30 HTML+CSS front-end development cases (11-15)
Xiaomi launches floating menu on the right
Implement code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>小米商城右侧悬浮菜单</title>
<link rel="stylesheet" href="iconfont/iconfont.css">
<style type="text/css">
body {
background-color: #ddd;
height: 2000px;
margin: 0;
padding: 0;
}
ul,
p {
margin: 0;
padding: 0;
list-style: none;
}
a {
text-decoration: none;
}
.sidebar {
width: 85px;
position: fixed;
right: 20px;
bottom: 100px;
}
.sidebar ul {
}
.sidebar ul li {
border-bottom: 1px solid #ddd;
width: 85px;
height: 85px;
background-color: white;
position: relative;
}
.sidebar ul li:last-child {
margin-top: 20px;
}
.sidebar ul li span {
width: 85px;
height: 50px;
display: block;
font-size: 28px;
color: #666;
text-align: center;
line-height: 50px;
}
.sidebar ul li p {
font-size: 14px;
text-align: center;
color: #666;
}
.sidebar ul li:hover span,
.sidebar ul li:hover p {
color: hotpink;
}
.sidebar ul li .wxin {
width: 100px;
height: 100px;
background: #fff;
position: absolute;
top: 0;
left: -130px;
padding: 15px;
display: none;
}
.sidebar ul li:hover .wxin {
display: block;
}
</style>
</head>
<body>
<div class="sidebar">
<ul>
<li>
<a href="">
<span class="iconfont icon-shouji"></span>
<p>手机APP</p>
</a>
<div class="wxin">
<img src="images/wx.png" alt="" width="100">
</div>
</li>
<li>
<a href="">
<span class="iconfont icon-gerenzhongxin"></span>
<p>个人中心</p>
</a>
</li>
<li>
<a href="">
<span class="iconfont icon-shouhouwuyou"></span>
<p>售后服务</p>
</a>
</li>
<li>
<a href="">
<span class="iconfont icon-kefu"></span>
<p>人工客服</p>
</a>
</li>
<li>
<a href="">
<span class="iconfont icon-gouwuchekong"></span>
<p>购物车</p>
</a>
</li>
<li>
<span class="iconfont icon-fanhuidingbu"></span>
<p>回顶部</p>
</li>
</ul>
</div>
</body>
</html>
renderings
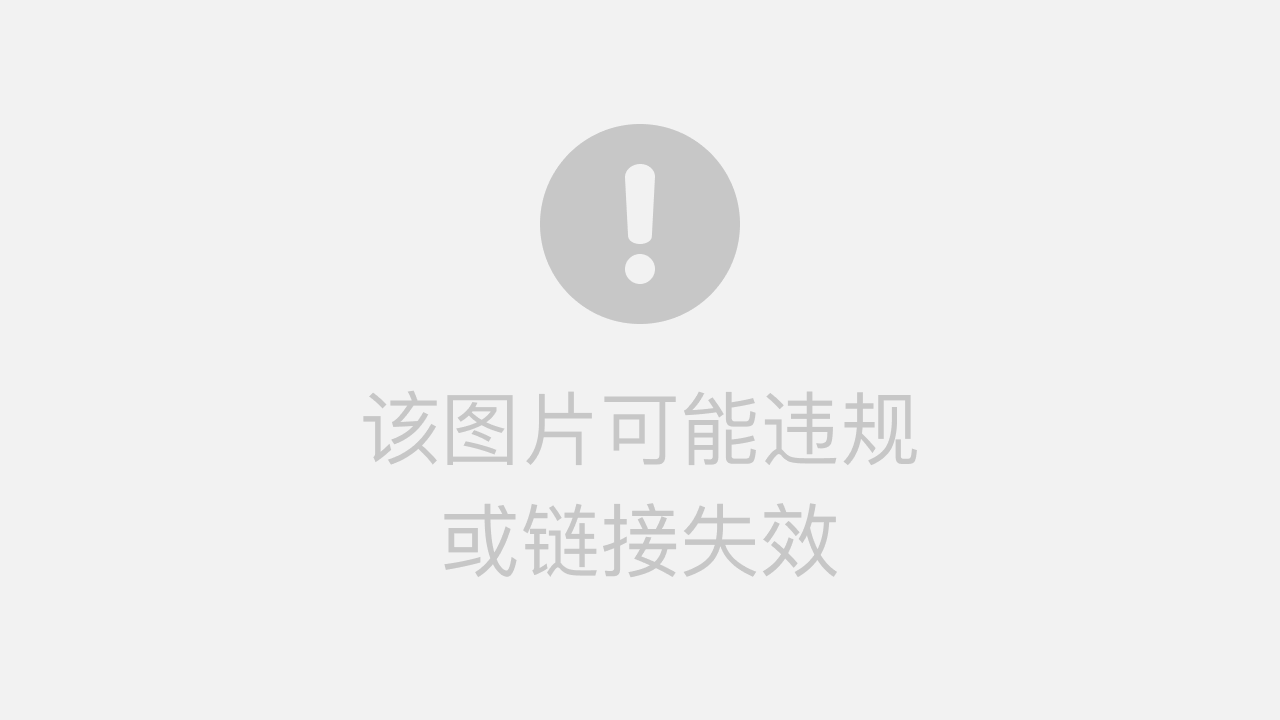
Automatic carousel effect
Implement code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>自动轮播图效果</title>
<style type="text/css">
body ul {
margin: 0;
padding: 0;
}
ul {
list-style: none;
}
.banner {
width: 1000px;
height: 466px;
margin: 50px auto 0;
background-color: aqua;
position: relative;
overflow: hidden;
}
.banner ul {
width: 10000px;
position: absolute;
left: 0;
top: 0;
}
.banner ul li {
width: 1000px;
height: 466px;
float: left;
}
.banner .prev,
.banner .next {
width: 41px;
height: 69px;
position: absolute;
top: 50%;
margin-top: -35px;
background: url('images/icon-slides.png');
}
.banner .prev {
left: 0;
background-position:-83px 0;
}
.banner .next {
right: 0;
background-position:-125px 0;
}
.banner .prev:hover {
background-position: 0 0;
}
.banner .next:hover {
background-position: -42px 0;
}
.banner .button{
width: 100%;
height: 50px;
background-color: rgba(0, 0, 0, 0.5);
position: absolute;
bottom: 0;
left: 0;
font-size: 0;
text-align: center;
line-height: 50px;
}
.banner .button span{
width: 10px;
height: 10px;
background-color: white;
display: inline-block;
border-radius: 50%;
margin: 0 3px;
vertical-align: middle;
}
.button span.current{
background-color: hotpink;
}
</style>
</head>
<body>
<div class="banner">
<ul>
<li><img src="images/slide-1.png" alt=""></li>
<li><img src="images/slide-2.png" alt=""></li>
<li><img src="images/slide-3.png" alt=""></li>
<li><img src="images/slide-4.png" alt=""></li>
<li><img src="images/slide-5.png" alt=""></li>
</ul>
<div class="prev"></div>
<div class="next"></div>
<div class="button">
<span class="current"></span>
<span></span>
<span></span>
<span></span>
<span></span>
</div>
</div>
</body>
</html>
renderings
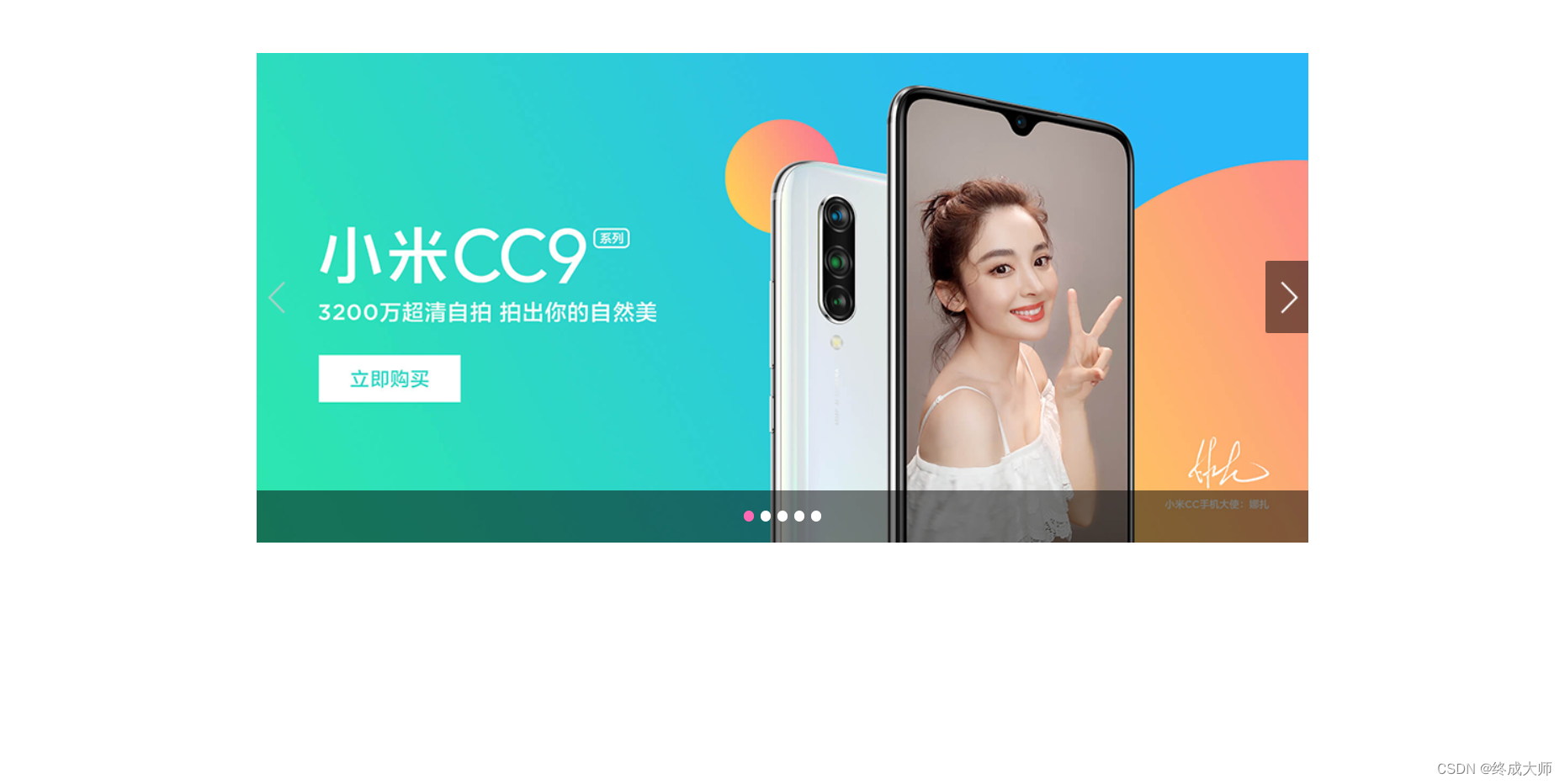
Xiaomi Mall secondary drop-down menu effect
Implement code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>二级下拉菜单效果</title>
<style type="text/css">
body,
ul {
margin: 0;
padding: 0;
}
ul {
list-style: none;
}
a {
text-decoration: none;
}
.clearfix::after {
content: '';
display: block;
clear: both;
}
.menu {
width: 100%;
height: 60px;
background-color: #fd6a88;
}
.menu .menu-con {
width: 1000px;
height: 60px;
margin: 0 auto;
}
.menu .menu-con ul {
}
.menu .menu-con ul li {
height: 60px;
float: left;
position: relative;
}
.menu .menu-con ul li a {
display: block;
height: 60px;
color: white;
padding: 0 40px;
line-height: 60px;
text-align: center;
}
.menu .menu-con ul li:hover {
background-color: #ee4d75;
}
.menu .menu-con ul li div {
width: 200px;
background-color: #fd6a88;
position: absolute;
left: 50%;
margin-left: -100px;
top: 60px;
display: none;
}
.menu .menu-con ul li div a {
height: 40px;
line-height: 40px;
padding: 0;
font-size: 14px;
}
.menu .menu-con ul li div a:hover {
background-color: #ee4d75;
}
.menu .menu-con ul li:hover div{
display: block;
}
</style>
</head>
<body>
<div class="menu">
<div class="menu-con">
<ul class="clearfix">
<li>
<a href="#">小米手机</a>
<div>
<a href="">Xiaomi Pro 12</a>
<a href="">Xiaomi 12</a>
<a href="">Xiaomi 青春活力版</a>
<a href="">Xiaomi 12X</a>
<a href="">Xiaomi civi</a>
</div>
</li>
<li>
<a href="#">Redmi小米</a>
<div><a href="">Xiaomi Pro 12</a>
<a href="">Xiaomi 12</a>
<a href="">Xiaomi 青春活力版</a>
<a href="">Xiaomi 12X</a>
<a href="">Xiaomi civi</a>
</div>
</li>
<li>
<a href="#">电视</a>
<div><a href="">Xiaomi Pro 12</a>
<a href="">Xiaomi 12</a>
<a href="">Xiaomi 青春活力版</a>
<a href="">Xiaomi 12X</a>
<a href="">Xiaomi civi</a>
</div>
</li>
<li>
<a href="#">笔记本</a>
<div><a href="">Xiaomi Pro 12</a>
<a href="">Xiaomi 12</a>
<a href="">Xiaomi 青春活力版</a>
<a href="">Xiaomi 12X</a>
<a href="">Xiaomi civi</a>
</div>
</li>
<li>
<a href="#">平板</a>
<div><a href="">Xiaomi Pro 12</a>
<a href="">Xiaomi 12</a>
<a href="">Xiaomi 青春活力版</a>
<a href="">Xiaomi 12X</a>
<a href="">Xiaomi civi</a>
</div>
</li>
<li>
<a href="#">路由器</a>
<div><a href="">Xiaomi Pro 12</a>
<a href="">Xiaomi 12</a>
<a href="">Xiaomi 青春活力版</a>
<a href="">Xiaomi 12X</a>
<a href="">Xiaomi civi</a>
</div>
</li>
</ul>
</div>
</div>
</body>
</html>
renderings
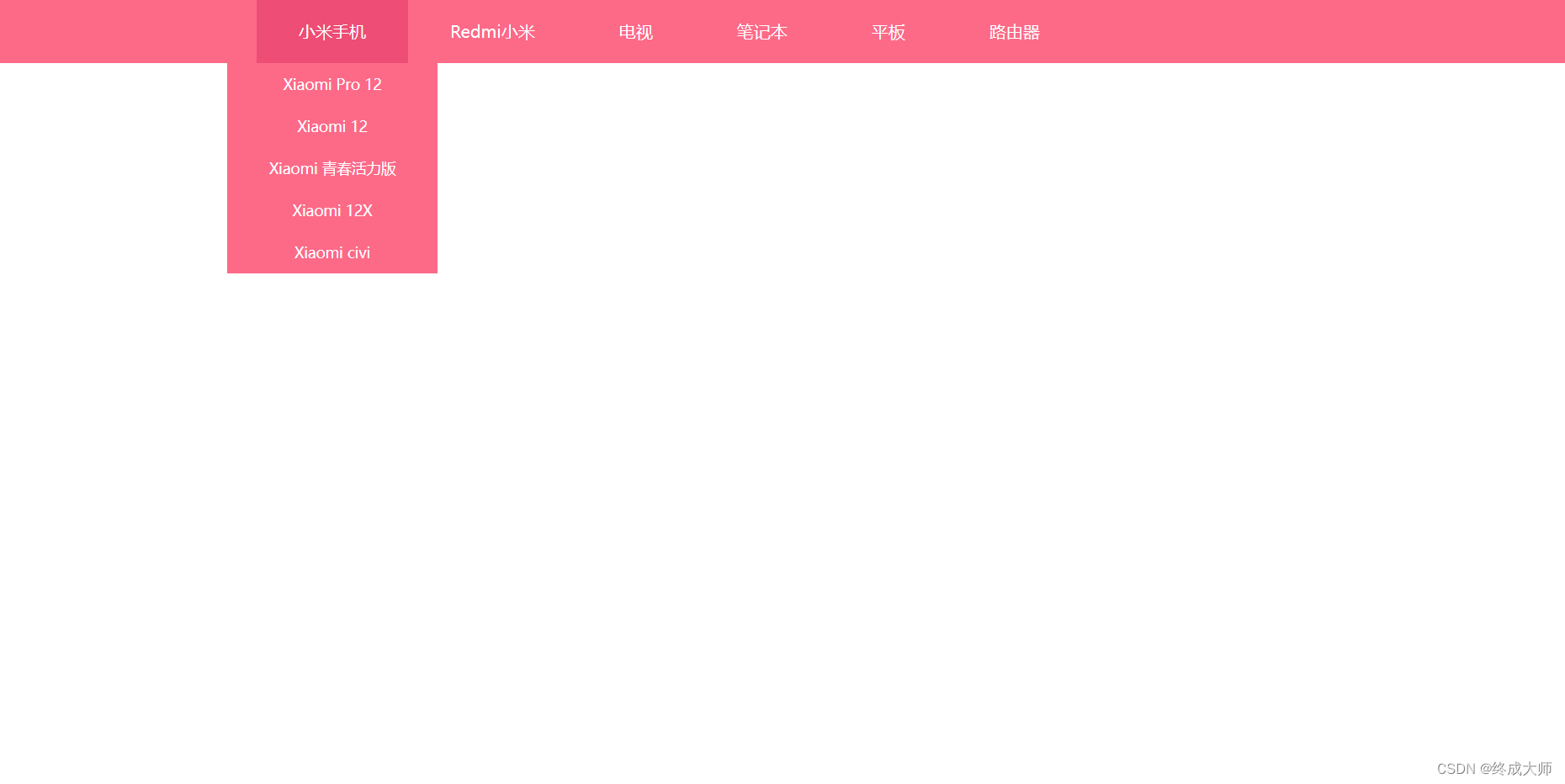
timeline effect
Implement code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>时间轴效果展示</title>
<style type="text/css">
body{
margin: 0;
padding: 0;
background-image: linear-gradient(to right,#fdf1d8,#b2bcf9);
}
body,ul,h3{
margin: 0;
padding: 0;
}
ul{
list-style: none;
}
a{
text-decoration: none;
}
.clearfix::after{
content: '';
display: block;
clear: both;
}
.time-axis{
width: 804px;
margin: 50px auto 0;
}
.time-axis .left{
width: 400px;
float: left;
border-right: 4px solid #b1bbf9;
position: relative;
}
.time-axis .right{
width: 400px;
float: right;
border-left: 4px solid #b1bbf9;
position: relative;
}
.time-axis .dot{
width: 10px;
height: 10px;
background-color: #fff;
display: block;
border-radius: 100%;
border: 2px solid #b1bbf9;
position: absolute;
top: 50%;
margin-top: -7px;
}
.time-axis .left .dot{
right: -9px;
}
.time-axis .right .dot{
left: -9px;
}
.time-axis .jiantou{
width: 32px;
height: 32px;
display: block;
position: absolute;
top: 50%;
margin-top: -18px;
}
.time-axis .left .jiantou{
background: url('images/r-jiantou.png');
right: 0;
}
.time-axis .right .jiantou{
background: url('images/l-jiantou.png');
left: 0;
}
.time-axis .con{
background-color: #fff;
padding: 15px;
border-radius: 10px;
text-align: center;
}
.time-axis .left .con{
margin-right: 22px;
}
.time-axis .right .con{
margin-left: 22px;
}
.time-axis .con h3{
font-weight: 400;
}
.time-axis .con h3 span{
font-size: 38px;
font-family: Arial;
color: #b1bbf9;
font-weight: 800;
}
</style>
</head>
<body>
<div class="time-axis clearfix">
<div class="left">
<span class="dot"></span>
<span class="jiantou"></span>
<div class="con">
<h3><span>“</span> 春季腹泻要当心,益生菌要这样... <span>”</span></h3>
<img src="images/axis01.jpg" alt="" width="300">
</div>
</div>
<div class="right">
<span class="dot"></span>
<span class="jiantou"></span>
<div class="con">
<h3><span>“</span> 春季腹泻要当心,益生菌要这样... <span>”</span></h3>
</div>
</div>
<div class="left">
<span class="dot"></span>
<span class="jiantou"></span>
<div class="con">
<h3><span>“</span> 春季腹泻要当心,益生菌要这样... <span>”</span></h3>
</div>
</div>
<div class="right">
<span class="dot"></span>
<span class="jiantou"></span>
<div class="con">
<h3><span>“</span> 春季腹泻要当心,益生菌要这样... <span>”</span></h3>
<img src="images/axis02.jpg" alt="" width="300">
</div>
</div>
</div>
</body>
</html>
renderings
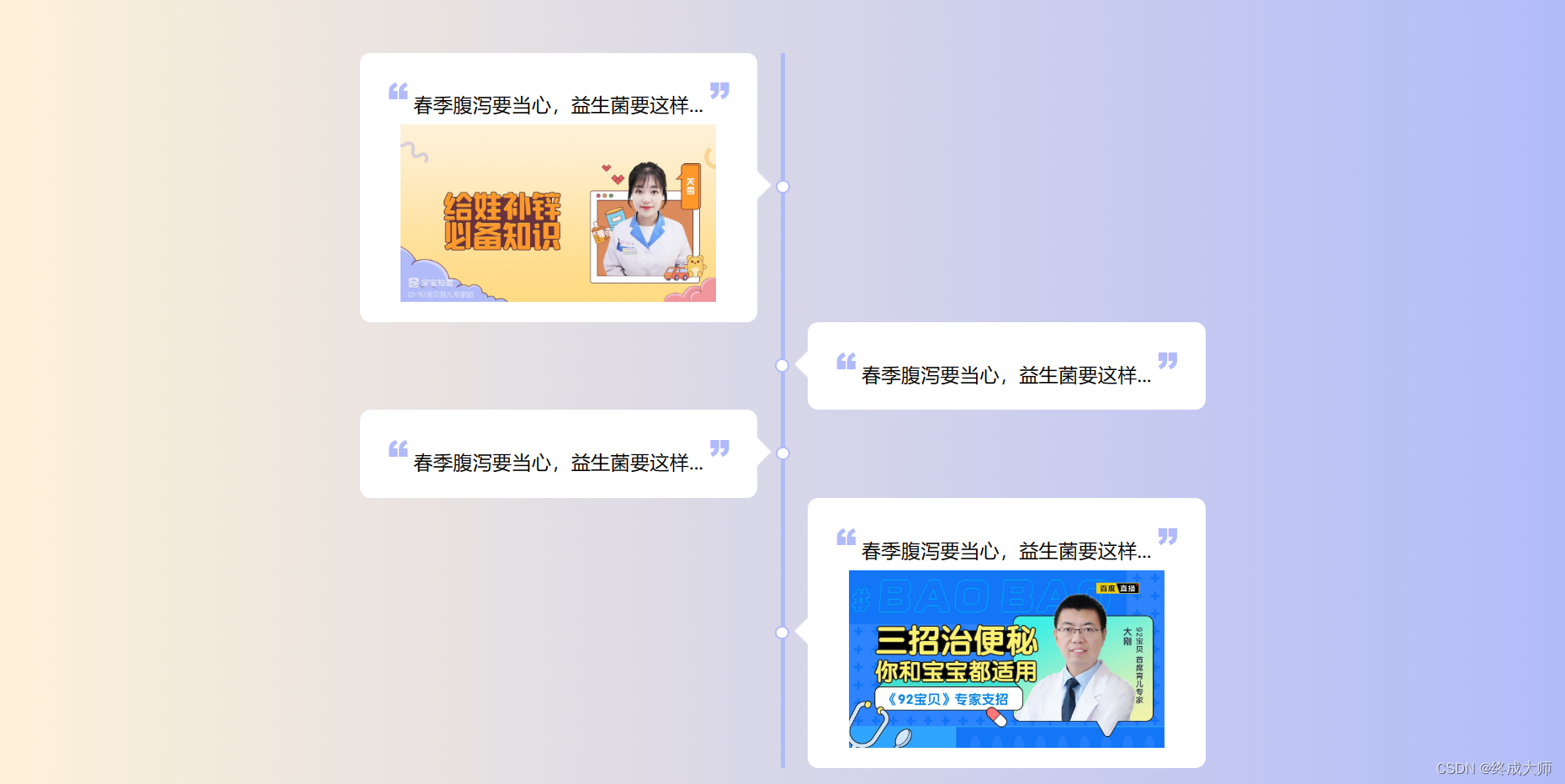
QQ music ranking effect
Implement code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>音乐排行榜效果</title>
<style type="text/css">
body {
margin: 0;
padding: 0;
}
a{
text-decoration: none;
}
.music-top {
width: 800px;
margin: 50px auto 0;
}
table {
width: 100%;
border-spacing: 0;
border-collapse: collapse;
}
table tr th {
height: 80px;
}
table tr td {
height: 80px;
}
.col1 {
width: 60px;
}
.col2 {
width: 80px;
}
.col3 {
wid
}
.col4 {
width: 120px;
}
.col5 {
width: 60px;
}
table tr:nth-of-type(odd) {
background-color: white;
}
table tr:nth-of-type(even) {
background-color: #f7f7f7;
}
table tr th {
background-color: #31c272;
color: #fff;
font-size: 16px;
font-weight: 400;
text-align: left;
}
table tr td:nth-child(1) {
font-size: 24px;
color: #333;
text-align: center;
}
table tr td:nth-child(1) span {
color: #ff4222;
}
table tr td:nth-child(2) {
font-size: 12px;
color: #999;
}
table tr td:nth-child(2) img {
display: block;
margin-left: 10px;
}
table tr td:nth-child(4),
table tr td:nth-child(5) {
font-size: 14px;
color: #999;
}
.con {
height: 70px;
position: relative;
}
.con .txt {
height: 70px;
position: absolute;
left: 90px;
top: 0;
right: 0;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
line-height: 70px;
}
.con .txt a{
color: #333;
}
.con .txt span {
color: #999;
}
.con .button {
width: 140px;
height: 36px;
position: absolute;
right: 0;
top: 17px;
display: none;
}
.con .button i {
width: 36px;
height: 36px;
display: inline-block;
background-image: url('images/icon-music.png');
}
.con .button i.play {
}
.con .button i.add {
background-position: 0 -80px;
}
.con .button i.word {
background-position: 0 -40px;
}
.con .button i.play:hover {
background-position: -40px 0;
}
.con .button i.add:hover {
background-position: -40px -80px;
}
.con .button i.word:hover {
background-position: -40px -40px;
}
table tr:hover .con .button {
display: block;
}
table tr:hover .con .txt {
right: 180px;
}
</style>
</head>
<body>
<div class="music-top">
<table>
<colgroup>
<col span="1" class="col1">
<col span="1" class="col2">
<col span="1" class="col3">
<col span="1" class="col4">
<col span="1" class="col5">
</colgroup>
<tr>
<th></th>
<th>排行</th>
<th>歌曲</th>
<th>歌手</th>
<th>时长</th>
</tr>
<tr>
<td><span>1</span></td>
<td>
<img src="images/up-jiantou.png" alt="">
158%
</td>
<td>
<div class="con">
<img src="images/music-img1.webp" alt="" height="70">
<div class="txt">爱丫爱丫<span>《爱情是从告白开始的》电视剧插曲</span></div>
<div class="button">
<i class="play"></i>
<i class="add"></i>
<i class="word"></i>
</div>
</div>
</td>
<td>BY2</td>
<td>03:51</td>
</tr>
<tr>
<td><span>2</span></td>
<td>
<img src="images/up-jiantou.png" alt="">
128%
</td>
<td>
<div class="con">
<img src="images/music-img2.webp" alt="" height="70">
<div class="txt">春泥(女版)<span></span></div>
<div class="button">
<i class="play"></i>
<i class="add"></i>
<i class="word"></i>
</div>
</div>
</td>
<td>旺仔小乔</td>
<td>03:03</td>
</tr>
<tr>
<td><span>3</span></td>
<td>
<img src="images/up-jiantou.png" alt="">
118%
</td>
<td>
<div class="con">
<img src="images/music-img3.webp" alt="" height="70">
<div class="txt">孤勇者(Live)<span>《英雄联盟:双城》</span></div>
<div class="button">
<i class="play"></i>
<i class="add"></i>
<i class="word"></i>
</div>
</div>
</td>
<td>永彬Ryan.B</td>
<td>03:14</td>
</tr>
<tr>
<td><span>4</span></td>
<td>
<img src="images/up-jiantou.png" alt="">
108%
</td>
<td>
<div class="con">
<a href=""><img src="images/music-img4.webp" alt="" height="70"></a>
<div class="txt">
<a href="">
爱丫爱丫<span>《爱情是从告白开始的》电视剧插曲</span>
</a>
</div>
<div class="button">
<i class="play"></i>
<i class="add"></i>
<i class="word"></i>
</div>
</div>
</td>
<td>张韶涵/信</td>
<td>04:30</td>
</tr>
</table>
</div>
</body>
</html>
renderings
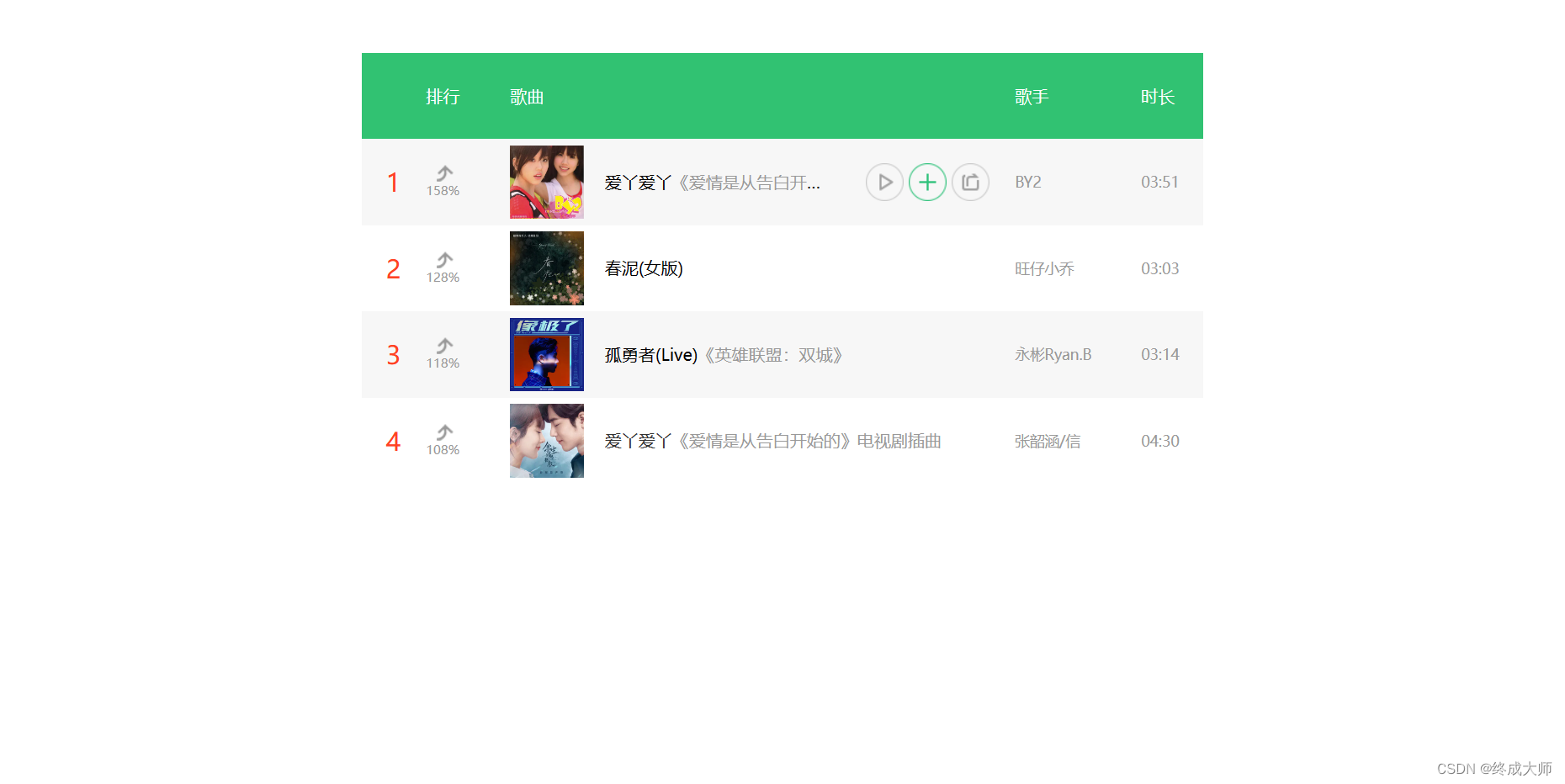
Resource package acquisition
All pictures and code, continuously updated...