1. Use a stack to convert decimal to octal
2. Methods and steps
Receive an integer entered by the user from the keyboard as the decimal number to be converted.
Use the bottom-up algorithm to calculate the octal number by dividing by 8 and taking the remainder: that is, divide the base number by 8, then use the remainder as the lower digit of the octal number, and then continue to divide the obtained quotient by 8, and so on until the quotient is 0.
Each remainder needs to be saved in a list, and the elements added to the list must be output first, that is, last in first out, and the stack structure is used for processing.
3. Code Analysis
importjava.util.Scanner;
importjava.util.Stack;
public classDecimalToOctalConvertor {
public static void main(String[] args) {
Scanner reader = new Scanner(System.in);
System.out.print("Please enter the decimal number to be converted:");
int decimalNum = reader.nextInt(); /*
Stack<Integer> octalStack = newStack<Integer>();
int temp = decimalNum;
do {
octalStack.push(temp % 8); temp /= 8; }
while (temp != 0);
System.out.print("Octal: 0");
while (!octalStack.isEmpty()) {
System.out.print(octalStack.pop());
}
System.out.println();
}
}
4. Results and analysis
Input decimal 17 outputs octal 021
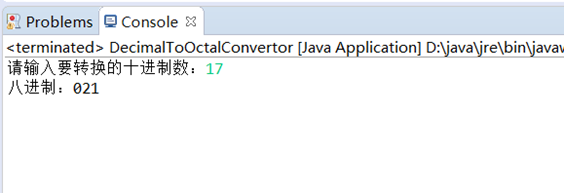