We previously learned the bytes occupied by each type of space size:
So how much space does the structure type we define occupy?
Structure memory alignment
Alignment rules
1. The first member of the structure is placed at offset 0. (It is the first byte space where the structure type is stored)
2. Starting from the second member, each subsequent member must be aligned to an integer multiple of a certain alignment number.
The member alignment number is: the smaller value of the member's own size and the default alignment number (VS's default alignment number is 8) (gcc has no default alignment number)
3. After all members are stored, the total size of the structure must be an integer multiple of the maximum alignment number among all members.
(If it is not an integer multiple, it will waste memory and require space alignment)
4. If the structure is nested, the nested structure is aligned to an integer multiple of its own maximum alignment number, and the overall size of the structure is an integer multiple of all the maximum alignment numbers (including the alignment number of nested structures) . (Similar to unpacking a nested structure)
struct test 1
//The eight bytes required in the end are exactly an integer multiple of the maximum alignment number 4, that is, they are already aligned.
verify
// The address of char a: 0x00007ff7e86fc1e8 and the address of short b: 0x00007ff7e86fc1ea are exactly one byte apart, so it is satisfied. If you are still not sure, pick up your "weapon" and start debugging it!
//In fact, offsetof can also be used here: calculate the offset of a member of a structure compared to its starting position.
struct test 2
//The final requirement is ten bytes, which is not an integer multiple of the maximum alignment number 4, that is: it takes two bytes of space to align a total of twelve bytes
struct test 3
//The maximum alignment number here is 8, and 16 is also a multiple of the larger alignment number 8.
Replenish
struct tes
{
double a;
char b;
int i;
};
struct test
{
char a;
struct tes b;
double d;
};
int main()
{
printf("%zu\n", sizeof(struct test));
return 0;
}
//Guess the result? Rule four
parse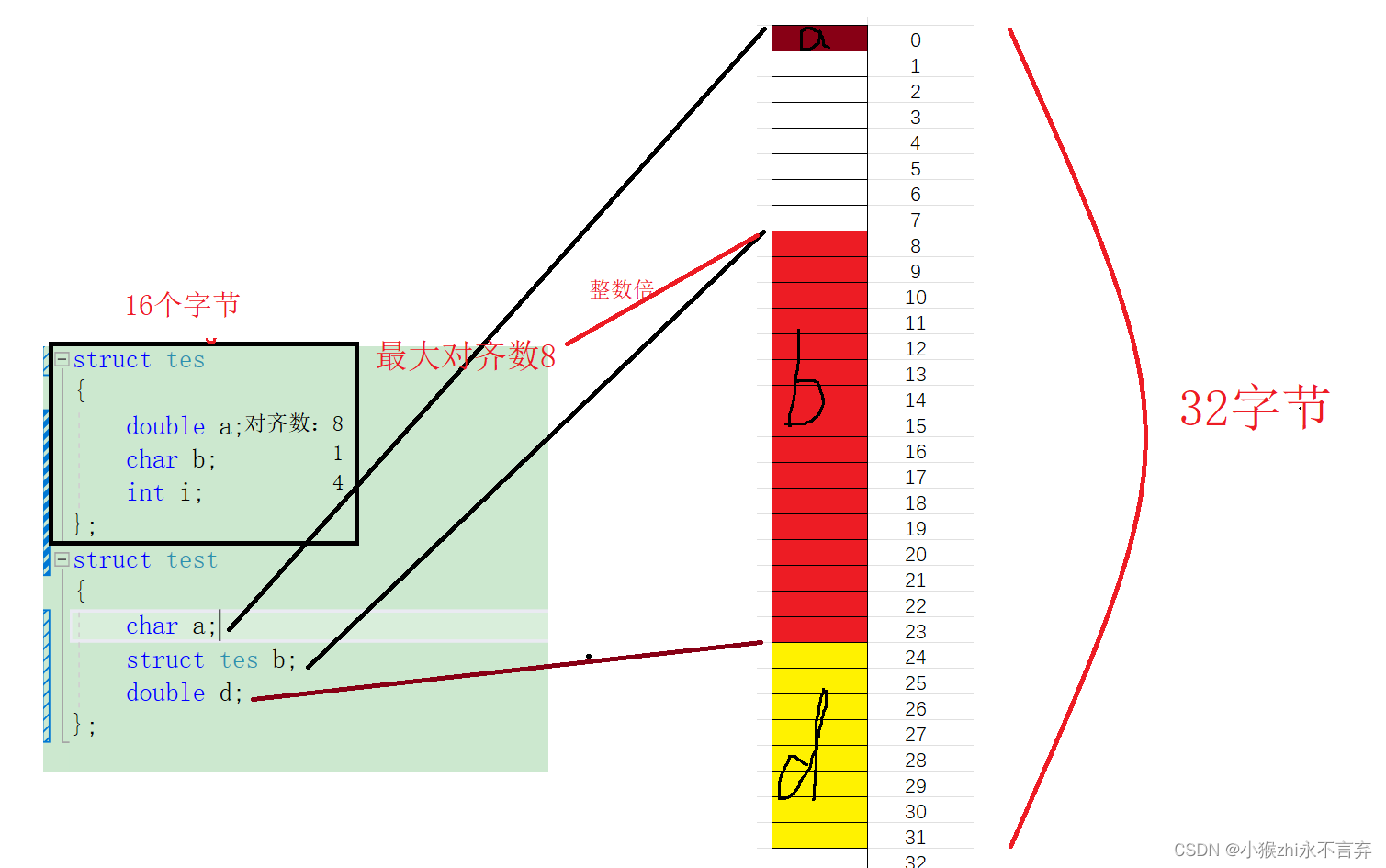
The overall size of the structure is an integer multiple of the maximum alignment of all members (including the alignment of nested structures). (Similar to taking apart a nested structure)
//The maximum alignment number here is 8, and 32 happens to be an integer multiple of 8
Modify the default alignment number
The default alignment number we used in VS calculations above is 8. If I don’t think 8 is inappropriate, you can modify it yourself, according to your ideas.
exhibit
//Just a reminder, although this is easy to use, we'd better not set an alignment number to embarrass ourselves.
Rank
Baidu Encyclopedia: Bit segment, C language allows the memory length occupied by its members to be specified in bit units in a structure . Such members in bit units are called "bit segments" or "bit fields"
Object: Generally an integer
Function: Ability to reduce the number of bits (bits) used to store data to save space
Definition: Information is generally accessed in bytes.
Example usage (VS platform)
typedef struct test
{
int a : 2;//占2个字节
int b : 5;//占5个字节
int c : 10;//占10个字节
int d : 30;//占30个字节
}test;
int main()
{
printf("%zu\n", sizeof(test));
return 0;
}
compile
You may say that with 47 bits, six bytes can be accommodated. Why are there two eight bytes?
Then please take a look!
Bit segment memory allocation
rule
1. The members of the bit field can be int, unsigned int or char type
2. The space of the bit segment is allocated in the form of four bytes (int) or one byte (char) as needed.
3. Bit segments involve many uncertain factors and are not cross-platform (different compilers may use bit segments differently)
So let’s use the VS compiler to see how it is allocated:
Example
typedef struct test
{
char a : 3;
char b : 4;
char c : 5;
char d : 4;
}test;
int main()
{
test k = { 0 };//初始化为全0
k.a = 9;
k.b = 8;
k.c = 7;
k.d = 6;
return 0;
}
parse
Compile
The above are only the results on VS, and may be different on other platforms. And VS opens up eight bits (one char) of space at a time. When the extra space is not enough to store the next data, the extra space is wasted and will not be used (and will not be used in the future).
//Bit segments save a lot of space compared to structures, but bit segments are not cross-platform.
The role of bit fields: It can save storage space in data units . This method is particularly important when a program requires thousands of data units.