Article directory
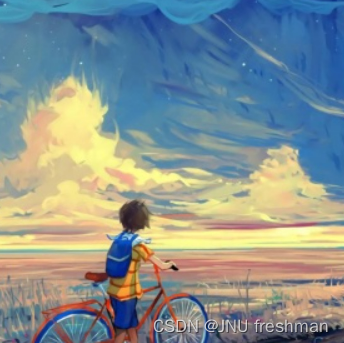
General introduction
When it comes to classes in Unity Vector3
, here are some commonly used methods and operations:
-
magnitude
Method : Returns the length of the vector.float length = vector.magnitude;
-
sqrMagnitude
Method : Returns the square length of a vector, usually used to compare vector sizes without performing the square root operation, thus improving efficiency.float squaredLength = vector.sqrMagnitude;
-
normalized
Method : Returns the unit vector of a vector, that is, a vector with length 1 but the same direction.Vector3 normalizedVector = vector.normalized;
-
Vector3.Dot
Method : Calculate the dot product (inner product) of two vectors, which is used to measure the similarity between the two vectors.float dotProduct = Vector3.Dot(vectorA, vectorB);
-
Vector3.Cross
Method : Calculate the cross product (outer product) of two vectors, which is used to calculate the vector perpendicular to the two vectors.Vector3 crossProduct = Vector3.Cross(vectorA, vectorB);
-
Vector3.Lerp
Method : Linear interpolation between two vectors.Vector3 interpolatedVector = Vector3.Lerp(startVector, endVector, t);
-
Vector3.Distance
Method : Calculate the distance between two vectors.float distance = Vector3.Distance(vectorA, vectorB);
-
Vector3.Angle
Method : Calculate the angle between two vectors.float angle = Vector3.Angle(vectorA, vectorB);
-
Vector3.Project
Method : Project one vector onto another vector to get a projected vector.Vector3 projection = Vector3.Project(vectorToProject, ontoVector);
-
Vector3.Reflect
Method : Calculate the reflection vector of a vector about a normal.Vector3 reflection = Vector3.Reflect(incidentVector, normal);
These methods can be used to perform various vector calculations, including measurements, transformations, rotations, collision detection, and more. Depending on your specific needs, choosing an appropriate method can help you handle vector operations more easily.
Small scale chopper
Here is a sample code that demonstrates how to use Vector3
the different methods of the class, with detailed commented instructions:
using UnityEngine;
public class VectorMethodsExample : MonoBehaviour
{
public Transform target; // 用于演示的目标对象
public Transform otherObject; // 用于演示的另一个对象
private void Update()
{
Vector3 moveDirection = target.position - transform.position;
// 获取向量的长度
float magnitude = moveDirection.magnitude;
// 获取向量的平方长度
float sqrMagnitude = moveDirection.sqrMagnitude;
// 归一化向量
Vector3 normalizedDirection = moveDirection.normalized;
// 计算两个向量的点积
float dotProduct = Vector3.Dot(moveDirection, otherObject.position - transform.position);
// 计算两个向量的叉积
Vector3 crossProduct = Vector3.Cross(moveDirection, otherObject.position - transform.position);
// 在两个向量之间进行线性插值
float t = Mathf.PingPong(Time.time, 1f); // 0 到 1 之间的插值参数
Vector3 interpolatedVector = Vector3.Lerp(moveDirection, otherObject.position - transform.position, t);
// 计算两个向量之间的距离
float distance = Vector3.Distance(transform.position, otherObject.position);
// 计算两个向量之间的夹角
float angle = Vector3.Angle(moveDirection, otherObject.position - transform.position);
// 将一个向量投影到另一个向量上
Vector3 projectedVector = Vector3.Project(moveDirection, otherObject.position - transform.position);
// 计算一个向量关于一个法线的反射向量
Vector3 normal = Vector3.up; // 示例法线
Vector3 reflection = Vector3.Reflect(moveDirection, normal);
Debug.Log("Magnitude: " + magnitude);
Debug.Log("Squared Magnitude: " + sqrMagnitude);
Debug.Log("Normalized Direction: " + normalizedDirection);
Debug.Log("Dot Product: " + dotProduct);
Debug.Log("Cross Product: " + crossProduct);
Debug.Log("Interpolated Vector: " + interpolatedVector);
Debug.Log("Distance: " + distance);
Debug.Log("Angle: " + angle);
Debug.Log("Projected Vector: " + projectedVector);
Debug.Log("Reflection: " + reflection);
}
}
Note that this is just an example to demonstrate Vector3
how the various methods can be used. In practical applications, you need to adjust according to the specific situation. Hope this example can help you better understand and use Vector3
the methods of the class.
Related description parameters
"Vector3" is generally a data structure or class that represents a vector in three-dimensional space, and its name indicates that it is a vector with three components. In many programming languages and math libraries, this type of vector is often used to represent concepts such as position, orientation, or displacement.
An ordinary three-dimensional vector itself usually contains only three components: x, y and z.
Look at a small example
This line of code is a common operation in many game development environments and is used to create a three-dimensional vector (Vector3) that represents a movement direction or displacement. Let's explain each part of this line of code step by step:
Vector3 moveDirection = new Vector3(horizontalInput, 0f, verticalInput);
-
Vector3
: This is a class or structure representing a three-dimensional vector. It usually contains three components: x, y and z, corresponding to the horizontal, vertical and depth directions in three-dimensional space. -
moveDirection
: This is a variable name used to store a three-dimensional vector representing the movement direction or displacement. You can think of it as a container for storing information such as position, direction or speed. -
new Vector3(horizontalInput, 0f, verticalInput)
: This is anVector3
expression that passes parameters to the constructor to create a new three-dimensional vector. Among them,horizontalInput
andverticalInput
are variables, which may represent the input of the input device (such as keyboard, controller), indicating the player's input value in the horizontal and vertical directions. These input values can be of float (float
) type.horizontalInput
: Represents the input value in the horizontal direction. Usually, left minus right is a common convention.0f
: This value represents movement in the y direction, usually set to 0, because in many games, the vertical direction is usually used to represent height, and in this case of up and down movement, the height does not change.verticalInput
: Represents the input value in the vertical direction. Usually, bottom and top are just a common convention.
So what the code means is to create a moveDirection
three-dimensional vector called that represents the direction of movement based on the player's input in the horizontal and vertical directions. This vector can be used to move various game objects, such as player characters, cameras, bullets, etc.