Some commonly used UI controls in Android are:
TextView (text box),
EditView (input box),
Button (button),
RadioButton (radio button),
ImageView (image view),
ToggleButton (switch button), etc.
1. TextView (text box)
Some property descriptions in TextView:
The layout layout code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/TextView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="这是 TextView(文本框)"
android:textColor="#FF0000"
android:textStyle="bold|italic"
android:textSize="30sp"
android:layout_gravity="center"
android:background="#00FF00"/>
</LinearLayout>
The page effect is as follows:
- In addition, if you want the text box and font to be centered,
the layout layout code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:background="#00FFFF">
<TextView
android:id="@+id/TextView1"
android:layout_width="200dp"
android:layout_height="200dp"
android:text="这是 TextView(文本框)"
android:textColor="#FF0000"
android:textStyle="bold|italic"
android:textSize="19sp"
android:gravity="center"
android:background="#00FF00"/>
</LinearLayout>
The page effect is as follows:
①TextView property with shadow
②TextView with border
Several nodes and attribute descriptions of the shapeDrawable resource file:
③TextView with pictures (drawableXxx)
Basic usage:
The core of setting pictures is actually: drawableXxx; You can set pictures in four directions:drawableTop(top),
drawableButton(bottom),
drawableLeft(left),
drawableRight(right)In addition, you can also use drawablePadding to set the spacing between pictures and text!
④ Use the autoLink attribute to identify the link type
When there are URLs, E-Mails, phone numbers, and maps in the text, we can set the autoLink attribute; when we click on the corresponding part of the text, we can jump to a default APP, such as a string of numbers, click Then jump to the dial interface and so on!
⑤ TextView uses HTML:
Commonly used tags:
2. EditText (input box)
It is very similar to TextView (text box), the biggest difference is: EditText can accept user editing input! EditText inherits from TextView, can edit and pass user information to Android program. You can also set a listener for the EditText control to test whether the content entered by the user is legal and so on.
hint Set the default prompt text. After editing the input text content, the prompt content disappears. After
getting the focus, select all the text content in the component.
Limit the input type.
Set the minimum line, maximum line, single line, multi-line, automatic line wrap...
The layout layout code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="30dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="账号:"
android:textSize="50sp"
android:textColor="#FF0000"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入账号"
android:textSize="30sp"
android:maxLines="2"/>
</LinearLayout>
The page effect is as follows:
3. Button and ImageButton (image button):
StateListDrawable is a kind of Drawable resource, which can set different image effects according to different states. The key node < selector >, we only need to set the background property of Button to this drawable resource to realize it easily. When the button is pressed, different Button color or background!
Properties set:
4. ImageView (image view):
scaleType sets the scale type value:
- Specify the image android:src="@drawable/image_1" in the layout
5. RadioButton (radio button) & Checkbox (check box):
RadioButton method to obtain relevant information;
RadioGroup is a radio combo box, which is composed of multiple radio buttons RadioButton to realize the radio state. A RadioButton (radio button) needs to be used in conjunction with a RadioGroup to provide two or more mutually exclusive sets of options.
The layout layout code is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center">
<LinearLayout
android:id="@+id/gender_line"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginRight="15dp"
android:layout_marginLeft="15dp"
android:layout_marginTop="10dp"
android:layout_marginBottom="10dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="性 别:"
android:textSize="14sp">
</TextView>
<RadioGroup
android:id="@+id/gender"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="男"
android:textSize="14sp"
android:layout_marginLeft="30dp"
android:layout_marginRight="20dp">
</RadioButton>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="女"
android:textSize="14sp"
android:layout_marginLeft="30dp"
android:layout_marginRight="20dp">
</RadioButton>
</RadioGroup>
</LinearLayout>
<LinearLayout
android:id="@+id/likes_line"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginRight="15dp"
android:layout_marginLeft="15dp"
android:layout_marginTop="10dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="喜 好:"
android:textSize="14sp">
</TextView>
<CheckBox
android:id="@+id/play_game"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="敲代码"
android:textSize="14sp"
android:layout_marginLeft="30dp"
android:layout_marginRight="20dp"
android:checked="true">
</CheckBox>
<CheckBox
android:id="@+id/play_balls"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="打球"
android:textSize="14sp"
android:layout_marginLeft="10dp"
android:layout_marginRight="20dp">
</CheckBox>
<CheckBox
android:id="@+id/play_man"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="打人"
android:textSize="14sp"
android:layout_marginLeft="10dp">
</CheckBox>
</LinearLayout>
</LinearLayout>
The page effect is as follows:
6. ToggleButton (switch button) & Switch (switch):
①Switch button related attributes:
②Switch related properties:
Note:
Likes, comments, and reprints are welcome. Please give the link to the original text in an obvious place on the article page
. Those who know, thank you for reading my article in the vast crowd.
Where is the signature without personality!
For details, please follow me
and continue to update
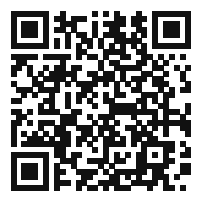
© 2021 05 - Guyu.com | 【Copyright All Rights Reserved】 |