<span style="font-family: Arial, Helvetica, sans-serif; background-color: rgb(255, 255, 255);">作为一名程序员,要不断的学习新的技术。不断汲取新的知识。</span>
CoordinatorLayout coordinate bureauAppBarLayout is used to replace the early actionbar
CollapsingToolbarLa yout collapsible toolbarToolbar title bar
Write a simple collapsible title bar through the above components. Look at the three pictures first.
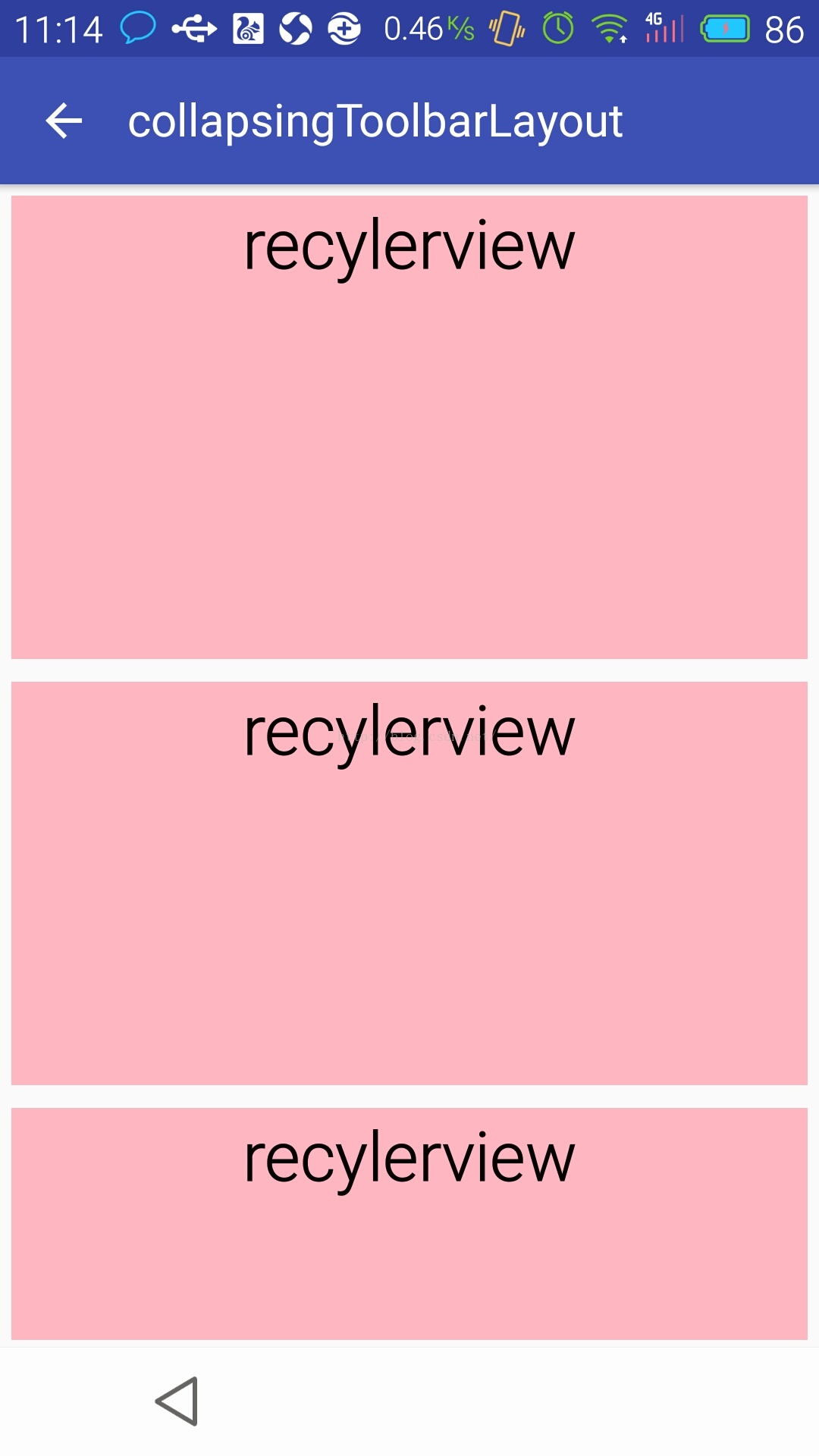
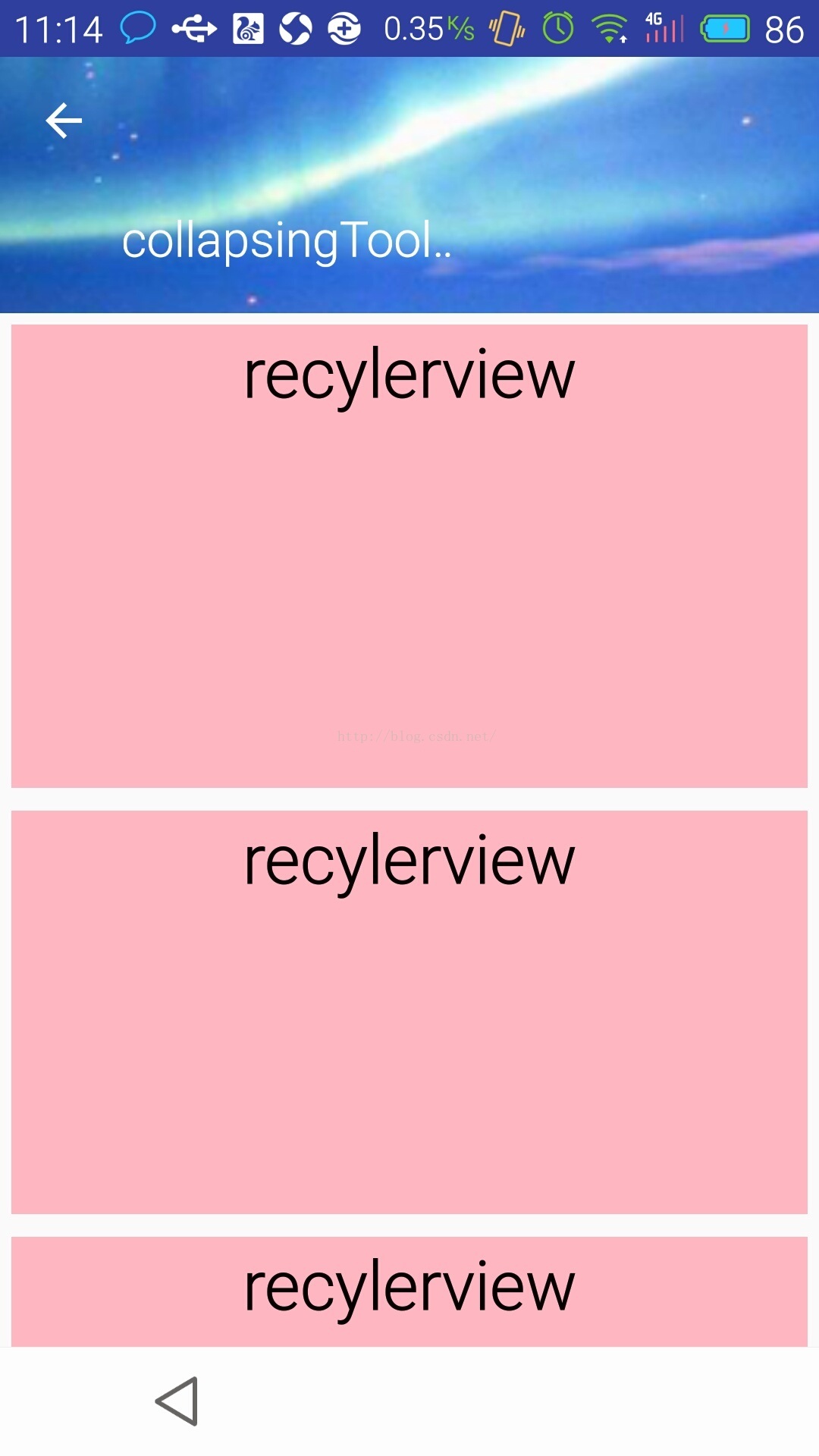
As can be seen from these three pictures, the size of the title bar will expand and collapse as the recyclerview slides
The following is a brief description of how to achieve
First upload the layout file
<?xml version="1.0" encoding="utf-8"?>
<!--做出一个可折叠的toolbar 必须做到以下三点
1,coordiantorlayout 作为父容器的布局
2,给需要滑动的组件设置 layout_behavior
3,collapsingtoolbarlayout 包含一个imageview 和 toolbar
-->
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
>
<!--appbar layout 新的android appbarlayout-->
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="256dp"
android:fitsSystemWindows="true"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
>
<!--包含一个可折叠的toolbarlayout
layout_scrollFlags
scroll 若想让toolbar 滚动就必须设置这个属性
enterAlways 向下移动时 立即显示view
exitUntilCollapsed 向上移动时 收缩view 但可以固定toolbar 在上面
enterAlwaysCollapsed 当你的View已经设置minHeight属性又使用此标志时,你的View只能以最小高度进入,只有当滚动视图到达顶部时才扩大到完整高度
-->
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/collap_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:contentScrim="?attr/colorPrimary"
app:expandedTitleMarginEnd="64dp"
app:expandedTitleMarginStart="48dp"
app:layout_scrollFlags="scroll|exitUntilCollapsed"
>
<!--layout_collapseMode 1,pin 没有视觉差! 2,parallax 有视觉差 可以配合layout_collapseParallaxMultiplier 视觉差因子 设置 val 0-1-->
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"
android:src="@drawable/toolbar"
app:layout_collapseParallaxMultiplier="0.7"
android:fitsSystemWindows="true"
app:layout_collapseMode="parallax"
/>
<!--toolbar-->
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:layout_collapseMode="pin"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light"
></android.support.v7.widget.Toolbar>
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<!--配合一个可滚动的视图-->
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
android:scrollbars="none" />
</android.support.design.widget.CoordinatorLayout>
布局文件也没什么好说的了 里面都有注释 。
直接上代码:
<pre name="code" class="java">package com.lefeng.lfvideo.myndk;
import android.os.Bundle;
import android.support.design.widget.CollapsingToolbarLayout;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.support.v7.widget.Toolbar;
import com.lefeng.lfvideo.myndk.adapter.RecylerAdapter;
import java.util.ArrayList;
import java.util.List;
/**
* Created by CYL on 2016/3/23.
* email:[email protected]
*/
public class CollapingActivity extends AppCompatActivity {
private Toolbar toolbar;
private CollapsingToolbarLayout collapsingToolbarLayout;
private RecyclerView recyclerView;
private List<String> dataSource;
private RecylerAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.applayout);
toolbar = (Toolbar) findViewById(R.id.toolbar);
collapsingToolbarLayout = (CollapsingToolbarLayout) findViewById(R.id.collap_layout);
recyclerView = (RecyclerView) findViewById(R.id.recyclerView);
<span style="white-space:pre"> </span>//这个主意下 这个activity的theme 设置为noactionbar
<span style="white-space:pre"> </span>//设置actionbar 为toolbar
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
<span style="white-space:pre"> </span>//这里设置标题的时候需要在collapsingtoolbarlayout上设置 否则没有效果
collapsingToolbarLayout.setTitle("collapsingToolbarLayout");
initData();
adapter = new RecylerAdapter(dataSource,getApplicationContext());
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(adapter);
}
private void initData(){
dataSource = new ArrayList<>();
for (int i = 0;i<100;i++){
dataSource.add("recylerview");
}
}
}
There is also an adapter for recylerview posted. It is actually very simple and similar to the adapter for listview.
package com.lefeng.lfvideo.myndk.adapter;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.lefeng.lfvideo.myndk.R;
import com.lefeng.lfvideo.myndk.utils.Sp2Px;
import java.util.List;
/**
* Created by CYL on 2016/3/22.
* email:[email protected]
*/
public class RecylerAdapter extends RecyclerView.Adapter<RecylerAdapter.MyViewHolder> {
private List<String> dataSource;
private LayoutInflater mInflater;
private Context context;
public RecylerAdapter(List<String> dataSource,Context context) {
this.dataSource = dataSource;
mInflater = LayoutInflater.from(context);
this.context = context;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
MyViewHolder viewHolder = new MyViewHolder(mInflater.inflate(R.layout.recycler_item,parent,false));
return viewHolder;
}
@Override
public void onBindViewHolder(MyViewHolder holder, final int position) {
ViewGroup.LayoutParams params = holder.container.getLayoutParams();
params.height = (int) Sp2Px.sp2px(mInflater.getContext(), (float) (100+(150*Math.random())));
holder.container.setLayoutParams(params);
holder.tv.setText(dataSource.get(position));
holder.tv.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(position%2==0){
removeItem(position);
}
else{
addItem();
}
}
});
}
public final class MyViewHolder extends RecyclerView.ViewHolder{
private TextView tv ;
private LinearLayout container;
public MyViewHolder(View itemView) {
super(itemView);
tv = (TextView) itemView.findViewById(R.id.t1);
container = (LinearLayout) itemView.findViewById(R.id.main_container);
}
}
@Override
public int getItemCount() {
return dataSource.size();
}
/**
* 给recyclerview 添加一个条目
*/
public void addItem(){
dataSource.add("hello recyler");
notifyDataSetChanged();
}
/**
* 根据指定的位置删除一个条目
* @param postion
*/
public void removeItem(int postion){
dataSource.remove(postion);
notifyDataSetChanged();
}
}
The layout file of each entry will not be posted, just a simple textview
In this way, a more gorgeous and collapsible title bar can be produced. . . .