This project uses C++ to implement an address book management system.
Address book management system
1 System requirements
Address book is a tool that can record information about relatives and friends.
This tutorial mainly uses C++ to implement an address book management system
The functions that need to be implemented in the system are as follows:
- Add contacts: Add new people to the address book. Information includes (name, gender, age, contact number, home address). Up to 1,000 people can be recorded;
- Show Contacts: Display all contact information in the address book
- Delete Contacts: Delete specified contacts by name
- Find a contact: View the specified contact information by name
- Modify contact: Re-modify the designated contact by name
- Clear contacts: Clear all information in the address book
- Exit address book: exit the current address book
2 Create project
The steps to create a project are as follows:
- Create new project
- add files
2.1 Create project
After opening Visual studio, click Create New Project to create a new C++ project
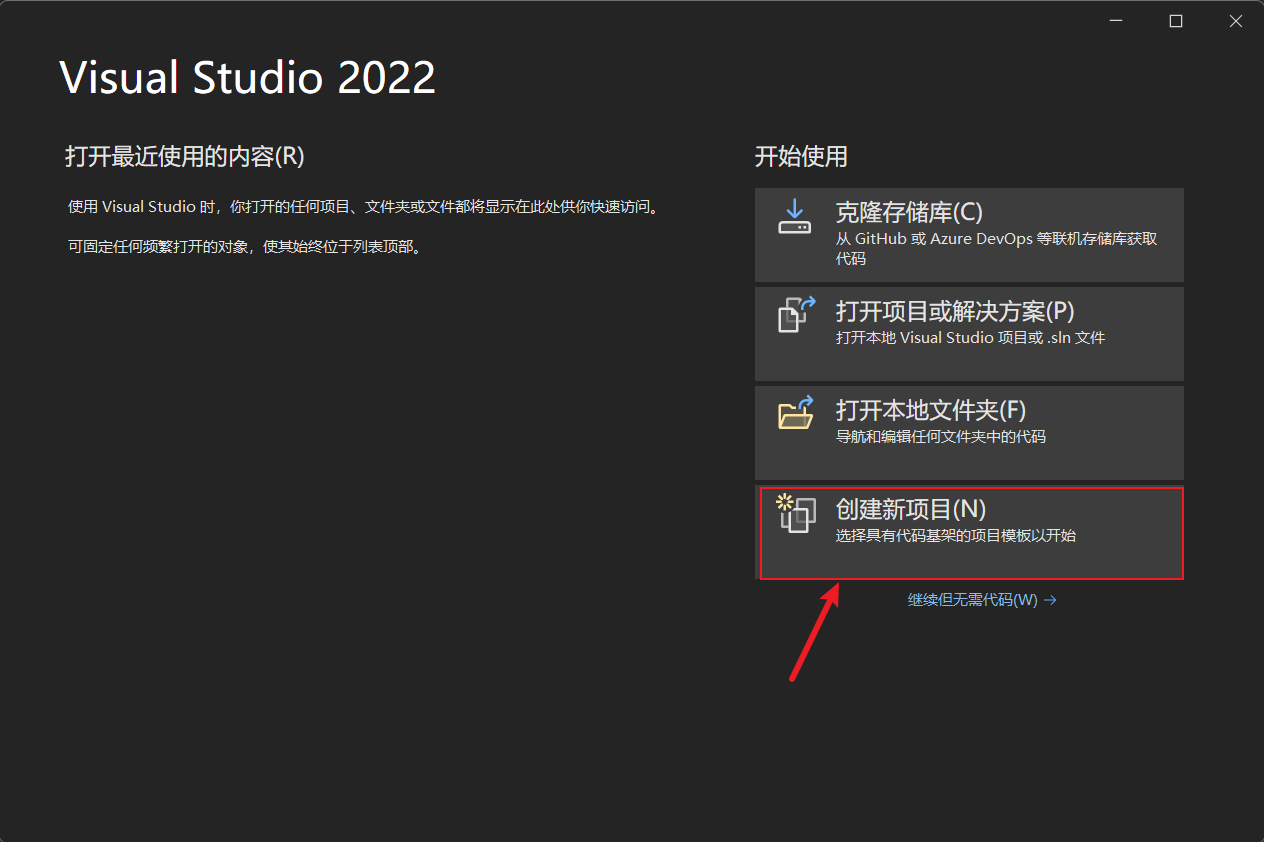
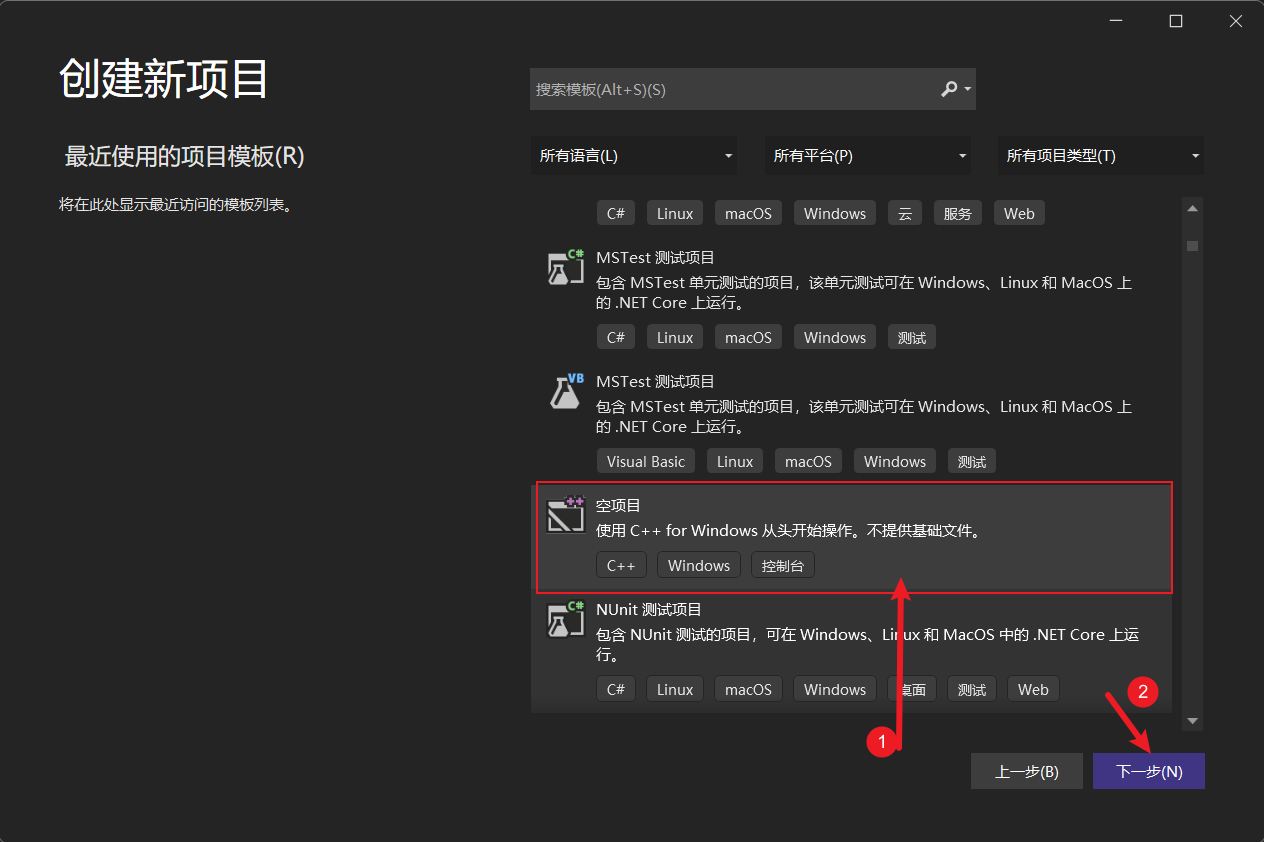
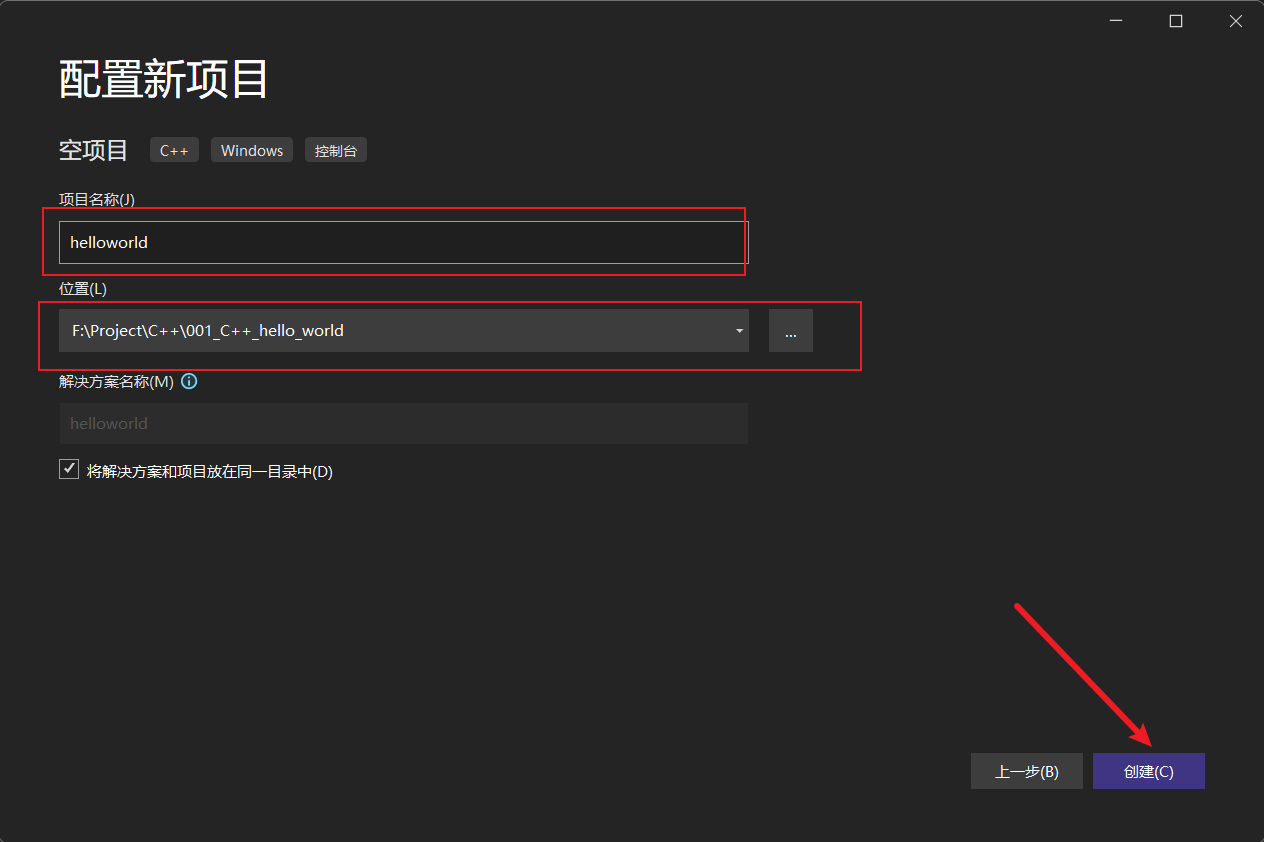
2.2 Create files
Right-click the source file and select Add->New Item
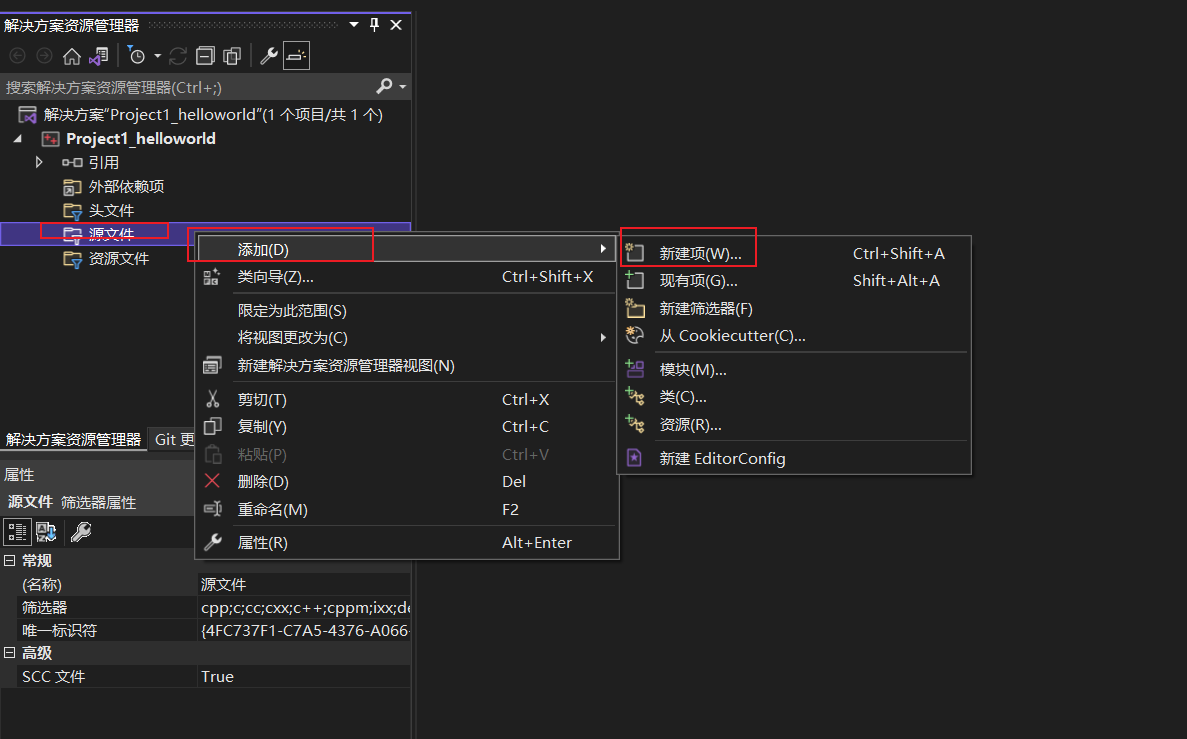
Give the C++ file a name and click Add.
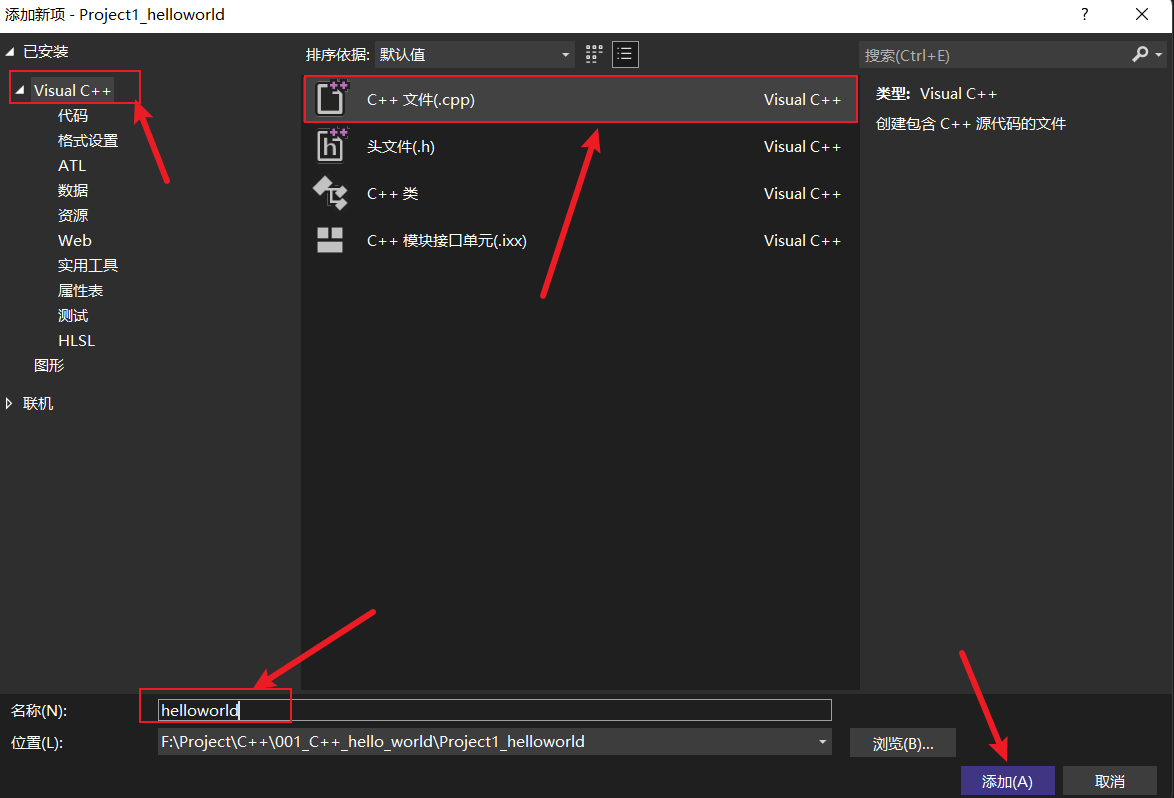
3 Menu functions
Function description : the user selects the program of the function
The effect of the menu interface is as follows:
Steps :
- The wrapper function displays the interface, such as
void showMenu()
- Call the encapsulated function in the main function
Code:
#include<iostream>
using namespace std;
//菜单界面
void showMenu() {
cout << "**************************" << endl;
cout << "***** 1.添加联系人 *****" << endl;
cout << "***** 2.显示联系人 *****" << endl;
cout << "***** 3.删除联系人 *****" << endl;
cout << "***** 4.查找联系人 *****" << endl;
cout << "***** 5.修改联系人 *****" << endl;
cout << "***** 6.清空联系人 *****" << endl;
cout << "***** 0.退出通讯录 *****" << endl;
cout << "**************************" << endl;
}
int main() {
//调用菜单
showMenu();
system("pause");
return 0;
}
4 Exit function
Function description: Exit the address book system
Idea: According to different choices of users, to enter different functions, you can choose the switch branch structure to build the entire architecture.
When the user selects 0, exit is executed. If the user selects other options, no operation will be performed first, and the program will not exit.
Code:
#include<iostream>
using namespace std;
//菜单界面
void showMenu() {
cout << "**************************" << endl;
cout << "***** 1.添加联系人 *****" << endl;
cout << "***** 2.显示联系人 *****" << endl;
cout << "***** 3.删除联系人 *****" << endl;
cout << "***** 4.查找联系人 *****" << endl;
cout << "***** 5.修改联系人 *****" << endl;
cout << "***** 6.清空联系人 *****" << endl;
cout << "***** 0.退出通讯录 *****" << endl;
cout << "**************************" << endl;
}
int main() {
int select = 0;//创建用户选择的输入的变量
while (true) {
//调用菜单
showMenu();
cin >> select;
switch (select) {
case 1://添加联系人
break;
case 2://显示联系人
break;
case 3://删除联系人
break;
case 4://查找联系人
break;
case 5://修改联系人
break;
case 6://清空联系人
break;
case 0://退出通讯录
cout << "欢迎下次使用!" << endl;
system("pause");//按任意键退出系统
return 0;
break;
default:break;
}
}
system("pause");
return 0;
}
Rendering:
5 Add contacts
Function description:
Implement the function of adding contacts. The upper limit of contacts is 1,000 people. Contact information includes (name, gender, age, contact number, home address)
Steps to add a contact:
- Design contact structure
- Design address book structure
- Create an address book in the main function
- Encapsulate the add contact function
- Test the function of adding contacts
5.1 Design the contact structure
Contact information includes: name, gender, age, contact number, home address
The design is as follows:
#include<string> //string头文件
//联系人结构体
struct Person
{
string m_Name;//姓名
int m_Sex;//性别
int m_Age;//年龄
string m_Phone;//电话
string m_Addr;//住址
};
5.2 Design the address book structure
When designing, you can maintain an array with a capacity of 1000 to store contacts in the address book structure, and record the number of contacts in the current address book.
The design is as follows:
#define MAX 1000 //
//通讯录结构体
struct Addressbooks{
struct Person personArray[MAX];//通讯录中保存的联系人数组
int m_Size;//通讯录中人员个数
}
5.3 Create address book in main function
After adding the contact function and encapsulating it, create an address book variable in the main function. This is the address book we need to maintain all the time.
Add at the beginning of the main function:
Addressbooks abs;//创建通讯录
abs.m_Size = 0;//初始化通讯录中的人数
5.4 Encapsulate the add contact function
Idea: Before adding contacts, first determine whether the address book is full. If it is full, no more additions will be made. If it is not full, add new contact information to the address book one by one.
Add contact code:
void addPerson(Addressbooks* abs) {
if (abs->m_Size == MAX) {
cout << "通讯录已满,无法添加!" << endl;
return;
}
else {
string name;
cout << "请输入姓名:" << endl;
cin >> name;
abs->personArray[abs->m_Size].m_Name = name;
int sex = 0;
cout << "请输入性别:" << "1 --- 男" << "2 --- 女" << endl;
while (true) {
cin >> sex;
if (sex == 1 || sex == 2) {
abs->personArray[abs->m_Size].m_Sex = sex;
break;
}
else {
cout << "输入错误,请重新输入:" << endl;
}
}
int age = 0;
cout << "请输入年龄:" << endl;
while (true) {
cin >> age;
if (age >= 0 || age <= 150) {
abs->personArray[abs->m_Size].m_Age = age;
break;
}
else {
cout << "输入错误,请重新输入:" << endl;
}
}
string phonenumber;
cout << "请输入联系电话:" << endl;
cin >> phonenumber;
abs->personArray[abs->m_Size].m_Phone = phonenumber;
string address;
cout << "请输入家庭住址:" << endl;
cin >> address;
abs->personArray[abs->m_Size].m_Addr = address;
abs->m_Size++;//更新通讯录人数
cout << "添加成功!" << endl;
system("pause");//按任意键继续
system("cls");//清屏操作
}
}
5.5 Test the function of adding contacts
In the selection interface, if the player selects 1, it means adding a contact. You can test this function.
In the switch case statement, add in case1:
case 1://添加联系人
addPerson(&abs);//利用地址传递,可以修饰形参
break;
Test renderings:
6 Show contacts
Function description: Display existing contact information in the address book
Steps to display contacts:
- Encapsulate display contact function
- Test the display of contacts function
6.1 Package display contact function
Idea: Determine if there are people in the current address book. If not, the record will be empty. If the number of people is greater than 0, the information in the address book will be displayed.
Show contact code:
//显示所有联系人
void showPerson(Addressbooks* abs) {
if (abs->m_Size == 0) {
cout << "通讯录为空。" << endl;
}
else {
for (int i = 0; i < abs->m_Size; i++) {
cout << "姓名:" << abs->personArray[i].m_Name<<"\t";
cout << "年龄:\t" << abs->personArray[i].m_Age << "\t";
cout << "性别:\t" << (abs->personArray[i].m_Sex == 1 ? "男" : "女") << "\t";
cout << "电话:\t" << abs->personArray[i].m_Phone << "\t";
cout << "地址:" << abs->personArray[i].m_Addr << endl;
}
}
system("pause");
system("cls");
}
6.2 Test the function of displaying contacts
In the switch case statement, add in case 2
case 2://显示联系人
showPerson(&abs);
break;
Test renderings:
7 Delete contacts
Function description: Delete specified contacts by name
Steps to delete a contact:
- Encapsulation detects whether a contact exists
- Encapsulate delete contact function
- Test the delete contact function
7.1 Encapsulation detects whether the contact exists
Design idea: Before deleting a contact, it is necessary to first determine whether the contact entered by the user exists. If it exists, delete it. If it does not exist, the user will be prompted that there is no contact to delete. Therefore, the detection of whether the contact exists is encapsulated into a function. If it exists, , returns the contact's position in the address book, returns -1 if it does not exist.
//检测联系人是否存在,如果存在,返回联系人所在数组中的具体位置,不存在返回-1
int isExist(Addressbooks* abs, string name) {
for (int i = 0; i < abs->m_Size; i++) {
if (abs->personArray[i].m_Name == name) {
return i;//找到,返回这个人在数组中的编号
}
}
return -1;//如果没有找到,返回-1
}
7.2 Encapsulate delete contact function
Determine whether there is this person in the address book based on the contact entered by the user, search for and delete it, and prompt that the deletion is successful; if the person cannot be found, it will prompt that the person is not found.
void deletePerson(Addressbooks* abs) {
cout << "请输入您要删除的联系人:" << endl;
string name;
cin >> name;
int ret = isExist(abs, name);
if (ret != -1) {
//删除李四,将李四后的数据前移
for (int i = ret; i < abs->m_Size; i++) {
abs->personArray[i] = abs->personArray[i + 1];//数据前移
}
abs->m_Size--;//更新通讯录中的人员数
cout << "删除成功!" << endl;
}
else {
cout << "查无此人!" << endl;
}
system("pause");
system("cls");
}
7.3 Test delete contact function
In the switch case statement, add in case3:
case 3://删除联系人
deletePerson(&abs);
break;
8 Find a contact
Function description: View specified contact information by name
Steps to find a contact
- Encapsulate the lookup contact function
- Test to find the specified contact
8.1 Encapsulating the function of finding contacts
Implementation idea: Determine whether the contact specified by the user exists. If it exists, display the information, otherwise it will prompt that the person is not found.
Find contact code:
void findPerson(Addressbooks* abs) {
cout << "请输入您要查找的联系人:" << endl;
string name;
cin >> name;
int ret = isExist(abs, name);
if (ret != -1) {
//找到联系人
cout << "姓名:" << abs->personArray[ret].m_Name << "\t";
cout << "年龄:\t" << abs->personArray[ret].m_Age << "\t";
cout << "性别:\t" << (abs->personArray[ret].m_Sex == 1 ? "男" : "女") << "\t";
cout << "电话:\t" << abs->personArray[ret].m_Phone << "\t";
cout << "地址:" << abs->personArray[ret].m_Addr << endl;
}
else {
cout << "查无此人!" << endl;
}
system("pause");
system("cls");
}
8.2 Test to find the specified contact
In the switch case statement, add in case4:
case 4://查找联系人
findPerson(&abs);
break;
9 Modify contacts
Function description: Re-modify the designated contact by name
Steps to modify a contact:
- Encapsulate the modify contact function
- Test the function of modifying contacts
9.1 Encapsulate the contact function
Implementation idea: Search for the contact person entered by the user. If the modification operation is successful, if the search fails, it will prompt that this person is not found.
Modify contact code:
void modifyPerson(Addressbooks* abs) {
cout << "请输入您要修改的联系人:" << endl;
string name;
cin >> name;
int ret = isExist(abs, name);
if (ret != -1) {
//找到联系人
string name1;
cout << "请输入姓名:" << endl;
cin >> name1;
abs->personArray[ret].m_Name = name1;
int sex = 0;
cout << "请输入性别:" << endl;
while (true) {
cout << "1 - 男" << " 2 - 女" << endl;
cin >> sex;
if (sex == 1 || sex == 2) {
abs->personArray[ret].m_Sex = sex;
break;
}
else {
cout << "输入有误,请重新输入:" << endl;
}
}
int age = 0;
cout << "请输入年龄:" << endl;
while (true) {
cin >> age;
if (age >= 0 || age <= 150) {
abs->personArray[ret].m_Age = age;
break;
}
else {
cout << "输入错误,请重新输入:" << endl;
}
}
string phonenumber;
cout << "请输入联系电话:" << endl;
cin >> phonenumber;
abs->personArray[ret].m_Phone = phonenumber;
string address;
cout << "请输入家庭住址:" << endl;
cin >> address;
abs->personArray[ret].m_Addr = address;
cout << "修改成功!" << endl;
system("pause");//按任意键继续
system("cls");//清屏操作
}
else {
cout << "查无此人!" << endl;
}
}
9.2 Test the function of modifying contacts
In the switch case statement, add in case5:
case 5://修改联系人
modifyPerson(&abs);
break;
10 Clear contacts
Function description: Clear all information in the address book
Steps to clear contacts
- Encapsulate clear contact function
- Test clearing contacts
10.1 Encapsulate the clear contact function
Implementation idea: Clear all contact information in the address book. Just set the number of contacts recorded in the address book to 0 and clear it logically.
Clear contact code:
void cleanPerson(Addressbooks* abs) {
abs->m_Size = 0;
cout << "通讯录已清空!" << endl;
system("pause");//按任意键继续
system("cls");//清屏操作
}
10.2 Test clearing contacts
In the switch case statement, add in case6:
case 6://清空联系人
cleanPerson(&abs);
break;
11 Overall Program
#include<iostream>
using namespace std;
#include<string> //string头文件
#define MAX 1000 //
//设计联系人的结构体
struct Person
{
string m_Name;//姓名
int m_Sex;//性别
int m_Age;//年龄
string m_Phone;//电话
string m_Addr;//住址
};
//通讯录结构体
struct Addressbooks {
struct Person personArray[MAX];//通讯录中保存的联系人数组
int m_Size;//通讯录中人员个数
};
//1.添加联系人
void addPerson(Addressbooks* abs) {
if (abs->m_Size == MAX) {
cout << "通讯录已满,无法添加!" << endl;
return;
}
else {
string name;
cout << "请输入姓名:" << endl;
cin >> name;
abs->personArray[abs->m_Size].m_Name = name;
int sex = 0;
cout << "请输入性别:" << "1 - 男" << " 2 - 女" << endl;
while (true) {
cin >> sex;
if (sex == 1 || sex == 2) {
abs->personArray[abs->m_Size].m_Sex = sex;
break;
}
else {
cout << "输入错误,请重新输入:" << endl;
}
}
int age = 0;
cout << "请输入年龄:" << endl;
while (true) {
cin >> age;
if (age >= 0 || age <= 150) {
abs->personArray[abs->m_Size].m_Age = age;
break;
}
else {
cout << "输入错误,请重新输入:" << endl;
}
}
string phonenumber;
cout << "请输入联系电话:" << endl;
cin >> phonenumber;
abs->personArray[abs->m_Size].m_Phone = phonenumber;
string address;
cout << "请输入家庭住址:" << endl;
cin >> address;
abs->personArray[abs->m_Size].m_Addr = address;
abs->m_Size++;//更新通讯录人数
cout << "添加成功!" << endl;
system("pause");//按任意键继续
system("cls");//清屏操作
}
}
//显示所有联系人
void showPerson(Addressbooks* abs) {
if (abs->m_Size == 0) {
cout << "通讯录为空。" << endl;
}
else {
for (int i = 0; i < abs->m_Size; i++) {
cout << "姓名:" << abs->personArray[i].m_Name<<"\t";
cout << "年龄:\t" << abs->personArray[i].m_Age << "\t";
cout << "性别:\t" << (abs->personArray[i].m_Sex == 1 ? "男" : "女") << "\t";
cout << "电话:\t" << abs->personArray[i].m_Phone << "\t";
cout << "地址:" << abs->personArray[i].m_Addr << endl;
}
}
system("pause");
system("cls");
}
//检测联系人是否存在,如果存在,返回联系人所在数组中的具体位置,不存在返回-1
int isExist(Addressbooks* abs, string name) {
for (int i = 0; i < abs->m_Size; i++) {
if (abs->personArray[i].m_Name == name) {
return i;//找到,返回这个人在数组中的编号
}
}
return -1;//如果没有找到,返回-1
}
void deletePerson(Addressbooks* abs) {
cout << "请输入您要删除的联系人:" << endl;
string name;
cin >> name;
int ret = isExist(abs, name);
if (ret != -1) {
//删除李四,将李四后的数据前移
for (int i = ret; i < abs->m_Size; i++) {
abs->personArray[i] = abs->personArray[i + 1];//数据前移
}
abs->m_Size--;//更新通讯录中的人员数
cout << "删除成功!" << endl;
}
else {
cout << "查无此人!" << endl;
}
system("pause");
system("cls");
}
void findPerson(Addressbooks* abs) {
cout << "请输入您要查找的联系人:" << endl;
string name;
cin >> name;
int ret = isExist(abs, name);
if (ret != -1) {
//找到联系人
cout << "姓名:" << abs->personArray[ret].m_Name << "\t";
cout << "年龄:\t" << abs->personArray[ret].m_Age << "\t";
cout << "性别:\t" << (abs->personArray[ret].m_Sex == 1 ? "男" : "女") << "\t";
cout << "电话:\t" << abs->personArray[ret].m_Phone << "\t";
cout << "地址:" << abs->personArray[ret].m_Addr << endl;
}
else {
cout << "查无此人!" << endl;
}
system("pause");
system("cls");
}
void modifyPerson(Addressbooks* abs) {
cout << "请输入您要修改的联系人:" << endl;
string name;
cin >> name;
int ret = isExist(abs, name);
if (ret != -1) {
//找到联系人
string name1;
cout << "请输入姓名:" << endl;
cin >> name1;
abs->personArray[ret].m_Name = name1;
int sex = 0;
cout << "请输入性别:" << endl;
while (true) {
cout << "1 - 男" << " 2 - 女" << endl;
cin >> sex;
if (sex == 1 || sex == 2) {
abs->personArray[ret].m_Sex = sex;
break;
}
else {
cout << "输入有误,请重新输入:" << endl;
}
}
int age = 0;
cout << "请输入年龄:" << endl;
while (true) {
cin >> age;
if (age >= 0 || age <= 150) {
abs->personArray[ret].m_Age = age;
break;
}
else {
cout << "输入错误,请重新输入:" << endl;
}
}
string phonenumber;
cout << "请输入联系电话:" << endl;
cin >> phonenumber;
abs->personArray[ret].m_Phone = phonenumber;
string address;
cout << "请输入家庭住址:" << endl;
cin >> address;
abs->personArray[ret].m_Addr = address;
cout << "修改成功!" << endl;
system("pause");//按任意键继续
system("cls");//清屏操作
}
else {
cout << "查无此人!" << endl;
}
}
void cleanPerson(Addressbooks* abs) {
abs->m_Size = 0;
cout << "通讯录已清空!" << endl;
system("pause");//按任意键继续
system("cls");//清屏操作
}
//菜单界面
void showMenu() {
cout << "**************************" << endl;
cout << "***** 1.添加联系人 *****" << endl;
cout << "***** 2.显示联系人 *****" << endl;
cout << "***** 3.删除联系人 *****" << endl;
cout << "***** 4.查找联系人 *****" << endl;
cout << "***** 5.修改联系人 *****" << endl;
cout << "***** 6.清空联系人 *****" << endl;
cout << "***** 0.退出通讯录 *****" << endl;
cout << "**************************" << endl;
}
int main() {
Addressbooks abs;//创建通讯录
abs.m_Size = 0;//初始化通讯录中的人数
int select = 0;//创建用户选择的输入的变量
while (true) {
//调用菜单
showMenu();
cin >> select;
switch (select) {
case 1://添加联系人
addPerson(&abs);//利用地址传递,可以修饰形参
break;
case 2://显示联系人
showPerson(&abs);
break;
case 3://删除联系人
deletePerson(&abs);
break;
case 4://查找联系人
findPerson(&abs);
break;
case 5://修改联系人
modifyPerson(&abs);
break;
case 6://清空联系人
cleanPerson(&abs);
break;
case 0://退出通讯录
cout << "欢迎下次使用!" << endl;
system("pause");//按任意键继续
return 0;
break;
default:break;
}
}
system("pause");
return 0;
}