A Simple Getting Started Demo for Hello World
①MainActivity code is as follows:
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
/**
* 步骤
* MainActivitt.java 代码中设置显示的内容视图 setContentView(R.layout.activity_main);
* activity_main.xml 中申明内容 tools:context=".MainActivity">
* AndroidManifest.xml 中文件注册申明名字 android:name=".MainActivity">
*
* 2021-5-7 13:55:15
*
*/
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
②The activity_main.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textColor="#FF0000"
android:textSize="30sp"
android:background="#00ff00"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:shadowColor="#F9F900"
android:shadowDx="10.0"
android:shadowDy="10.0"
android:shadowRadius="3.0"
android:text="带阴影的 Hello World!"
android:textColor="#9C27B0"
android:textSize="30sp"
tools:ignore="MissingConstraints"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
③AndroidManifest.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/Theme.MyApplication" >
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
The display page effect is as follows:
Example Demo01: Click the control switch to display the prompt
①The code of src/main/java/ MainActivity01.java is as follows:
package com.example.myapplication;
import android.os.Bundle;
import android.widget.CompoundButton;
import android.widget.Switch;
import android.widget.Toast;
import android.widget.ToggleButton;
import androidx.appcompat.app.AppCompatActivity;
/**
* 步骤:
* MainActivity01 代码中设置显示的布局内容视图 setContentView(R.layout.activity_main01);
* activity_main01.xml 中申明内容 tools:context=".MainActivity01">
* AndroidManifest.xml 中申明名字 android:name=".MainActivity01">
*
*
*/
public class MainActivity01 extends AppCompatActivity implements CompoundButton.OnCheckedChangeListener{
private ToggleButton tbtn_open;
private Switch swh_status;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main01);
tbtn_open = (ToggleButton) findViewById(R.id.tbtn_open);
swh_status = (Switch) findViewById(R.id.swh_status);
tbtn_open.setOnCheckedChangeListener(this);
swh_status.setOnCheckedChangeListener(this);
}
设置监听事件 toggleButton 是通过 checked 属性来控制开关
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
switch (compoundButton.getId()){
case R.id.tbtn_open:
if(compoundButton.isChecked()) Toast.makeText(this,"打开声音",Toast.LENGTH_SHORT).show();
else Toast.makeText(this,"关闭声音",Toast.LENGTH_SHORT).show();
break;
case R.id.swh_status:
if(compoundButton.isChecked()) Toast.makeText(this,"开关:ON",Toast.LENGTH_SHORT).show();
else Toast.makeText(this,"开关:OFF",Toast.LENGTH_SHORT).show();
break;
}
}
}
②The code of src /main/res/layout/ activity_main01.xml is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity01">
<!--开关-->
<ToggleButton
android:id="@+id/tbtn_open"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:layout_gravity="center"
android:textOff="关闭声音"
android:textOn="打开声音" />
<Switch
android:id="@+id/swh_status"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
/>
</LinearLayout>
③The code of src/main/AndroidManifest.xml is as follows:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/Theme.MyApplication" >
<activity
android:name=".MainActivity01">
<!-- 修改类名 android:name=".MainActivity02">-->
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
④ After the usb data cable is connected to the device, run it to demonstrate in the device. Click to display the following content:
Example Demo02: Click the button to display the prompt
①MainActivity02 code is as follows:
package com.example.myapplication;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
/**
* 点击按钮示例 步骤 参考示例: https://blog.csdn.net/a772304419/article/details/114752848
*
* 1、首先在layout布局创建 activity_text_view.xml 为应用添加一个id为hellotextView的textview和一个id为hellobutton的button,
* 2、代码和控件用到的字符串定义在 values 下的 string.xml 中声明显示文字信息
* 3、主程序中 MainActivity02 定义 button 点击后改变 textview 显示的文本,并且弹出 Toast 提示信息
* 4、AndroidManifest.xml 中申明主程序的类名
*
*/
public class MainActivity02 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_text_view);
//得到按钮实例
Button hellobtn = (Button)findViewById(R.id.hellobutton);
//设置监听按钮点击事件
hellobtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//得到textview实例
TextView hellotv = (TextView)findViewById(R.id.hellotextView);
//弹出Toast提示按钮被点击了
Toast.makeText(MainActivity02.this,"Clicked!你点击了该按钮!!!",Toast.LENGTH_SHORT).show();
//读取strings.xml定义的interact_message信息并写到textview上
hellotv.setText(R.string.interact_message);
}
});
}
}
②The code of src/main/res/values/strings.xml is as follows:
<resources>
<string name="app_name">My Application</string>
<string name="button_send">说点什么吧!</string>
<string name="default_message">点击下面的按钮!</string>
<string name="interact_message">你点击了该按钮!</string>
</resources>
③The activity_text_view.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/hellotextView"
android:layout_width="fill_parent"
android:layout_height="380dp"
android:gravity="center"
android:text="@string/default_message"
android:textColor="#FF9800" />
<Button
android:id="@+id/hellobutton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/button_send"
android:textColor="#FF0000" />
</LinearLayout>
④The AndroidManifest.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/Theme.MyApplication" >
<activity
android:name=".MainActivity02">
<!-- 修改类名 android:name=".MainActivity02">-->
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Example Demo03: RelativeLayout (relative layout) button remote control
The code of src/main/res/layout/day5241.xml is as follows:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/day524"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- 设置一个UI控件:文本框视图,定义标题上边距水平居中和文本字体大小加粗颜色-->
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center_horizontal"
android:layout_marginTop="20dp"
android:background="#00FFFF"
android:text="相对布局的实例"
android:textSize="35dp"
android:textStyle="bold"
android:textColor="#000000" />
<!-- 定位五个按钮,把按钮3排版好位于父类容器的中部为参考按钮-->
<Button
android:id="@+id/button_3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:textColor="#FF0000"
android:text="中间-3" />
<!-- 方法一:相对于控件布局,设置按钮1在按钮3的上边且在该控件的左边-->
<Button
android:id="@+id/button_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@id/button_3"
android:layout_toLeftOf="@id/button_3"
android:text="左上-1" />
<!-- 方法二:相对于父布局,设置按钮1对齐在整个布局上边且在该整个布局的左边-->
<!-- <Button-->
<!-- android:id="@+id/button_1"-->
<!-- android:layout_width="wrap_content"-->
<!-- android:layout_height="wrap_content"-->
<!-- android:layout_alignParentTop="true"-->
<!-- android:layout_alignParentLeft="true"-->
<!-- android:text="左上-1" />-->
<Button
android:id="@+id/button_2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@id/button_3"
android:layout_toRightOf="@id/button_3"
android:text="右上-2" />
<Button
android:id="@+id/button_4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/button_3"
android:layout_toLeftOf="@id/button_3"
android:text="左下-4" />
<Button
android:id="@+id/button_5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/button_3"
android:layout_toRightOf="@id/button_3"
android:text="右下-5" />
</RelativeLayout>
The page effect is as follows:
Example Demo04: TableLayout (table layout) button stretch
The code of src/main/res/layout/day5242.xml is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<!-- 定义一个单独按钮和一个表格布局内三按钮,指定第2列允许收缩,第3列允许拉伸 注都是从0开始!-->
<TableLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:shrinkColumns="1"
android:stretchColumns="2">
<!--单独的按钮 放在TableLayout里面占据一行-->
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button" />
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1按钮" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2被指定收缩" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="3被指定拉伸" />
</TableRow>
</TableLayout>
<!--
定义1个表格布局,指定第2列隐藏,collapseColumns为隐藏列从0开始,
如下定义collapseColumns="1" 表示为三个按钮隐藏第二个-->
<TableLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:collapseColumns="1">
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1后一个被隐藏" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2被指定隐藏按钮" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="3前一个被隐藏" />
</TableRow>
</TableLayout>
<!--定义第2个表格布局,指定第2列和第3列可以被拉伸-->
<TableLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:stretchColumns="1,2">
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1按钮" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2拉伸" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="3拉伸" />
</TableRow>
<TableRow
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1按钮" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2拉伸" />
</TableRow>
</TableLayout>
</LinearLayout>
The page effect is as follows:
Example Demo05: GridLayout (grid layout) button counter
The code of src/main/res/layout/day525.xml is as follows:
<?xml version="1.0" encoding="utf-8"?>
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:columnCount="4"
android:rowCount="6">
<!-- GridLayout 网格布局 设置多个按钮组件显示网格布局
columnCount="4":网格布局设置 4 列
rowCount="6":网格布局设置 6 行
android:layout_columnSpan="4":表示当前子控件占 4 列。
android:layout_marginLeft="5dp":设置控件内容距离左侧的距离
android:layout_gravity="fill":设置内容对齐方式为填充
-->
<TextView
android:layout_columnSpan="4"
android:layout_gravity="fill"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:layout_marginTop="5dp"
android:background="#FFCCCC"
android:text="0"
android:textSize="50sp" />
<Button
android:layout_columnSpan="2"
android:layout_gravity="fill"
android:text="回退" />
<Button
android:layout_columnSpan="2"
android:layout_gravity="fill"
android:text="清空" />
<Button
android:layout_gravity="fill"
android:text="+" />
<Button
android:layout_gravity="fill"
android:text="1" />
<Button
android:layout_gravity="fill"
android:text="2" />
<Button
android:layout_gravity="fill"
android:text="3" />
<Button
android:layout_gravity="fill"
android:text="-" />
<Button
android:layout_gravity="fill"
android:text="4" />
<Button
android:layout_gravity="fill"
android:text="5" />
<Button
android:layout_gravity="fill"
android:text="6" />
<Button
android:layout_gravity="fill"
android:text="*" />
<Button
android:layout_gravity="fill"
android:text="7" />
<Button
android:layout_gravity="fill"
android:text="8" />
<Button
android:layout_gravity="fill"
android:text="9" />
<Button
android:layout_gravity="fill"
android:text="/" />
<Button
android:layout_width="wrap_content"
android:layout_gravity="fill"
android:text="." />
<Button
android:layout_gravity="fill"
android:text="0" />
<Button
android:layout_gravity="fill"
android:text="=" />
</GridLayout>
The page effect is as follows:
Example Demo06: ConstraintLayout (constraint layout) click the button to switch pictures
①MainActivity0706 code is as follows:
package com.example.myapplication
import android.os.Bundle
import android.widget.Button
import android.widget.ImageView
import androidx.appcompat.app.AppCompatActivity
/**
* 点击按钮切换显示图片 示例:
*
* 1、首先在layout布局创建 day706_01.xml 为应用添加一个ImageView和一个button,
* 2、将显示的 ImageView 图片定义在 res/drawable 中修改图片文件名信息,如:image_1.jpg...
* 3、在主程序中 MainActivity0706 定义 一组图片的数组,并且一个按钮 点击切换查看数组图片
* 4、清单文件 AndroidManifest.xml 中申明主程序的类名
*
*/
class MainActivity0706 : AppCompatActivity() {
var iv: ImageView? = null
var btn: Button? = null
//声明图片资源数组
val imgs:Array<Int> = arrayOf(
R.drawable.a1,
R.drawable.a2,
R.drawable.a3,
R.drawable.a4,
R.drawable.a5,
R.drawable.a6,
R.drawable.a7,
R.drawable.a8,
R.drawable.a9,
R.drawable.a10,
R.drawable.a11,
R.drawable.a12,
R.drawable.a13,
R.drawable.image_1,
R.drawable.image_2
)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.day706_01)
var index:Int = 0
//找到控件
iv = findViewById(R.id.iv)
btn = findViewById(R.id.btn)
//设置图片点击监听器
btn?.setOnClickListener {
iv?.setImageResource(imgs[index++%imgs.size])
}
}
}
②The picture of src/main/res/drawable is as follows:
...The picture size format is generally 640×1136, as shown below, refer to picture a6.jpg
③day706_01.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/iv"
android:layout_width="match_parent"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHeight_percent="0.99"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="切换图片"
android:textColor="#00FF00"
app:layout_constraintBottom_toBottomOf="@+id/iv"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
④The AndroidManifest.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/Theme.MyApplication" >
<activity
android:name=".MainActivity0706">
<!-- 修改类名 android:name=".MainActivity02">-->
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Click the switch picture button page renderings are as follows:
Through this example, you will think of how to make it automatically switch pictures. The following example uses the RxJava timed task to set a group of pictures to automatically switch picture effect code examples in 2 seconds.
Example Demo07: ConstraintLayout (constraint layout) automatic switching of picture timing
①MainActivity0706 code is as follows:
package com.example.myapplication
import android.annotation.SuppressLint
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.example.myapplication.databinding.ActivityMainBinding
import io.reactivex.Observable
import io.reactivex.ObservableTransformer
import io.reactivex.android.schedulers.AndroidSchedulers
import io.reactivex.schedulers.Schedulers
import java.util.concurrent.TimeUnit
/**
*
* 点击按钮切换显示图片 示例:
*
* 1、首先在 layout 布局创建 activity_main.xml 为应用添加一个 ImageView 和一个 button,
* 2、将显示的 ImageView 图片定义在 res/drawable 中修改图片文件名信息,如:image_1.jpg...
* 3、在主程序中 MainActivity 定义 一组图片的数组,并且一个按钮 点击切换查看数组图片
* 4、清单文件 AndroidManifest.xml 中申明主程序的类名为 .MainActivity
*
* 注:
* 在 Activity 或 Dialog 中,加载界面后,通过 findViewById 方法,来给声明的控件赋值;
* 1、在没有加载之前,获取的控件为 null
* 2、控件多了, findViewById 特麻烦
* 解决方法:
* 1、使用 'kotlin-android-extensions' 插件
* 在 Build.gradle 中,添加插件;在代码中,直接使用控件的 id 来作为控件本身,此方法已被弃用;
* 2、ViewBinging 的使用
* 1-1、在 Build.gradle 中,添加字段 buildFeatures 为 true
* 在代码中①声明一个 binding var binding: ActivityMainBinding? = null
* ②初始化 binding binding = ActivityMainBinding.inflate(layoutInflater)
* ③设置布局 setContentView(binding!!.root)
*
* 1-2、在代码中通过 binding 来引用控件
* binding?.tvMsg?.text = "测试文本"
*
* 3、使用 RxJava 引包 implementation 'io.reactivex.rxjava2:rxandroid:2.1.1'
* 方法二:采用 RXJava 定时任务比 for 循环遍历更简单
*/
class MainActivity0706 : AppCompatActivity() {
//声明binding
var binding: ActivityMainBinding?= null
// var iv: ImageView? = null
// var btn: Button? = null
@SuppressLint("CheckResult")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
//初始化binding
binding = ActivityMainBinding.inflate(layoutInflater)
//设置布局界面
setContentView(binding?.root)
// setContentView(R.layout.activity_main)
//声明图片资源数组
val imgs:Array<Int> = arrayOf(
R.drawable.a1,
R.drawable.a2,
R.drawable.a3,
R.drawable.a4,
R.drawable.a5,
R.drawable.a6,
R.drawable.a7,
R.drawable.a8,
R.drawable.a9,
R.drawable.a10,
R.drawable.a11,
R.drawable.a12,
R.drawable.a13,
R.drawable.image_1,
R.drawable.image_2
)
//记录当前图片数组的索引
var index:Int = 0
//找到控件
// iv = findViewById(R.id.iv)
// btn = findViewById(R.id.btn)
//设置图片点击监听器
// btn?.setOnClickListener {
// iv?.setImageResource(imgs[index++%imgs.size])
// }
//设置控件及控件的事件
binding?.btn?.setOnClickListener {
//设置图片;每次点击时将 index向有移一位,
binding?.iv?.setImageResource(imgs[++index % imgs.size])
}
//方法一:for 循环 遍历
// for(i in 0 until 2000){
// //每隔2秒,切张图片
// binding?.iv?.setImageResource(imgs[++index % imgs.size])
// val observable = Observable.timer(2000L*i, TimeUnit.MILLISECONDS)
// .compose(ObservableTransformer<Any, Any> { upstream -> //线程调度
// upstream.subscribeOn(Schedulers.io())//在子线程中发生
// .observeOn(AndroidSchedulers.mainThread()) //在主线程中处理
//
// })
// .subscribe {
// //处理结果
// binding?.iv?.setImageResource(imgs[++index % imgs.size])
// }
// }
//方法二:采用RXJava 定时任务
Observable.interval(2000,TimeUnit.MILLISECONDS)
.compose(ObservableTransformer<Any,Any>{upstream ->
upstream.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
})
.subscribe {
binding?.iv?.setImageResource(imgs[++index % imgs.size])
}
}
}
②app/build.gradle configuration code is as follows:
plugins {
id 'com.android.application'
id 'kotlin-android'
id 'kotlin-android-extensions'
}
android {
compileSdkVersion 30
buildToolsVersion "30.0.3"
defaultConfig {
applicationId "com.example.myapplication"
minSdkVersion 16
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
//
buildFeatures {
viewBinding = true
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.1.0'
implementation 'com.google.android.material:material:1.1.0'
implementation 'androidx.constraintlayout:constraintlayout:1.1.3'
testImplementation 'junit:junit:4.+'
androidTestImplementation 'androidx.test.ext:junit:1.1.1'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0'
implementation "androidx.core:core-ktx:+"
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
//导入RXJava包
implementation 'io.reactivex.rxjava2:rxandroid:2.1.1'
}
repositories {
mavenCentral()
}
In Build.gradle:
add pluginapply plugin: ‘com.android.application’
apply plugin: ‘kotlin-android’
apply plugin: ‘kotlin-android-extensions’Add bound view
buildFeatures {
viewBinding = true
}Import the RxJava package
implementation ‘io.reactivex.rxjava2:rxandroid:2.1.1’
After synchronization, the running page effect will automatically switch pictures in 2s
Example Demo08: LinearLayout (linear layout) slot machine definition picture rotation random selection of a picture
①MainActivity0722 code is as follows:
package com.example.myapplication
import android.annotation.SuppressLint
import android.os.Bundle
import android.widget.ImageView
import androidx.appcompat.app.AppCompatActivity
import com.example.myapplication.databinding.Day719Binding
import io.reactivex.Observable
import io.reactivex.ObservableTransformer
import io.reactivex.Scheduler
import io.reactivex.android.schedulers.AndroidSchedulers
import io.reactivex.disposables.Disposable
import io.reactivex.schedulers.Schedulers
import java.util.concurrent.TimeUnit
/**
* 2021-7-22 11:39:43
* 老虎机实例:
* 定义八张图片旋转随机停止落选某张图片
*
*/
class MainActivity0722 : AppCompatActivity() {
//Day722Binding 命名为布局名组合+绑定; 声明的是布局文件不为空
var binding : Day0722Binding? = null
//声明图片控件数组
val imgs: ArrayList<ImageView?> = ArrayList()
//
var observable : Disposable? = null
//onCreate方法用来初始化Activity实例对象
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
//layoutInflater 布局增压泵;对于一个没有被载入或者想要动态载入的界面,
// 都需要使用inflate来载入.之后需定义声明布局文件不为空
binding = Day722Binding.inflate(layoutInflater)
//加载布局文件方法
// setContentView(R.layout.main);
setContentView(binding?.root)
//设置图片控件大小 之后需定义声明图片控件数组
imgs.add(binding?.iv1)
imgs.add(binding?.iv2)
imgs.add(binding?.iv3)
imgs.add(binding?.iv4)
imgs.add(binding?.iv5)
imgs.add(binding?.iv6)
imgs.add(binding?.iv7)
imgs.add(binding?.iv8)
//计算屏宽,得到iv宽高
val ivSize = resources.displayMetrics.widthPixels / 3
//遍历控件设置大小
for (item in imgs) {
//先拿回原本的布局属性
var params = item?.layoutParams
//再修改其长宽高 布局属性 3×3 ,之后需定义计算屏宽,得到iv 宽高
params?.width = ivSize
params?.height = ivSize
//最后修改后的属性设置回去
item?.layoutParams = params
}
//ivCenter 设置图片中间设置大小
var params = binding?.ivCenter?.layoutParams
//设置中间的宽高
params?.width = ivSize
params?.height = ivSize
//重设回来
binding?.ivCenter?.layoutParams = params
//点击按钮触发事件,为 Button 注册监听器
binding?.btnStart?.setOnClickListener {
//先生成一个随机停止的落选数
val runNumber = (Math.random()*100).toInt()
//启动定时器,设置每隔一秒自动切换一张图片
// var index = 1L
var index = 1
//利用RxJava 之后需先定义 observable
/* observable = Observable.interval(index*100, TimeUnit.MILLISECONDS)
.compose(ObservableTransformer<Any,Any> { upstream ->
upstream.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
})
.subscribe {
//控制 src 属性, 之后需先定义设置图片的显示位置设置
setImgSrc((index ++ %imgs.size).toInt())
observable?.dispose()
} */
setDelayDisplay(index*10,index,runNumber)
}
}
//延迟显示指定的位置,delay:延迟时间;Index:在哪个控件上显示
@SuppressLint("CheckResult")
fun setDelayDisplay(delay: Int, index: Int, stopIndex: Int) {
Observable.timer(delay.toLong(), TimeUnit.MILLISECONDS)
.compose(ObservableTransformer<Any,Any> {
upstream ->
upstream.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
})
.subscribe {
setImgSrc((index%imgs.size).toInt())
//再次延迟操作
if (index < stopIndex) {
val nextIndex = index+1
setDelayDisplay(nextIndex*10,nextIndex,stopIndex)
}
}
}
//设置图片的src 且只显示一张,其它都不显示
fun setImgSrc(index : Int) {
//计算图片总个数
val totalCount = imgs.size
//遍历循环
for (i in 0 until totalCount) {
//
if (index == i) {
imgs[i]?.setImageResource(R.drawable.img_selected)
} else {
imgs[i]?.setImageResource(0)
}
}
}
}
②The picture of src/main/res/drawable is as follows:
...The picture size format is generally 147×148, as shown below, refer to picture b1.jpg
③day722_01.xml code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity0722 ">
<!-- 图片 -->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:layout_weight="1">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/iv1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b1"
android:padding="10dp"
android:src="@drawable/img_selected"/>
<ImageView
android:id="@+id/iv2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b2"
android:padding="10dp" />
<ImageView
android:id="@+id/iv3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b3"
android:padding="10dp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/iv8"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b1"
android:padding="10dp" />
<ImageView
android:id="@+id/ivCenter"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b1"
android:padding="10dp"
android:visibility="invisible"/>
<ImageView
android:id="@+id/iv4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b2"
android:padding="10dp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/iv7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b3"
android:padding="10dp" />
<ImageView
android:id="@+id/iv6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b2"
android:padding="10dp" />
<ImageView
android:id="@+id/iv5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/b1"
android:padding="10dp" />
</LinearLayout>
</LinearLayout>
<Button
android:id="@+id/btnStart"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="开始" />
</LinearLayout>
④The code of src/main/res/drawable/img_selected.xml is as follows:
From fast to slow, the transparent effect of the rotation shows the mark box
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<stroke
android:width="15dp"
android:color="#8FFF" />
</shape>
⑤ The app/build.gradle configuration code is as follows:
plugins {
id 'com.android.application'
id 'kotlin-android'
id 'kotlin-android-extensions'
}
android {
compileSdkVersion 30
buildToolsVersion "30.0.3"
defaultConfig {
applicationId "com.example.myapplication"
minSdkVersion 16
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
buildFeatures {
viewBinding = true
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.1.0'
implementation 'com.google.android.material:material:1.1.0'
implementation 'androidx.constraintlayout:constraintlayout:1.1.3'
testImplementation 'junit:junit:4.+'
androidTestImplementation 'androidx.test.ext:junit:1.1.1'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0'
implementation "androidx.core:core-ktx:+"
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
//导入RXJava包
implementation 'io.reactivex.rxjava2:rxandroid:2.1.1'
}
repositories {
mavenCentral()
}
Click the start button and the page will randomly stop to a certain picture according to the rotation from fast to slow. The effect is as follows:
-
RxJava [findViewById] link:
Link: https://pan.baidu.com/s/1ASWWozcc6DDIFKLYzaVRMQ
Extraction code: 49ew
Note:
Likes, comments, and reprints are welcome. Please give the link to the original text in an obvious place on the article page
. Those who know, thank you for reading my article in the vast crowd.
Where is the signature without personality!
For details, please follow me
and continue to update
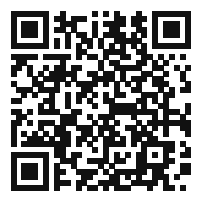
© 2021 05 - Guyu.com | 【Copyright All Rights Reserved】 |