I was born again, and this article explores classes and objects in C++ in depth.
Table of contents
1. Introduction and definition of class
2. Access qualifiers and class encapsulation
1. Introduction and definition of class
Introduction of class: In C language, only variables or structures can be defined inside the structure. In C++, the structure is upgraded -> class. In C++ classes, both variables and functions can be defined. Variables in a class are called attributes or member variables; functions are called member functions or methods. A class is an encapsulation of properties and methods for manipulating properties.
class definition:
class classname
{//类体
//属性
//操作属性的一些方法
};//注意,这里有分号
Class method declarations and definitions can also be separated:
//main.h
class A
{
int a;
int b;
void func();//成员函数声明
};
//main.cpp
void A::func()//成员函数的定义
{
cout<<"func()"<<endl;
}
When the declaration and definition of a member function are separated, the name of the member function must be preceded by the class name:: to limit the defined function to be in this class.
Member variable naming habits: The naming of member variables is generally preceded by _ for example
int _a;
2. Access qualifiers and class encapsulation
class classname
{
private:
int _date;
public:
void ModifyDate(int date)//通过共有成员函数来访问类的成员变量(属性)
{
_date=date;
}
};
3. Class instantiation
Can the class be used directly? the answer is negative. Defining a class does not allocate actual memory space. To use this template, you need to use the class to create an object (apply for space), and we can use this object. This is the process of class instantiation.
A class can create any number of objects, and each object is instantiated based on the template of this class. Each object does not interfere with each other and is an independent individual.
#include<iostream>
using namespace std;
class People
{
private://私有域
int _age;
int _height;
public://公有域
void eat()
{
cout << "吃饭" << endl;
}
void sleep()
{
cout << "睡觉" << endl;
}
};
int main()
{
People man;//用类创建对象,类的实例化
man.eat();//实例化后使用对象
return 0;
}
4. Class size
How is the class size calculated?
cout << sizeof(man) << endl;
cout << sizeof(People) << endl;
It should be explained here that since the functions of member functions of different objects of the same class are the same, member functions are stored in the common code area, and only member variables are stored in objects. That is, the object size is the sum of the member variable sizes (pay attention to memory alignment).
Five. this pointer
Consider this question:
#include<iostream>
using namespace std;
class People
{
private://私有域
int _age;
int _height;
public://公有
void inti(int age,int height)
{
_age=age;
_height=height;
}
};
int main()
{
People p1;
People p2;
p1.inti(20,170);
p2.inti(25,175);
return 0;
}
Since the inti member function in the People class is in the public code area, and there is no distinction between different objects in the function body, how does inti distinguish between initializing the member variables of p1 or initializing the member variables of the p2 object? When p1 calls the inti function, how does the function know whether to set the p1 object or the p2 object?
void inti(int age,int height)
{
_age=age;
_height=height;
}
Actually it is:
void inti(People* this,int age,int height)
{
_age=age;
_height=height;
}
and
p1.inti(20,170);
Actually it is:
inti(&p1,20,170);
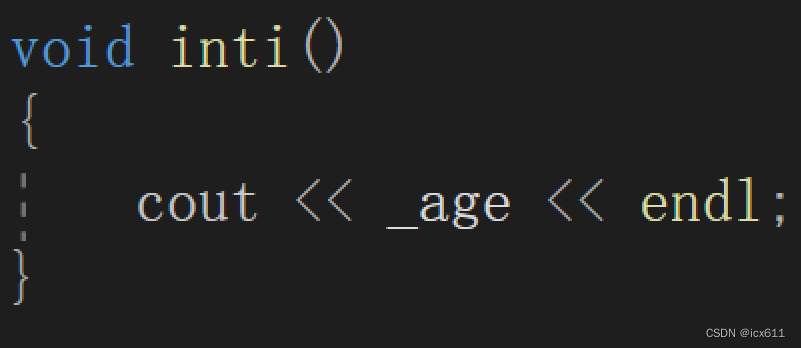
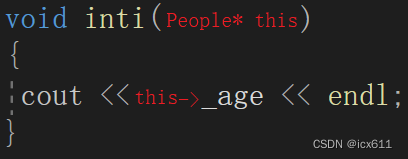