Preface
Learning is like sailing against the current, especially since the IT industry is changing with each passing day. We must seize every opportunity to learn and improve. So there is no retreat.
Even if you change jobs during interviews, it is still a learning process. Only a comprehensive review can enable us to better enrich ourselves, arm ourselves, and make our interview journey no longer bumpy!
Even when looking for a new job opportunity, the interview process itself is a learning experience. Comprehensive review of relevant knowledge will help you perform better in interviews and pave the way for your future career.
A complete interview not only examines the technical strength, but also the soft skills of the interviewer. Many times, although your technical level has met the requirements, the interview always fails. There are usually two reasons:
- Insufficient preparation before interview
- During the interview, the intention of the interviewer's question was not truly understood, resulting in an answer that failed to hit the point. If this happens during the interview, it is because of a lack of basic interview skills.
In this year's interview, the main knowledge points involved are: JVM, multi-threading, database, microservices, distribution, message middleware, source code, etc. Today, I have compiled a set of interview tips for you!
In addition to the knowledge points mentioned above, this collection also contains real interview experiences from well-known companies such as Alibaba, Ant Financial, ByteDance, Pinduoduo, JD.com, Didi, Tencent and other well-known companies, as well as self-summaries and reflections. I hope this guide can help you better prepare for interviews and improve your interviewing abilities and skills.
Test abilities and skills.
1.Java related
-
The difference between ArrayList and Vector
-
Let’s talk about the storage performance and characteristics of ArrayList, Vector, and LinkedList
-
What is the difference between fail-fast and fail-safe?
-
hashmap data structure
-
How does HashMap work?
-
When will Hashmap be expanded?
-
What are the characteristics of each of the three interfaces List, Map, and Set when accessing elements?
-
The elements in Set cannot be repeated, so what method is used to distinguish whether they are repeated or not? Should you use == or equals()? What is the difference between them?
-
Two objects have the same value (x.equals(y) == true), but they can have different hash codes. Is this correct?
-
What is the difference between heap and stack.
-
What are the basic interfaces of the Java collection class framework?
-
What is the difference between HashSet and TreeSet?
-
What is the underlying implementation of HashSet?
-
How is LinkedHashMap implemented?
-
Why do collection classes not implement Cloneable and Serializable interfaces?
-
What is an iterator?
-
What is the difference between Iterator and ListIterator?
-
What is the difference between array (Array) and list (ArrayList)? When should you use Array instead of ArrayList?
-
What are the best practices for Java collection class framework?
-
The elements in Set cannot be repeated, so how to distinguish whether they are repeated or not? Should I use == or equals()? What's the difference?
2. Android interview related
1.Activity
● 说下Activity生命周期
● Activity A 启动另一个Activity B 会调用哪些方法?如果B是透明主题的又或则是个DialogActivity呢
● 说下onSaveInstanceState()方法的作用 ? 何时会被调用?
● Activity的启动流程
● onSaveInstanceState(),onRestoreInstanceState的掉用时机
● activity的启动模式和使用场景
● Activity A跳转Activity B,再按返回键,生命周期执行的顺序
● 横竖屏切换,按home键,按返回键,锁屏与解锁屏幕,跳转透明Activity界面,启动一个 Theme 为 Dialog 的 Activity,弹出Dialog时Activity的生命周期
● onStart 和 onResume、onPause 和 onStop 的区别
● Activity之间传递数据的方式Intent是否有大小限制,如果传递的数据量偏大,有哪些方案
● Activity的onNewIntent()方法什么时候会执行
● 显示启动和隐式启动
● scheme使用场景,协议格式,如何使用
● ANR 的四种场景
● onCreate和onRestoreInstance方法中恢复数据时的区别
● activty间传递数据的方式
● 跨App启动Activity的方式,注意事项
● Activity任务栈是什么
● 有哪些Activity常用的标记位Flags
● Activity的数据是怎么保存的,进程被Kill后,保存的数据怎么恢复的
2.ContentProvider
● 什么是ContentProvider及其使用
● ContentProvider的权限管理
● ContentProvider,ContentResolver,ContentObserver之间的关系
● ContentProvider的实现原理
● ContentProvider的优点
● Uri 是什么
3.Handler
● Handler的实现原理
● 子线程中能不能直接new一个Handler,为什么主线程可以主线程的Looper第一次调用loop方法,什么时候,哪个类
● Handler导致的内存泄露原因及其解决方案
● 一个线程可以有几个Handler,几个Looper,几个MessageQueue对象
● Message对象创建的方式有哪些 & 区别?
● Message.obtain()怎么维护消息池的Handler 有哪些发送消息的方法
● Handler的post与sendMessage的区别和应用场景
● handler postDealy后消息队列有什么变化,假设先 postDelay 10s, 再postDelay 1s, 怎么处理这2条消息
● MessageQueue是什么数据结构
● Handler怎么做到的一个线程对应一个Looper,如何保证只有一个MessageQueue ThreadLocal在Handler机制中的作用
● HandlerThread是什么 & 好处 &原理 & 使用场景
● IdleHandler及其使用场景
● 消息屏障,同步屏障机制
● 子线程能不能更新UI
● 为什么Android系统不建议子线程访问UI
● Android中为什么主线程不会因为Looper.loop()里的死循环卡死
● MessageQueue#next 在没有消息的时候会阻塞,如何恢复?
● Handler消息机制中,一个looper是如何区分多个Handler的
● 当Activity有多个Handler的时候,怎么样区分当前消息由哪个Handler处理
● 处理message的时候怎么知道是去哪个callback处理的
● Looper.quit/quitSafely的区别
● 通过Handler如何实现线程的切换
● Handler 如何与 Looper 关联的
● Looper 如何与 Thread 关联的
● Looper.loop()源码
● MessageQueue的enqueueMessage()方法如何进行线程同步的
● MessageQueue的next()方法内部原理
● 子线程中是否可以用MainLooper去创建Handler,Looper和Handler是否一定处于一个线程
● ANR和Handler的联系
4.View drawing
● View绘制流程
● MeasureSpec是什么
● 子View创建MeasureSpec创建规则是什么
● 自定义Viewwrap_content不起作用的原因
● 在Activity中获取某个View的宽高有几种方法
● 为什么onCreate获取不到View的宽高
● View#post与Handler#post的区别
● Android绘制和屏幕刷新机制原理
● Choreography原理
● 什么是双缓冲
● 为什么使用SurfaceView
● 什么是SurfaceView
● View和SurfaceView的区别
● SurfaceView为什么可以直接子线程绘制
● SurfaceView、TextureView、SurfaceTexture、GLSurfaceView
● getWidth()方法和getMeasureWidth()区别
● invalidate() 和 postInvalidate() 的区别
● Requestlayout,onlayout,onDraw,DrawChild区别与联系
● LinearLayout、FrameLayout 和 RelativeLayout 哪个效率高
● LinearLayout的绘制流程
● 自定义 View 的流程和注意事项
● 自定义View如何考虑机型适配
● 自定义控件优化方案
● invalidate怎么局部刷新
● View加载流程(setContentView)
5.View event distribution
● View事件分发机制
● view的onTouchEvent,OnClickListerner和OnTouchListener的onTouch方法 三者优先级
● onTouch 和onTouchEvent 的区别
● ACTION_CANCEL什么时候触发
● 事件是先到DecorView还是先到Window
● 点击事件被拦截,但是想传到下面的View,如何操作
● 如何解决View的事件冲突
● 在 ViewGroup 中的 onTouchEvent 中消费 ACTION_DOWN 事件,ACTION_UP事件是怎么传递
● Activity ViewGroup和View都不消费ACTION_DOWN,那么ACTION_UP事件是怎么传递的
● 同时对父 View 和子 View 设置点击方法,优先响应哪个
● requestDisallowInterceptTouchEvent的调用时机
Due to the large content and limited space, the information has been organized into PDF documents. If you need the complete document with answers to the most comprehensive Android intermediate and advanced interview questions in 2023, you can scan the QR code below to get it for free! !
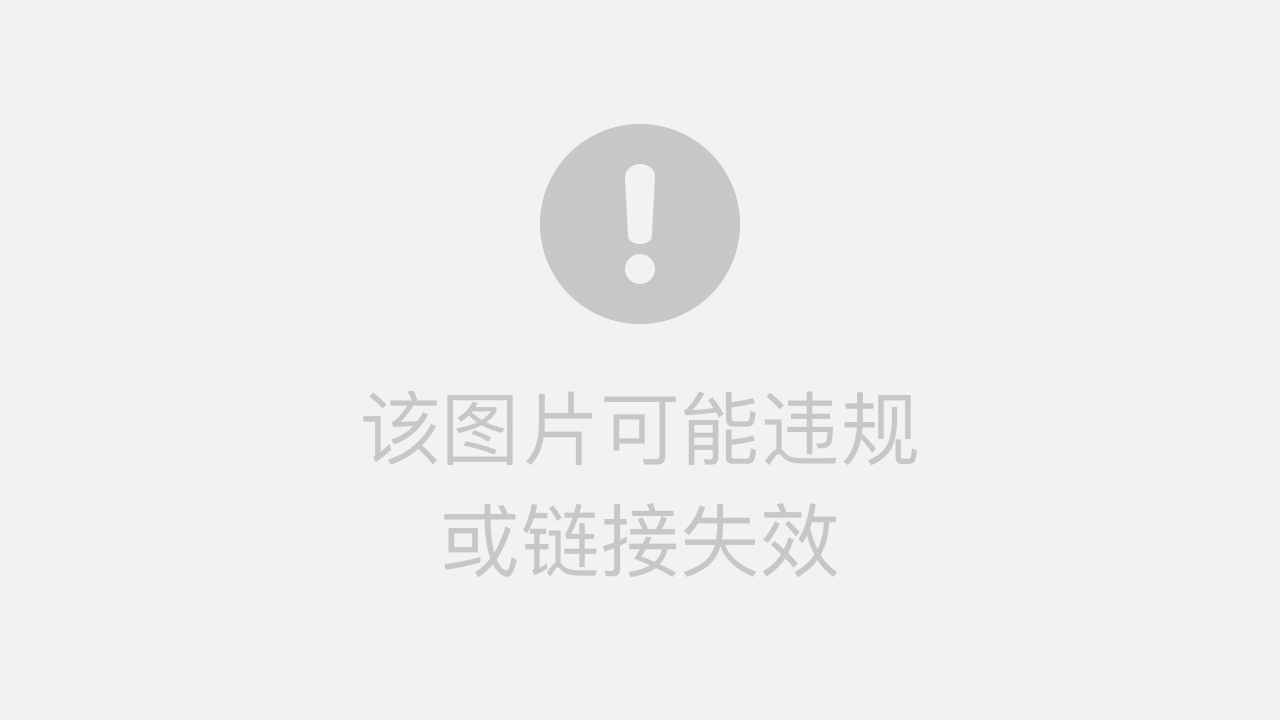
Table of contents
Chapter 1 Java
●Java basics
●Java collection
●Java multithreading
●Java virtual machine
Chapter 2 Android
●Related to the four major components of Android
●Android asynchronous tasks and message mechanism
●Android UI drawing related
●Android performance tuning related
●IPC in Android
●Android system SDK related
●Third-party framework analysis
●Comprehensive technology
●Data structure aspect
●Design pattern
●Computer network aspects
●Kotlin aspect
Chapter 3 Audio and video development high-frequency interview questions
●Why can a huge original video be encoded into a small video? What is the technology involved?
●How to optimize live streaming instantly?
●What is the most important role of histogram in image processing?
●What are the methods of digital image filtering?
●What features can be extracted from images?
●What are the criteria for measuring the quality of image reconstruction? How to calculate?
Chapter 4 Flutter high-frequency interview questions
●Dart part
●Flutter part
Chapter 5 Algorithm High Frequency Interview Questions
●How to find prime numbers efficiently
●How to use binary search algorithm
●How to effectively solve rainwater problems
●How to remove duplicate elements from an ordered array
●How to perform modular exponentiation operation efficiently
●How to find the longest palindromic substring
Chapter 6 Andrio Framework
●Analysis of system startup process interview questions
●Binder interview question analysis
●Handler interview question analysis
●Analysis of AMS interview questions
Chapter 7 174 common interview questions in companies
●SD card
●Android data storage method
●Broadcast Receiver
●What are the consequences of frequent sp operations? How much data can sp store?
●The difference between dvm and jvm
●ART
●Activity life cycle
●Can Application start Activity?
●…