Article directory
- python installation
- Output function print()
- Escape characters and original characters
- Binary and character encoding
- Identifiers and reserved words
- Definition and use of variables
- type of data
- Comment
- Use of input function
- operator
- program organization structure
- list
- dictionary
- Common operations on dictionaries
- tuple
- gather
- relationship between sets
- string
-
- String creation and persistence mechanisms
- Common operations on strings
- String case conversion
- String content alignment operations
- String splitting operation
- String judgment operation
- Other operations on strings
- String comparison operations
- String slicing operation
- Format string
- String encoding conversion
- function
- bug
- object
- module
- Run as main program
- packages in python
- Commonly used content modules in python
- Installation and use of third-party modules
- document
- Common methods of file objects
- with statement
- Common functions of the os module
python installation
idle: a simple development environment that comes with python
Output function print()
1. Print will output
- number
- String (must be quoted)
- Expressions containing operators (for example, 3+1 where 3 and 1 are operands and + is the operator) (operate)
#输出数字
print (98.5)
#输出字符串
print ("Hello, clovershrub")
print('hello,world')
#输出表达式
print(3+1)
2. The print() function can also set the destination of the content output
- monitor
- document:
fp=open(‘D:/text.txt’,”a+”)#文件不存在就创建,存在就在文件内容后面继续追加
print(‘helloworld‘,file=fp)
fp.close()
Note: The specified drive letter exists, use file=fp
3. The output form of the print() function
- newline
- No line breaks: use, split,
print(‘hello’,’world‘,’Python‘)
Escape characters and original characters
+ escape the first letter of the function
换行:\n(newline)
回车:\r (return)
如print(‘hello\rworld’) 在hello的下一行输出world
水平制表符:\t (tab)
如print(‘hello\tworld’) 输出 hello world
而print(helloooo\tworld) 输出helloooo world
因为hell这四个字母成为一个字表位,o后\t 占用了其余三个
若是无剩余 则会新开一个字表位
退格:\b (backspace)
如print(‘hello\bworld’) 退格输出hellworld
*原字符:不希望字符串中转义字符起作用,就会使用原字符,即在字符串上加上R或者r
如print(r‘hello\nworld’) 输出hello\nworld
Binary and character encoding
8bit (bit) — 1 byte (byte) — 256 states — 256 symbols 1024byte
— 1KB
1024KB — 1MB
1024MB — 1GB
1024GB — 1TB
Identifiers and reserved words
1. Reserved words: given special meaning and cannot be used in object naming
- Create new keyword.demo
- import keyword
- print(keyword.kwist) outputs a keyword list
2. The names given to variables/functions/classes/modules and other objects are called identifiers
3. Rules: - Letters, numbers, underline
- cannot start with a number
- Cannot be a reserved word
- Must be strictly case sensitive
Definition and use of variables
type of data
Integer type - int - 98, can represent positive numbers, negative numbers, 0
Floating point type - float - 3.1415926
Boolean type - bool - True, False
String type - str - "Life is short, I use Python" (with quotation marks, whether it is single quotation mark, double quotation mark or triple quotation mark, it is a string)
integer type
1. Representation of integers in different bases
- Decimal - Default
- Binary - starts with 0b
- Octal - starts with 0o
- Hexadecimal - starting with 0x
floating point type
Boolean type
string
type conversion
str()
Different from other languages, when strings and other data types are connected through "+", other data types will not be automatically converted to strings, but an error will be reported. The solution is to perform type conversion.
a=10
b=198.8
c=False
print(type(a),type(b),type(c))
# 输出:<class ‘int’><class ‘float’> <class ‘bool’>
print(str(a),str(b),str(c),type(a),type(b),type(c))
# 输出:10 198.8 False <class ‘str’> <class ‘str’> <class ‘str’>
int()
Convert str to int type. str must be an integer string, otherwise an error will be reported. Converting float to int will intercept the integer part of float.
s1='128'
f1=98.7
s2="45.6"
ff=True
s3="hello"
print(type(s1),type(f1),type(s2),type(ff),type(s3))
print(int(s1),type(int(s1))) #字符串为数字串
print(int(f1),type(int(f1))) #截取整数部分,小数舍掉
#print(int(s2),type(int(s2))) #字符串为小数串
print(int(ff),type(int(ff)))
#print(int(s3),type(int(s3))) #字符串必须为数字串且为整形
float()
- Conversion is not allowed for non-numeric strings
- Boolean value true is converted to 1.0, false is converted to 0.0
s1='128.98'
s2="76"
ff=True
s3="hello"
i=98
print(type(s1),type(s2),type(ff),type(s3),type(i))
print(float(s1),type(float(s1)))# 128.98
print(float(s2),type(float(s2)))# 76.0
print(float(ff),type(float(ff)))# 1.0
#print(float(s3),type(float(s3))) #非数字串不允许转换
print(float(i),type(float(i))) # 98.0
Comment
Contains three types of comments
- Single line comments - start with # and end with newline
- Multi-line comments - a pair of three-quote code multi-line comments
'''多行注释
多行哦'''
- Chinese encoding declaration comment - specify the encoding format of the source code file at the beginning of the file
Use of input function
The parameters received by input() are questions asked by the program to the user, and the user's input will be assigned to the present variable.
#输入函数input
prasent=input('大圣想要什么礼物呢?')
print(prasent,type(prasent))# 输出:用户键盘输入的内容,类型为str
# 从键盘录入两个整数,计算两个整数的和
a=int(input('请输入一个加数:'))
b=int(input('请输入一个被加数:'))
print(type(a),type(b))
print(a+b)
#print(int(a)+int(b))
operator
Operators in Python: arithmetic operators, assignment operators, comparison operators, Boolean operators, bitwise operators
arithmetic operators
Standard arithmetic operators: +、-、*、/、整除//
remainder operator: %
exponentiation operator:**
print(1+1) #加
print(1-1) #减
print(2*4) #乘
print(11/2) #除
print(11//2) #整除运算
print(11%2) #取余
print(2**3) #幂运算 2的3次方
print(9//4)# 2
print(-9//-4)# 2
#一正一负进行整除时,向下取整
print(9//-4)# -3
print(-9//4)# -3
# 一正一负进行取余时,遵循公式:公式 余数=被除数-除数*商
print(9%-4)# 9-(-4)*(-3) 9-12-->-3
print(-9%4)# -9-4*(-3) -9+12-->3
assignment operator
a=3+4
print(a)# 7
b=c=d=20
# 以下3个输出都是同一个值
print(d,id(d))# 14645450672
print(b,id(b))# 14645450672
print(c,id(c))# 14645450672
a=20
a+=30
print(a)# 50
a*=2
print(a)# 100
a/=30
print(a)#3.33333333
a%=3
print(a)# 0.11111111
# 支持系列解包赋值,变量个数和值的个数一定要一致,否则会报错
a,b,c=1,2,3
print(a,b,c)# 1,2,3
# 系列解包赋值的用处:交换两个变量
a,b=10,20
print('交换前',a,b)# 10,20
a,b=b,a
print('交换后',a,b)# 20,10
comparison operator
The result of the comparison operator is of type Boolean
a,b=10,20
print('a>b吗?',a>b)
print(a<b)
print(a<=b)
print(a>=b)
print(a==b)# false
print(a!=b)# true
a=10
b=10
print(a==b) #True 说明,a与b的value相等
print(a is b) #True 说明:a与b的id标识相等
print(a is not b)# false
lst1=[11,22,33,44]
lst2=[11,22,33,44]
print(lst1==lst2)# true
print(lst1 is lst2 )# false
print(id(lst1))# 2525441564
print(id(lst2))# 2525665484
print(lst1 is not lst2)# true
boolean operator
a,b=1,2
print(a==1 and b==2)# true
print(a==1 and b<2)# false
print(a!=1 and b==2)# false
print(a!=1 and b!=2)# false
print(a==1 or b==2)# true
print(a==1 or b<2)# true
print(a!=1 or b==2)# true
print(a!=1 or b!=2)# false
#not 对bool类型操作数取反
f=True
f2=False
print(not f)# false
print(not f2)# true
s='helloworld'
print('w' in s)# true
print('k' in s)# false
print('w' not in s)# false
print('k' not in s)# true
Bit operations
print(4&8) # 输出0。按位与&,同时为1结果为1
print(4|8) # 输出12。按位或|,同时为0结果为0
print(4<<1) # 输出8。向左移动1位 相当于乘以2
print(4<<2) # 输出16。向左移动2位
print(4>>1) # 输出2。向右移动1位,相当于除以二
print(4>>2) # 输出1。除以4
operator precedence
Summary: (), arithmetic operations, bit operations, comparison operations, bool operations, assignment operations
- ()
- ** A assignment operator: multiplication and division first, then addition and subtraction, exponentiation first if there is any exponentiation operation
- *、/、//、%
- +、-
- <<, >> B bit operators
- &
- |
-
,< ,>=,<=,==,! =C comparison operator
- and
- or D Boolean operator
- =E assignment operator
program organization structure
Any simple or complex algorithm can be composed of sequential structure, selection structure (if statement) and loop structure (while for-in statement)
sequential structure
Program starts - code 1 - code 2 - code 3... code N - program ends
Boolean value of object
Everything in Python is an object. All objects have a Boolean value.
To get the Boolean value of an object, use the built-in function bool()
#以下对象bool值均为False
print(bool(False))
print(bool(0))
print(bool(0.0))
print(bool(None))
print(bool(''))
print(bool(""))
print(bool([])) #空列表
print(bool(list())) #空列表
print(bool(())) #空元组
print(bool(tuple())) #空元组
print(bool({
})) #空字典
print(bool(dict())) #空字典
print(bool(set())) #空集合
#其他对象bool值均为True
branch structure
if conditional expression:
if the condition is met, execute the indented code
else:
if the condition is not met, execute the indented code in else
#单分支结构
money=1000
s=int(input('请输入取款金额:'))
if money>=s:
money=money-s
print('取款成功,余额为:',money)
#双分支结构
num=int(input('请输入一个整数:'))
if num/2==0:
print(num,'是偶数')
else:
print(num,'是奇数')
#多分支结构
score=float(input('请输入一个成绩:'))
if 90<=score<=100:
print('A')
elif 80<=score<90:
print('B')
elif 70<=score<80:
print('C')
elif 60<=score<70:
print('D')
elif 0<=score<60:
print('E')
else:
print('不是有效成绩')
#嵌套if的使用
answer=input('您是会员吗?y/n')
money=float(input('请输入您的购物金额:'))
if answer=='y':
if money>=200:
print(money*0.8)
elif money>=100:
print(money*0.9)
else:
print(money)
else:
if money>=200:
print(money*0.95)
else:
print(money)
Conditional expressions and pass statements
The conditional expression is if...else (abbreviation), syntax structure: x if 判断条件 else y
, if the Boolean value of the judgment condition is True, the return value of the conditional expression is x, otherwise the return value of the conditional expression is y
# 从键盘中输入两个整数,判断两个整数的大小
num1=int(input('请输入第一个整数'))
num2=int(input('请输入第二个整数'))
'''if num1>=num2:
print(num1,'大于等于',num2)
else:
print(num1,'小于',num2)'''
print(str(num1)+'大于等于'+str(num2) if num1>=num2 else str(num1)+'小于'+str(num2))
'''
pass语句什么都不做,只是一个占位符,用在需要语句的地方
#使用时间:先搭建语法结构,还没好代码怎么写的时候
配套使用:
1. if 语句的条件执行体
2. for--in语句的循环体
3. 定义函数的函数体
'''
answer=input('您是会员吗?y/n')
if answer=='y':
pass
else:
pass
Use of range() function
#range()的三种创建方式
# 第一种创建方式
r=range(10) #默认从零开始,默认相差1称为步长
print(r) #range(0,10)
print(list(r)) #用于查看range对象中的整数序列 -->list是列表的意思
# 输出:[0,1,2,3,4,5,6,7,8,9]
# 第二种创建方式
r=range(1,10) #指定起始值,从1开始到10结束(不包含10)
print(list(r))
# 输出:[1,2,3,4,5,6,7,8,9]
#第三种创建方式
r=range(1,10,2) #步长为2
print(list(r))# 输出:[1,3,5,7,9]
# 判断指定的m
print(9 in r)
print(8 not in r)
while loop
sum=0
a=0
while a<5:
sum+=a
a+=1
print(sum)
# 1到100之间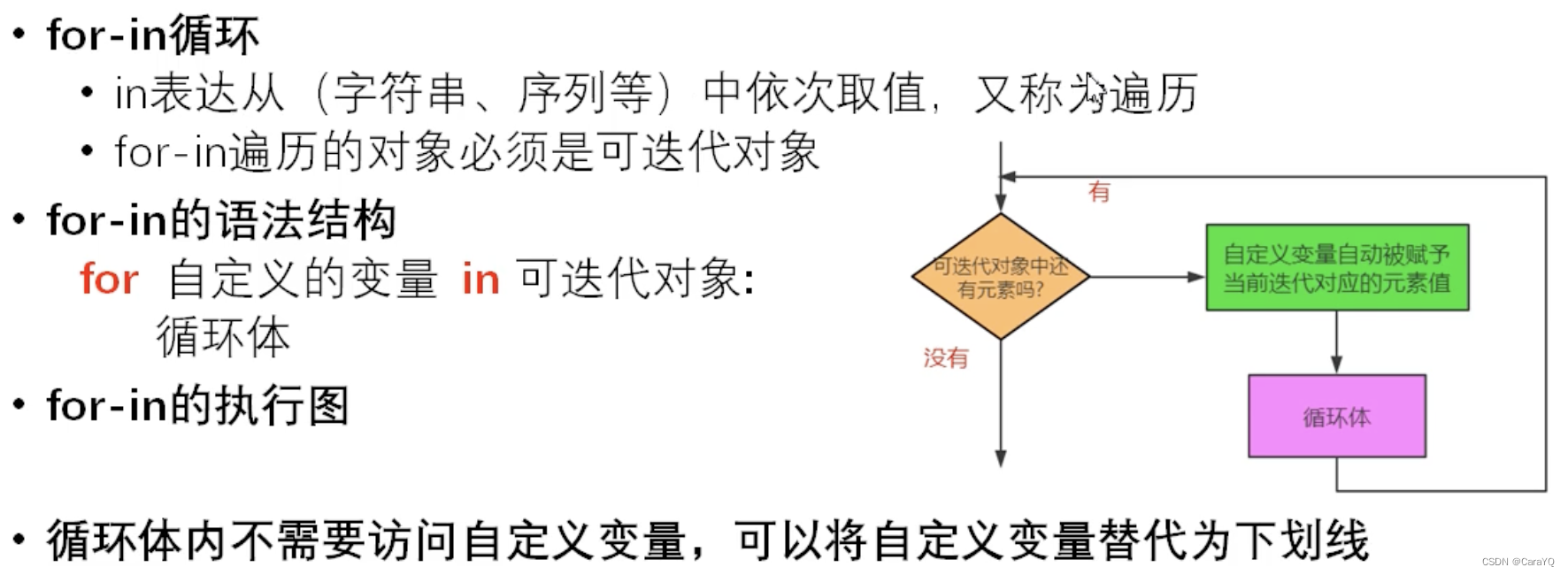
的偶数和
sum=0
a=1
while a<=100:
if not bool(a%2): # if a%2==0:
sum+=a
a+=1
print(sum)
for_in loop
for item in 'python':
print(item)
# range()产生的整数序列,也是一个可迭代对象
for i in range(10):
print(i)
# 如果在循环体中不需要使用到自定义变量,可将自定义变量写为“_“
for _ in range(5):
print('人生苦短')
# 100到999之间的水仙花数
for item in range(100,1000):
ge=item%10
shi=item//10%10
bai=item//100
#print(bai,shi,ge)
if ge**3+shi**3+bai**3==item:
print(item)
Flow control statements break and continue
a=0
while a<3:
pwd=input('请输入密码:')
if pwd=='8888':
print('密码正确')
break
else:
print('密码不正确')
a+=1
for item in range (1,51):
if item%5!=0:
continue
print(item)
else statement
Used with while and for: as long as the loop is executed normally, else will be executed.
for item in range(3):
pwd=input('请输入密码:')
if pwd=='8888':
print('密码正确')
break
else:
print('密码不正确')
else:
print('对不起,三次密码均输入错误')
Nested loops
for i in range(1,4): #行表
for j in range(1,5):
print('*',end='\t') #不换行输出
print() #打行
for i in range(1,10):
for j in range(1,i+1):
print(i,'*',j,'=',i*j,end='\t')
print()
Break and continue of double loop
The break and continue in the double loop are used to control the loop of this level.
for i in range(5):
for j in range(1,11):
if j%2==0:
#break
continue
print(j,end='\t')
print()
list
List creation
# 列表对象
# 创建列表的第一种方式,[]
lst=['hello','world',98,'hello']
print(id(lst))
print(type(lst))
print(lst)
print(lst[0],lst[-4])
# 创建列表的第二种方式,list()
lst2=list(['hello','world',98])
print(id(lst2))
print(type(lst2))
print(lst2)
List features
Get a single element in a list
lst=['hello','world',98,'hello']
print(lst.index('hello')) #列表中有相同元素只返回列表中相同元素的的第一个元素的索引
# print(lst.index('python')) #ValueError: 'python' is not in list
# print(lst.index('hello',1,3)) #ValueError: 'hello' is not in list 1-'world' 2-98 不包括3
print(lst.index('hello',1,4))
lst=['hello','world',98,'hello','world',234]
# 获取索引为2的
print(lst[2])
# 获取索引为-3的
print(lst[-3])
# 获取索引为10的
print(lst[10]) # IndexError: list index out of range
Get multiple elements in a list - slicing operation
lst=[10,20,30,40,50,60,70,80]
print(lst[1:6:1])# [20,30,40,50,60]
print(lst[1:6]) #默认步长为1 [20,30,40,50,60]
print(lst[1:6:])#默认步长为1 [20,30,40,50,60]
print(lst[1:6:2]) #步长为2 [20,40,60]
print(lst[:6:2])# [10,30,50]
print(lst[1::2])# [20,40,60,80]
print(lst[::2])# [10,30,50,70]
print(lst[::])# [10,20,30,40,50,60,70,80]
# 步长为负数
print(lst[::-1])# [80,70,60,50,40,30,20,10]
print(lst[7::-1])# [80,70,60,50,40,30,20,10]
print(lst[6:0:-2])# [70,50,30]
# 新切出来的是一个新的列表对象
print('原列表',id(lst))# 2005509464960
lst2=lst[1:6:1]
print('切的片段',id(lst2))# 2005510479424
Judgment and traversal of list elements
lst=[10,20,'python','hello']
print(10 in lst) #判断
print(10 not in lst)
for item in lst: #遍历
print(item)
Add, delete and modify list elements
Adding list elements
# 向列表末尾添加元素
lst=[10,20,30]
print(lst,id(lst))# 2181409792960
lst.append(100)
print(lst,id(lst))# 2181409792960
lst2=['hello','world']
# lst.append(lst2) # 将lst2作为一个元素添加到列表末尾 [10,20,30,['hello','world']]
lst.extend(lst2) # 向列表末尾添加多个元素 [10,20,30,'hello','world']
print(lst)
lst.insert(1,90) # 在任意位置上添加一个元素,在索引为1的位置上添加一个90
print(lst)# [10,90,20,30,'hello','world']
lst3=[True,False,'hello']
lst[1:]=lst3 # 在任意位置添加N个元素,从第一个元素开始后面的元素全删除(切掉),然后再添加元素
print(lst)# [10,True,False,'hello']
Deletion of list elements
lst=[10,20,30,40,50,60,30]
lst.remove(30) #从列表中移除一个元素,如果有重复元素只移除第一个元素
print(lst)# [10,20,40,50,60,30]
# lst.remove(100) #ValueError: list.remove(x): x not in list
#pop()根据索引移除元素
lst.pop(1)
print(lst)# [10,40,50,60,30]
# lst.pop(5) #IndexError: pop index out of range
lst.pop() #如果不指定参数,将删除最后一个参数
print(lst)# [10,40,50,60]
# 切片操作会产生一个新的列表对象,切片相当于从列表中取数
new_lst=lst[1:3]
print('原列表',lst)# [10,40,50,60]
print('切片后列表',new_lst)# [40,50]
# 不产生新的列表对象,而是删除原列表中的内容
lst[1:3]=[]
print(lst)# [10,60]
lst.clear() #清除列表中的所有元素
print(lst)
del lst #将列表对象删除
# print(lst) # NameError: name 'lst' is not defined. Did you mean: 'list'?
Modification operation of list elements
#修改一个值
lst=[10,20,30,40]
lst[2]=100
print(lst)# [10,20,100,40]
# 使用切片修改多个值
lst[1:3]=[300,400,500,600]
print(lst)# [20,300,400,500,600,40]
Sorting operations on list elements
lst=[20,40,10,98,54]
print('排序前',lst,id(lst))# [20,40,10,98,54] 1632705897408
lst.sort() #升序
print('排序后',lst,id(lst))# [10,20,40,54,98] 1632705897408
# 指定关键字参数,实现列表元素的降序排序
lst.sort(reverse=True) #降序
print(lst)# [98,54,40,20,10]
lst.sort(reverse=False) #升序
print(lst)# [10,20,40,54,98]
# 使用内置函数sorted()对列表进行排序,将产生一个新的列表
lst=[20,40,10,98,54]
new_lst=sorted(lst) #产生一个新列表 升序
print(lst)# [20,40,10,98,54]
print(new_lst)# [10,20,40,54,98]
# 指定关键字参数,实现列表元素的降序排序
desc_lst=sorted(lst,reverse=True) #降序
print(desc_lst)# [98,54,40,20,10]
List expression
lst=[i*i for i in range(1,10)]
print(lst)
lst2=[i for i in range(2,11,2)]
print(lst2)
dictionary
Variable sequence: a sequence that can be added, deleted, or modified.
The list is an ordered sequence.
The key is an immutable sequence, such as str. Immutable sequences cannot perform addition, deletion, and modification operations.
Dictionary creation
Using the built-in function dict() creates a dictionary in the form of assignment, so '' is not added on the left side, and whether it is added on the right side depends on the data type on the right side.
# 使用花括号
scores={
'张三':100,'李四':98,'王五':45}
print(scores)
# 使用内置函数
stu=dict(name='jack',age=20)
print(stu)
Common operations on dictionaries
Get dictionary elements
# 获取字典中的值,使用[]
print(scores['张三'])
# print(scores['陈六']) # KeyError: '陈六'
# 使用get()
print(scores.get('张三'))
print(scores.get('陈六')) # None
# 如果查找的key不存在,返回某个默认值
print(scores.get('马七',99)) # 99是在查找‘马七’所对的value不存在时,提供的一个默认值
Add, delete, and modify dictionary elements
scores={
'张三':100,'李四':98,'王五':45}
print("张三" in scores)# true
print("张三" not in scores)# false
del scores['张三'] # 删除指定的key-value对,输出:{'李四':98,'王五':45}
# scores.clear() # 清空字典的元素
print(scores)
scores['陈六']=98 #新增元素
print(scores)# {'李四':98,'王五':45,'陈六':98}
scores['陈六']=100 # 修改元素
print(scores)# {'李四':98,'王五':45,'陈六':100}
Get dictionary view
scores={
'张三':100,'李四':98,'王五':45}
# 获取所有的key
keys=scores.keys()
print(keys)# dict_keys(['张三','李四','王五'])
print(type(keys))# dict_keys
# scores.keys()得到的数据的类型是dict_keys,我们可以将所有的key组成的视图转成列表
print(list(keys))# ['张三','李四','王五']
# 获取所有的value
values=scores.values()
print(values)# dict_values([100,98,45])
print(type(values))# dict_values
print(list(values))# [100,98,45]
#获取所有的key-value对
items=scores.items()
print(items)# dict_items([('张三':100),('李四':98),('王五':45)])
print(list(items)) # 转换之后的列表元素是由元组组成:[('张三':100),('李四':98),('王五':45)]
Traversing dictionary elements
item is the name of the key traversed, score is the name of the dictionary to be traversed
scores={
'张三':100,'李四':98,'王五':45}
# 字典元素的遍历
for item in scores:
print(item,scores[item],scores.get(item))
Dictionary Features
d={
'name':'张三','name':'李四'} # key不允许重复,重复的key会覆盖原来的值
print(d)# {'name':'李四'}
d={
'name':'张三','nikename':'张三'} # value允许重复
print(d)# {'name':'李四','name':'张三','nikename':'张三'}
dictionary generation
items=['Fruits','Books','Others']
prices=[96,78,85,100,120]
# 遍历两个list中的值,分别存放在item,price中,item,price是要放在字典中的,所以最外层用了{},里面写作item:price,如果想要items大写,那么使用item.upper()
d={
item.upper():price for item,price in zip(items,prices)}
print(d)# 当两个list的个数不一致时,以短的那个为准
lst=zip(items,prices)
print(list(lst))
tuple
'''可变序列 列表,字典:可以进行增删改,即列表/字典进行增删改后,列表/字典在内存中的地址不变'''
lst=[10,20,45]
print(id(lst))# 1808459865024
lst.append(200)
print(id(lst))# 1808459865024
'''不可变序列 字符串,元组:不可以进行增删改,
即不可变序列进行增删改并非在原序列上操作,而是在内存中新开辟一个序列,所以不可变序列进行增删改后在内存中的地址会改变'''
s='hello'
print(id(s))# 2753280992112
s=s+'world'
print(id(s))# 2753284295600
print(s)
How tuples are created
# 第一种,用()
t=('Python','world',98)
print(t)# ('Python','world',98)
print(type(t))# tuple
t2='Python','world',98 # 省略了小括号
print(t2)# ('Python','world',98)
print(type(t2))# tuple
t=('Python',) # 只包含一个元组的元素需要使用逗号和小括号,不然为str类型(此处)
print(t)# ('Python')
print(type(t))# tuple
# 第二种创建方式,内置函数tuple()
t1=tuple(('Python','world',98))
print(t1)# ('Python','world',98)
print(type(t1))# tuple
# 空列表
lst=[]
lst1=list()
# 空字典
d={
}
d1=dict()
# 空元组
t=()
t1=tuple()
print('空列表',lst,lst1)
print('空字典',d,d1)
print('空元组',t,t1)
Why should tuples be designed as immutable sequences?
Once an immutable type object is created, all data within the object cannot be modified, which avoids errors caused by modifying the data. In addition, for immutable type objects, in a multi-tasking environment, there is no need to lock when operating objects at the same time.
The data stored in the tuple is (10, [20, 30], 9), 10 and 9 are both immutable objects. Other values cannot be referenced. If I want to change the data at [20,30] to 100, this is not possible because [20,30] is a mutable object, and the reference of a mutable object cannot be changed. It can only be 100 is appended to [20,30], and the memory address originally pointing to [20,30] in the tuple remains unchanged.
t=(10,[20,30],9)
print(type(t))# tuple
print(t[0],type(t[0]),id(t[0]))# 10 int 140716189915072
print(t[1],type(t[1]),id(t[1]))# [20,30] list 2223714708224
print(t[2],type(t[2]),id(t[2]))# 9 int 140716189915040
# 尝试将t[1]修改为100
print(id(100))# 140716189917952
#t[1]=100 #元组不允许修改元素
#由于[20,30]列表,而列表是可变序列,所以可以向列中添加元素,而列表的内存地址不变
t[1].append(100) #向列表中添加元素
print(t,id(t[1]))# [20,30,100] 2223714708224
Traversal of tuples
#元组的遍历
t=('Python','world',98)
#1.索引
print(t[0])
print(t[1])
print(t[2])
# print(t[3]) #IndexError: tuple index out of range
#2.遍历
for item in t:
print(item)
gather
# 集合的创建方式
# 1.{}
s={
2,3,4,5,6,7,7} #集合中的元素不允许重复
print(s)# {2,3,4,5,6,7}
#2.set()
s1=set(range(6))
print(s1,type(s1))# {0,1,2,3,4,5} set
# 通过set()将列表中的元素转成集合
s2=set([1,2,4,5,5,5,6,6])
print(s2,type(s2))# {1,2,3,5,6} set
# 通过set()将元组中的元素转成集合
s3=set((1,2,4,4,5,65)) #集合中的元素是无序的
print(s3,type(s3))# {1,2,4,5,65} set
# 通过set()将字符串中的元素转成集合
s4=set('python')
print(s4,type(s4))# {'n','h','p','y','t','y'} set
# 通过set()将字典中的元素转成集合
s5=set({
12,4,34,55,66,44,4})
print(s5,type(s5))# {34,66,4,55,12,44} set
# 定义一个空集合
s6={
} #dict字典类型
print(type(s6))# dict
s7=set()
print(type(s7))# set
Collection related operations
#集合的相关操作
#判断操作
s={
10,2,0,30,40,50}
print(10 in s)# true
print(100 in s)# false
print(0 not in s)# false
#新增操作
s.add(80) #一次添加一个
print(s)# {10,80,2,30,0,40,50},无序的
s.update({
200,300,4000}) #一次至少添加一个元素,把一个集合添加到指定集合中
print(s)# {200,300,10,80,2,30,0,40,50,4000},无序的
# 也可以以列表和元组的形式批量往里添加数据
s.update([100,99,6])
s.update((78,56,48))
print(s)# {78,200,56,300,10,100,80,48,2,6,30,0,40,99,50,4000}
#删除操作
s.remove(10)
print(s)# {78,200,56,300,100,80,48,2,6,30,0,40,99,50,4000}
# s.remove(500) #KeyError: 500
# print(s)
# 如果集合中有500则删除,没有也不报错
# s.discard(500) #没有,不报错
# print(s)
s.pop() #只能删除第一个,且不能添加参数
print(s)# {200,56,300,10,100,80,48,2,6,30,0,40,99,50,4000}
s.clear() #清空集合
print(s)# set()
relationship between sets
# 两个集合是否相等(元素相同,就相等)
s={
10,20,30,40}
s2={
20,30,40,10}
print(s==s2) #T
print(s!=s2) #F
#子集和超集
s1={
10,20,30,40,50,60}
s2={
10,20,30,40}
s3={
10,20,90}
print(s2.issubset(s1)) #T s2的所有数据都在s1中,所以s2是s1的子集
print(s3.issubset(s1)) #F
print(s1.issuperset(s2)) #T s2的所有数据都在s1中,所以s1是s2的超集
print(s1.issuperset(s3)) #F
#交集
print(s2.isdisjoint(s3)) #F - 有交集
s4={
100,200,300}
print(s2.isdisjoint(s4)) #T - 没有交集
Collection data operations
# 集合的数学操作
#(1)交集
s1={
10,20,30,40}
s2={
20,30,40,50,60}
print(s1.intersection(s2))# {40,30,20}
print(s1&s2)# &与intersection等价,都是求交集操作,输出:{40,30,20}
#(2)并集
print(s1.union(s2))# {40,30,20,10,50,60}
print(s1|s2)# |与union等价,都是求并集操作,输出:{40,30,20,10,50,60}
#(3)差集
print(s1.difference(s2)) #s1有s2没有,输出:[10]
print(s1-s2)# -与difference等价,都是求差集操作,输出:[10]
print(s2.difference(s1))# s2有s1没有,输出:[50,60]
#(4)对称差集
print(s1.symmetric_difference(s2)) #集合交集以外的元素,输出:[10,50,60]
print(s1^s2)# ^与symmetric_difference等价,都是求对称差集操作,输出:[10,50,60]
set production
s={
i*i for i in range(10)}
print(s)
Summarize
string
String creation and persistence mechanisms
#字符串
#字符串的定义及驻留机制
a='python'
b="python"
c='''python'''
print(a,id(a))# python 1874508798960
print(b,id(b))# python 1874508798960
print(c,id(c))# python 1874508798960
Residency mechanism: A way to save only one copy of the same and immutable string.
Interactive mode: Execute python under cmd to enter interactive mode.
Strings that match identifiers: String b containing alphanumeric underscores
is completed when the program is compiled. For splicing, c uses join to complete the splicing when the program is running. If a is c is false,
you can use sys to implement forced residence.
Common operations on strings
String query operations
#字符串查询操作
s='hello,hello'
print(s.index('lo')) #第一次出现的位置,输出:3
print(s.find('lo'))#第一次出现的位置,输出:9
print(s.rindex('lo')) #最后一次出现的位置
print(s.rfind('lo'))#第一次出现的位置,输出:9
# print(s.index('k')) #ValueError: substring not found 不存在
print(s.find('k')) #-1 不存在
String case conversion
#字符串的大小写转换操作
s='HelloWorld,wa'
print(s.upper(),id(s.upper())) #全转成大写会产生一个新的字符串对象,输出:HRLLOWORLD,WA 2133868981488
print(s,id(s))#helloworld,wa 2134125876016
print(s.lower()) #全转成小写,如果原来的字符串就是小写的,在执行了lower()后依旧会产生一个新的字符串对象,二者的id()依旧不一样
print(s.swapcase()) #大转小,小转大
print(s.capitalize()) #第一个字符大写,其余小写
print(s.title()) #每个单词首字母大写,其余小写
String content alignment operations
#字符串内容对齐操作
s='hello,Python'
#居中对齐
print(s.center(20,'*')) #****hello,Python****
#左对齐
print(s.ljust(20,'*')) #hello,Python********
print(s.ljust(10))#hello,Python
print(s.ljust(20))#hello,Python
#右对齐
print(s.rjust(20,'*'))#********hello,Python
print(s.rjust(20))# hello,Python
print(s.rjust(10))#hello,Python
#右对齐,左边用0填充
print(s.zfill(20))#00000000hello,Python
print(s.zfill(10))#hello,Python
print('-8910'.zfill(8))#-0008910
String splitting operation
#字符串的劈分操作
s='hello world Python'
print(s.split())# 默认以空格拆分,输出:['hello','world','Python']
s1='hello|world|Python'
print(s1.split())# ['hello world Python']
print(s1.split(sep='|'))# 指定分隔符,['hello','world','Python']
print(s1.split(sep='|',maxsplit=1))#['hello','world|Python']
#从右侧分
print(s1.rsplit(sep='|',maxsplit=1))# ['hello|world','Python']
String judgment operation
#字符串判断的相关方法
#合法标识符
s='hello,python'
print(s.isidentifier()) #F
print('hello'.isidentifier()) #T
print('张三_123'.isidentifier()) #T
#空白字符
print('\t'.isspace()) #T
print(' '.isspace()) #T
#字母
print('abc'.isalpha()) #T
print('张三'.isalpha()) #T
print('张三1'.isalpha()) #F
#十进制数字
print('123'.isdecimal()) #T
print('123四'.isdecimal()) #F
print('ⅡⅢⅣ'.isdecimal()) #F
#数字
print('123'.isnumeric()) #T
print('123四'.isnumeric()) #T
print('ⅡⅢⅣ'.isnumeric()) #T
print('壹贰叁'.isnumeric()) #T
#字母和数字
print('abc1'.isalnum()) #T
print('张三123'.isalnum()) #T
print('abc!'.isalnum()) #F
Other operations on strings
#字符串的其他操作
#字符串的替换
s='hello,Python'
print(s.replace('Python','Java'))# 用Java替换python,输出:hello,Java
s1='hello,Python,Python,Python'
print(s1.replace('Python','Java',2)) #第三个次数指定替换次数,输出hello,Java,Java,Python
#字符串的合并
# 连接数组元素
lst=['hello','java','python']
print('|'.join(lst))# hello|java|python
print(''.join(lst))# hellojavapython
# 连接元组元素
t=('hello','java','python')
print(''.join(t))# hellojavapython
# 连接字符串的元素
print('*'.join('python'))# p*y*t*h*o*n
String comparison operations
#字符串的比较操作
print('apple'>'app') #T
print('apple'>'banana') #F, 97>98 F
print(ord('a'),ord('b')) #原始值这里是字母的ascll码
print(ord('刘'))
print(chr(97),chr(98))# 获取ascii码为97的值,即a。chr()和ord()互为相反的两个方法
print(chr(21016))
'''==和is的区别
==比较的是value
is 比较的是 id'''
a=b='python'
c='python'
print(a==b) #T
print(b==c) #T
print(a is b) #T
print(a is c) #T
print(id(a)) #2383540093936
print(id(b)) #2383540093936
print(id(c)) #2383540093936
String slicing operation
#字符串的切片操作
s='hello,python'
print(s[:5]) #没有指定起始位置,从0开始,输出:hello
print(s[6:]) #没有指定结束位置,所以切到字符串最后一个元素,输出:python
s1='!'
newstr=s[:5]+s1+s[6:]
print(newstr) # hello!python
print(id(s))# 1990114866608
print(id(s[:5]))# 1990110831472
print(id(s[6:]))# 1990110735152
print(id(s1))# 1989860883760
print(id(newstr))# 1990110735216
print(s[1:5:1]) #完整写法 从一切到四,步长为一,输出:ello
print(s[::2]) #从0开始切到最后,输出:hlopto
print(s[::-1]) #从最后一个元素开始往前到第一个元素,步长为1,输出:nohtyp,olleh
print(s[-6::1])#从索引为-6的开始,到字符串的最后一个元素,步长为1,输出:python
Format string
Formatted string: a string output in a certain format
#格式化字符串
# % 占位符
name='张三'
age=20
print('我叫%s,今年%d岁' % (name,age))
# {},0代表取format的第一个参数,所有用到0的地方都使用这个参数的值
print('我叫{0},今年{1}岁,我真的叫{0}'.format(name,age))
# f-string,前面加f代表格式化字符串
print(f'我叫{
name},今年{
age}岁')
#宽度和精度
print('%10d' % 99) #10个宽度,输出: 99
print('%.3f' % 3.1415926) #.3表示小数点后三位,输出:3.142
print('%10.3f' % 3.1415926) #宽度为10,保留三位小数,输出: 3.142
print('hellohello')
# 下面的0均指format第一个参数
print('{0:.3}'.format(3.1415926)) #.3表示一共三位数,输出:3.14
print('{0:.3f}'.format(3.1415926)) #.3f表示3位小数,输出:3.142
print('{0:10.3f}'.format(3.1415926)) #宽度是10位,3位是小数,输出
String encoding conversion
#字符串的编码转换
s='天涯共此时'
#编码
print(s.encode(encoding='GBK')) #GBK这种编码格式中,一个中文占两个字节
print(s.encode(encoding='UTF-8')) #UTF-8中,一个中文三个字节
#解码
#byte代表的就是一个二进制数据(字节类型的数据)
byte=s.encode(encoding='GBK') #编码
print(byte.decode(encoding='GBK')) #解码
byte=s.encode(encoding='UTF-8')
print(byte.decode(encoding='UTF-8'))
function
Function definition and calling
#函数的定义和调用
def calc(a,b): #a,b为形式参数,形参位置是在函数的定义处
c=a+b
return c
result=calc(10,20) #10,20称为实际参数的值,实参的位置是函数的调用处
print(result)
#函数参数的传递
res=calc(b=10,a=20) #=左边的变量名称为 关键字参数
print(res)
#函数参数传递的内存分析
def fun(arg1,arg2):
print('arg1=',arg1)
print('arg2=',arg2)
arg1=100
arg2.append(10)
print('arg1=',arg1)
print('arg2=',arg2)
n1=11
n2=[22,33,44]
print('n1=',n1)
print('n2=',n2)
print('-------')
fun(n1,n2)
print('n1=',n1)
print('n2=',n2)
'''在函数调用过程中,进行参数的传递
如果是不可变对象,在函数体的修改不会形象实参的值 arg1修改为100,不会影响n1的值
如果是可变对象,在函数体的修改会影响到实参的值 arg2的修改,append(10),会影响到n2的值'''
function return value
#函数的返回值
def fun(num):
odd=[]
even=[]
for i in num:
if i % 2: #bool(0)=False
odd.append(i)
else:
even.append(i)
return odd,even
print(fun([10,29,34,23,44,53,55]))
'''
(1)如果函数没有返回值【函数执行完毕后,不需要给调用处提供数据】 return可以省略
(2)如果函数有一个返回值,直接返回原类型
(3)如果函数有多个返回值,返回结果为元组
'''
def fun1():
print('hello')
#return
fun1()
def fun2():
return 'hello'
res=fun2()
print(res)
def fun3():
return 'hello','world'
print(fun3())
'''函数在定义时,是否需要返回值,视情况而定'''
Function parameter definition
#默认值参数
def fun(a,b=10): #b为默认值
print(a,b)
#函数的调用
fun(100)# 100,10
fun(20,30)# 20,30
Looking at the source code of print, we found that:
this end is the default value parameter, which defaults to line wrapping. If you don’t want to wrap the line, you can pass other values to end:
print('hello',end='\t')
print('world')
# 输出:hello world
#函数的参数定义
def fun(*args): #函数定义时,个数可变的位置参数
print(args)
fun(10)
fun(10,20,30)
def fun1(**args): #个数可变的关键字参数
print(args)
fun1(a=10)
fun1(a=20,b=20,c=40)
''' def fun2(*args,*a):
pass
报错,个数可变的位置参数,只能是一个
def fun2(*args,*a):
pass
报错,个数可变的关键字参数,只能是一个'''
def fun2(*args1,**args2):
pass
''' def fun3(**args1,*args2):
pass
在一个函数定义的过程中,既有个数可变的关键字参数,也有个数可变的位置形参,要求个数可变的位置形参,放在个数可变的关键字形参之前'''
#函数参数总结
def fun(a,b,c): # a,b,c在函数的定义处,所以是形式参数
print('a=',a)
print('b=',b)
print('c=',c)
#函数的调用
fun(10,20,30) #函数调用时的参数传递,称为位置传参
'''
输出:
a=10
b=20
c=30
'''
lst=[11,22,33]
fun(*lst) #函数调用时,将列表的每个元素都转换为位置实参传入
'''
输出:
a=11
b=22
c=33
'''
print('--------')
fun(a=100,c=300,b=200) #关键字实参
'''
输出:
a=100
b=200
c=300
'''
dic={
"a":111,'b':222,'c':333}
fun(**dic) #在函数调用时,将字典的键值对都转换为关键字实参传入
'''
输出:
a=111
b=222
c=333
'''
def fun4(a,b,*,c,d): #从*之后的参数,在函数调用时,只能采用关键字参数传递
print('a=',a)
print('b=',b)
print('c=',c)
print('d=',d)
#fun4(10,20,30,40) #位置实参传递
fun4(a=10,b=20,c=10,d=40) #关键字实参传递
fun4(10,20,c=30,d=40) #前两个位置实参传递,后两个关键字实参传递
'''函数定义时形参的顺序问题'''
def fun5(a,b,*,c,d,**args):
pass
def fun6(*args,**args1):
pass
def fun7(a,b=10,*args,**args1):
pass
variable scope
#变量的作用域
def fun(a,b):
c=a+b #c,就称为局部变量,因为c在函数体内进行定义的变量,a,b为函数的形参,作用范围也是函数内部,相当于局部变量
print(c)
name='刘' #全局变量
print(name)
def fun1():
print(name)
fun1()
def fun3():
global age #函数内部定义的变量,局部变量,其用global声明,这个变量变为全局变量
age=20
print(age)
fun3()
print(age)
recursive function
#递归函数
def fac(n):
if n==1:
return 1
else:
return n*fac(n-1)
print(fac(9))
#斐波那契数列
def fib(n):
if n==1:
return 1
elif n==2:
return 1
else:
return fib(n-1)+fib(n-2)
print(fib(6))
for i in range(1,7):
print(fib(i))
bug
#bug的常见类型
#1.
age=input('请输入你的年龄:')
print(type(age))
# if age>=18: #TypeError: '>=' not supported between instances of 'str' and 'int'
if int(age)>=18:
print('你是成年人了')
#2.
# while i<10:
# print(i)
i=0
while i<10:
print(i)
i+=1
#3.
for i in range(3):
uname=input('请输入用户名:')
pwd=input('请输入密码:')
# if uname='admin' and pwd='admin':
if uname=='admin' and pwd=='admin':
print('登陆成功!')
break
# else
else:
print('输入有误')
else:
print('对不起,三次均输入错误')
#4.索引越界问题IndexError
lst=[11,22,33,44]
# print(lst[4]) #list index out of range
print(lst[3])
#5.append()方法的使用不熟练
lst=[]
# lst=append('A','B','C')
lst.append('A')
lst.append('B')
lst.append('C')
print(lst)
#被动掉坑问题
''' n1=int(input('请输入一个整数:'))
n2=int(input('请输入另一个整数:'))
result=n1/n2
print('结果为:',result)'''
Python’s exception mechanism: try…exception
When there are multiple exceptions that need to be caught, multiple except structures can be used:
#try...except结构
try:
n1=int(input('请输入一个整数:'))
n2=int(input('请输入另一个整数:'))
result=n1/n2
print('结果为:',result)
except ZeroDivisionError:
print('对不起,除数不允许为零')
except ValueError:
print('不能将字符转换为数字')
print('程序结束')
#try...except...else结构
try:
n1=int(input('请输入一个整数:'))
n2=int(input('请输入另一个整数:'))
result=n1/n2
except BaseException as e:
print('出错了',e)
else:
print('结果为:',result)
#try...except...else...finally结构
try:
n1=int(input('请输入一个整数:'))
n2=int(input('请输入另一个整数:'))
result=n1/n2
except BaseException as e:
print('出错了',e)
else:
print('结果为:',result)
finally:
print('谢谢您的使用')
print('程序结束')
Common exception types in python
#常见的异常类型
# print(10/0) #ZeroDivisionError
lst=[11,22,33,44]
# print(lst[4]) #IndexError
dic={
'name':'张三','age':20}
# print(dic['gender']) #KeyError
# print(num) #NameError
# int a=20 #SyntaxError
#a=int('hello') #ValueError
Use of traceback module
#traceback模块的使用
import traceback
try:
print('--------------')
print(1/0)
except:
traceback.print_exc()
object
Everything in python is an object
Class creation
Whether you write self in the instance method or not, there will be a self (if you don’t write a program, you will get one for free). Self is not allowed to be written in the static method, and cls needs to be passed in the class method.
#定义python中的类
class Student: #Student为类的名称(类名)由一个或多个单词组成,每个单词首字母大写,其余小写
native_place='吉林' #直接写在类里的变量,成为类属性
def __init__(self,name,age):
self.name=name #self.name 称为实例属性,进行了一个赋值操作,将局部变量的name的值赋给实体属性/实例变量
self.age=age
#实例方法
def eat(self):
print(self.name+'在吃饭')
#静态方法
@staticmethod
def sm():
print('静态方法')
#类方法
@classmethod
def cm(cls):
print('类方法')
#在类之外定义的称为函数,再类之内定义的称为方法
def drink():
print('喝水')
#创建Student类的对象
stu1=Student('张三',20)
stu2=Student('李四',30)
print(id(stu1))# 1421362237888
print(type(stu1))# <class '__main__.Student'>
print(stu1)# <__main__.Student object at 0x0000014AEFCA91C0>
# 1421362237888的十六进制为0x0000014AEFCA91C0
# 实例对象会有一个类指针指向类对象
print(id(Student))# 2218905389200
print(type(Student))# <class ‘type’>
print(Student)# <class '__main__.Student'>
# 下面两行都可以调用类的实例方法:实例对象.实例方法 或者 类对象.实例方法(实例对象)
stu1.eat() #对象名.方法名()
Student.eat(stu1) #类名.方法名(类的对象)-->实际上就是方法定义处的self
print(stu1.name)
print(stu1.age)
#类属性的使用方式
#print(Student.native_place)
print(stu1.native_place)# 吉林
print(stu2.native_place)# 吉林
Student.native_place='天津'
print(stu1.native_place)# 天津
print(stu2.native_place)# 天津
#类方法的使用方式
Student.cm()
#静态方法的使用方式
Student.sm()
#动态绑定属性和方法
stu2.gender='女' #动态绑定性别属性
# print(stu1.name,stu1.age,stu1.gender) #AttributeError: 'Student' object has no attribute 'gender'
print(stu2.name,stu2.age,stu2.gender)
stu1.eat()
stu2.eat()
def show():
print('定义在类之外的,称为函数')
stu1.show=show #动态绑定方法
stu1.show()
# stu2.show() #AttributeError: 'Student' object has no attribute 'show'
Three major characteristics of object-oriented
encapsulation
#封装
class Car:
def __init__(self,brand):
self.brand=brand
def start(self):
print('汽车已启动...')
car=Car('宝马x5')
car.start()
print(car.brand)
class Student:
def __init__(self,name,age):
self.name=name
self.__age=age #年龄不希望在类的外部被使用,所以加__
def show(self):
print(self.name,self.__age)
stu=Student('张三',20)
stu.show()
#在类的外部使用name和age
print(stu.name)
# print(stu.__age) #AttributeError: 'Student' object has no attribute '__age'
# print(dir(stu)) #获取对象所有的属性和方法
print(stu._Student__age) #在类的外部可以通过 _Student__age 进行访问,输出:20
inherit
When defining a class, write the parent class in parentheses. For example, the parent class of Person above is object, and the parent class of Student is Person. Python supports multiple inheritance. For example, a inherits object, b inherits object, and c can inherit a and b at the same time:class c(a,b)
#继承
class Person(object):
def __init__(self,name,age):
self.name=name
self.age=age
def info(self):
print(self.name,self.age)
#定义子类
class Student(Person):
def __init__(self, name, age,score):
super().__init__(name, age)# 在子类的init方法中使用super().__init__调用父类的初始化方法进行赋值
self.score=score# 然后再对自己内部的属性进行初始化
def info(self): #方法的重写,重写父类的info()
super().info()# 如果还想用到父类的info()的方法体,使用super().info()调用
print(self.score)
class Teacher(Person):
def __init__(self, name, age,teachofyear):
super().__init__(name, age)
self.teachofyear=teachofyear
def info(self): #方法的重写
super().info()
print(self.teachofyear)
#测试
stu=Student('Jack',20,'1001')
stu.info()
tea=Teacher('Tom',40,20)
tea.info()
object class
#object类 -- 所有类的父类
class Student:
def __init__(self,name,age):
self.name=name
self.age=age
def __str__(self):
return '我的名字是{0},今年{1}岁'.format(self.name,self.age)
stu=Student('jack',20)
print(dir(stu))
print(stu)# 默认会调用__str__(self)方法,如果没有覆写,会输出实例对象的内存地址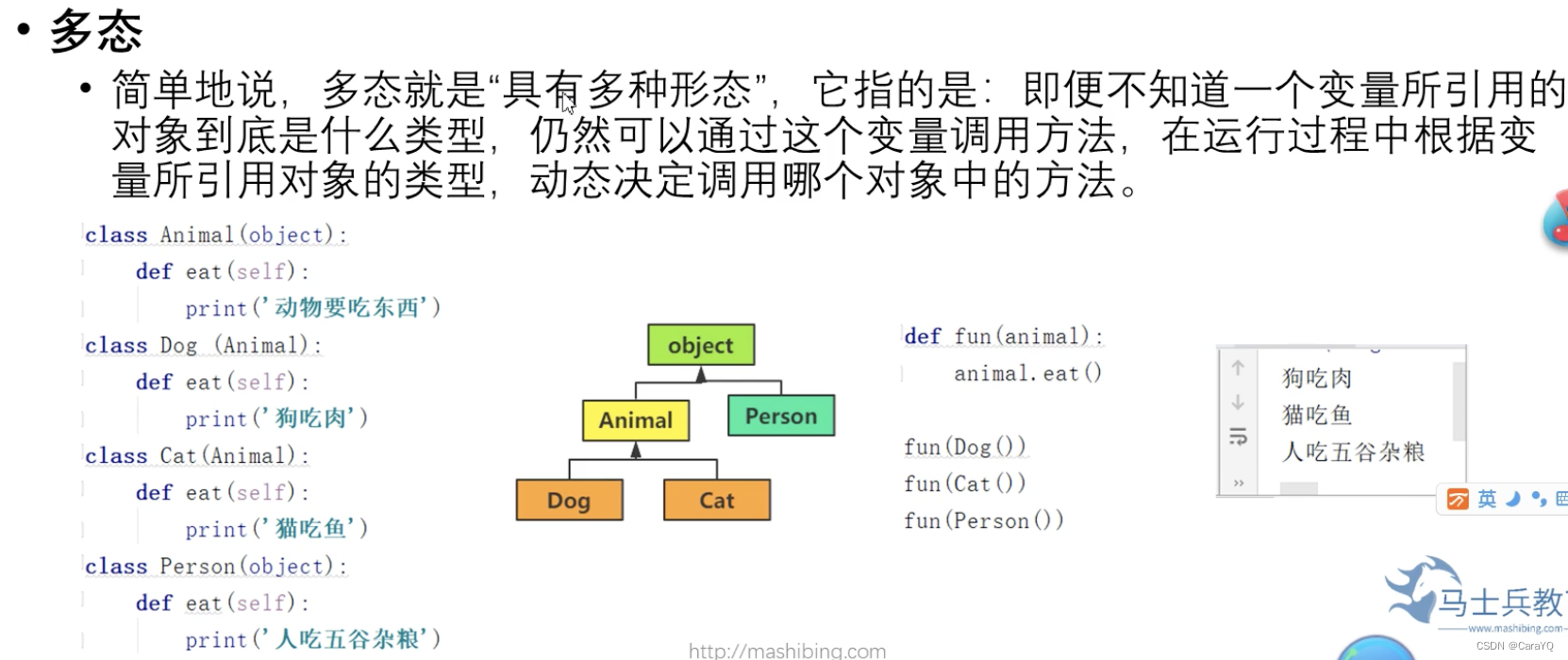
print(type(stu))
Polymorphism
A static language like Java needs three necessary conditions to achieve polymorphism: inheritance, method overriding, and parent class pointing to child class objects. However, python is a dynamic language. It only cares about whether you have this method and does not care about the rest.
#多态
class Animal(object):
def eat(self):
print('动物会吃')
class Dog(Animal):
def eat(self):
print('狗吃骨头')
class Cat(Animal):
def eat(self):
print('猫吃鱼')
class Person:
def eat(self):
print('人吃五谷杂粮')
#定义一个函数
def fun(obj):
obj.eat()
#调用
fun(Cat())# 动物会吃
fun(Dog())
fun(Animal())
fun(Person())
Special methods and special properties
#特殊属性
class A:
pass
class B:
pass
class C(A,B):
def __init__(self,name,age):
self.name=name
self.age=age
class D(A):
pass
#创建C类对象
x=C('jack',20) #x是C类型的一个实例对象
print(x.__dict__) #查看实例对象的属性字典
print(C.__dict__)# 查看类对象的属性字典
print(x.__class__) #输出对象所属的类型 <class '__main__.C'>
print(C.__bases__) #C类的父类类型的元组:(<class '__main__.A'>,<class '__main__.B'>)
print(C.__base__) #类的基类,C继承A和B,所以输出<class '__main__.A'>,如果C继承B和A,则输出<class '__main__.B'>,C多继承时,谁在前就输出谁
print(C.__mro__) #类的层次结构:(<class '__main__.C'>,<class '__main__.A'>,<class '__main__.B'>,<class 'object'>)
print(A.__subclasses__()) #子类的列表:[<class '__main__.C'>,<class '__main__.D'>]
#类的特殊方法
a=20
b=100
c=a+b #两个整数类型的对象的相加操作
d=a.__add__(b)# 两个整数类型的对象的相加操作,底层调用的是__add__()进行相加
print(c)# 120
print(d)# 120
class Student:
def __init__(self,name):
self.name=name
stu1=Student('张三')
stu2=Student('李四')
s=stu1+stu2 #报错:Student类型不能进行相加,但是我想要相加怎么办呢?给Student类定义一个__add__()方法,如下所示
print(len(stu1))# 同理,如果我想输出长度,也会报错,但是我又想输出怎么办呢?给Student类定义一个__len__()方法,如下所示
class Student:
def __init__(self,name):
self.name=name
def __add__(self,other): #特殊方法
return self.name+other.name
def __len__(self):
return len(self.name)
stu1=Student('张三')
stu2=Student('李四')
s=stu1+stu2 #实现两个对象的加法运算
print(s)# 张三李四
s=stu1.__add__(stu2)
print(s)# 张三李四
lst=[11,22,33,44]
print(len(lst))# 4,调用len(lst)时底层执行了lst.__len__()
print(lst.__len__())# 4
print(len(stu1))# 2
__new__ and __init__ create objects
class Person(object):
def __new__(cls,*args,**kwargs):
print('__new__被调用执行了,cls的id值为{0}'.format(id(cls)))
obj=super().__new__(cls)
print('创建的对象的id为:{0}'.format(id(obj)))
return obj
def __init__(self,name,age):
print('__init__被调用了,self的id值为:{0}'.format(id(self)))
self.name=name
self.age=age
print('object这个类对象的id为:{0}'.format(id(object)))
print('Person这个类对象的id为:{0}'.format(id(Person)))
#创建person类的实例对象
p1=Person('张三',20)
print('p1这个Person类的实例对象的id:{0}'.format(id(p1)))
'''
程序的执行结果:
object这个类对象的id为:140717796113232
Person这个类对象的id为:2690679899360
__new__被调用执行了,cls的id值为2690679899360
创建的对象的id为:2690436007104
__init__被调用了,self的id值为:2690436007104
p1这个Person类的实例对象的id:2690436007104
'''
Shallow copy and deep copy of class
#类的浅拷贝与深拷贝
class CPU:
pass
class Disk:
pass
class Computer:
def __init__(self,cpu,disk):
self.cpu=cpu
self.disk=disk
#(1)变量的赋值
cpu1=CPU
cpu2=cpu1
print(cpu1)# <__main__.CPU object at 0x000002B4D38D8490>
print(cpu2)# <__main__.CPU object at 0x000002B4D38D8490>
#(2)类的浅拷贝
disk=Disk() #创建一个硬盘类的对象
computer=Computer(cpu1,disk) #创建一个计算机类的对象
#浅拷贝
import copy
print(disk)# <__main__.Disk object at 0x000001782E443490>
computer2=copy.copy(computer)
print(computer,computer.cpu,computer.disk)# <__main__.Computer object at 0x000001781F3284C0> <__main__.CPU object at 0x000001781F238490> <__main__.Disk object at 0x000001782E443490>
print(computer2,computer2.cpu,computer2.disk)# <__main__.Computer object at 0x000001781F328190> <__main__.CPU object at 0x000001781F238490> <__main__.Disk object at 0x000001782E443490>
#深拷贝
computer3=copy.deepcopy(computer)
print(computer,computer.cpu,computer.disk)# <__main__.Computer object at 0x000001813E8284C0> <__main__.CPU object at 0x000001813E738490> <__main__.Disk object at 0x000001814D913490>
print(computer3,computer3.cpu,computer3.disk)# <__main__.Computer object at 0x000001813E8281F0> <__main__.CPU object at 0x000001814D943A30> <__main__.Disk object at 0x000001814EA626A0>
module
import math
print(id(math))
print(type(math))
print(math)
print(math.pi)
print(dir(math))
print(math.pow(2,3))
print(math.ceil(9.001))
print(math.floor(9.999))
from math import pi
# from math import pow
print(pi)
print(pow(2,3))
# print(math.pow(2,3))
If I customize a module calc.py now, I want to import it in the demo5.py module. If I write it directly, an import calc
error will be reported. I need to set the package where demo5.py is located as source root.
Run as main program
If a calc2.py is defined, the content is as follows:
def add(a,b):
return a+b
print(add(10,20))
Then we introduced it in demo7.py and executed it print(calc2.add(100,200))
. It will print out 30 300 because it first executed the printing of calc2.py. If we only want to output 300 and not let it execute the printing of calc2.py, we can so:
def add(a,b):
return a+b
if __name__ == '__main__': #只有在这个模块运行时,才执行下述代码
print(add(10,20))
packages in python
There are countless packages in a Python program, each package has countless modules, and each module has countless classes, methods, and statements. Create a package in python:
import the modules in the package in demo8.py
Commonly used content modules in python
import sys
import time
import urllib.request
import math
print(sys.getsizeof(24))# 28:24占了28个字节
print(sys.getsizeof(45))# 28
print(sys.getsizeof(True))# 28
print(sys.getsizeof(False))# 24
print(time.time())
print(time.localtime(time.time()))
print(urllib.request.urlopen('http://www.baidu.com').read())
print(math.pi)
Installation and use of third-party modules
#第三方模块的安装 pip install 模块名
import schedule
import time
def job():
print('hh')
schedule.every(3).seconds.do(job)
while True:
schedule.run_pending()
time.sleep(1)
document
Encoding format
When operating Chinese text files, garbled characters often appear.
How to read files
The interpreter calls the resources of the operating system to read the .py file and operate the file on the disk (read and write the file).
The content of a.txt is:
china
beautiful
#读
file=open('a.txt','r')
print(file.readlines())# ['中国\n','美丽'],输出一个数组
file.close()
Write hello to b.txt file
# 写
file=open('b.txt','w')# 如果没有b.txt文件则创建,如果有b.txt文件,因为第二个参数传的w,所以会清除文件原有的内容,重新写入新内容
file.write('hello')
file.close()
#追加
file=open('b.txt','a')# 如果没有b.txt文件则创建,如果有b.txt文件,因为第二个参数传的a,所以会在原有内容上追加新内容
file.write('hello')
file.close()
#复制图片
src_file=open('logo.png','rb')
target_file=open('copylogo.png','wb')
target_file.write(src_file.read())# 边读边写
target_file.close()
src_file.close()
Common methods of file objects
#读
file=open('a.txt','r')
print(file.read(5)) #读取size个字节或字符
print(file.readline()) #读取一行内容,输出:中国
print(file.readlines()) #把文本文件中的每一行都作为独立的字符对象,并将这些对象放入列表返回。输出:['中国\n','美丽']
#写
file=open('c.txt','a')
# file.write('hello')
lst=['java','go','python']
file.writelines(lst)# hellojavagopython
file.close()
#移动文件指针
file=open('c.txt','r')
file.seek(2) #将文件移动到新的位置,一个汉字占2字节,这里写1会报错,写2会从国开始输出
print(file.read())
'''
国
美丽
'''
print(file.tell()) #返回指针当前所处位置。如果文件写完了,file.tell()返回文件长度
file.close()
file=open('d.txt','a')
file.write('hello')
file.flush() #把缓冲区的内容写入文件,但不关闭文件
file.write('world')
file.close()
with statement
#with语句(上下文管理器)
with open('a.txt','r') as file:# 打开a.txt文件,为打开的这个文件起别名:file,file就是上下文管理器
print(file.read())
#不用手动关闭!
will open('a.txt','r')
be called a context expression, and its execution result file is a context manager
Context manager: If a class object implements
__enter__()
and For the context manager, first execute the method, then execute the with statement body, then exit the context manager and execute the method. If an exception occurs when executing the with statement body, the method will also be called, indicating that in the context manager, the method will be called regardless of whether there is an exception or not .__exit__()
__enter__()
__exit__()
__exit__()
__enter__()
__exit__()
Use the with statement to copy the aforementioned image:
with open('logo.png','rb') as src_file
with open('copylogo.png','wb') as target_file
target_file.write(src_file.read())# 边读边写
Common functions of the os module
#os模块与操作系统相关的一个模块
import os
os.system('notepad.exe')# 打开系统中的记事本(应用程序)
os.system('calc.exe')# 打开系统中的计算器(应用程序)
#直接调用可执行文件
os.startfile('C:\\Program Files (x86)\\Tencent\\QQ\\Bin\\QQ.exe')
import os
print(os.getcwd()) #返回当前的工作目录(绝对路径)
lst=os.listdir('python') #返回指定路径下的文件和目录信息,这里参数要写相对路径
print(lst)
os.makedirs('TXT/1.txt') #创建多级目录
os.mkdir('TXT/0.txt') #创建目录
os.rmdir('TXT') #删除目录
os.removedirs('TXT/1.txt') #删除多级目录
os.chdir('C:\\') #改变工作路径
print(os.getcwd())
Common methods of path module
#os.path模块
import os.path
print(os.path.abspath('1.py')) #获取文件的绝对路径
print(os.path.exists('1.py'),os.path.exists('2.py')) #判断文件是否存在T/F
print(os.path.join('C:\\download','1.py')) #目录和文件拼接操作,输出:C:\\download\\1.py
print(os.path.split('C:\\Users\\26066\\Desktop\\program\\VScode\\.vscode\\python\\helloworld.py')) #拆分路径和文件,输出:('C:\\Users\\26066\\Desktop\\program\\VScode\\.vscode\\python','helloworld.py')
print(os.path.splitext('helloworld.py')) #拆分文件和后缀名,输出:('helloworld','.py')
print(os.path.basename('C:\\Users\\26066\\Desktop\\program\\VScode\\.vscode\\python\\helloworld.py')) #提取文件名,输出:helloworld.py
print(os.path.dirname('C:\\Users\\26066\\Desktop\\program\\VScode\\.vscode\\python\\helloworld.py')) #提取目录名,输出:C:\\Users\\26066\\Desktop\\program\\VScode\\.vscode\\python
print(os.path.isdir('C:\\Users\\26066\\Desktop\\program\\VScode\\.vscode\\python')) #判断是否是路径(目录)
#获取当前目录下的.txt文件
import os
path=os.getcwd()# 获取当前目录
lst=os.listdir(path)# 获取路径下的所有文件
for filename in lst:
if filename.endswith('.txt'):# 如果文件名以.txt结尾
print(filename)
#
import os
path=os.getcwd()
lst_files=os.walk(path) #遍历目录下的所有文件夹及其子文件
for dirpath,dirname,filename in lst_files:
# print(dirpath)# 当前路径
# print(dirname)# 文件夹
# print(filename)# 文件
for dir in dirname:
print(os.path.join(dirpath,dir))
for file in filename:
print(os.path.join(dirpath,file))