→ springboot integrates Swagger2 ←
Table of contents
1. Case
This time directly use the spring-boot of 2.5.6.
-
rely:
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.5.6</version> <relativePath/> <!-- lookup parent from repository --> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!--swagger3--> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-boot-starter</artifactId> <version>3.0.0</version> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> </dependencies>
-
Annotate the startup class
@EnableOpenApi
-
New test class
@RestController @RequestMapping("/test") public class SwaggerController { @GetMapping("/get") public String getStr(String str) { return "SELECT " + str; } @PostMapping("/post") public String postStr(String str) { return "CREATE " + str; } @PutMapping("/put") public String putStr(String str) { return "UPDATE " + str; } @NoSwagger @PatchMapping("/patch") public String patchStr(String str) { return "UPDATE " + str; } @DeleteMapping("/delete") public String deleteStr(String str) { return "DELETE " + str; } }
-
Visit http://127.0.0.1:8080/swagger-ui.html , yes, it is Error page again
Reference for this part: https://blog.csdn.net/mmmm0584/article/details/117786055
In swagger3.0, the location of swagger-ui.html has changed:
→
So the path has also changed: http://127.0.0.1:8080/swagger-ui.html → http://127.0.0.1:8080/ swagger-ui/index.html
- Visit http://127.0.0.1:8080/swagger-ui/index.html
2. info configuration
Create a new configuration class:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.service.VendorExtension;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import java.util.HashSet;
@Configuration
public class Swagger3Conf {
@Bean
public Docket createDocket() {
return new Docket(DocumentationType.OAS_30)// 指定 Swagger3 版本号
.apiInfo(createApiInfo());
}
@Bean
public ApiInfo createApiInfo() {
// // 写法一
// return new ApiInfoBuilder()
// .title("Swagger3 文档案例")
// .description("Swagger :一套围绕 Open API 规范构建的一款 RESTful 接口的文档在线自动生成和功能测试 API 。")
// .version("1.0.1")
// .contact(
// // name url email
// new Contact("364.99°的文档", // 文档发布者名称
// "https://blog.csdn.net/m0_54355172", // 文档发布者的网站地址
// "[email protected]" // 文档发布者的邮箱
// )
// )
// .build();
// 写法二
return new ApiInfo(
"Swagger3 文档案例",
"Swagger :一套围绕 Open API 规范构建的一款 RESTful 接口的文档在线自动生成和功能测试 API 。",
"1.0.1",
"https://blog.csdn.net/m0_54355172",
new Contact("364.99°的文档", // 文档发布者名称
"https://blog.csdn.net/m0_54355172", // 文档发布者的网站地址
"[email protected]" // 文档发布者的邮箱
),
"364.99°",
"https://blog.csdn.net/m0_54355172",
new HashSet<>());
}
}
Docket
Instance of Swagger, Swagger can be configured through Docket object;ApiInfo
A configuration object described by a file.
3. Docket configuration
1. Switch configuration
@Bean
public Docket createDocket() {
return new Docket(DocumentationType.OAS_30)// 指定 Swagger3 版本号
.enable(false)// 关闭文档
.apiInfo(createApiInfo());
}
Note: Generally, Swagger is only used in the test environment, and it will be turned off in the production environment for safety and efficiency.
2. Scan path
.select()
.apis(RequestHandlerSelectors.basePackage("com.chenjy.swagger2.controller"))
.build()
3. Path matching
Create a new controller:
@Api(tags = "other 接口 API")
@RestController
@RequestMapping("/other")
public class OtherController {
@GetMapping("getInfo")
public String getInfo() {
return "information";
}
}
.select()
.paths(PathSelectors.ant("/other/**"))
.build()
4. Group management
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import java.util.HashSet;
@Configuration
public class Swagger3Conf {
@Bean
public Docket createDocket01() {
return new Docket(DocumentationType.OAS_30)// 指定 Swagger3 版本号
.groupName("other 组")
.enable(true)// 关闭文档
.select()
.paths(PathSelectors.ant("/other/**"))
.build()
.apiInfo(createApiInfo());
}
@Bean
public Docket createDocket02() {
return new Docket(DocumentationType.OAS_30)
.groupName("test 组")
.select()
.apis(RequestHandlerSelectors.basePackage("com.chenjy.swagger2.controller"))
.build()
.apiInfo(
new ApiInfoBuilder()
.title("Swagger3 文档案例")
.build()
);
}
@Bean
public ApiInfo createApiInfo() {
// // 写法一
// return new ApiInfoBuilder()
// .title("Swagger3 文档案例")
// .description("Swagger :一套围绕 Open API 规范构建的一款 RESTful 接口的文档在线自动生成和功能测试 API 。")
// .version("1.0.1")
// .contact(
// // name url email
// new Contact("364.99°的文档", // 文档发布者名称
// "https://blog.csdn.net/m0_54355172", // 文档发布者的网站地址
// "[email protected]" // 文档发布者的邮箱
// )
// )
// .build();
// 写法二
return new ApiInfo(
"Swagger3 文档案例",
"Swagger :一套围绕 Open API 规范构建的一款 RESTful 接口的文档在线自动生成和功能测试 API 。",
"1.0.1",
"https://blog.csdn.net/m0_54355172",
new Contact("364.99°的文档", // 文档发布者名称
"https://blog.csdn.net/m0_54355172", // 文档发布者的网站地址
"[email protected]" // 文档发布者的邮箱
),
"364.99°",
"https://blog.csdn.net/m0_54355172",
new HashSet<>());
}
}
4. Common annotations
1. Description
Like Swagger2 , its commonly used annotations are as follows:
Common Notes | Notes | common attributes | property description |
---|---|---|---|
@Api |
Class annotations, often used to alias controllers in documents | tags | alias |
@ApiOperation |
Method/class annotations, often used to describe methods | value notes |
Method Description Method Remarks |
@ApiParam |
Parameter/method/property annotations, commonly used to describe parameters | name value required |
Whether the supplementary description of the parameter name is required |
@ApiIgnore |
Method/property annotation, so that the annotated method does not generate documentation | - | - |
@ApiImplicitParam |
Method annotation, describing a parameter | name value name required paramType dataType defaultValue |
Parameter name Supplementary instructions Whether to pass parameter position (header, query, path) parameter type (default String) default value |
@ApiImplicitParams |
Used together with @ApiImplicitParam to describe a set of parameters | ![]() |
|
@ApiResponse |
Method annotation, expressing an error response message | code message response |
Integer string exception information (default String type) |
@ApiResponses |
Use with @ApiResponse, refer to @ApiImplicitParams | ||
@ApiModel |
Class annotation, when this entity class is used as the return type in the interface method in the API help document, this annotation is parsed | value description |
Entity class name supplementary description |
@ApiModelProperty |
Attribute annotation, used with @ApiModel | value example hidden |
property name example hidden |
2. Case
import com.chenjy.swagger2.dto.TestDto;
import io.swagger.annotations.*;
import org.springframework.web.bind.annotation.*;
import springfox.documentation.annotations.ApiIgnore;
@Api(tags = {
"test 接口 API"})
@RestController
public class SwaggerController {
@ApiResponses({
@ApiResponse(code = 1, message = "查询成功"),
@ApiResponse(code = -1, message = "查询失败")
})
@ApiImplicitParams({
@ApiImplicitParam(name = "name", value = "角色名", required = false, defaultValue = "张三"),
@ApiImplicitParam(name = "num", value = "序列号", required = true)
}
)
@PostMapping("/getInfo")
public TestDto getInfo(String name, Integer num) {
return new TestDto(name, num);
}
@ApiIgnore
@GetMapping("/getStr")
public String getStr(String name) {
return name;
}
}
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@NoArgsConstructor
@AllArgsConstructor
@ApiModel( value = "角色实体类", description = "存储数据并返回")
public class TestDto {
@ApiModelProperty( value = "姓名", example = "张三", hidden = false)
private String name;
@ApiModelProperty( value = "序列号", example = "1")
private Integer num;
}
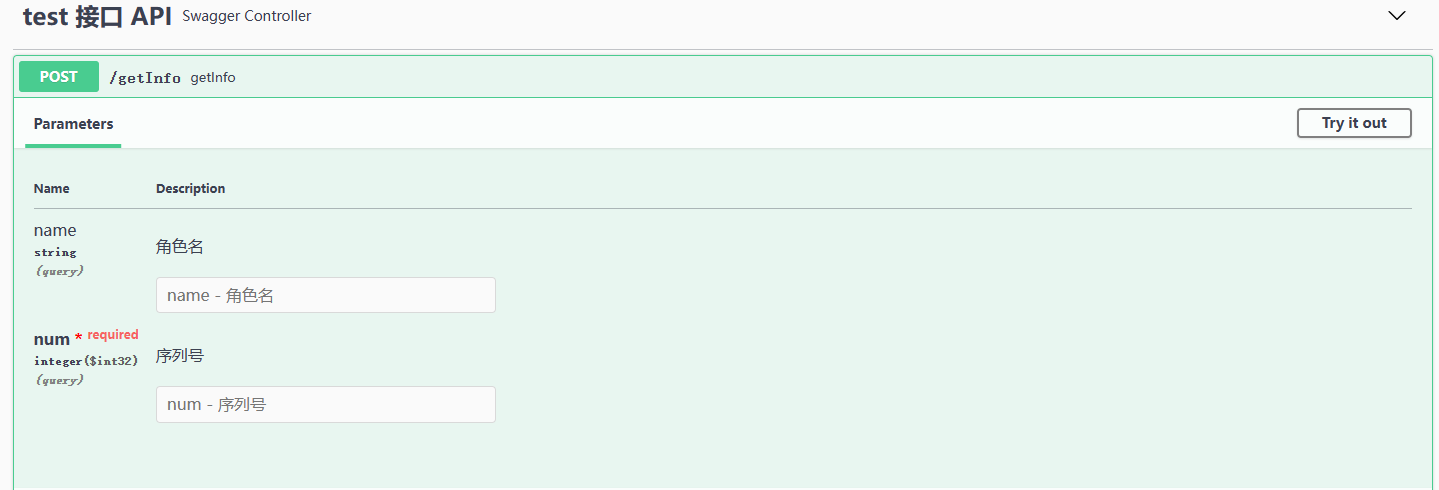
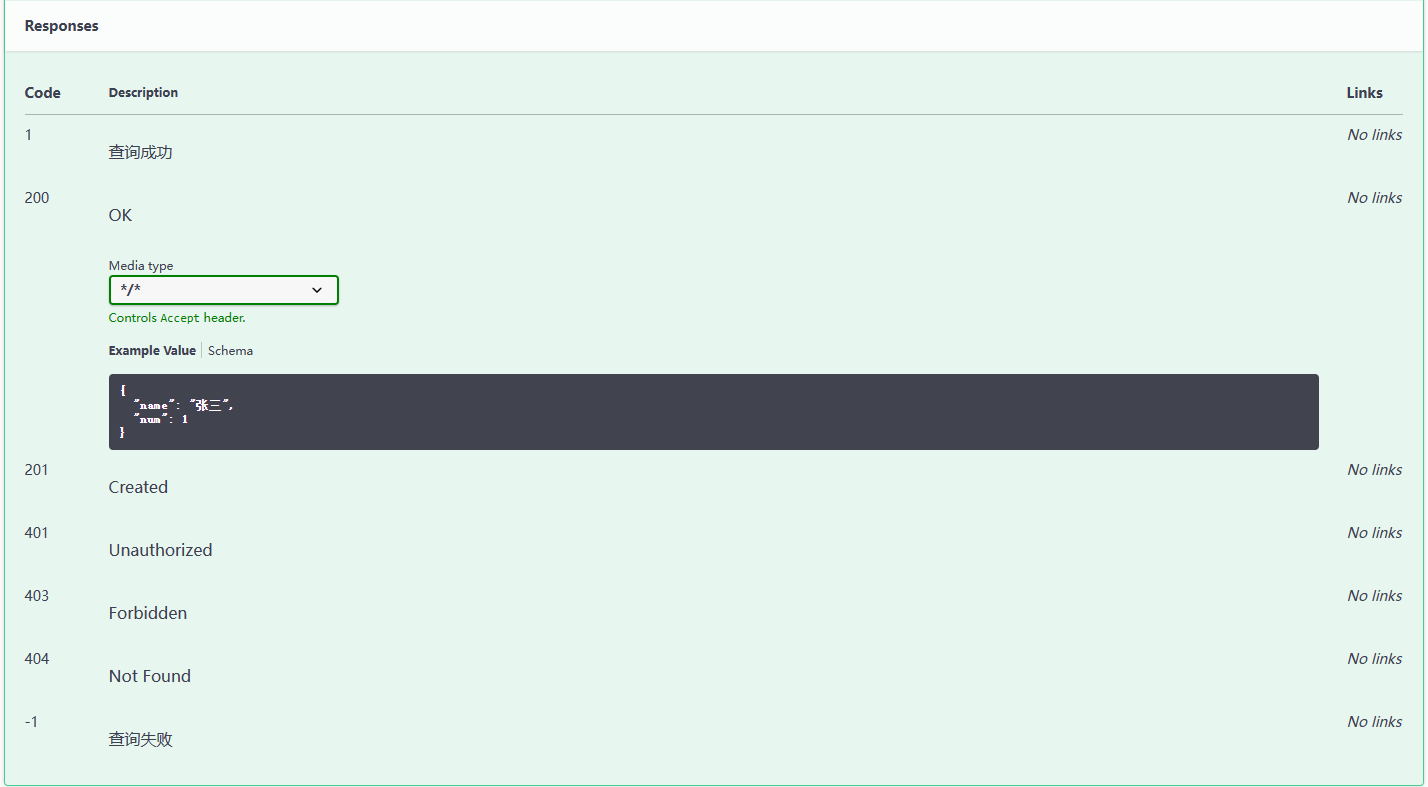
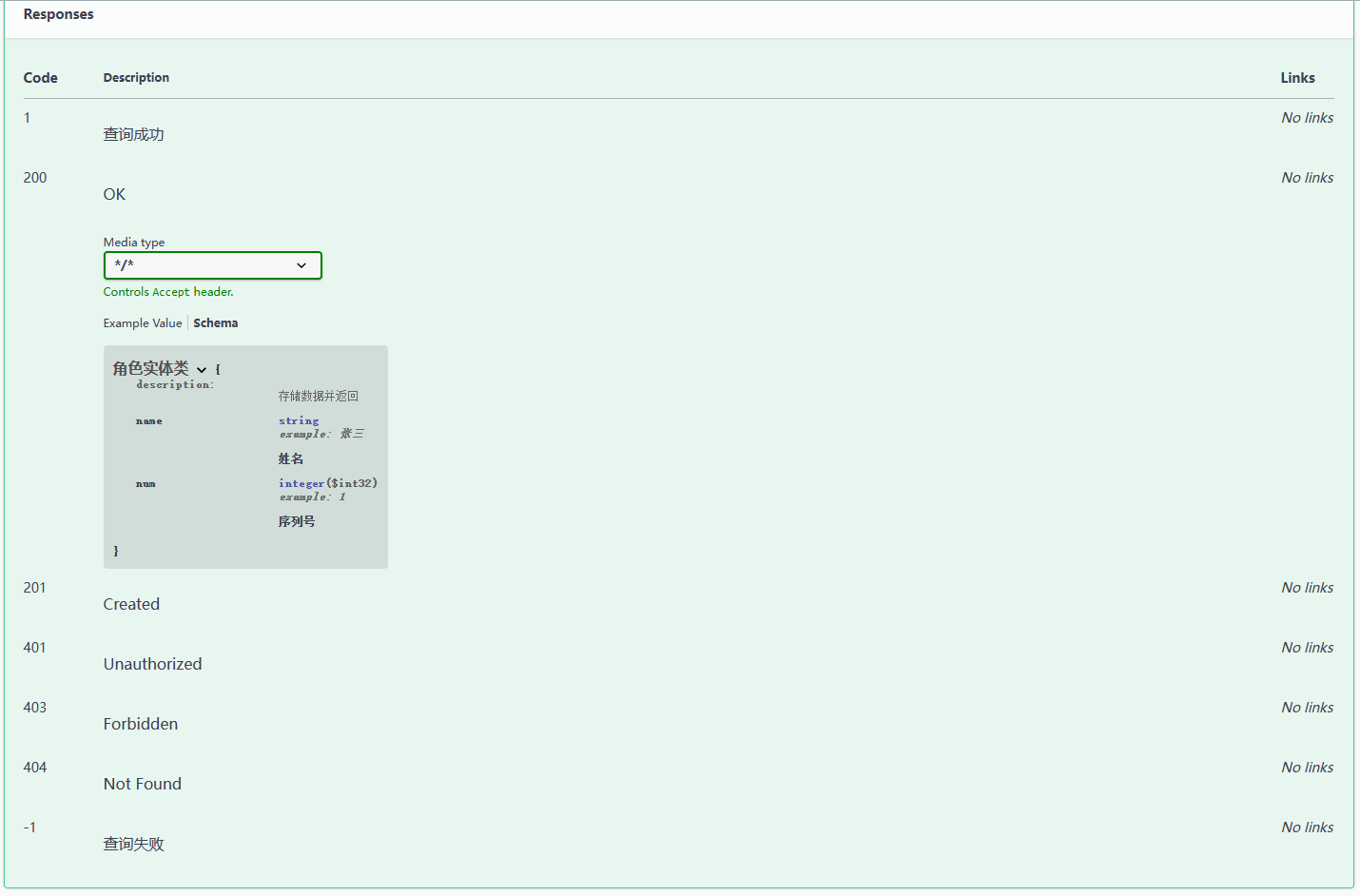