Spring Boot+Redis implements a lightweight message queue
Article directory
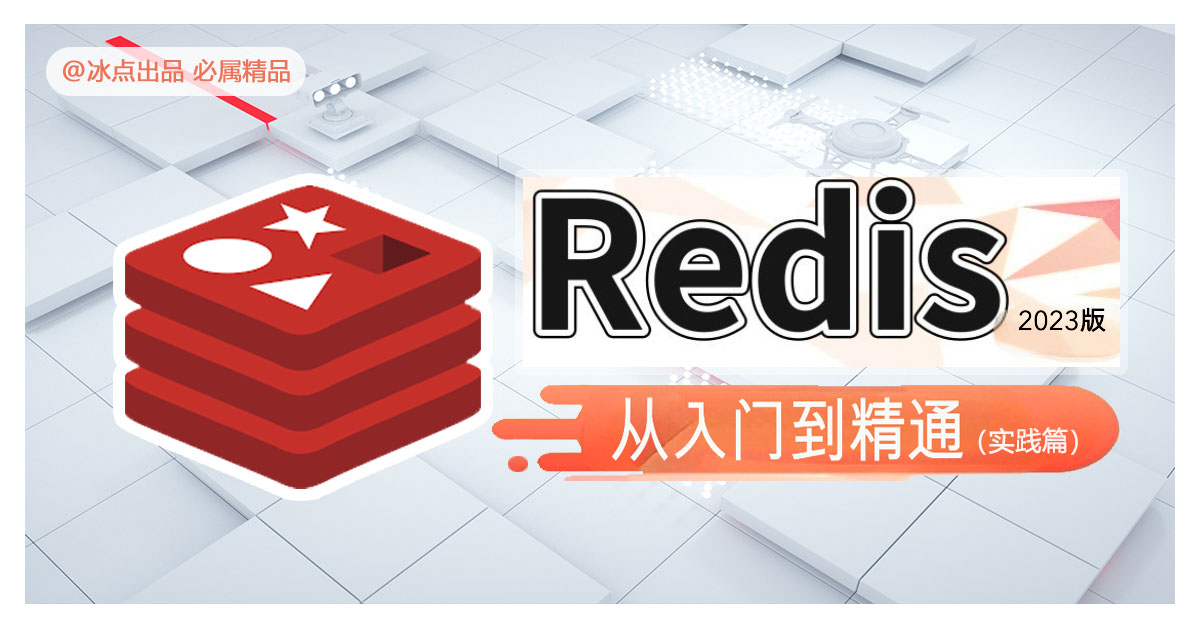
0. Preface
This article will introduce a practical example of how to use Spring Boot and Redis to implement a message queue. Message queue is a commonly used mechanism for decoupling and asynchronous communication, which can realize efficient message delivery and processing in the system. As a high-performance in-memory database, Redis has a publish/subscribe function, which is very suitable for building a message queue system.
In this practical example, we will use the Spring Boot framework and Redis as the basis to implement a simple message queue through Redis' publish/subscribe model. How to publish and subscribe to messages through message channels.
Before you start, you need to have some understanding of Spring Boot and Redis, and make sure your development environment is properly configured. This article will provide actual code examples and detailed step-by-step instructions, and can be extended and customized according to specific business needs.
1. Basic introduction
Redis provides a publish/subscribe (Publish/Subscribe) mode for broadcasting and asynchronous communication of messages. The following is an introduction to the Redis publish/subscribe model:
Publish/Subscribe mode (Pub/Sub) :
Publish/Subscribe mode is a message communication mode in which the publisher of the message (Publisher) sends the message to a specific channel (Channel), and the subscriber (Subscriber) can subscribe to one or Multiple channels to receive messages. This pattern allows broadcasting and asynchronous delivery of messages, with decoupling between sender and receiver.
Redis publish/subscribe function :
Redis provides a native publish/subscribe function, enabling developers to use Redis as a message middleware to achieve efficient message delivery. The following are the key concepts and operations related to Redis publish/subscribe:
Here is an example of Redis publish/subscribe functionality organized into a table:
concept | illustrate |
---|---|
Channel | A channel in Redis for publishing and subscribing messages. Each message is published to a specific channel, and subscribers can choose to subscribe to one or more channels of interest. |
Publish | By using the PUBLISH command, the publisher can send the message to the specified channel. Once a message is published to a channel, all clients subscribed to the channel will receive the message. |
Subscribe to a channel (Subscribe) | By using the SUBSCRIBE command, a client can subscribe to one or more channels. Once the subscription is successful, the client will become a subscriber of the channel and can receive messages published on the channel. |
Unsubscribe from a channel (Unsubscribe) | By using the UNSUBSCRIBE command, a client can unsubscribe from one or more channels. When a client is no longer interested in a channel, it can choose to unsubscribe. |
Pattern Subscription | Redis supports pattern matching subscriptions using the PSUBSCRIBE command. By specifying a pattern, multiple channels matching that pattern can be subscribed to. |
Through the publish/subscribe function of Redis, an efficient message system can be built to realize the broadcast and asynchronous delivery of messages. Publishers publish messages to specific channels, and subscribers can choose to subscribe to interested channels to receive messages. The advantages of this mode include decoupling, asynchronous communication, and real-time performance. It is suitable for many scenarios, such as real-time notification, event-driven systems, and message passing between distributed systems.
In this article, we implement a lightweight message queue through Spring Boot + Redis.
2. Steps
2.1. Introducing dependencies
<dependencies>
<!-- Spring Data Redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
</dependencies>
2.2. Configuration file
# Redis连接配置
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=your_password
spring.redis.database=0
# Redis连接池配置
spring.redis.jedis.pool.max-active=50
spring.redis.jedis.pool.max-idle=10
spring.redis.jedis.pool.min-idle=5
spring.redis.jedis.pool.max-wait=-1
In the above configuration, you can modify the following properties according to the actual situation:
spring.redis.host
: The hostname or IP address of the Redis server.spring.redis.port
: The port number of the Redis server.spring.redis.password
: The password of the Redis server (if any).spring.redis.database
: The index of the Redis database, the default is 0.
In addition, you can also configure the properties of the Redis connection pool to control the behavior of the connection pool. In the example configuration, the following connection pool properties are set:
spring.redis.jedis.pool.max-active
: The maximum number of active connections in the connection pool.spring.redis.jedis.pool.max-idle
: The maximum number of idle connections in the connection pool.spring.redis.jedis.pool.min-idle
: The minimum number of idle connections in the connection pool.spring.redis.jedis.pool.max-wait
: The maximum waiting time (in milliseconds) for obtaining a connection from the connection pool, -1 means infinite waiting.
If you are using a configuration file in YAML format ( application.yml
), you can convert the above configuration to the corresponding format:
spring:
redis:
host: 127.0.0.1
port: 6379
password: your_password
database: 0
redis.jedis.pool:
max-active: 50
max-idle: 10
min-idle: 5
max-wait: -1
Please adjust it according to your actual Redis server configuration, and add other relevant configurations as needed, such as timeout settings, SSL configuration, etc.
2.3. Core source code
When using Spring Boot and Redis to implement a message queue, you can use the publish/subscribe function of Redis to handle the publication and subscription of messages. Below is
Next, create a message publisher and a message subscriber class. In the publisher class, use RedisTemplate
to publish the message, and in the subscriber class, MessageListener
process the received message by implementing the interface.
// 消息发布者
@Component
public class MessagePublisher {
private final RedisTemplate<String, String> redisTemplate;
private final ChannelTopic topic;
public MessagePublisher(RedisTemplate<String, String> redisTemplate, ChannelTopic topic) {
this.redisTemplate = redisTemplate;
this.topic = topic;
}
public void publishMessage(String message) {
redisTemplate.convertAndSend(topic.getTopic(), message);
}
}
message subscriber
// 消息订阅者
@Component
public class MessageSubscriber implements MessageListener {
@Override
public void onMessage(Message message, byte[] pattern) {
String receivedMessage = message.toString();
// 处理接收到的消息
System.out.println("Received message: " + receivedMessage);
}
}
Next, configure the Redis message listener container to start the message listener:
@Configuration
public class RedisConfig {
@Bean
public ChannelTopic topic() {
return new ChannelTopic("messageQueue"); // 定义消息队列的通道名称
}
@Bean
public MessageListenerAdapter messageListenerAdapter(MessageSubscriber messageSubscriber) {
return new MessageListenerAdapter(messageSubscriber);
}
@Bean
public RedisMessageListenerContainer redisContainer(RedisConnectionFactory redisConnectionFactory,
MessageListenerAdapter messageListenerAdapter,
ChannelTopic topic) {
RedisMessageListenerContainer container = new RedisMessageListenerContainer();
container.setConnectionFactory(redisConnectionFactory);
container.addMessageListener(messageListenerAdapter, topic);
return container;
}
}
Finally, where the message needs to be published, inject MessagePublisher
and call publishMessage()
the method to publish the message:
@Service
public class MyService {
private final MessagePublisher messagePublisher;
public MyService(MessagePublisher messagePublisher) {
this.messagePublisher = messagePublisher;
}
public void doSomethingAndPublishMessage() {
// 执行一些操作
String message = "Hello, Redis message queue!";
messagePublisher.publishMessage(message);
}
}
In this way, when the method MyService
in is called doSomethingAndPublishMessage()
, the message will be published to the message queue of Redis, and be received and processed by the subscriber.
Please note that the above example is a simplified implementation, just to demonstrate the basic message publishing and subscribing process. In the actual production environment, more details may need to be considered, such as error handling, message confirmation mechanism, concurrent processing, etc.
4. Summary
Through the publish/subscribe model of Redis, Spring Boot uses RedisTemplate to publish messages to the specified Redis channel, and uses MessageListenerAdapter to bind subscribers to the message channel. When a message is published to the channel, Redis will broadcast the message to all clients subscribed to the channel, and then the MessageListenerAdapter will pass the received message to the corresponding message listener for processing.
In this way, using the publish/subscribe function of Redis, we can implement a message queue system based on Spring Boot and Redis to publish and subscribe messages. In this asynchronous way, message publishers and subscribers can be decoupled, improving the scalability and performance of the system.
answer questions
Last time I introduced Redis's pub and sub, as well as Stream. Many students have doubts about which one should be used in the project. So let's talk about it here
. Redis provides two different functions to implement message queues: publish/subscribe (Pub/Sub) and stream (Stream). Which function you choose to use as a message queue depends on your specific needs and usage scenarios.
features | Redis publish/subscribe (Pub/Sub) | Redis style (Stream) |
---|---|---|
Applicable scene | Simple message broadcast and asynchronous communication scenarios | More complex message queue requirements, including task queues, event sourcing, log collection, etc. |
message persistence | Persistence and history of messages are not supported | Support message persistence and history |
News subscription method | Subscribers choose to subscribe to channels of interest to receive messages | Multiple consumers consume messages |
Messages published before subscribing | Unable to get messages prior to subscription | You can get the message published before the subscription |
real-time | Suitable for scenarios requiring instant notification, real-time event processing and real-time data delivery | High real-time performance, but affected by the processing speed of consumers |
Consumer Group Management | Does not support consumer group management | Support consumer group management |
message sequence | The order of messages is not guaranteed | message order guarantee |
example use case | Instant chat applications, real-time notification systems, publish/subscribe messaging | Task queue, event tracing, log collection, etc. |
So if your needs are simple message broadcasting and asynchronous communication, and you don't have high requirements for message persistence and history, you can choose to use the Redis publish/subscribe function as a message queue. And if you need richer message queuing functions, including message persistence, support for multiple consumers, and message history, then Redis Stream is a better choice. According to the specific usage scenarios and requirements, select the appropriate function to implement the message queue.
5. Reference documents
-
Official Redis Documentation - Publish/Subscribe:
- Link: https://redis.io/topics/pubsub ↗
- This document provides a detailed description of the Redis publish/subscribe function, including basic concepts, command usage, sample code, and FAQs, etc.
-
Redis command documentation - PUBLISH:
- Link: https://redis.io/commands/publish ↗
- This document introduces the syntax, parameters, and usage of the PUBLISH command, as well as notes related to publishing messages.
-
Redis command documentation - SUBSCRIBE:
- Link: https://redis.io/commands/subscribe ↗
- This document details the syntax, parameters and usage of the SUBSCRIBE command, and how to subscribe to a channel and receive published messages.
-
Redis command documentation - UNSUBSCRIBE:
- Link: https://redis.io/commands/unsubscribe ↗
- This document provides a description of the UNSUBSCRIBE command, including the syntax and parameters for unsubscribing a channel.
-
Redis command documentation - PSUBSCRIBE:
- Link: https://redis.io/commands/psubscribe ↗
- This document introduces the usage of the PSUBSCRIBE command for pattern matching subscription, which can subscribe to multiple channels matching the specified pattern.
6. Redis from entry to proficiency series of articles
- "Redis uses Lua script and Redisson to ensure atomicity and consistency in inventory deduction"
- "SpringBoot Redis uses Lettuce and Jedis to configure sentinel mode"
- "Redis [Application] RedisTemplate Basic Operation"
- "Redis From Getting Started to Mastering [Practice] SpringBoot Configuring Redis Multiple Data Sources"
- "Redis From Getting Started to Mastering [Advanced] Three Minutes to Understand Redis HyperLogLog Data Structure"
- "Redis From Getting Started to Mastering [Advanced] Three Minutes to Understand Redis Geographical Location Data Structure GeoHash"
- "Redis From Getting Started to Mastering [Advanced Chapter] Detailed Explanation of Highly Available Sentinel Mechanism (Redis Sentinel)"
- Detailed Explanation of Redis Master-Slave Replication from Getting Started to Mastering [Advanced Chapter]
- "Redis from entry to proficiency [advanced chapter] Redis affairs detailed explanation"
- Detailed Explanation of the Object Mechanism of Redis from Getting Started to Mastering [Advanced Chapter]
- "Redis From Getting Started to Mastering [Advanced Chapter]: Detailed Explanation of the Messaging Publishing and Subscribing Model"
- "Redis From Getting Started to Mastering [Advanced Chapter] Detailed Explanation of Persistent AOF"
- "Redis From Getting Started to Mastering [Advanced Chapter] Detailed Explanation of Persistent RDB"
- "Redis from entry to proficiency [advanced articles] detailed explanation of the underlying data structure dictionary (Dictionary)"
- "Redis from entry to proficiency [high-level articles] detailed explanation of the underlying data structure quick table QuickList"
- "Redis from entry to proficiency [high-level articles] detailed explanation of the underlying data structure simple dynamic string (SDS)"
- "Redis from entry to proficiency [high-level articles] detailed explanation of the underlying data structure compression list (ZipList)"
- "Redis from entry to proficiency [Advanced Chapter] Detailed Explanation and Example of Data Type Stream"
Hello everyone, I am Freezing Point, today's Spring Boot+Redis implements a practical example of message queuing, and this is all the content. If you have questions or opinions, you can leave a message in the comment area.