Table of contents
Method 1 (only for positive numbers):
Method 2 (universal for positive and negative numbers):
First, let’s take a look at the inverse and complement codes of the original code:
Secondly, let’s take a look at the shift operator << >>:
Finally, let’s take a look at the bit operator & | ^:
Method three (optimization of method two)
topic:
Write a function that returns the number of 1's in the parameter binary.
For example: 15 0000 1111 4 1
Prepare:
Create a function:
#include<stdio.h>
int CountOne(int num)
{
}
int main()
{
int num = 0;
scanf("%d", &num);
int ret = CountOne(num);
printf("%d\n",ret);
return 0;
}
Method 1 (only for positive numbers):
Ideas:
When we want to calculate a certain number of decimal parameters, we can use the modulo operation to make the parameter %10,
Thus, the last digit of the parameter is obtained, and then the parameter /10 is calculated, and then the modulo operation is performed...
In binary, we can also use the same method, we need to let the parameter %2,
You can get the last digit of the parameter, then let the parameter/2, and then perform the modulo operation...
Let’s first look at how to count the number of 1’s in decimal:
int CountOne(int num)
{
int count = 0;
while (num)
{
if (num % 10 == 1)
{
count++;
}
num /= 10;
}
return count;
}
answer:
By analogy, we can write to count the number of 1's in binary:
int CountOne(int num)
{
int count = 0;
while (num)
{
if (num % 2 == 1)
{
count++;
}
num /= 2;
}
return count;
}
By comparison, it is not difficult to see that only the numbers after % and / have been changed. In the same way, the number of a certain number in octal can also be calculated in this way.
Method 2 (universal for positive and negative numbers):
Foreword:
First, let’s take a look at the inverse and complement codes of the original code:
Secondly, let’s take a look at the shift operator << >>: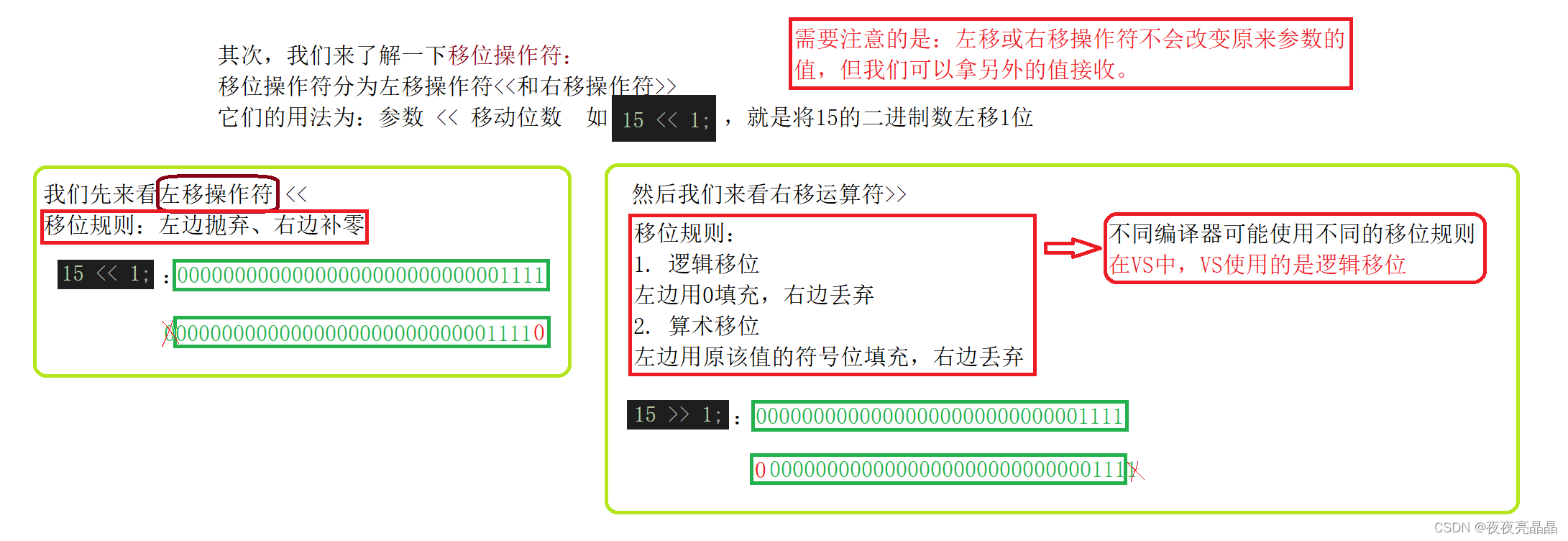
Of course, computers always shift the complement of integers.
Finally, let’s take a look at the bit operator & | ^:
Bitwise AND:
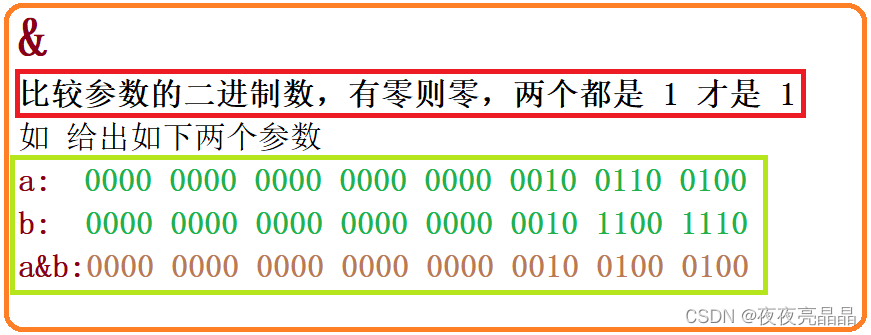
Bitwise OR |:
Bitwise XOR^: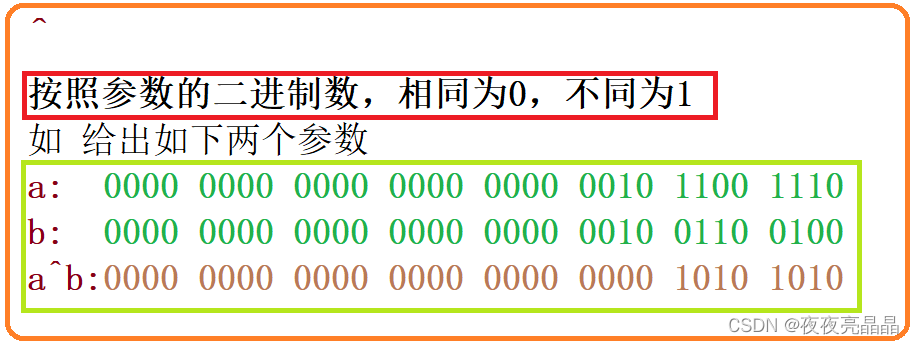
summary:
Knowing this, we can draw some small conclusions:
a&1=1 a|0=a a^0=a a^a=0
Ideas:
Through the shift operator, we can find each bit of the parameter binary to determine whether the result of each bit is 1.
answer:
We can think of moving the parameter by 1 bit each time, and then letting it be bitwise ANDed by 1 to determine whether its value is 1, but we also know that the shift operator will not change the value of the parameter, so we can move the parameter every time Move i position, each time i is moved, it will +1
int CountOne(int num)
{
int count = 0;
for (int i = 0; i < 32; i++)
{
if ((num >> i) & 1)
count++;
}
return count;
}
Method three (optimization of method two)
Ideas:
From method two, we can know that it will loop 32 times at any time, so how to optimize it? In fact, a very clever method is used here:
When the binary sequence n becomes n-1, the last 1 will become 0. When n&(n-1), because the last digits are different, the last 1 will be changed to 0, and the cycle repeats until When n is 0, stop looping. Let’s take 15 as an example:
answer:
int CountOne(int num)
{
int count = 0;
while (num)
{
count++;
num = num & (num - 1);
}
return count;
}