Today, I will make a complete summary of the hardware and programming of the serial communication of Puzhong Technology's single-chip microcomputer, record it and pick it up later if needed.
The circuit diagram of the serial port part of the microcontroller. A serial port communication circuit is integrated on the development board, which is a USB-to-serial port module, which can not only download programs but also realize serial port communication functions.
Briefly analyze the input and output of this module.
Describe the function of the CH340 chip. ( Take you to fully analyze the USB-to-serial chip CH340 - Findic.com )
The realized function is: the 51 single-chip computer communicates with the PC through the serial port (UART), and the serial port of the 51 single-chip computer receives the data sent by the PC and returns it to the PC for display.
The structure of serial port of single chip microcomputer
Serial related registers.
We generally choose the serial port to work in mode 1, and timer 1 to work in mode 2 (8-bit automatic reload).
Timer (Timer 1) vs. baud rate.
Introduction to SBUF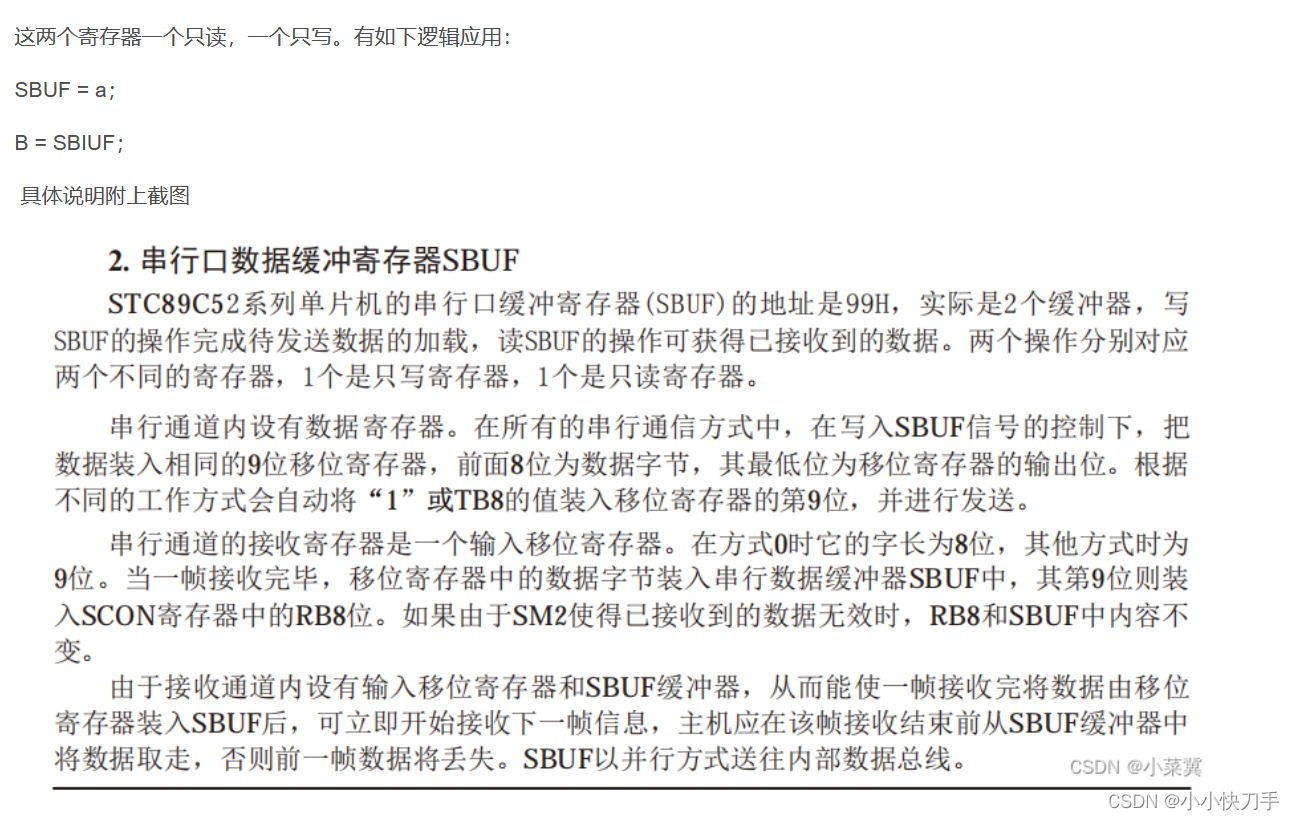
Serial port initialization.
void uart_init(u8 baud)
{
TMOD|=0X20; //设置计数器工作方式 2
SCON=0X50; //设置为工作方式 1
PCON=0X80; //波特率加倍
TH1=baud; //计数器初始值设置
TL1=baud;
ES=1; //打开串口中断
EA=1; //打开总中断
/*定时器1的方式2是8位自动重装,定时器一旦溢出,就自动重装,与中断无关,所以定时器1进入中断后无事可做,所以无需打开定时器1的中断*/
TR1=1; //打开计数器
}
void main()
{
uart_init(0XFA);//波特率为 9600
while(1)
{
}
}
void uart() interrupt 4 //串口通信中断函数
{
u8 rec_data;
RI = 0; //清除接收中断标志位
rec_data=SBUF; //存储接收到的数据
SBUF=rec_data; //将接收到的数据放入到发送寄存器
while(!TI); //等待发送数据完成
TI=0; //清除发送完成标志位
}
The complete program is as follows
#include "reg52.h"
typedef unsigned int u16; //对系统默认数据类型进行重定义
typedef unsigned char u8;
/*******************************************************************************
* 函 数 名 : uart_init
* 函数功能 : 串口通信中断配置函数,通过设置TH和TL即可确定定时时间
* 输 入 : baud:波特率对应的TH、TL装载值
* 输 出 : 无
*******************************************************************************/
void uart_init(u8 baud)
{
TMOD|=0X20; //设置计数器工作方式2
SCON=0X50; //设置为工作方式1
PCON=0X80; //波特率加倍
TH1=baud; //计数器初始值设置
TL1=baud;
ES=1; //打开接收中断
EA=1; //打开总中断
TR1=1; //打开计数器
}
/*******************************************************************************
* 函 数 名 : main
* 函数功能 : 主函数
* 输 入 : 无
* 输 出 : 无
*******************************************************************************/
void main()
{
uart_init(0XFA);//波特率为9600
while(1)
{
}
}
void uart() interrupt 4 //串口通信中断函数
{
u8 rec_data;
RI = 0; //清除接收中断标志位
rec_data=SBUF; //存储接收到的数据
SBUF=rec_data; //将接收到的数据放入到发送寄存器
while(!TI); //等待发送数据完成
TI=0; //清除发送完成标志位
}
Some serial code I saw on other blogs.
#include <REGX52.H>
/* **
* @brief 串口初始化 //[email protected]
* @param 无
* @retval 无
*/
void UART_Init(void) //[email protected]
{
PCON |= 0x80; //使能波特率倍速位SMOD
SCON = 0x50; //8位数据,可变波特率
TMOD &= 0x0F; //清除定时器1模式位
TMOD |= 0x20; //设定定时器1为8位自动重装方式
TL1 = 0xF4; //设定定时初值
TH1 = 0xF4; //设定定时器重装值
ET1 = 0; //禁止定时器1中断
TR1 = 1; //启动定时器1
EA = 1; //启动所有中断
ES = 1; //启动串口中断
}
/* * 串口发送模板
* @brief 串口发送一个字节数据
* @param Byte 要发送的一个数据
* @retval 无
*/
void UART_SendByte(unsigned char Byte)
{
SBUF = Byte;
while(TI == 0); //一执行完就要复位
TI = 0; //TI为发射控制器;RI为接受控制器;
}
/*串口中断函数模板
void UART_Routine() interrupt 4
{
if(RI = 1)
{
RI = 0;
}
}*/
The process of sending and receiving data by the serial port has nothing to do with the serial port interrupt. It does not mean that the serial port communication of the MCU is prohibited without opening the serial port interrupt. After sending the data, if you want to stop the serial communication at this time and perform other tasks such as data processing, no matter whether the interrupt is enabled or not, as long as the data is put into SBUF, the data will be sent out. The same is true for the timer, regardless of whether it is interrupted or not, the timer is always timing, but in some working modes, after timing once, the initial value will change when it is timed again (working mode 2 will be automatically reloaded).
When sending data through the serial port, you don’t need to consider the start bit and stop bit, and write the data into SBUF (there must be no start bit and stop bit), and the single-chip microcomputer will automatically pack the data when sending, adding start and stop bits.
Serial debugging tool
I just wrote a PC-side program to control the LED on and off of the single-chip microcomputer.
#include "reg52.h"
typedef unsigned int u16; //对系统默认数据类型进行重定义
typedef unsigned char u8;
sbit LED1=P2^0; //将P2.0管脚定义为LED1
/*******************************************************************************
* 函 数 名 : uart_init
* 函数功能 : 串口通信中断配置函数,通过设置TH和TL即可确定定时时间
* 输 入 : baud:波特率对应的TH、TL装载值
* 输 出 : 无
*******************************************************************************/
void uart_init(u8 baud)
{
TMOD|=0X20; //设置计数器工作方式2
SCON=0X50; //设置为工作方式1
PCON=0X80; //波特率加倍
TH1=baud; //计数器初始值设置
TL1=baud;
ES=1; //打开接收中断
EA=1; //打开总中断
TR1=1; //打开计数器
}
void delay_10us(u16 ten_us)
{
while(ten_us--);
}
/*******************************************************************************
* 函 数 名 : main
* 函数功能 : 主函数
* 输 入 : 无
* 输 出 : 无
*******************************************************************************/
void main()
{
uart_init(0XFA);//波特率为9600
while(1)
{
}
}
void send_Byte(u8 rec_data)
{
SBUF=rec_data; //将接收到的数据放入到发送寄存器
while(!TI); //等待发送数据完成
TI=0; //清除发送完成标志位
}
void uart() interrupt 4 //串口通信中断函数
{
if(RI)
{
u8 rec_data;
rec_data=SBUF; //存储接收到的数据
if(rec_data == 'G' || rec_data == 'g')
{
LED1=0; //点亮
delay_10us(50000); //大约延时450ms
LED1=1; //熄灭
delay_10us(50000);
}
send_Byte(rec_data);
RI = 0; //清除接收中断标志位
}
}
Introduction to printf redirection
char putchar(char c)
{
SendByte(c);
return c;
}
void SendByte(unsigned char data)
{
SBUF = data;
while(!TI);
TI = 0;
}
appendix: