Requirement: A certain table in the backend management system needs to be imported and exported
This article is based on vue and antdesign
Table import:
First, you need to download the import template. If the imported table does not correspond to the table in the project, the backend will not recognize it after passing it to the backend.
Downloading the import template is to send a request directly to the backend, and convert the data into a blob stream when requesting (a key step)
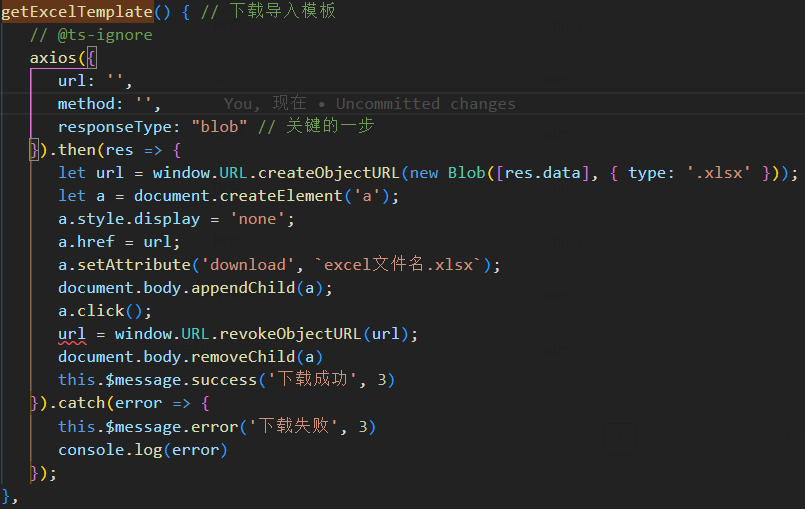
Part of the code above:
let url = window.URL.createObjectURL(new Blob([res.data], { type: '.xlsx' }));
let a = document.createElement('a');
a.style.display = 'none';
a.href = url;
a.setAttribute('download', ``);
document.body.appendChild(a);
a.click();
url = window.URL.revokeObjectURL(url);
document.body.removeChild(a)
this.$message.success('下载成功', 3)
1.2. After downloading the import template, import this file into the project form
There is an a-upload upload component in the antd component library. The important point is action. The value of this props should be action="requested base path (axios.defaults.baseURL + import interface)". If it is dynamic, it needs Add a colon before action.
html:
<a-upload name="file" :action="actionURL+''" @change="handleChange":showUploadList="false" method="post" :data="file.file" :headers="headers">
<a>导入</a>
</a-upload>
js:
handleChange(info) { // 导入
this.file['file'] = info.file.name
if (info.file.status !== 'uploading') {
// console.log(info.file, info.fileList);
}
if (info.file.status === 'done') {
this.$message.success('导入成功', 3)
} else if (info.file.status === 'error') {
this.$message.error('文件导入失败', 3);
this.isImportExcel = false
}
},
1.3. You can also import files without using components, just call the interface as usual (optional in 1.2 and 1.3)
importExcel(info) {
let input = document.createElement("input");
input.type = "file";
input.onchange = this.handleUpload;
input.click();
},
handleUpload(event) {
let file = event.target.files[0];
POST_IMPORT_FILE(file).then((res) => {
console.log(res)
if (res.data.code === 0) {
this.$message.success('导入成功', 3);
this.pagination.current = 1
this.getList();
} else {
this.$message.error('导入失败', 3);
}
});
},
// axios请求封装
export const POST_IMPORT_FILE = (data) => {
let formData=new FormData()
formData.append('file',data);
formData.append('type',0);
return axios.post(`接口`, formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
});
}
2. Table export
html:
<a-button type="primary" style="margin-left:20px"
loading>导出</a-button>
js:
async exportExcel() { // 导出
axios({
method: '',
url: '/',
params: {},
responseType: "blob"
}).then((res) => {
let url = window.URL.createObjectURL(new Blob([res.data], { type: '.xlsx' }));
let a = document.createElement('a');
a.style.display = 'none';
a.href = url;
a.setAttribute('download', `维护.xlsx`);
document.body.appendChild(a);
a.click();
url = window.URL.revokeObjectURL(url);
document.body.removeChild(a)
this.$message.success('导出成功', 3)
}).catch(error => {
this.$message.error('导出失败', 3)
});
}
}
At this point, the import and export is completed. The above code can be pasted and copied, and the requested content can be changed by yourself.
Summarize:
Analyze needs, find solutions, and gradually improve! If there is any discrepancy, please let us know so as not to mislead others. Thank you!