WPF template selector
Since multiple sets of templates need to be defined in the program in advance, it will affect the performance and overhead of the program, so use it with caution;
Project structure:
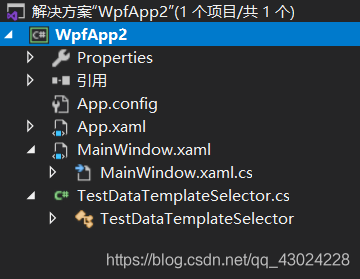
Create a template selection class TestDataTemplateSelector:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
namespace WpfApp2
{
public class TestDataTemplateSelector: DataTemplateSelector
{
public DataTemplate HighTemplate { get; set; }
public DataTemplate LowTemplate { get; set; }
public override DataTemplate SelectTemplate(object item, DependencyObject container)
{
var stu = (Student)item;
if (stu.Result > 60)
return HighTemplate;
else
return LowTemplate;
}
}
}
MainWindow.xaml code:
Two templates HighTemplate and LowTemplate are defined in Window.Resources.
<Window x:Class="WpfApp2.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp2"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Window.Resources>
<DataTemplate x:Key="HighTemplate">
<StackPanel>
<TextBlock Text="{Binding Name}"/>
<TextBlock Text="考的非常好!"/>
</StackPanel>
</DataTemplate>
<DataTemplate x:Key="LowTemplate">
<StackPanel Background="Red">
<TextBlock Text="{Binding Name}"/>
<TextBlock Text="电话家长警告!"/>
</StackPanel>
</DataTemplate>
</Window.Resources>
<Grid>
<ListBox x:Name="list" ItemsSource="{Binding Model}">
<ListBox.ItemTemplateSelector>
<local:TestDataTemplateSelector HighTemplate="{StaticResource HighTemplate}" LowTemplate="{StaticResource LowTemplate}"/>
</ListBox.ItemTemplateSelector>
</ListBox>
</Grid>
</Window>
MainWindow.xaml.cs code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WpfApp2
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
List<Student> stuList = new List<Student>();
stuList.Add(new Student() { Name = "A", Result = 40 });
stuList.Add(new Student() { Name = "B", Result = 50 });
stuList.Add(new Student() { Name = "C", Result = 60 });
stuList.Add(new Student() { Name = "D", Result = 70 });
stuList.Add(new Student() { Name = "E", Result = 80 });
list.DataContext = new { Model = stuList};
}
}
public class Student
{
public string Name { get; set; }
public int Result { get; set; }
}
}
operation result:
Select different templates to display data according to the results of students' grades.
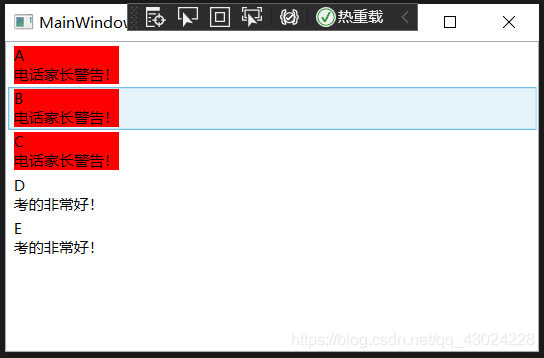