1. Introduction to stored procedures
A stored procedure is a collection of SQL statements that have been compiled in advance and stored in the database. Calling a stored procedure can simplify a lot of work for application developers, reduce the transmission of data between the database and the application server, and is helpful for improving the efficiency of data processing. Benefits. The concept of stored procedures is very simple, it is code encapsulation and reuse at the SQL language level of the database.
Features
- Encapsulation, reuse
- Can receive parameters and return data
- Reduce network interaction and improve efficiency
2. Basic syntax of stored procedures
2.1 Create
CREATE PROCEDURE 存储过程名称( [参数列表] )
BEGIN
SQL 语句
END;
2.2 Call
CALL name([parameter])
2.3 View
--Query the stored procedures and status information of the specified database
SELECT* FROM INFORMATION_SCHEMA.ROUTINES WHERE ROUTINE_SCHEMA = '对应的数据库名称'
--Query the definition of a stored procedure
SHOW CREATE PROCEDURE 存储过程名称
2.4 Delete
DROP PROCEDURE [ IFEXISTS ] 存储过程名称
3. Variables in stored procedures
3.1 System variables
System variables are provided by the MySQL server, not user-defined, and belong to the server level. Divided into global variables (GLOBAL) and session variables (SESSION).
--View system variables
SHOW [SESSION | GIOBAL] VARIABLFS; --View all system variables
SHOW [SESSION | GLOBAL] VARUABLES LIKE.....; --You can find variables through UKL fuzzy matching
SELECT @@[SESSION | GLOBAL] System variable name; --View the value of the specified variable
--Set system variables
SET [ SESSION | GLOBAL] system variable name = value;
SET @@[SESSION | GLOBAL] system variable name = value;
Note:
If SESSION/GLOBAL is not specified, the default is SESSION, the session variable.
After the mysql service is restarted, the set global parameters will become invalid. If you want them not to become invalid, you can configure them in /etc/my.cnf.
3.2 User-defined variables
User-defined variables are variables defined by users according to their own needs. User variables do not need to be declared in advance. They can be used directly with "@variable name" when using them. Its scope is the current connection.
--Assignment
SET @var_name = expr [,@var_name = expr ]... ;
SET @var_name := expr [,@var_name := exprl] ... ;
SELECT @var_name :=expr [,@var_name :=expr ] ..- ;
SELECT field name INTO@var_name FROM table name;
--Use
SELECT @var_name;
Notice:
User-defined variables do not need to be declared or initialized. If not declared or initialized, the value obtained will be NULL. As shown below: @abc is not declared. If you view the custom variable @abc, you will get null.
3.3 Local variables
Local variables are variables that are defined as needed and take effect locally. Before accessing, a DECLARE statement is required. Can be used as local variables and input parameters within stored procedures. The scope of local variables is the BEGIN.... END block declared within it.
Declare
DECLARE variable name variable type [DEFAULT...];
the variable type is the database field type: INT, BIGINT, CHAR.VARCHAR.DATE, TIME, etc.
Assignment
SET variable name = value;
SET variable name:= value;
SELECT field name INTO variable name FROM table name....;
4. Stored procedure – process control
4.1 Stored procedure-if judgment
Syntax:
IF condition 1 THEN
ELSEIF condition 2 THEN -- optional
ELSE -- optional
END IF;
Define the stored procedure to complete the following requirements:
determine the score level corresponding to the current score based on the defined score scre variable.
1.score >=85 points, the level is excellent.
2. Score >=60 points and score <85 points, the grade is passing.
3.Score<60 points, the grade is failing.
4.2 Stored procedure-parameters
usage:
CREATE PROCEDURE stored procedure name ([IN/OUT/INOUT parameter name parameter type])
BEGIN
--SQL statement
END;
Define the stored procedure to complete the following requirements:
1. Based on the incoming parameter score, determine the score level corresponding to the current score and return it.
①Score >=85 points, the level is excellent.
②Score >= 60 points and score <85 points, the grade is passing.
③Score<60 points, the grade is failing.
2. Convert the incoming 200-point score into a hundred-point system, and then return.
4.3 case
Define the stored procedure to complete the following requirements:
Based on the incoming month, determine the season to which the month belongs (case structure is required).
1. January to March is the first quarter.
2. April to June is the second quarter.
3. July to September is the third quarter.
4. October to December is the fourth quarter.
5. Stored procedure - loop
5.1 Loop-while
Define the stored procedure to complete the following requirements:
Calculate the value accumulated from 1 to n, where n is the passed parameter value.
5.2 Loop-repeat
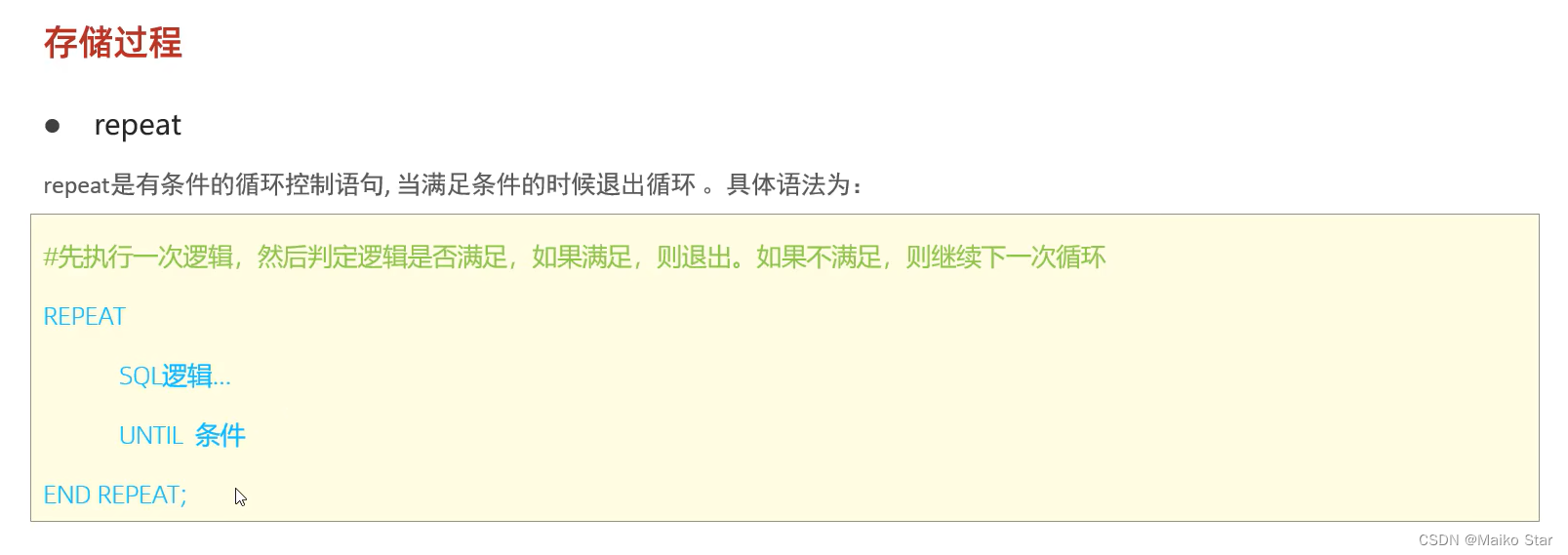
The repeat loop control statement will be executed at least once and exit when the conditions are met.
Define the stored procedure to complete the following requirements:
Calculate the value accumulated from 1 to n, where n is the passed parameter value.
5.3 loop-loop
LOOP implements a simple loop. If you do not add conditions for exiting the loop in the SQL logic, you can use it to implement a simple infinite loop. LOOP can be used with the following two statements:
① LEAVE: Used in conjunction with the cycle to exit the cycle.
② ITERATE: must be used in a loop. Its function is to skip the remaining statements of the current loop and directly enter the next loop.
grammar:
[begin_label:] LOOP
SQL逻辑..
END LOOP [end_ label];
LEAVE label; --Exit the loop body of the specified label
ITERATE label; --Enter the next loop directly
Define the stored procedure to complete the following requirements:
1. Calculate the value accumulated from 1 to n, where n is the parameter value passed in.
2. Calculate the accumulated even number value from 1 to n, where n is the passed parameter value.
6. Stored procedure-cursor-cursor
Cursor (CURSOR) is a data type used to store query result sets. Cursors can be used in stored procedures and functions to process the result set in a loop. The use of cursors includes cursor declaration, OPEN, FETCH and CLOSE. The syntax is as follows.
Declare the cursor
DECLARE cursor name CURSOR FOR query statement;
open the cursor
OPEN cursor name;
get the cursor record
FETCH cursor name INTO variable [, variable];
close the cursor
CLOSE cursor name;
Define the stored procedure to complete the following requirements:
According to the incoming parameter uage, query the user table tb_user for all users whose age is less than or equal to uage’s user name and profession, and insert the user’s name and profession into the created new table tb_user_pro (
id ,name,profession).
--Logic:
① Declare the cursor and store the query result set
②Preparation: Create table structure
③Open the cursor
④Get the records in the cursor
⑤Insert data into the new table
⑥Close the cursor
The complete stored procedure statement is as follows:
create procedure p1l(in uage int)
begin
declare uname varchar(100);
decLare upro varchar(100);
declare u_cursor cursor for select name,profession from tb_user where age <= uage;
当 条件处理程序的处理的状态码为02000的时候,就会退出。
declare exit handler for SQLSTATE '02000'close u_cursor;
drop table if exists tb_user_pro;
create table if not exists tb_user_pro(
id int primary key auto_increment,
name varchar(100),
profession varchar(100)
);
open u_cursor;
while true do
fetch u_cursor into uname,Upro;
insert into tb_user_pro values(null,uname,Upro);
end while;
close u_cursor;
end;
7. Stored procedure-condition handler-handle
8. Storage function
Define a storage function to complete the following requirements:
Calculate the value accumulated from 1 to n, where n is the passed parameter value.