One: UGUI production
1. First, create the Slider component in the UI under [Hierarchy]. Set its corresponding width and height.
2. Adjust the fill color of the Slider slider. The general sound color is yellow, so we also tune it to yellow.
We try to slide the value inside the Slider.
a. Before sliding.
b. Slide halfway.
c. Finish sliding.
From the above sliding value, we can know that the filling effect is controlled by the value. Making the blood bar is similar, depending on what it is used for, we make a sliding control volume bar, so we need to get the sound first.
Three: Create an empty object to mount the sound
We create an empty object named BGM, then add the AudioSource sound source component , and add the size of the sound that needs to be controlled in the component.
At this point we have mounted the sound that needs to be controlled, and then we create a script to realize that the volume of the sound changes with the value of the Slider component .
Four: Code implementation to control the volume of the sound
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class BGM : MonoBehaviour { // Start is called before the first frame update //声音控制器 public Slider AudioSlider; //声音控制器文本 public Text AudioText; //音乐 public AudioSource BGMsource; void Start() { //默认一开始声音为0.6 AudioSlider.value = 0.6f; } //控制声音大小方法 public void AudioCtrl() { //把value的值赋值给 BGMsource.volume BGMsource.volume = AudioSlider.value; //文本显示当前声音大小 AudioText.text = AudioSlider.value.ToString(); } }
Five: Back to the Unity engine
1. In order to be able to see it clearly, we have done more detailed above, adding a text to display the sound level.
So we need to create a Text text in Unity and set the size of the Text text.
2. Mount components and objects
3. run
a. The default sound is 0.6
b. Slide the Slider component in the game scene, and the corresponding sound volume value will change accordingly.
To make a sound controller, we should also have a button, check it to have a sound, disable it and the sound will be gone. Next, let's implement it.
Six: Sound controller switch
1. Create the Toggle component
2. Add the corresponding components to the code
After improvement: the following is the overall code.
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class BGM : MonoBehaviour { // Start is called before the first frame update //声音控制器 public Slider AudioSlider; //声音控制器文本 public Text AudioText; //声音开关 public Toggle AudioSwitchToggle; //音乐 public AudioSource BGMsource; void Start() { //默认一开始声音为0.6 AudioSlider.value = 0.6f; } //控制声音大小方法 public void AudioCtrl() { //把value的值赋值给 BGMsource.volume BGMsource.volume = AudioSlider.value; //文本显示当前声音大小 AudioText.text = AudioSlider.value.ToString(); } //控制声音开关 public void AudioSwitchToggleCtrl() { //如果声音控制按钮勾选了,那么我们就有声音 if (AudioSwitchToggle.isOn) { //激活声音对象为自动播放 BGMsource.gameObject.SetActive(true); //调用移动滑块控制声音大小 AudioCtrl(); } else { //关闭声音对象 BGMsource.gameObject.SetActive(false); } } }
3. Mount tgoole object and add method
Seven: Operation effect diagram
1: runtime
2: After clicking the toggle button
Here we run the exploit so that we can see that the BGM object is directly disabled , and no sound can be heard at runtime.
Therefore, it has been realized to check to turn on the sound, and to disable the effect of unchecking the sound.
One more thing to add: If we need to keep the sound playing, we can check the Loop on the AudioSource component to play in a loop. After checking, the sound can be played in a loop. Generally, sound is essential in the production process of us using Unity.
at last
The above steps are the whole process of making the sound switch controller and the sound slider to control the volume of the sound.
Friends who have seen it, click three times with one button. Your support makes me more motivated to create and share. I hope it will always bring you surprises and gains.
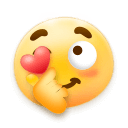