js judges whether it is null, undefined, NaN, empty string or empty object
Write directory title here
special value
null
Is a special value that represents an empty or non-existent object reference, indicating that a variable is artificially set to an empty object, not the original state.
undefined
When a variable is declared but not assigned a value, its default value is undefined. Also, when accessing non-existing object properties or array elements, the result is undefined.
NaN
(Not a Number) is a special value that indicates that when performing a mathematical operation, the result is not a legal number. NaN is of type Number, which is not equal to any value, including NaN itself.
In the case of NaN:
- Invalid operation in math operation, for example:
0 / 0
orMath.sqrt(-1)
- When coercing a non-numeric value to a number, for example:
parseInt("abc")
orNumber("xyz")
empty string ("")
An empty string is a zero-length string. It's not null or undefined, but a perfectly valid string, except that it has no content.
empty object ({})
An empty object is an object without any properties. It's not null, undefined, or an empty string, but a valid JavaScript object.
typeof function
Basic types: String, Number, Boolean, Symbol, Undefined, Null
Reference type: Object (object, array, function)
typeof '' // "string"
typeof 'Bill Gates' // "string"
typeof 0 // "number"
typeof 3.14 // "number"
typeof false // "boolean"
typeof true // "boolean"
typeof Symbol() // "symbol"
typeof Symbol('mySymbol'); // "symbol"
typeof undefined // "undefined"
typeof null // "object"
typeof {name:'Bill', age:62} // "object"
typeof [0, 1, 2] // "object"
typeof function(){} // "function"
typeof (()=>{}) // "function"
typeof testnull // "function" testnull是函数名
Judgmentundefined
let a = undefined;
if (typeof (a) === "undefined" || a === undefined) {
console.log("undefined");
}

judge null
have to be aware of istypeof(null) = object
let b = null;
console.log(typeof (b));
if (b === null) {
console.log("null1");
}
// !key && typeof(key)!=undefined 过滤完之后只剩 null 和 0 了,再用一个 key!=0 就可以把 0 过滤掉了
if (!b && typeof (b) != "undefined" && b != 0) {
console.log("null2");
}
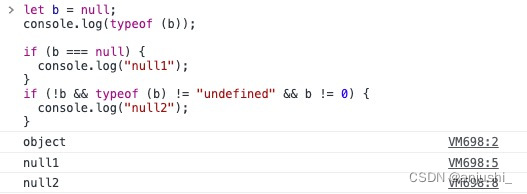
undefined and null
if(a == null) { // 等同于 a === undefined || a === null
console.log("为null");
}
undefined and null are equal when compared with ==
The type of null is object, and the type of undefined is undefined
== is to convert the left and right sides into the same type first, and then distinguish
Understanding of === and ==
=== : Strictly equal, the type will be compared first, and then the value will be compared
== : Loose equality is to convert the left and right sides into the same type first, and then distinguish them. Only the values are required to be equal, and the type is not required. When true is converted
to an integer, it is 1, and when false is converted to an integer, it is 0
https://cloud.tencent.com/developer/article/1703891
https://blog.csdn.net/qq_42533666/article/details/129190944
Judge NaN
NaN has the characteristic of non-reflexivity. The so-called non-reflexivity means that NaN is not equal to anyone, including itself, but it will return true under NaN != NaN, which can be judged according to this characteristic
let num = NaN;
let num2 = 0/0;
if (typeof (num) === "number" && isNaN(num)) {
console.log("num is NaN1");
}
if (num2 != num2) {
console.log('num is NaN2');
}
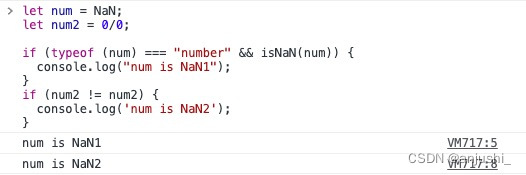
https://blog.csdn.net/Lu5957/article/details/122609879
https://blog.csdn.net/qq_43563538/article/details/103815482
https://www.cnblogs.com/k1023/p/11851943.html
Judge empty string
Compare it directly with the empty string, or call the trim() function to remove the spaces before and after, and then judge the length of the string
- Judging that the string is empty (space counts as empty)
let str = " ";
if (str === '' || str.trim().length === 0){
console.log('empty str 1');
}
if (typeof str == 'string' && str.length > 0) {
console.log('not empty str');
}
- Judging that the string is not empty (spaces are not empty)
if (typeof s == 'string' && s.length > 0) {
console.log('not empty str');
}
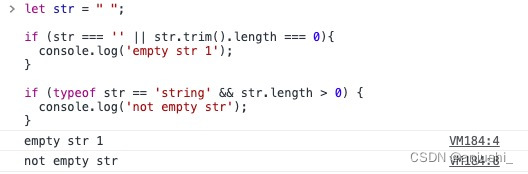
https://blog.csdn.net/K346K346/article/details/113182838
Judgment object is empty
Use JSON.stringify()
Convert the JavaScript value into a JSON string, and then determine whether the string is "{}"
let data = {};
if (JSON.stringify(data) === '{}') {
console.log('empty obj 1');
}
Use the method Object.keys() of es6
if (Object.keys(data).length === 0) {
console.log('empty obj 2');
}
for in loop judgment
Traverse the properties of variables through the for...in statement
if (judge(data)) {
console.log('empty obj 3');
}
function judge(data) {
for (let key in data) {
return false;
}
return true;
}
Use the getOwnPropertyNames method of the Object object
Use the getOwnPropertyNames method of the Object object to get the property names in the object, store them in an array, return the array object, and judge whether the object is empty by judging the length of the array
if (Object.getOwnPropertyNames(data).length == 0) {
console.log('empty obj 4');
}
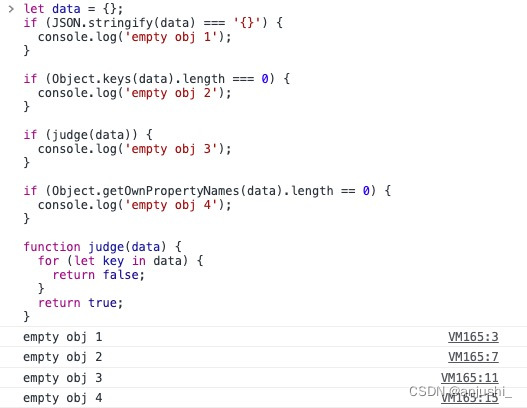
https://www.jb51.net/article/209387.htm
https://www.cnblogs.com/shanhubei/p/16969190.html
https://blog.csdn.net/qq_38709383/article/details/123224833
Judge empty array
First determine whether the variable is an array, and then determine it through the length attribute
let arr = [];
if (arr.length == 0) {
console.log('empty arr 1');
}
if (arr instanceof Array && !arr.length) {
console.log('empty arr 2');
}
if (Array.isArray(arr) && !arr.length) {
console.log('empty arr 3');
}
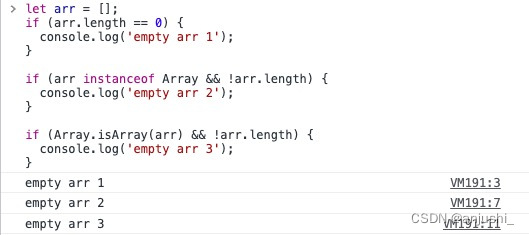