1. What are the array methods encapsulated in Vue, and how to implement page update
In Vue, Object.defineProperty is used to intercept data for responsive processing, and this method cannot monitor internal changes in the array, changes in the length of the array, changes in the interception of the array, etc., so these operations need to be hacked, so that Vue changes can be monitored.
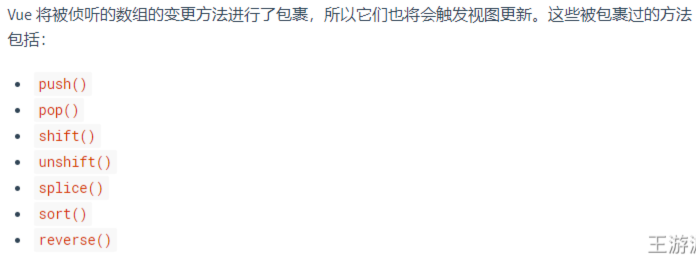
So how does Vue realize the real-time update of these array methods? The following is the encapsulation of these methods in Vue:
// 缓存数组原型
const arrayProto = Array.prototype;
// 实现 arrayMethods.__proto__ === Array.prototype
export const arrayMethods = Object.create(arrayProto);
// 需要进行功能拓展的方法
const methodsToPatch = [
"push",
"pop",
"shift",
"unshift",
"splice",
"sort",
"reverse"
];
/**
* Intercept mutating methods and emit events
*/
methodsToPatch.forEach(function(method) {
// 缓存原生数组方法
const original = arrayProto[method];
def(arrayMethods, method, function mutator(...args) {
// 执行并缓存原生数组功能
const result = original.apply(this, args);
// 响应式处理
const ob = this.__ob__;
let inserted;
switch (method) {
// push、unshift会新增索引,所以要手动observer
case "push":
case "unshift":
inserted = args;
break;
// splice方法,如果传入了第三个参数,也会有索引加入,也要手动observer。
case "splice":
inserted = args.slice(2);
break;
}
//
if (inserted) ob.observeArray(inserted);// 获取插入的值,并设置响应式监听
// notify change
ob.dep.notify();// 通知依赖更新
// 返回原生数组方法的执行结果
return result;
});
});
To put it simply, the original methods in the array are rewritten. First, get the __ob__ of the array, which is its Observer object. If there is a new value, call observeArray to continue to observe the change of the new value (that is, The type of the array instance is changed by target__proto__ == arrayMethods), and then manually call notify to notify the rendering watcher and execute update.