EditText listens for carriage return
When using EditText, sometimes we need to monitor the input carriage return to perform some operations. Or you need to change the carriage return to "Search", "Send" or "Complete" etc.
EditText provides us with an attribute imeOptions to replace the appearance of the enter key in the soft keyboard. For example, actionGo will change the appearance to "Go". Need to be set at the same time android:inputType="text"
.
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:imeOptions="actionGo"
android:inputType="text" />
Several commonly used attributes and replaced text appearance:
Attributes | illustrate | Corresponds to static variables |
---|---|---|
actionUnspecified | unspecified | EditorInfo.IME_ACTION_UNSPECIFIED |
actionNone | action | EditorInfo.IME_ACTION_NONE |
actionGo | go to | EditorInfo.IME_ACTION_GO |
actionSearch | search | EditorInfo.IME_ACTION_SEARCH |
actionSend | send | EditorInfo.IME_ACTION_SEND |
actionNext | Next item | EditorInfo.IME_ACTION_NEXT |
actionDone | Finish | EditorInfo.IME_ACTION_DONE |
The setting method can be set in the layout file android:imeOptions="actionNext"
or in the codemUserEdit.setImeOptions(EditorInfo.IME_ACTION_NEXT);
Next, set the listening event for the Enter keysetOnEditorActionListener
et1.setOnEditorActionListener(mOnEditorActionListener);
// ......
private TextView.OnEditorActionListener mOnEditorActionListener = new TextView.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
Log.d(TAG, "actionId: " + actionId);
Log.d(TAG, "event: " + event);
return false;
}
};
If onEditorAction returns true, it means that this event is consumed.
The actionId above corresponds to android.view.inputmethod.EditorInfo
the constant in .
public static final int IME_ACTION_UNSPECIFIED = 0x00000000;
public static final int IME_ACTION_NONE = 0x00000001;
public static final int IME_ACTION_GO = 0x00000002;
public static final int IME_ACTION_SEARCH = 0x00000003;
public static final int IME_ACTION_SEND = 0x00000004;
public static final int IME_ACTION_NEXT = 0x00000005;
public static final int IME_ACTION_DONE = 0x00000006;
public static final int IME_ACTION_PREVIOUS = 0x00000007;
EditText cursor movement and selection
Mainly introduces the setSelection method.
setSelection has:
- setSelection(int start, int stop) selection range
- setSelection(int index) moves the cursor to the specified position
Example: Assume there is EditText and the variable name is mEt1 .
- Move the cursor to the front mEt1.setSelection(0);mEt1.setSelection(mEt1.getText().length());mEt1.setSelection(mEt1.getSelectionEnd() + 1);mEt1.setSelection(mEt1.getSelectionEnd() - 1); It should be noted that if the incoming index exceeds the range of text, a report will be reported.
java.lang.IndexOutOfBoundsException
Therefore, in actual projects, it is necessary to determine whether the incoming position is within the length range of the existing content of EditText. - Move the cursor to the end
- Move the cursor one position to the right
- Move the cursor one position to the left
- Select all currently input textmEt1.setSelection(0, mEt1.getText().length());
Monitor input content
Dynamically limit input length in code
Using TextWatcher
mQueryEt.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
}
@Override
public void afterTextChanged(Editable s) {
// 如果EditText中的数据不为空,且长度大于指定的最大长度
if (!TextUtils.isEmpty(s) && s.length() > 15) {
// 删除指定长度之后的数据
s.delete(15, s.length() - 1);
}
}
});
Share one last time
[Produced by Tencent Technical Team] Getting started with Android from scratch to mastering it, Android Studio installation tutorial + full set of Android basic tutorials
Android programming introductory tutorial
Java language basics from entry to familiarity
Kotlin language basics from entry to familiarity
Android technology stack from entry to familiarity
Comprehensive learning on Android Jetpack
For novices, it may be difficult to install Android Studio. You can watch the following video to learn how to install and run it step by step.
Android Studio installation tutorial
With the Java stage of learning, it is recommended to focus on video learning at this stage and supplement it with book checking and filling in gaps. If you mainly focus on books, you can type the code based on the book's explanations, supplemented by teaching videos to check for omissions and fill in the gaps. If you encounter problems, you can go to Baidu. Generally, many people will encounter entry-level problems and give better answers.
You need to master basic knowledge points, such as how to use the four major components, how to create Service, how to layout, simple custom View, animation, network communication and other common technologies.
A complete set of zero-based tutorials has been prepared for you. If you need it, you can add the QR code below to get it for free.
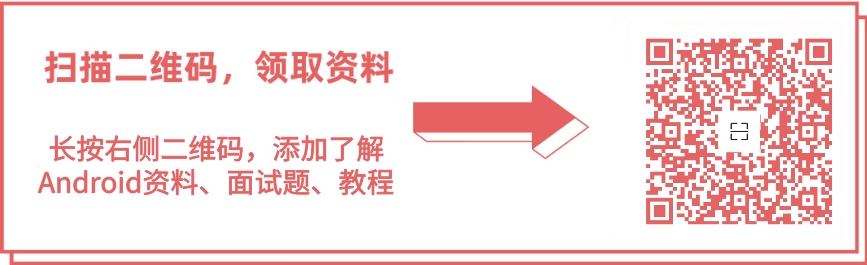
A complete set of basic Android tutorials