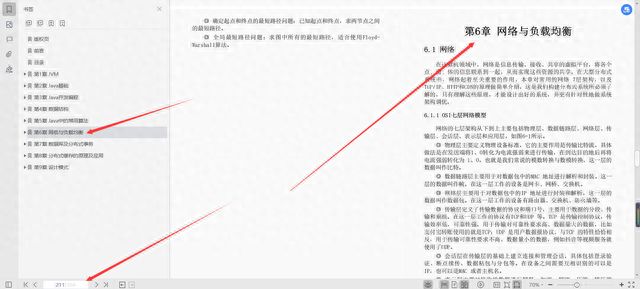
Chapter 7 Database is an essential component in software development. Whether it is a relational database MySQL, Oracle, PostgreSQL, or a NoSQL database HBase, MongoDB, or Cassandra, they all solve different problems for different application scenarios. This chapter will not introduce the use of these databases in detail, because readers have used these databases more or less, but the underlying principles of the database, especially the storage engine, database locks and distributed transactions, are easy to ignore, and these principles are very important to the database. It is more important for tuning and solving difficult problems, so this chapter will introduce the database storage engine, database index, stored procedure, database lock and distributed transaction. I hope readers can understand these principles at a higher level so that Make correct judgments when database performance bottlenecks occur.
7.1 Basic concepts and principles of database
7.2 Concurrent operations and locks of database
7.3 Database distributed transactions
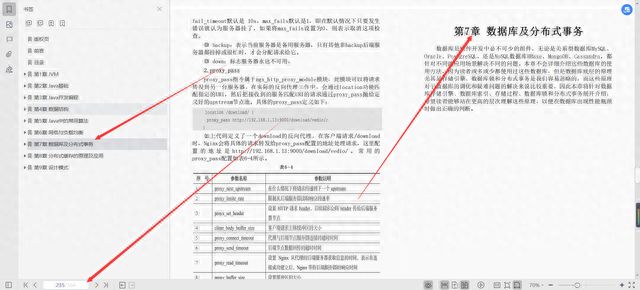
Chapter 8 Caching refers to a technology that stores frequently accessed data in memory to speed up user access. Caching is divided into process-level caching and distributed caching. Process-level caching refers to caching data within the service and realizing storage through structures such as Map and List. Distributed caching refers to storing cached data separately in a distributed system to facilitate the unification of the cache. Management and access. Commonly used distributed cache systems include Ehcache, Redis and Memcached.
8.1 Introduction to distributed cache
8.2 Principle and application of Ehcache
8.3 Principle and application of Redis
8.4 Core issues in distributed cache design
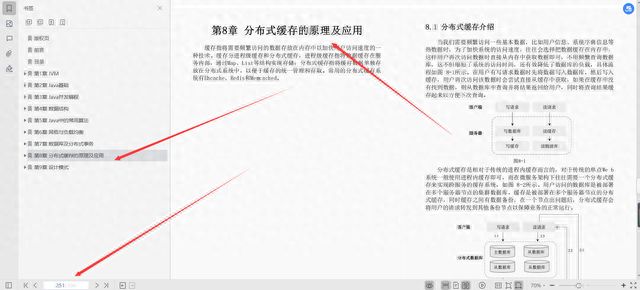
Chapter 9 Design Pattern is a highly abstract summary of code design experience that can be used repeatedly in programming.
The correct use of design patterns can effectively improve the readability, reusability and reliability of the code. Writing code that conforms to the design pattern specifications is not only beneficial to the stability and reliability of the own system, but also conducive to the docking of external systems. In system engineering that uses good design patterns, it is of great help whether it is to meet current needs or to adapt to future needs, whether it is the docking of modules between its own systems or the docking of external systems. .
This chapter explains design patterns, involving 23 common classic design patterns.
9.4 Concept and Java implementation of singleton pattern
9.5 Concept and Java implementation of builder pattern
9.6 Concept and Java implementation of prototype pattern
9.7 Concept and Java implementation of adapter pattern
9.8 Concept and Java implementation of decorator pattern
9.9 Concept and Java implementation of proxy pattern
9.10 Concept and Java implementation of appearance pattern
9.11 Concept and Java implementation of bridge mode
9.12 Concept and Java implementation of combination pattern
9.13 Concept and Java implementation of flyweight pattern
9.14 Concept and Java implementation of strategy pattern
9.15 Concept and Java implementation of template method pattern
9.16 Concept of Observer Pattern and Java Implementation
9.17 The concept and Java implementation of the iterator pattern
9.18 Concept and Java implementation of chain of responsibility model
9.19 Concept of command mode and Java implementation
9.20 Concept and Java implementation of memo pattern
9.21 Concept and Java implementation of state pattern
9.22 Concept and Java implementation of visitor pattern
9.23 Concept and Java implementation of mediator pattern
9.24 Concept and Java implementation of interpreter mode
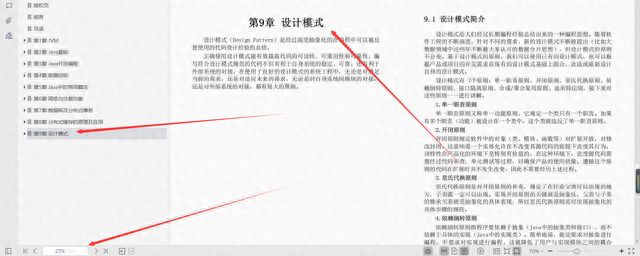
Suggestions for readers reading this article
The table of contents is detailed. Readers are advised to use the table of contents as a reference after reading this article to review the past and learn the new, so as to achieve a comprehensive understanding.
It is recommended that readers spend 3 weeks to read carefully and understand the knowledge points, code and architecture diagram in the article in detail;
Spend another two days reviewing, recalling knowledge points from the table of contents, and promptly checking and filling in any missing parts that you can’t remember;
Spend another 3 hours reviewing before the interview to fully grasp the knowledge points in this article.
In this way, readers can more fully understand the breadth and depth of each knowledge point in the article, and be confident and confident during the interview.
I hope you can read this article carefully, slowly understand the true meaning of this article, slowly increase the breadth and depth of your technology, and make yourself more valuable!
I hope this article can help everyone, please study hard! !
Due to space limitations, it is not possible to display all the information in the article. The information in the article has been organized, packaged and stored in the network disk. Friends who need the full version of the PDF can click on the business card below to pick it up!