For more articles, please follow my personal blog: https://seven777777.github.io/myblog/
Date object is used to handle date and time
1. new Date()
By default, new Date() will return the current date and time in the following format
var date = new Date() //Tue Sep 29 2020 16:38:00 GMT+0800 (中国标准时间)
Create a specific time and pass the parameters as follows:
- new Date(“month dd,yyyy hh:mm:ss”);
- new Date(“month dd,yyyy”);
- new Date(yyyy,mth,dd,hh,mm,ss);
- new Date(yyyy,mth,dd)
- new Date(ms)
month: Indicates the name of the month in English, from January to December
mth: represents the month as an integer, from 0 (January) to 11 (December)
dd: indicates the day of the month, from 1 to 31
yyyy: four-digit year
hh: hour, from 0 (midnight) to 23 (11 p.m.)
mm: minutes, an integer from 0 to 59
ss: seconds, an integer from 0 to 59
ms: milliseconds, an integer greater than or equal to 0
2. new Date()——>time stamp, year, month, day, etc.
transfer timestamp
The timestamp refers to the total number of seconds from Greenwich Mean Time on January 1, 1970 (00:00:00 GMT) to the current time
var date = new Date()
date.valueOf() // 1601368680782
date.getTime() // 1601368680782
Number(date) // 1601368680782
// 以上返回的都是毫秒数,转成时间戳需要将毫秒转成秒
// 如:Math.round(new Date().getTime()/1000)
Math.round(date.getTime()/1000) // 1601368681
Get year, month and day
var date = new Date()
// 年
date.getFullYear() // 2020
// 月(实际月份为 结果 + 1,当前为9月)
date.getMonth() // 8
// 日
date.getDate() // 29
// 星期几
date.getDay() // 2 返回结果为0~6,表示一周中的某一天,0表示周日
Get hours, minutes and seconds
var date = new Date()
// 时
date.getHours() // 16
// 分
date.getMinutes() // 38
// 秒
date.getSeconds() // 0
// 毫秒
date.getMilliseconds() // 782
By obtaining the year, month, day, hour, minute and second, you can perform time difference calculations between multiple times. There are still many actual business scenarios, but there are many libraries now, such as moment.js, which encapsulate some operations and are easy to use
. convenient. But it is still necessary to understand the native implementation.
Convert Date object to string
Often used for a more intuitive display of the current Date.
var date = new Date()
date.toTimeString() // 16:38:00 GMT+0800 (中国标准时间)
date.toDateString() // Tue Sep 29 2020
date.toLocaleString()// 2020/9/29 下午4:38:00
date.toLocaleTimeString()// 下午4:38:00
date.toLocaleDateString()// 2020/9/29
Regarding toLocaleString
, there are some interesting applications. If you are interested, you can read this article of mine: toLocaleString is also very useful! (Super convenient to convert to thousandths, Chinese numbers, etc.)
3. Timestamp——>Normal time
var timestamp = 1601368681
new Date(timestamp * 1000).toLocaleString() // 2020/9/29 下午4:38:01
new Date(timestamp * 1000).toLocaleDateString() // 2020/9/29
In fact, it is to convert the timestamp into a Date object, and then you can use all the methods of the Date object above
To put it bluntly, converting a timestamp into a Date object is the fifth method of creating a specific time listed at the beginning of the article: new Date(ms)
multiply the timestamp by 1000 to get ms.
Above, the time formatting function can be encapsulated according to the above method. Here we only provide some basic methods. When I have time, I may publish an article on the function encapsulation of formatting time.
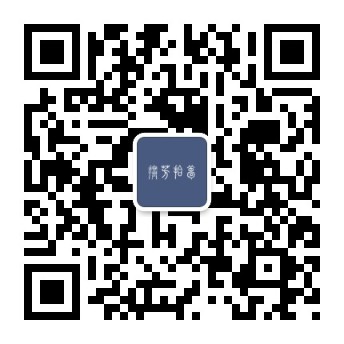