1. What is a function?
Wikipedia definition of function: subroutine
· In computer science, a subroutine (English: Subroutine, procedure, function, routine, method,
subprogram, callable unit) is a certain part of code in a large program, consisting of one or more statement blocks
. It is responsible for completing a specific task and is relatively independent from other code.
· Generally, there are input parameters and return values, providing encapsulation and hiding of details of the process. These codes are usually integrated into software
libraries.
2. Classification of functions
Functions can be divided into two types: library functions and custom functions
2.1 Library functions
When we write programs, we may frequently use some functions, such as scanf, printf... and it would be too cumbersome to define them every time we use them.
So these describe the basic functions, they are not business code. Every programmer may use it during our development process. In order to support portability and improve program efficiency, the basic library of C language provides a series of similar library functions to facilitate programmers to develop software.
We introduce a website here: Reference - C++ Reference (cplusplus.com)
Here, we can access various library functions. To use these functions, we must include the corresponding header files #include.
2.2 Custom functions
I think custom functions refer to functions with special meaning used in specific scenarios.
The specific scenario can be: I forgot which library pow is in, and I need to use it, I can customize a pow function.
2.2.1 Syntax of custom functions
3. Function parameters
3.1 Actual parameters (actual parameters)
The parameters that are actually passed to the function are called actual parameters.
Actual parameters can be: constants, variables, expressions, functions, etc.
No matter what type of quantities the actual parameters are, they must have definite values when making a function call so that these values can be passed to the formal parameters.
3.2 Formal parameters (formal parameters)
Formal parameters refer to the variables in parentheses after the function name. Because formal parameters are only instantiated (memory units allocated) when the function is called, they are called formal parameters. Formal parameters are automatically destroyed after the function call is completed. Therefore formal parameters are only valid within functions.
3.3 Examples
We want to write a swap function. Let's look at two different ways of writing it.
After the program runs, we can see that Swap1 is invalid and Swap2 is valid. Let’s look at the function calls to understand this part!
4. Function call
4.1 Call by value
The formal parameters and actual parameters of a function occupy different memory blocks respectively, and modifications to the formal parameters will not affect the actual parameters.
The Swap1 above is a call by value, which is equivalent to the system opening up another space to store the parameters passed in, so only the values of the formal parameters are exchanged without changing the values of the actual parameters.
After the formal parameter is instantiated, it is actually equivalent to a temporary copy of the actual parameter.
4.2 Call by address
1. Calling by address is a way of calling a function by passing the memory address of the variable created outside the function to the function parameter.
2. This method of passing parameters can establish a real connection between the function and the variables outside the function, that is, the variables outside the function can be directly manipulated inside the function .
When a formal parameter needs to modify the value of an actual parameter, we can directly pass the address of the actual parameter to the function, so that the actual parameter that needs to be modified can be found directly through the address.
5. Nested calls and chained access of functions
Functions can be combined according to actual needs, that is, they can call each other.
5.1 Nested calls
In the main function, I just called three_line, but "hehe\n" was not printed in three_line. This is because new_line is nested in my three_line function and the new_line function is executed three times. It should be noted that the functions need to be defined separately. If I define new_line in three_line, this situation is not allowed.
Functions can be called nested, but not defined.
5.2 Chain access
Use the return value of one function as a parameter of another function.
Let's write a function:
The innermost printf printed 43 and the return value was 2; the middle printf printed 2 and the return value was 1; the last printf printed 1
Isn’t it interesting chained access?
6. Function declaration and definition
6.1 Function declaration
1. Tell the compiler what the function is called, what the parameters are, and what the return type is. But whether it exists or not cannot be determined by the function declaration.2. The declaration of a function generally appears before the use of the function. It must be declared first and then used .3. The function declaration is generally placed in the header file.
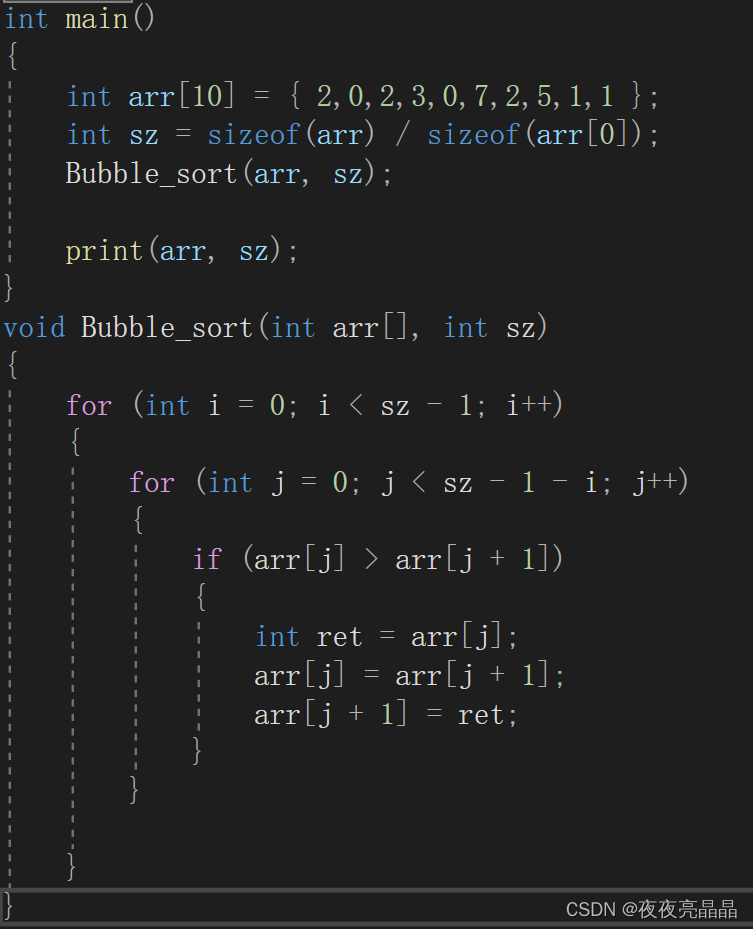
As above, if I write a bubble sort function, but I put it below the main function, can my main function call it?
The answer is yes, but because the compiler executes statements from top to bottom, you need to use function declarations.
Add a ; to the first line of the function you need to use and place it above the main function to complete the declaration and the compiler can use it normally.
6.2 Definition of function
The definition of a function refers to the specific implementation of the function and explains the functional implementation of the function.
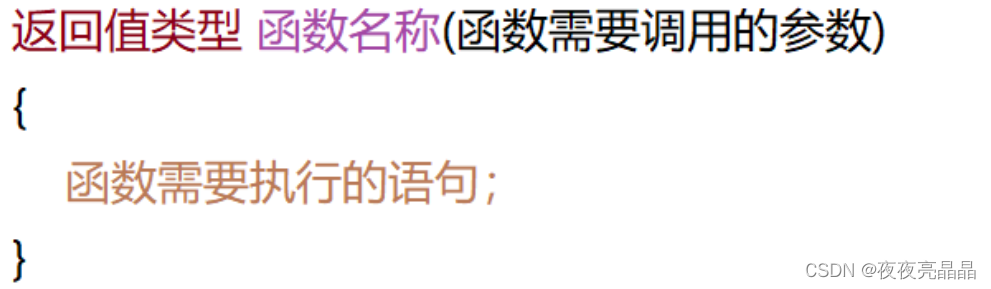
6.3 How to store functions in header files and source files
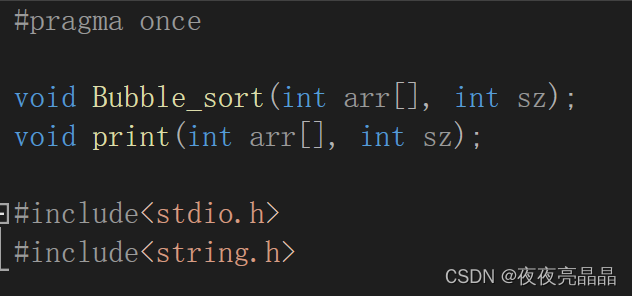
In the .c file, we can define the function . Before defining, we need to put #include ".h file". The .h file is different from stdio.h and needs to be "" instead of <>
When we have such .h and .c files, we can directly use #include ".h file" in our main file to implement the function call operation, which is equivalent to building a library function ourselves.
7. Recursion of functions (difficulty)
7.1 What is recursion?
The programming technique in which a program calls itself is called recursion.Recursion as an algorithm is widely used in programming languages. A process or function has a method of directly or indirectly calling itself in its definition or description. It usually transforms a large and complex problem into a smaller problem similar to the original problem to solve. The recursive strategy only A small number of programs are needed to describe the multiple repeated calculations required in the problem-solving process, which greatly reduces the amount of program code.The main way of thinking about recursion is to make big things smaller
7.2 Two necessary conditions for recursion
· There is a restriction condition . When this restriction condition is met, the recursion will not continue.· Each recursive call gets closer and closer to this limit.
7.3 Examples
We can see that recursion must have a limiting condition, and every time it is called, its value will change to a certain extent, and this change will change according to requirements.
7.4 Recursion and iteration
7.4.1 Stack overflow
Let’s write a Fibonacci sequence: 1, 1, 2, 3, 5, 8, 13, 21, 34…
Then let's output some results. We can find that when we enter 5 and 10, the program can still run normally, but when we enter 50, we can see that the cursor is flashing but the program does not respond. This is what we The recursive value is too large, causing the program to crash.
Every time we call a function recursively, the stack area of our computer will actually open up corresponding space for the function. However, when the number of recursions is too many, a stack overflow will occur.
The stack space allocated to the program by the system is limited, but if an infinite loop or (dead recursion) occurs, this may cause the stack space to be continuously allocated, and eventually the stack space will be exhausted. This phenomenon is called stack overflow. .
7.4.2 Iteration
Iteration is actually a loop