jvm memory model
Oracle official website explains:
Chapter 17. Threads and Locks (oracle.com)
jvm memory structure
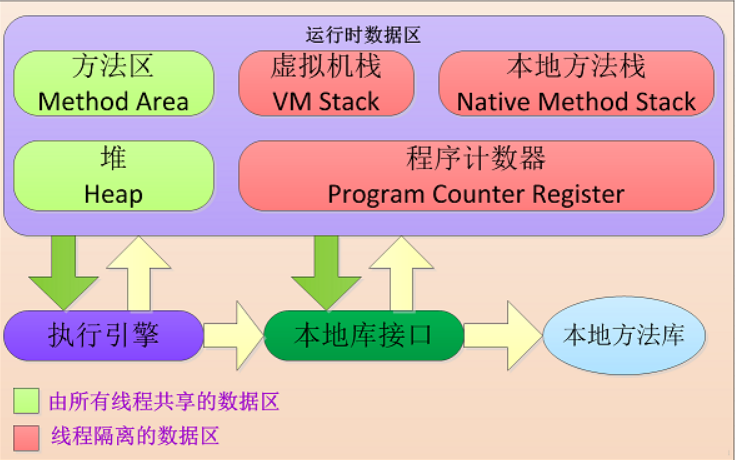
method area
Sometimes it also becomes the permanent generation. Garbage collection rarely occurs in this area, but it does not mean that GC does not occur. The GC performed here is mainly to unload the constant pool and types in the method area
The method area is mainly used to store data such as information of classes loaded by the virtual machine, constants, static variables, and code compiled by the just-in-time compiler.
This area is shared by threads.
There is a runtime constant pool in the method area, which is used to store literals and symbol references generated by static compilation. The constant pool is dynamic, which means that constants are not necessarily determined at compile time, and constants generated at runtime will also exist in this constant pool.
Virtual machine stack:
The virtual machine stack is what we usually call stack memory. It serves java methods. Each method will create a stack frame when it is executed to store information such as local variable tables, operand stacks, dynamic links, and method exits. .
The virtual machine stack is private to the thread, and its life cycle is the same as that of the thread.
The local variable table stores basic data types, returnAddress types (pointing to the address of a bytecode instruction) and object references. This object reference may be a pointer pointing to the starting address of the object, or it may be a handle representing the object or The location associated with the object. The memory space required for local variables is determined between compilers
The function of the operand stack is mainly used to store the operation results and the operands of the operation. It is different from the local variable table accessed through the index, but the way of pushing and popping the stack
Each stack frame contains a reference to the method to which the stack frame belongs in the runtime constant pool. This reference is held to support dynamic linking during the method call. Dynamic linking is to convert symbolic references in the constant pool at runtime for direct reference.
Native method stack:
The local method stack is similar to the virtual machine stack, except that the local method stack serves the Native method.
heap :
The java heap is a piece of memory shared by all threads. It is created when the virtual machine starts, and almost all object instances are created here, so garbage collection operations often occur in this area.
Program counter:
The memory space is small, and the bytecode interpreter can select the next bytecode instruction to be executed by changing the count value when it is working. Functions such as branching, looping, jumping, exception handling, and thread recovery all rely on this counter to complete. This memory region is the only one where the java virtual machine specification does not specify any OOM conditions.
jvm class loading process
Load→Validate→Parse→Initialize
load
The first process of class loading during loading. At this stage, three things will be done:
Get the binary stream of a class by its fully qualified name.
Convert the static storage structure in the binary stream into a method to run the data structure.
Generate the Class object of this class in memory as the data access entry of this class.
verify
The purpose of verification is to ensure that the information in the byte stream of the Class file will not harm the virtual machine. At this stage, the following four verifications are mainly completed:
File format verification: Verify whether the byte stream conforms to the specification of the Class file, such as whether the major and minor version numbers are within the range of the current virtual machine, and whether the constants in the constant pool have unsupported types.
Metadata verification: perform semantic analysis on the information described by the bytecode, such as whether this class has a parent class, whether it integrates classes that are not inherited, etc.
Bytecode verification: It is the most complicated stage in the entire verification process. By verifying the analysis of data flow and control flow,
Determine whether the semantics of the program are correct, mainly for the verification of the method body. Such as: whether the type conversion in the method is correct, whether the jump instruction is correct, etc.
Symbol reference verification: This action occurs during the subsequent parsing process, mainly to ensure that the parsing action can be executed correctly.
Prepare
The preparation stage is to allocate memory for the static variables of the class and initialize them to default values, and these memories will be allocated in the method area. The preparation phase does not allocate the memory of the instance variables in the class, and the instance variables will be allocated in the Java heap along with the object when the object is instantiated.
analyze
This stage mainly completes the conversion action from symbolic reference to direct reference. The parsing action is not necessarily before the initialization action is completed, and it may be after the initialization.
initialization
The last step of class loading during initialization, the previous class loading process, except that the user application can participate in the loading phase through the custom class loader, the rest of the actions are completely dominated and controlled by the virtual machine. In the initialization phase, the Java program code defined in the class is actually executed.
Parental Delegation Mechanism
There are 3 default class loaders in the JVM:
1. Bootstrap Class Loader (Bootstrap Class Loader)
2. Extension Class Loader
3. Application Class Loader
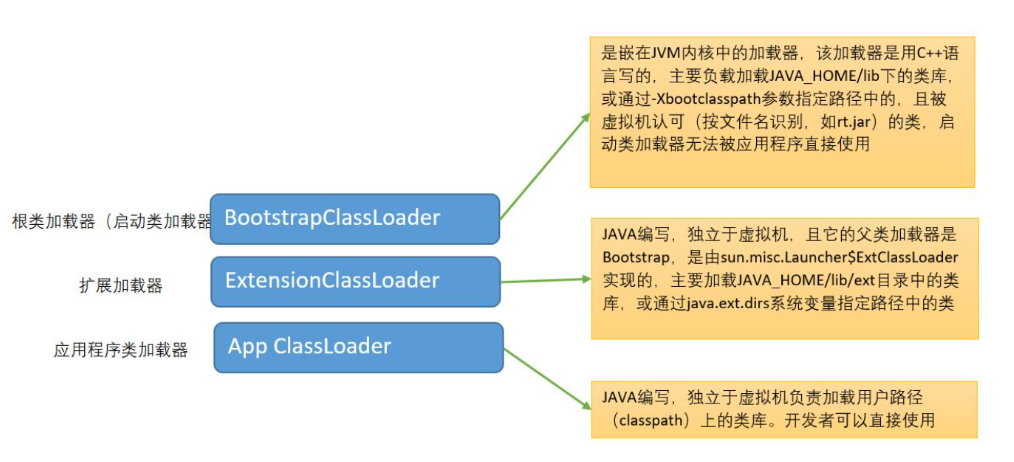
The parental delegation model requires that all class loaders except the top-level startup class loader should have their own parent class loader.
The Java platform loads classes through a delegation model. Every class loader has a parent loader.
1. When a class needs to be loaded, the parent loader of the loader of the current class will be assigned first to load this class.
2. If the parent loader cannot load this class, try to load this class in the loader of the current class.
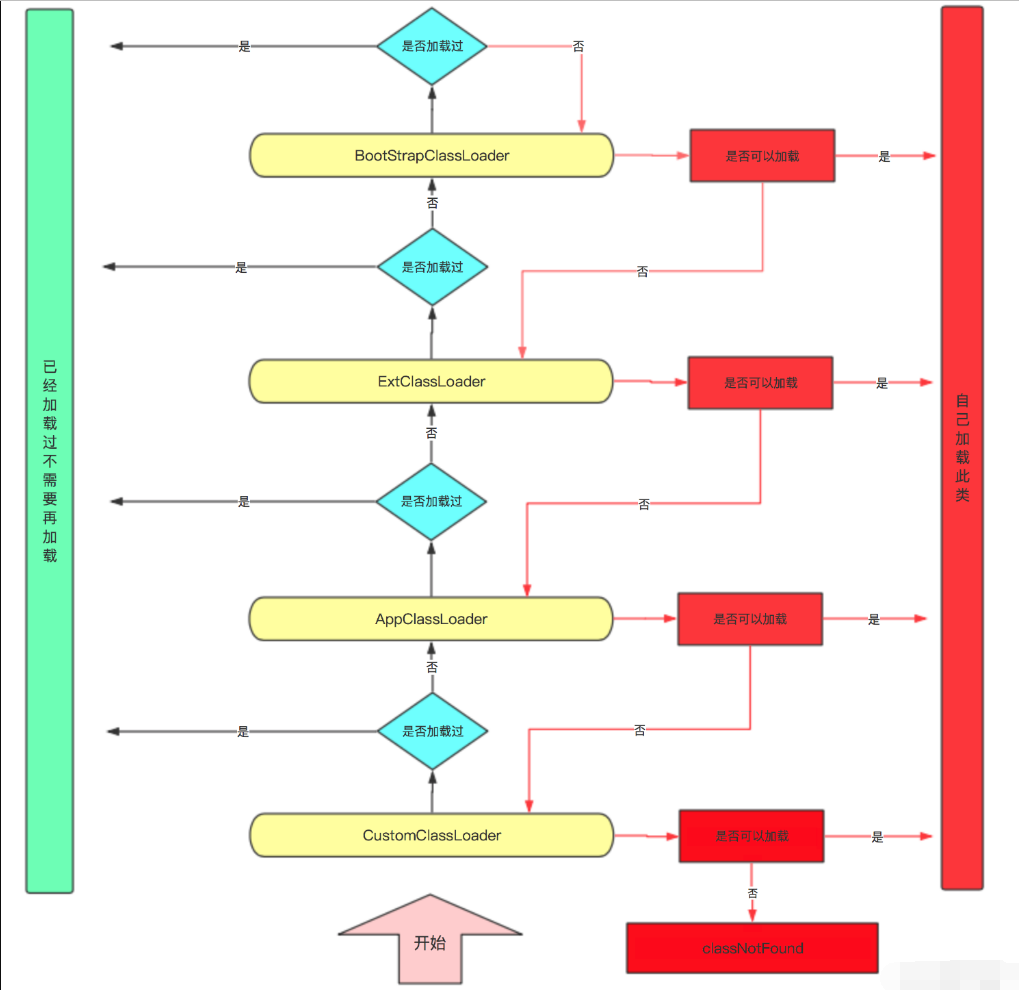
1. Because the parent delegation is loaded upward, it can ensure that the class is only loaded once, avoiding repeated loading
共享功能:一些framework层级的类一旦被顶层加载器加载,缓存在内存。在其他任何地方用到时,都遵守双亲加载机制,派发到顶层加载器因已经加载,所以都不需要重新加载。
2. Avoid serial modification of core classes: Java’s core APIs are loaded through the bootstrap class loader. If someone else defines a class with the same path, such as java.lang.Integer, the class loader delegates upwards, two Integers, Then the Integer class of jdk should be loaded in the end, not our custom one, so as to avoid the risk of us maliciously tampering with the core package
隔离功能:保证核心类库的纯净和安全,防止恶意加载。