Table of contents
Dynamic memory management functions
Common memory allocation usage error cases
Several classic written test questions:
malloc function
#include<stdlib.h>
int main()
{
void *malloc( size_t size );//size : 要开辟的字节数
return 0;
}
Definition: Allocate a block of memory.
Header file: <stdlib.h>
Note:
- This function applies for a contiguous available space from memory and returns a pointer to this space.
- If the allocation is successful, a pointer to the allocated space is returned.
- If the opening fails, a NULL pointer is returned , so the return value of malloc must be checked.
- The type of the return value is void* , so the malloc function does not know the type of the opened space, and the user can decide by himself when using it.
- If the parameter size is 0 , the behavior of malloc is undefined by the standard and depends on the compiler.
example:
#include<stdio.h>
#include<stdlib.h>
#define MAX 10
int main()
{
int* ptr = (int*)malloc(MAX);
if ( NULL != ptr)
{
//使用空间
}
else
{
perror("malloc");
}
return 0;
}
free function
#include<stdlib.h>
int main()
{
void free(void* ptr);
return 0;
}
Definition: Reclaim/release memory blocks
Header file: <stdlib.h>
Note:
- The free function frees a memory block (memblock) previously allocated by calling callloc, malloc, or realloc. The number of bytes freed is equal to the number of bytes requested when the block was allocated (or reallocated in the case of realloc). If memblock is NULL, the pointer is ignored and free returns immediately.
- Attempting to free an invalid pointer (a pointer to a memory block not allocated by calloc, malloc, or realloc) may affect subsequent allocation requests and cause errors ( if the space pointed to by the parameter ptr is not dynamically allocated, the behavior of the free function is undefined ) .
example:
#include<stdio.h>
#include<stdlib.h>
#define MAX 10
int main()
{
//申请空间
int* ptr = (int*)malloc(MAX);
if ( NULL != ptr)
{
//使用空间
}
else
{
perror("malloc");
}
//释放空间
free(ptr);
ptr = NULL;//防止ptr再次使用,造成非法访问
return 0;
}
calloc function
#include<stdlib.h>
int main()
{
void* calloc(size_t num, size_t size);
return 0;
}
Definition: Allocate an array in memory whose elements are initialized to 0.
Header file: <stdlib.h>
Note:
- The function of the function is to open up a space for num elements whose size is size , and initialize each byte of the space to 0 .
- The only difference from the function malloc is that calloc will initialize each byte of the requested space to all 0s before returning the address .
example:
#include<stdio.h>
#include<stdlib.h>
#define MAX 10
int main()
{
int* ptr = calloc(MAX, sizeof(int));
if (NULL != ptr)
{
//使用空间
}
else
{
perror("calloc");
}
free(ptr);
ptr = NULL;
return 0;
}
realloc function
#include<stdlib.h>
int main()
{
void* realloc(void* ptr, size_t size);
return 0;
}
Definition: Reallocate a block of memory
Header file: <stdlib.h>
Note:
- ptr is the memory address to be adjusted
- New size after size adjustment
- The return value is the memory starting position after adjustment.
- On the basis of adjusting the size of the original memory space, this function will also move the data in the original memory to the new space.
- There are two situations when realloc adjusts the memory space:
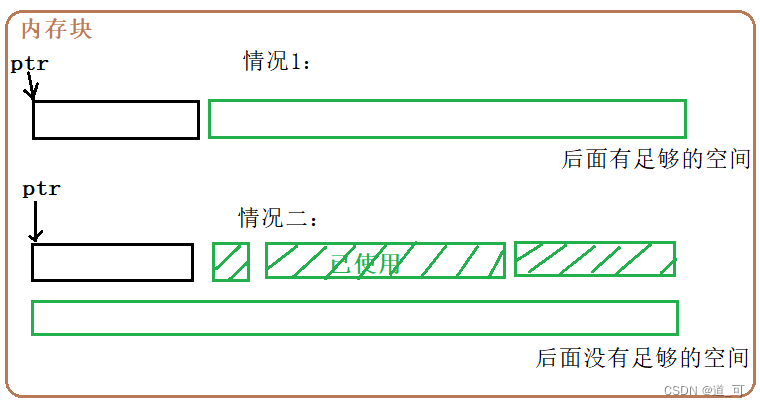
Case 2: When there is not enough space after the original space, the expansion method is to find another continuous space of appropriate size on the heap space to use. This function returns a new memory address.
example:
#include<stdio.h>
#include<stdlib.h>
#define MAX 10
int main()
{
//申请空间
int* ptr = (int*)malloc(MAX);
if (NULL != ptr)
{
//使用空间
}
else
{
perror("malloc");
}
ptr = (int*)realloc(ptr, 100);
if (NULL != ptr)
{
//使用空间
}
else
{
perror("realloc");
}
//释放空间
free(ptr);
ptr = NULL;//防止ptr再次使用,造成非法访问
return 0;
}
Common memory allocation usage error cases:
Several classic written test questions:
What's wrong with the code below?
1.
Answer: The main function calls the test function, enters the test function to create a null pointer str, and passes str to the getmemory function. At this time, p is a temporary copy of the str pointer variable. The starting address of the space opened by malloc is passed to p. For p The modification will not affect str, and the getmemory function will be used, and the formal parameters will be automatically destroyed, causing memory leaks . At this time, inside the test function, str is a null pointer, and the null pointer is passed to the strcpy function, and the null pointer is dereferenced, causing the program to crash .
2.
Answer: The array p is a temporary variable and is destroyed when the getmemory function exits. At this time, the space opened by the p array has been reclaimed by the operating system. p is a wild pointer and has no permission to use it. However, the address of p is passed to str, so it is illegal. access space
3.
Answer: Forgot to release the space requested by malloc
4.
Answer: The space applied for by str has been released by free, but str was not set to a null pointer in time. Here, str has become a wild pointer after free, and dereference causes illegal access.