1. Create a new project
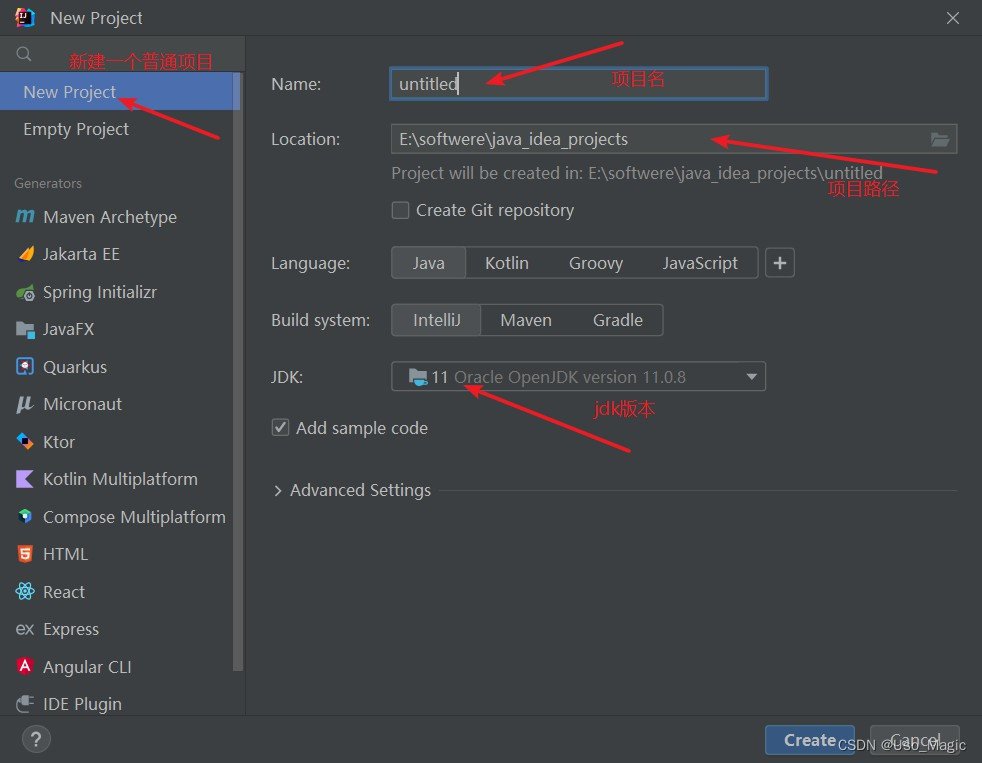
click create
2. Add web project framework
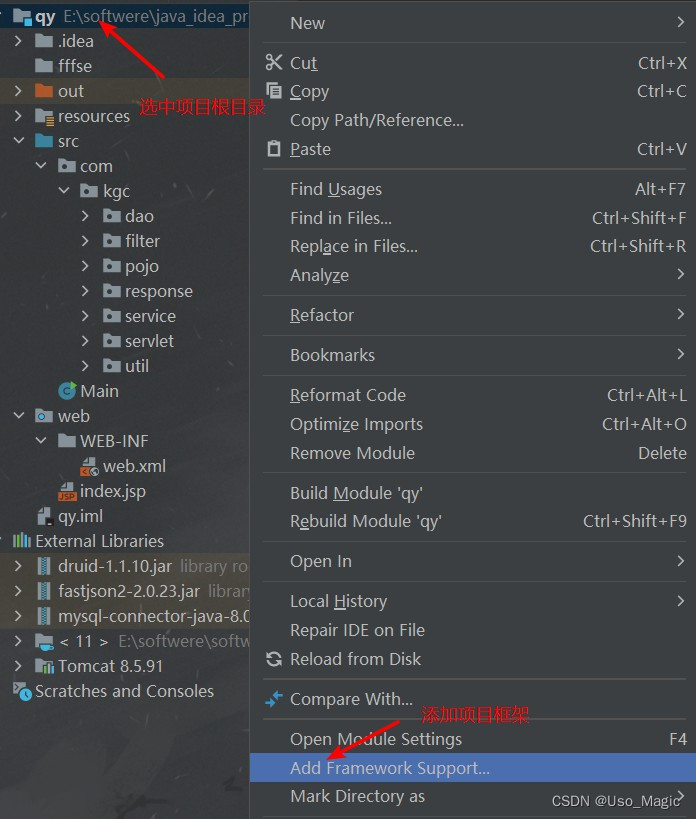
3. Select the web framework
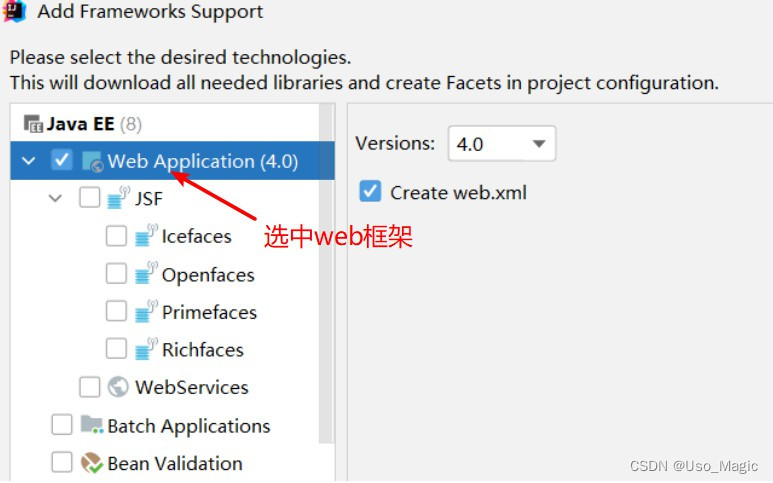
click ok
4. Add tomcat
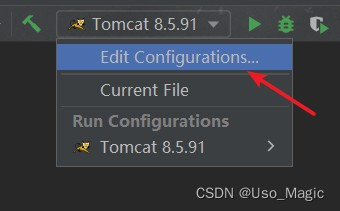
5. Select tomcat
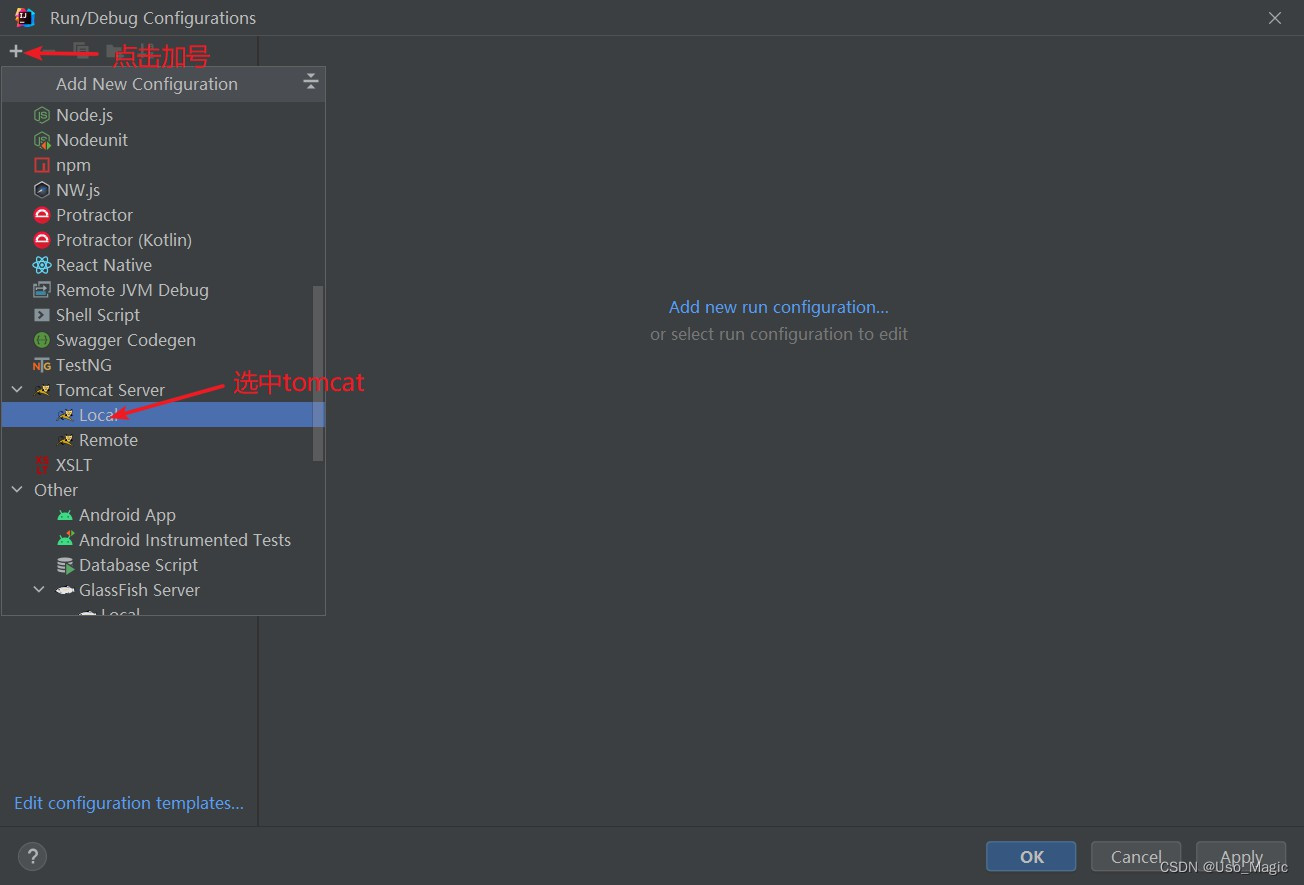
Click apply and click ok
6. Configure tomcat path and related settings
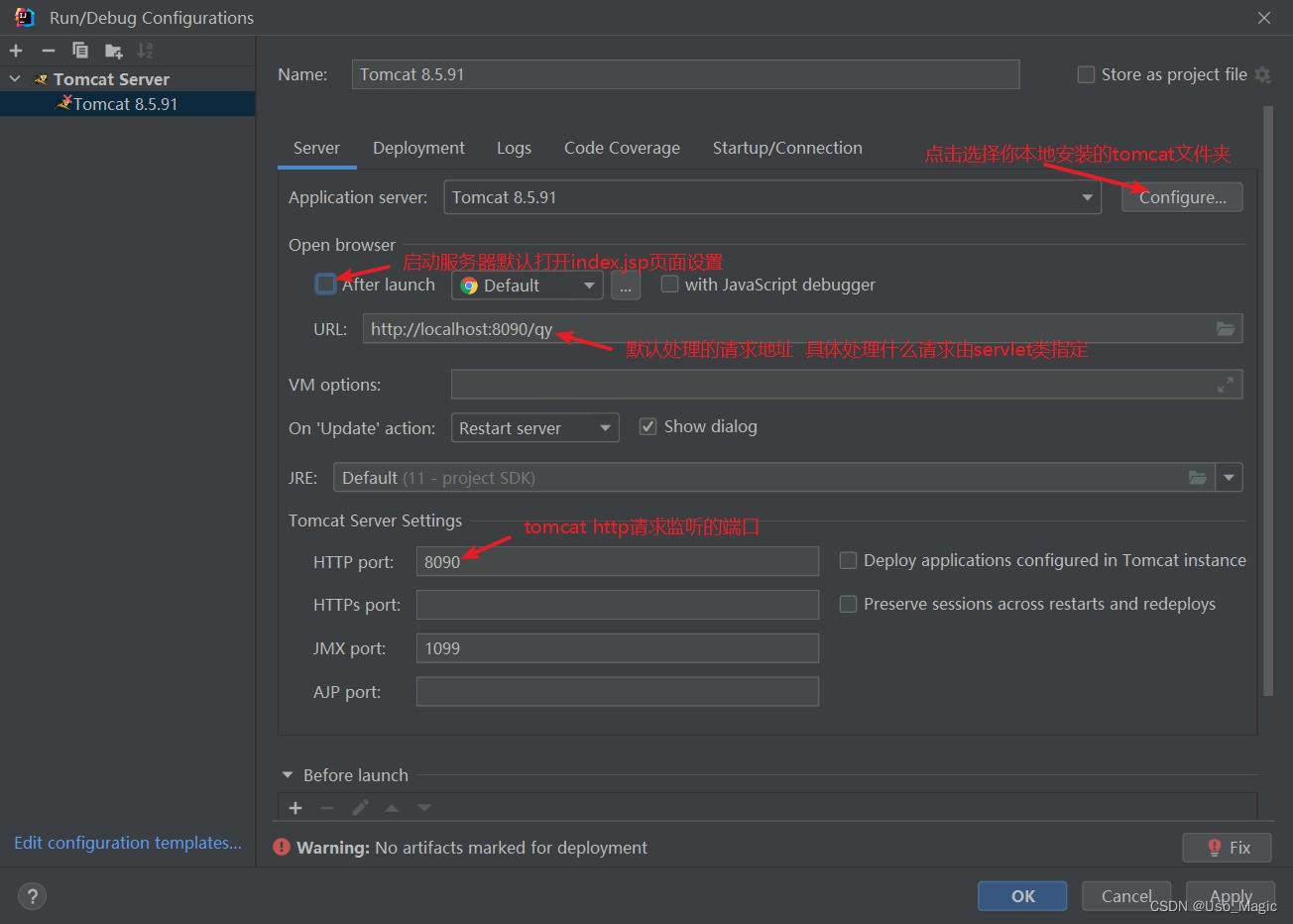
Click apply and click ok
7. Deploy the war package of the web project to tomcat
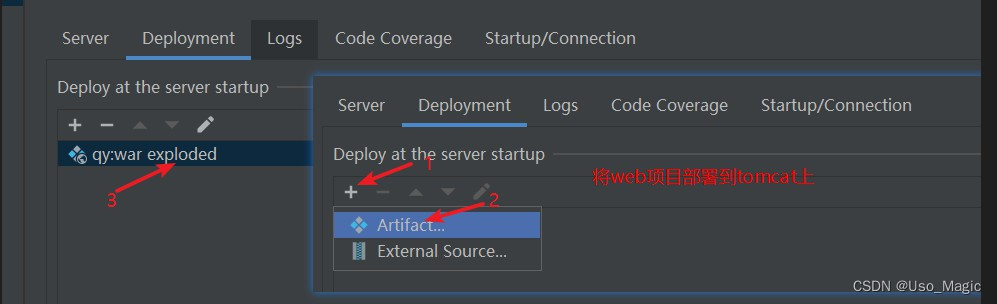
8. Make sure processing requests are consistent
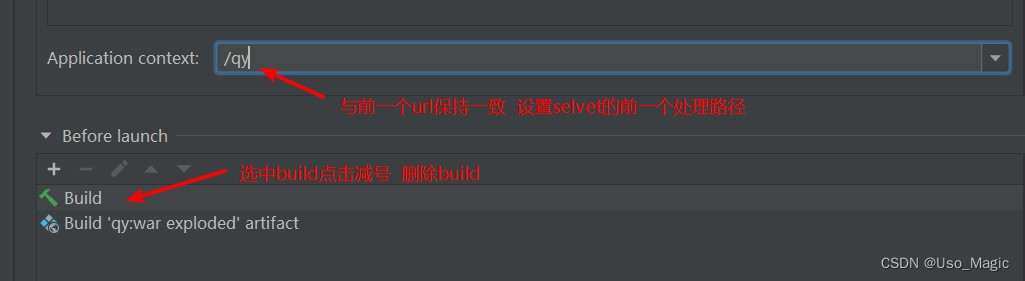
Click apply and click ok
9. Add project dependencies
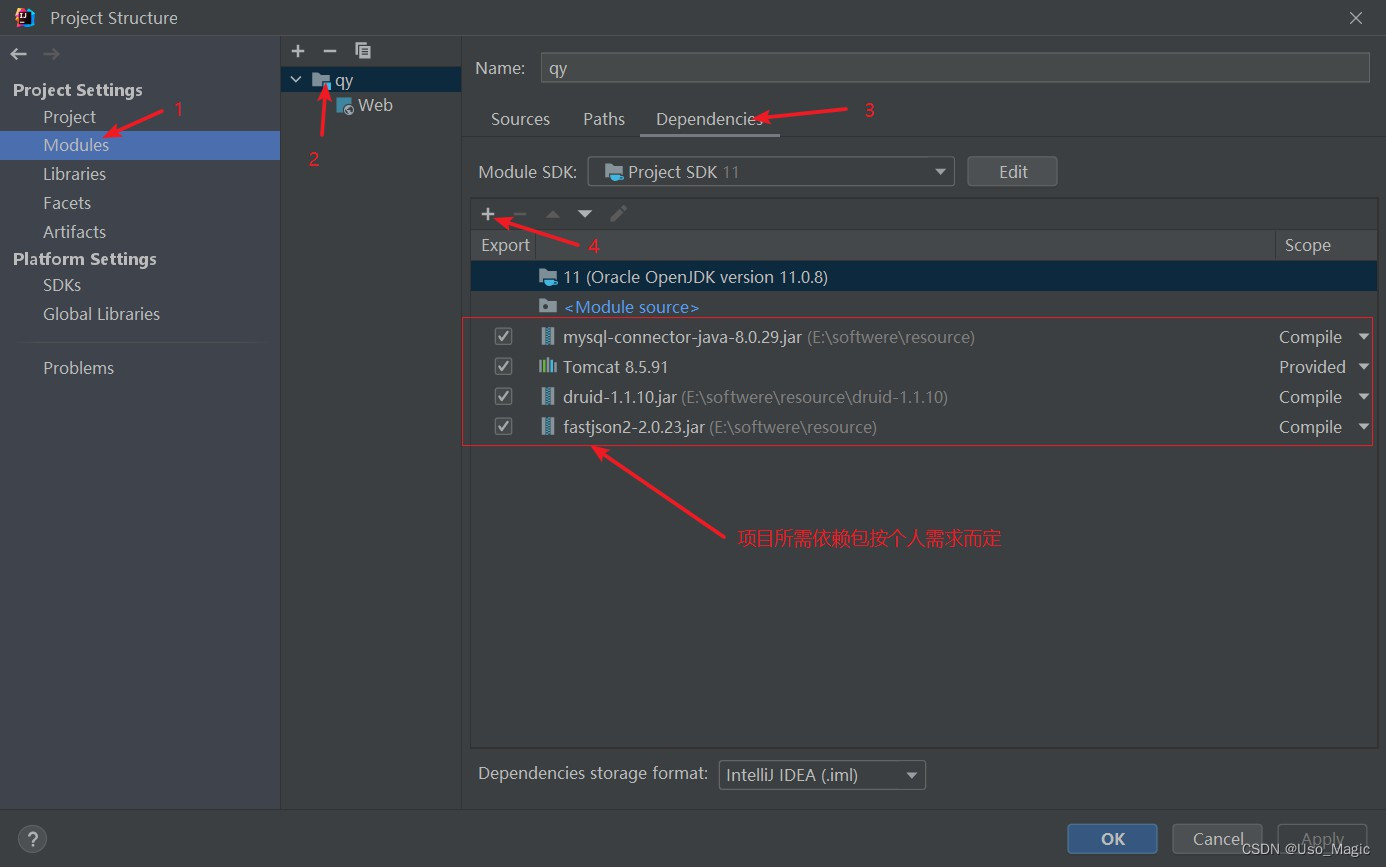
Click apply and click ok
10. Check whether the dependencies are added to the war package
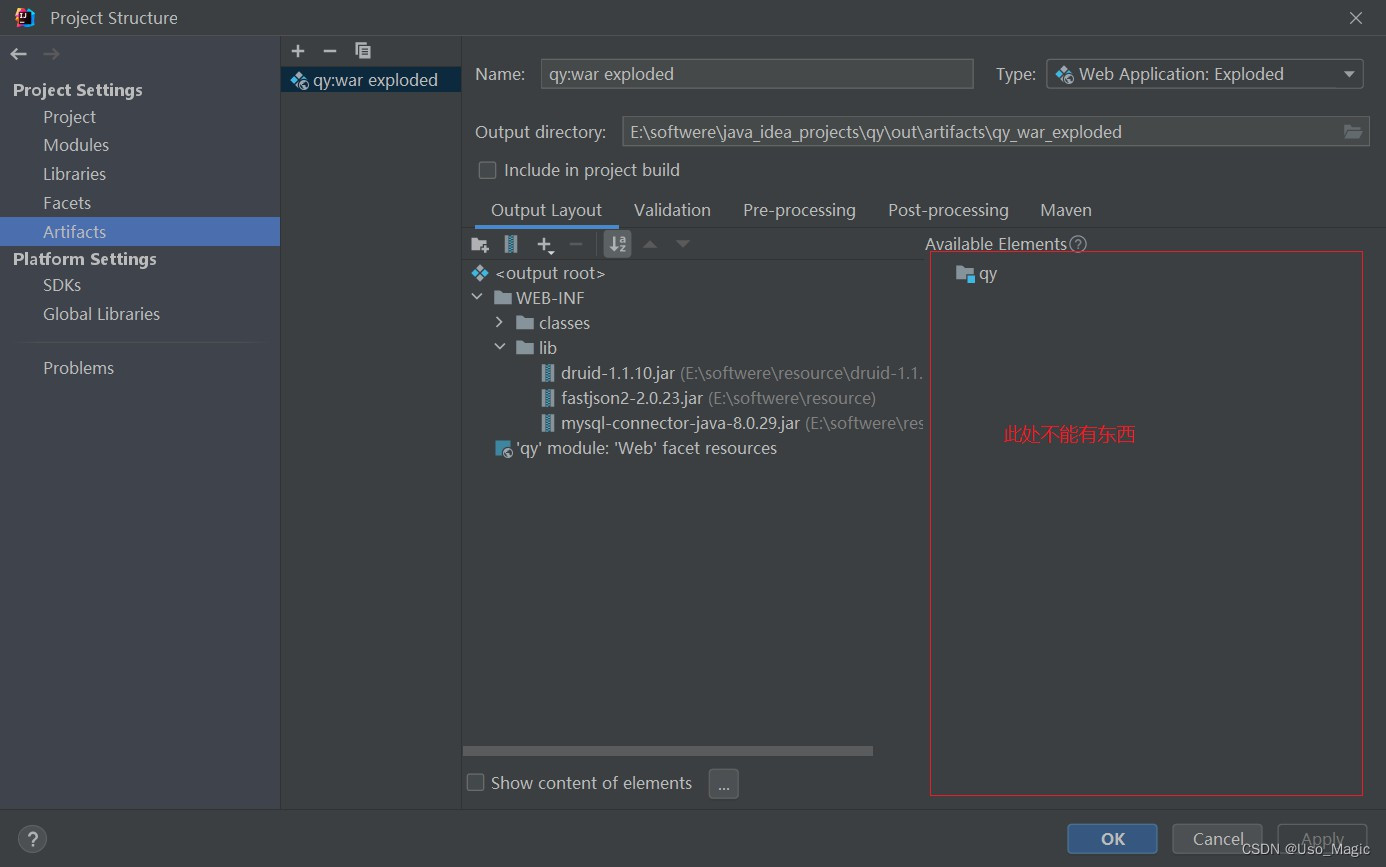
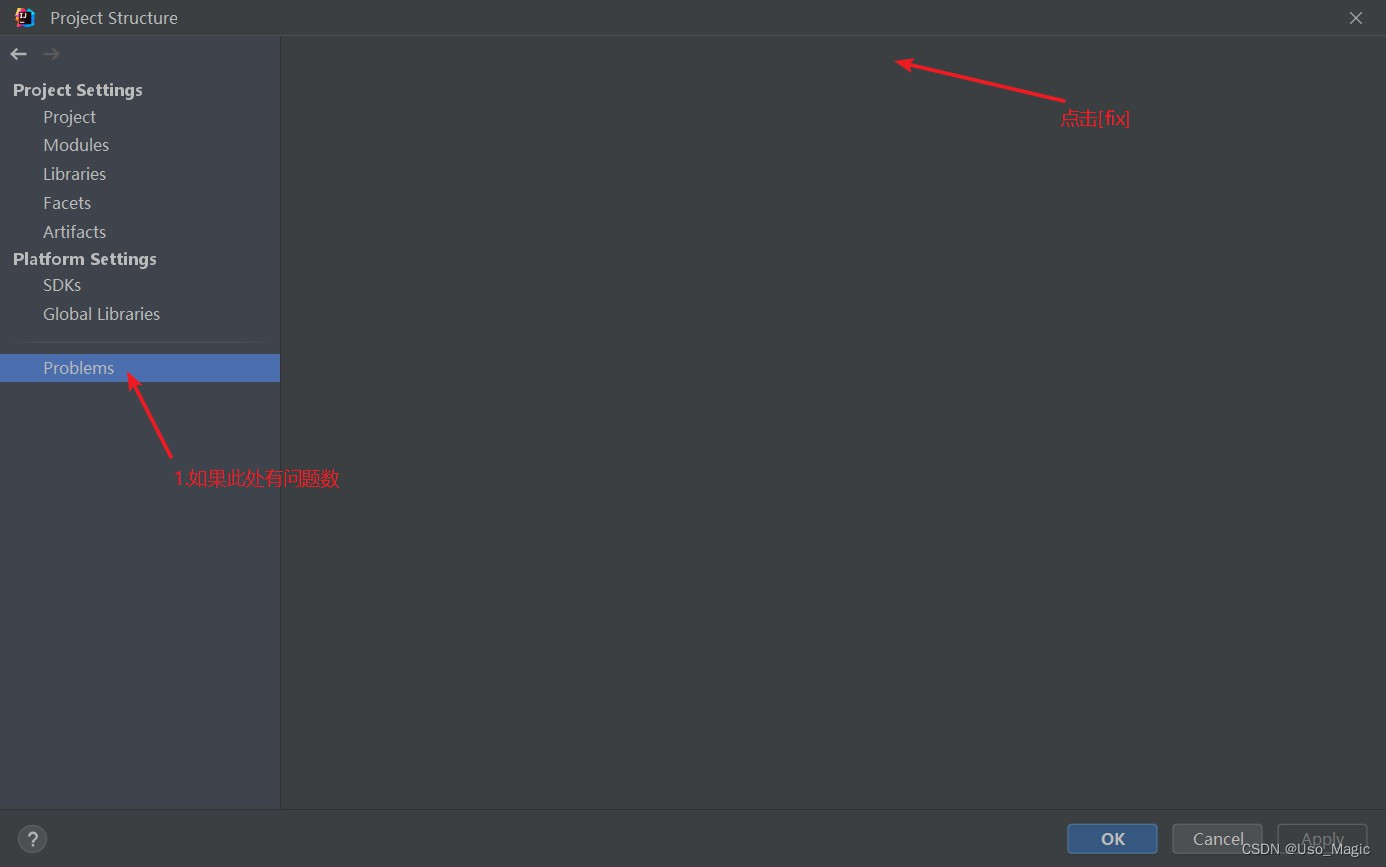
Such a basic web project configuration is complete
Set the basic file hierarchy of the project
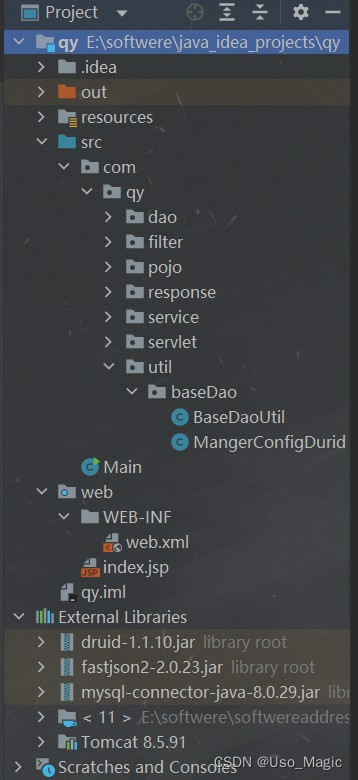
Folder tagging settings for configuration files
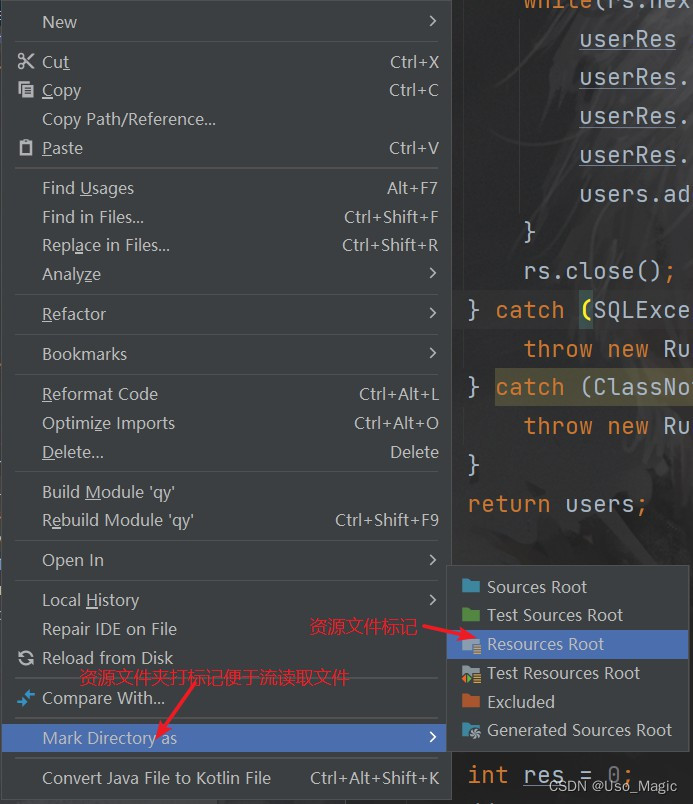
Text encoding filter settings
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<filter>
<filter-name>EncodingFilter</filter-name>
<filter-class>com.kgc.filter.EncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>htmlShow</param-name>
<param-value>text/html;charset=utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>EncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
package com.qy.filter;
import javax.servlet.*;
import java.io.IOException;
/**
* @author Magic
* @version 1.0
*/
public class EncodingFilter implements Filter {
// 获取web.xml文件中的配置utf-8
private String encoding;
private String htmlShow;
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// 从web.xml的过滤器的init-param中,对应的值,在赋值给全局变量
encoding = filterConfig.getInitParameter("encoding");
htmlShow =filterConfig.getInitParameter("htmlShow");
}
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
// 设置编码;
servletRequest.setCharacterEncoding(encoding);
servletResponse.setCharacterEncoding(encoding);
servletResponse.setContentType(htmlShow);
// 放行
filterChain.doFilter(servletRequest, servletResponse);
}
@Override
public void destroy() {
}
}