just-in-time compiler
The HotSpot virtual machine contains two just-in-time compilers, namely the client-side compiler (referred to as C1) which takes a short time to compile but has a low degree of output code optimization; and the server-side compiler which takes a long time to compile but has a higher output code optimization quality. (referred to as C2), usually they cooperate with the interpreter under a layered compilation mechanism to form the execution subsystem of the HotSpot virtual machine.
Since JDK 10, a new just-in-time compiler has been added to HotSpot: the Graal compiler. The Graal compiler debuts as a replacement for the C2 compiler.
Runtime data area
1. Program counter
The Program Counter Register is a small memory space. It can be regarded as a line number indicator of the bytecode executed by the current thread, and stores the offset of the bytecode executed by the current thread . In the conceptual model of the Java virtual machine, the bytecode interpreter works by changing the value of this counter to select the next bytecode instruction that needs to be executed. It is an indicator of program control flow, branches, loops, and jumps . , exception handling, thread recovery and other basic functions all need to rely on this counter to complete.
Since the multi-threading of the Java virtual machine is implemented by switching threads in turn and allocating processor execution time, at any given moment , a processor (for a multi-core processor, a core) will only execute one thread. instructions in the thread . Therefore, in order to return to the correct execution position after thread switching, each thread needs to have an independent program counter . The counters between each thread do not affect each other and are stored independently. We call this type of memory area "thread private" of memory.
2. Java virtual machine stack
Like the program counter, the Java Virtual Machine Stack is also thread-private and its life cycle is the same as the thread . The virtual machine stack describes the thread memory model of Java method execution: when each method is executed, the Java virtual machine will simultaneously create a stack frame (Stack Frame) to store local variable tables, operand stacks, dynamic connections, and methods. export information . The process from each method being called until execution is completed corresponds to the process of a stack frame being pushed into the virtual machine stack and popped out of the stack.
Some people often divide the Java memory area into general heap memory (Heap) and stack memory (Stack). This division method is directly inherited from the memory layout structure of traditional C and C++ programs, and it seems a bit rough in the Java language. The actual division of memory areas is more complicated than this. However, the popularity of this division method also indirectly shows that the areas that programmers are most concerned about and most closely related to object memory allocation are the "heap" and "stack". "Stack" usually refers to the virtual machine stack mentioned here, or in more cases just refers to the local variable table part of the virtual machine stack .
The local variable table stores various Java virtual machine basic data types (boolean, byte, char, short, int, float, long, double) and object references (reference type) that are known during compilation. It is not equivalent to the object itself, and may It is a reference pointer pointing to the starting address of the object, or it may point to a handle representing the object or other location related to this object) and the returnAddress type (pointing to the address of a bytecode instruction).
The storage space of these data types in the local variable table is represented by local variable slots (Slots). The 64-bit long and double type data will occupy two variable slots, and the other data types will only occupy one . The memory space required for the local variable table is allocated during compilation . When entering a method, it is completely determined how much local variable space this method needs to allocate in the stack frame. The size of the local variable table will not change during the running of the method. . Please note that the "size" mentioned here refers to the number of variable slots and how much memory space the virtual machine actually uses (for example, one variable slot occupies 32 bits, 64 bits, or more) to implement a variable slot. , this is a matter entirely left to the discretion of the specific virtual machine implementation.
Supplement-- stack frame:
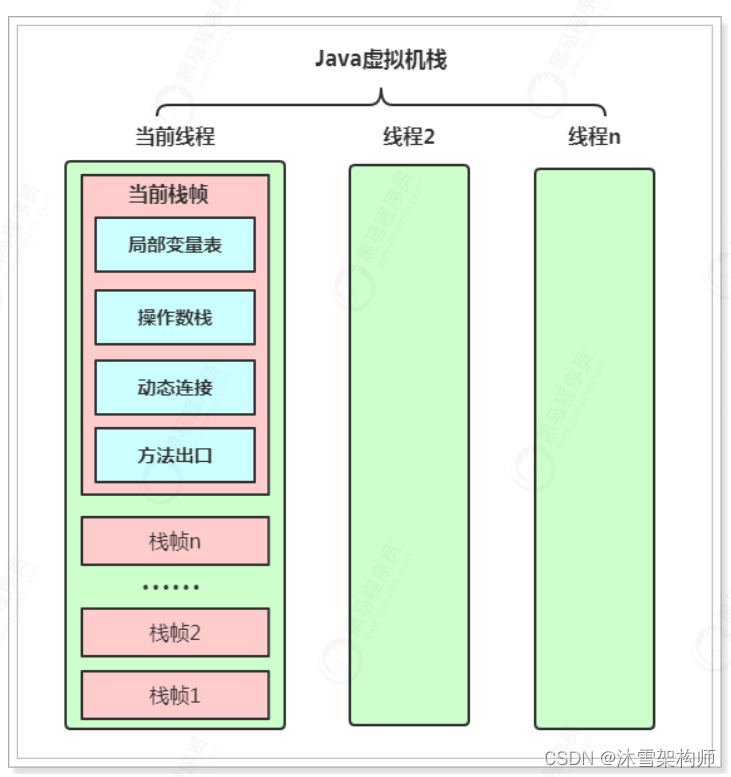
(1) The virtual machine stack is based on threads: even if you only have one main() method, it runs in a threaded manner. During the life cycle of a thread, the method stack frames participating in execution will be pushed and popped from the stack frequently. The life cycle of the virtual machine stack is the same as that of the thread.
(2) Stack size: The default size of each virtual machine stack is 1M.
(3) Stack overflow: The stack frame is deeply pushed onto the stack but not popped out, resulting in insufficient stack space and throwing java.lang.StackOverflowError. A typical one is recursive call.
(4) Composition of stack frame: Stack frame generally contains four areas: (local variable table, operand stack, dynamic connection, return address), as shown in the following figure:
(1), Local variable table: stores our local variables (variables within the method). First of all, it is a 32-bit length. It mainly stores the eight basic data types of our Java. Generally, 32 bits can be stored. If it is 64 bits, it can be stored by occupying two high and low bits. If it is a local variable, For an object, just store its reference address.
(2) Operand stack: It stores the operands executed by Java methods. It is also a stack. The elements of the operation can be any Java data type. When a method just starts, the operand stack is empty. The essence of the operand stack is The above is a workspace of the JVM execution engine. The operand stack will not be operated until the method is executed.
(3) Dynamic linking: Java language feature polymorphism
(4) Completion exit: normal return (call the address in the program counter as the return), in case of exception (determined by the exception handler table <in non-stack frame>)
3 Local method stack
The functions played by Native Method Stacks and virtual machine stacks are very similar. The difference is that the virtual machine stack serves the virtual machine to execute Java methods (that is, bytecode), while the native method stack serves the virtual machine. Native method services used by the machine.
The "Java Virtual Machine Specification" does not impose any mandatory requirements on the language, usage and data structure of methods in the local method stack. Therefore, specific virtual machines can freely implement it according to needs, and even some Java virtual machines (such as Hot-Spot Virtual machine) directly combines the local method stack and the virtual machine stack into one. Like the virtual machine stack, the local method stack will also throw StackOverflowError and OutOfMemoryError exceptions respectively when the stack depth overflows or the stack expansion fails.
4 Java heap
For Java applications, the Java heap (Java Heap) is the largest piece of memory managed by the virtual machine. The Java heap is a memory area shared by all threads and created when the virtual machine starts. The sole purpose of this memory area is to store object instances , and "almost" all object instances in the Java world allocate memory here.
The Java heap is a memory area managed by the garbage collector, so it is also called the "GC heap" (Garbage Collected Heap, fortunately not translated as "garbage heap") in some information.

5 method area
The Method Area, like the Java heap, is a memory area shared by each thread . It is used to store type information, constants, static variables, code cache compiled by the just-in-time compiler and other data that have been loaded by the virtual machine . Although the "Java Virtual Machine Specification" describes the method area as a logical part of the heap, it has an alias called "Non-Heap" to distinguish it from the Java heap.
5.1 Runtime constant pool
The Runtime Constant Pool is part of the method area . In addition to the description information of the class version, fields, methods, interfaces, etc., the Class file also has a constant pool table (Constant Pool Table), which is used to store various literals and symbol references generated during compilation . This part The content will be stored in the runtime constant pool in the method area after the class is loaded .
6 direct memory
Direct memory (Direct Memory) is not part of the virtual machine runtime data area, nor is it a memory area defined in the "Java Virtual Machine Specification". However, this part of memory is also frequently used, and may also cause OutOfMemoryError exceptions, so we will explain it here.
The allocation of local direct memory will not be limited by the size of the Java heap. However, since it is memory, it will definitely be limited by the size of the total local memory (including physical memory, SWAP partition or paging file) and the processor addressing space. When configuring virtual machine parameters, generally server administrators will set -Xmx and other parameter information based on actual memory, but often ignore direct memory, making the sum of each memory area greater than the physical memory limit (including physical and operating system-level limits) , resulting in an OutOfMemoryError exception during dynamic expansion.
Reference article: [3] Java virtual machine JVM bytecode instruction set bytecode operation code instruction classification usage mnemonic - Tencent Cloud Developer Community - Tencent Cloud