Those who are full of heart are as good as others when they walk alone
1. Register an Alibaba Cloud account and activate the OSS service
1. Log in to your Alibaba Cloud account
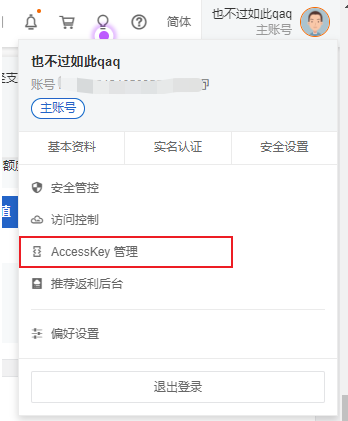
2. Create a bucket
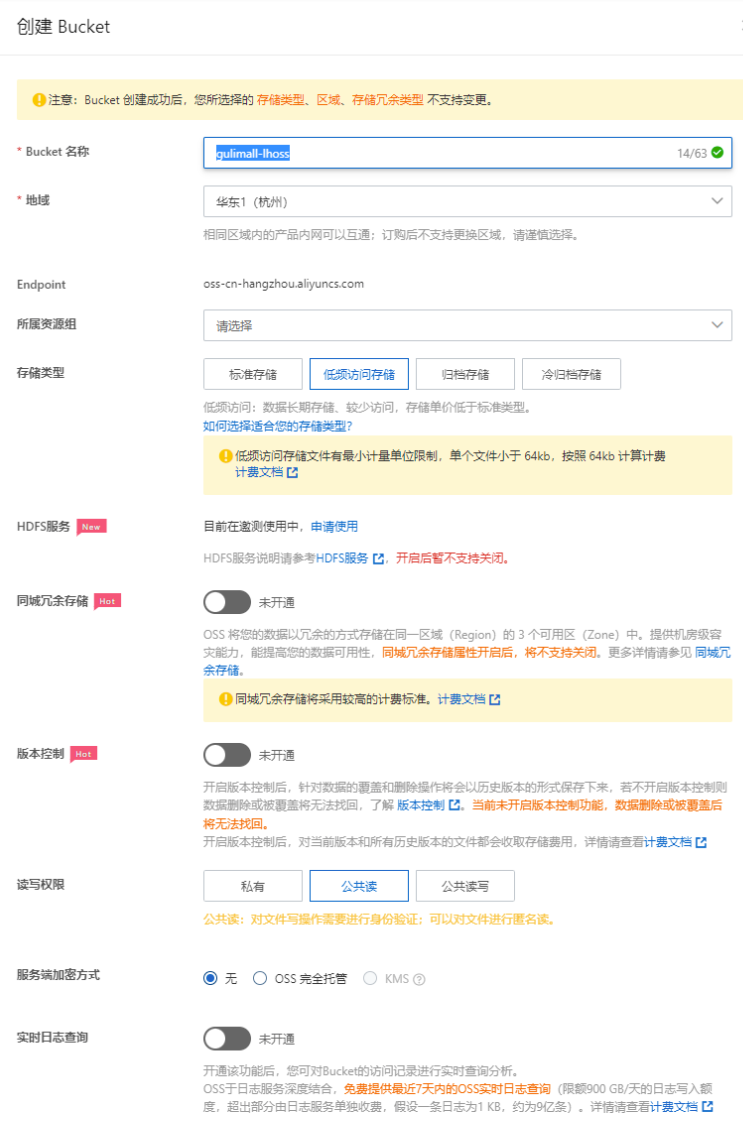
In actual projects, the read and write permissions can be private, that is, authentication is required for both the read and write permissions of files in the storage space.
3. Create a sub-user
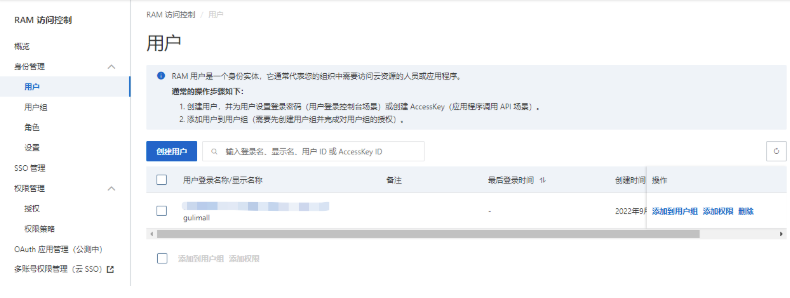
4. Assign permissions
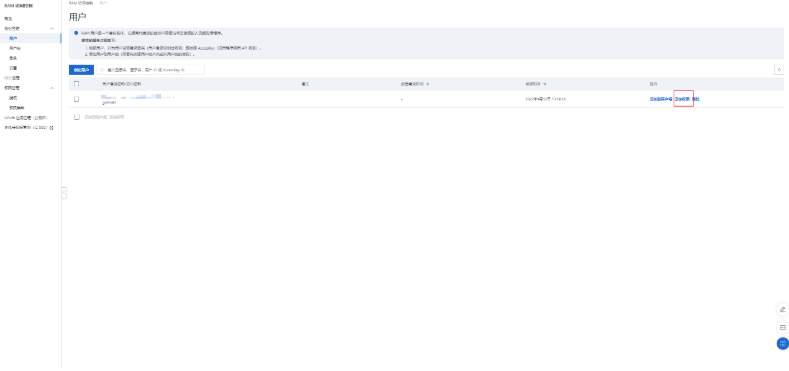
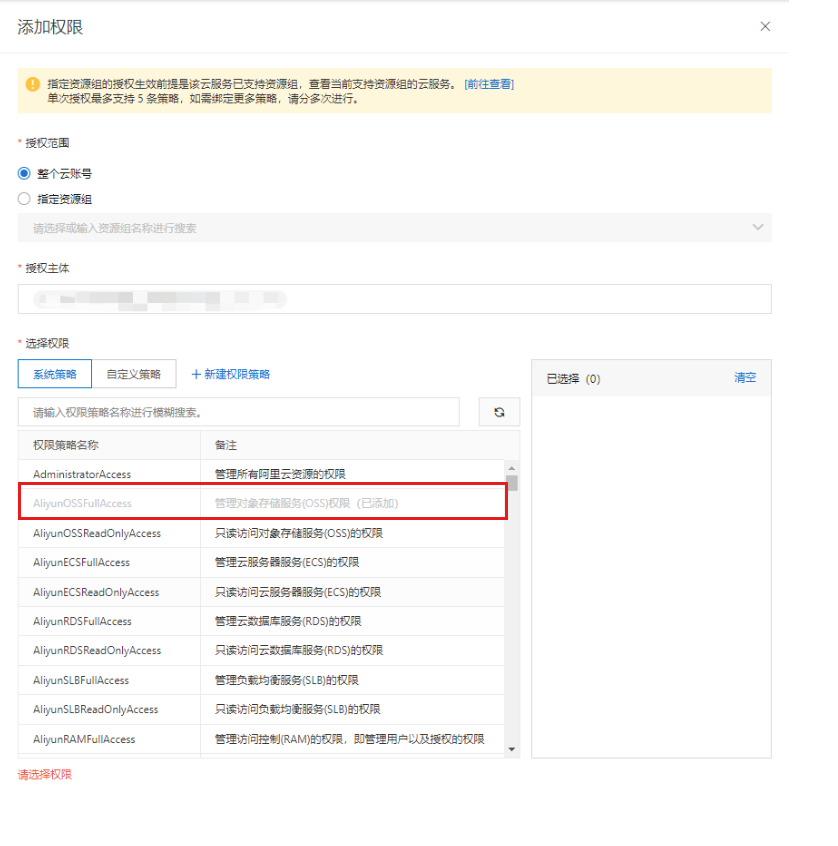
5. Add upload cross-domain rules
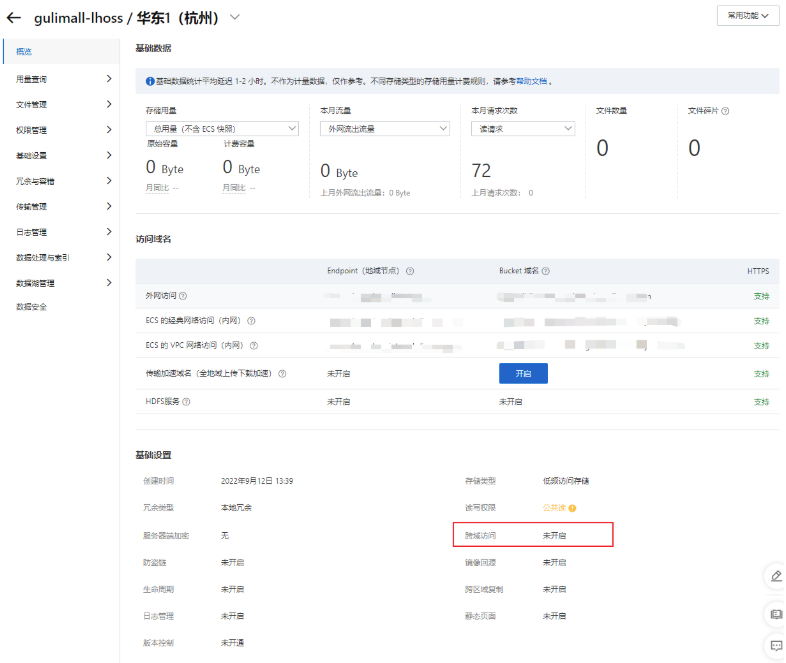
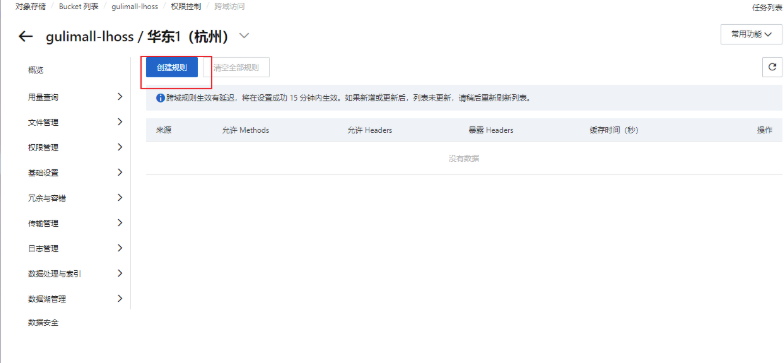
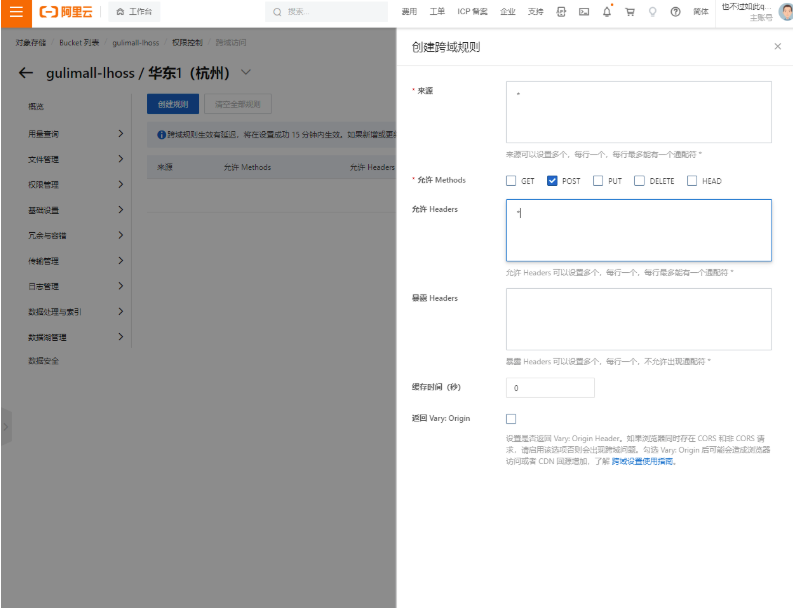
2. Project example
spring simple upload
Integrate Springboot
Combat demo
Introduce maven dependency
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>aliyun-oss-spring-boot-starter</artifactId>
</dependency>
Add relevant Alibaba Cloud configuration to the configuration file
#AliyunOSS
AccessKeyID:
AccessKeySecret:
RoleArn:
TokenExpireTime:
PolicyFile:
Endpoint:
BucketName:
OssInternalEndpoint:
RegionId:
ProjectName:
EndpointStorage:
Alibaba cloud oss object storage tool class
/**
* 阿里云oss对象存储工具类
* @author lh
*/
public class AliyunSdkUtil
{
private static final Logger LOG = LoggerFactory.getLogger(AliyunSdkUtil.class);
/**
* 图片格式
*/
private static final List<String> PICTURE_TYPE = Lists.newArrayList("bmp", "jpg", "jpeg", "png", "tif", "gif",
"pcx", "tga", "exif", "fpx", "svg", "psd", "cdr", "pcd", "dxf", "ufo", "eps", "ai", "raw", "WMF", "webp",
"avif", "mp4");
private static Map<String, String> pictureFromateMap;
static
{
pictureFromateMap = new HashMap<String, String>();
pictureFromateMap.put("FFD8FF", "jpg");
pictureFromateMap.put("89504E", "png");
pictureFromateMap.put("474946", "gif");
}
public static String endpoint = ContextApplicationConst.ENDPOINT;
public static String accessKeyId = ContextApplicationConst.ACCESSKEYID;
public static String accessKeySecret = ContextApplicationConst.ACCESSKEYSECRET;
public static String bucketName = ContextApplicationConst.BUCKETNAME;
public static String regionId = ContextApplicationConst.REGIONID;
public static String projectName = ContextApplicationConst.PROJECTNAME;
protected static String roleArn = ContextApplicationConst.ROLEARN;
protected static long tokenExpireTime = ContextApplicationConst.TOKENEXPIRETIME;
protected static String policyFile = ContextApplicationConst.POLICYFILE;
/**
* 获取阿里云Token
*/
public static Map<String, String> getAliyunToken() throws IOException
{
Map<String, String> respMap = new LinkedHashMap<String, String>();
respMap.put("AccessKeyId", "");
respMap.put("AccessKeySecret", "");
respMap.put("SecurityToken", "");
respMap.put("Expiration", "");
String point = "";
if (!CommonUtil.isEmpty(endpoint))
{
point = endpoint.substring(0, endpoint.indexOf("."));
}
long durationSeconds = tokenExpireTime;
String policy = AliyunUtil.readJson(AliyunSdkUtil.class.getResourceAsStream("/" + policyFile));
// 此处必须为 HTTPS
ProtocolType protocolType = ProtocolType.HTTPS;
try
{
final AssumeRoleResponse stsResponse = assumeRole(accessKeyId, accessKeySecret, roleArn,
AliyunUtil.ROLE_SESSION_NAME, policy, protocolType, durationSeconds);
respMap.put("status", "200");
respMap.put("AccessKeyId", stsResponse.getCredentials().getAccessKeyId());
respMap.put("AccessKeySecret", stsResponse.getCredentials().getAccessKeySecret());
respMap.put("SecurityToken", stsResponse.getCredentials().getSecurityToken());
respMap.put("Expiration", stsResponse.getCredentials().getExpiration());
respMap.put("bucket", bucketName);
respMap.put("region", regionId);
respMap.put("tokenExpireTime", String.valueOf(tokenExpireTime));
respMap.put("endpoint", point);
} catch (com.aliyuncs.exceptions.ClientException e)
{
respMap.put("status", e.getErrCode());
}
return respMap;
}
@SuppressWarnings("deprecation")
protected static AssumeRoleResponse assumeRole(String accessKeyId, String accessKeySecret, String roleArn,
String roleSessionName, String policy, ProtocolType protocolType, long durationSeconds)
throws com.aliyuncs.exceptions.ClientException
{
try
{
IClientProfile profile = DefaultProfile.getProfile(AliyunUtil.REGION_CN_HANGZHOU, accessKeyId,
accessKeySecret);
DefaultAcsClient client = new DefaultAcsClient(profile);
final AssumeRoleRequest request = new AssumeRoleRequest();
request.setVersion(AliyunUtil.STS_API_VERSION);
request.setMethod(MethodType.POST);
request.setProtocol(protocolType);
request.setRoleArn(roleArn);
request.setRoleSessionName(roleSessionName);
request.setPolicy(policy);
request.setDurationSeconds(durationSeconds);
// 发起请求,并得到response
final AssumeRoleResponse response = client.getAcsResponse(request);
return response;
} catch (com.aliyuncs.exceptions.ClientException e)
{
throw e;
}
}
/**
* 文件上传
* @param filePath 文件路径
*/
public static String uploadDocument(String filePath) throws IOException
{
/*Socket socket=new Socket();
SocketAddress remoteAddr=new InetSocketAddress("192.168.6.248",8081);
//等待建立连接的超时时间为1分钟
socket.connect(remoteAddr, 60000);*/
//fileId便是阿里云的alikey
String fileId = CommonUtil.getUUID();
OSSClient ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
try
{
File file = new File(filePath);
ossClient.putObject(new PutObjectRequest(bucketName, fileId, file));
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileId;
}
/**
* 文件预览
* @param aliKeyId 阿里附件ID
* @param suffix 文件后缀
* @return 预览路径
* @throws Exception
*/
public static String previewByAliKeyId(String aliKeyId, String suffix) throws Exception
{
if (CommonUtil.isEmpty(aliKeyId))
{
throw new NormalRuntimeException("请选择需要预览的文件信息!");
}
if (CommonUtil.isEmpty(suffix))
{
throw new NormalRuntimeException("文件类型不能为空!");
}
if (PICTURE_TYPE.contains(suffix.toLowerCase()))
{
return getProvisionalUrl(aliKeyId).toString();
}
boolean flag = CommonUtil.isEmpty(suffix) ? false : CommonUtil.isCanPreview(suffix.toLowerCase());
if (!flag)
{
throw new NormalRuntimeException("该文件类型不可预览!");
}
return getPreview(aliKeyId);
}
/**
* 批量获取访问地址
* @param aliKeyList
* @return
*/
public static Map<String, URL> getUrlList(List<String> aliKeyList)
{
Map<String, URL> dataMap = Maps.newHashMap();
OSSClient ossClient = null;
try
{
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
// 设置URL过期时间为1小时。
Date expiration = new Date(System.currentTimeMillis() + 3600 * 1000);
URL url = null;
for (String aliKey : aliKeyList)
{
url = ossClient.generatePresignedUrl(bucketName, aliKey, expiration);
dataMap.put(aliKey, url);
}
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return dataMap;
}
@SuppressWarnings("deprecation")
public static String getPreview(String alikey) throws Exception
{
//处理业务逻辑
String srcUri = "oss://" + bucketName + "/" + alikey;
//调试所用
//srcUri = "oss://booway-tz/ZBGmMK_1589855755833.docx";
DefaultProfile profile = DefaultProfile.getProfile(regionId, accessKeyId, accessKeySecret);
IAcsClient client = new DefaultAcsClient(profile);
GetOfficePreviewURLRequest request = new GetOfficePreviewURLRequest();
request.setRegionId(regionId);
request.setProject(projectName);
request.setSrcUri(srcUri);
request.setActionName("GetOfficePreviewURL");
String result = "";
try
{
GetOfficePreviewURLResponse response = client.getAcsResponse(request);
result = new Gson().toJson(response);
} catch (ClientException e)
{
throw new Exception(e);
}
return result;
}
// 获取上传文件地址
public static URL getProvisionalUrl(String aliKey)
{
OSSClient ossClient = null;
URL url = null;
try
{
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
// 设置URL过期时间为1小时。
Date expiration = new Date(System.currentTimeMillis() + 3600 * 1000);
// 生成以GET方法访问的签名URL,访客可以直接通过浏览器访问相关内容。
url = ossClient.generatePresignedUrl(bucketName, aliKey, expiration);
// System.out.println(url);
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return url;
}
public static String uploadDocument(InputStream stream, String fileName)
{
//fileId便是阿里云的alikey
String fileId = CommonUtil.getUUID() + fileName;
OSSClient ossClient = null;
try
{
// 创建上传Object的Metadata
ObjectMetadata meta = new ObjectMetadata();
// 设置上传文件长度
meta.setContentLength(stream.available());
// 设置上传内容类型
meta.setContentType("text/plain");
meta.setContentDisposition("attachment;filename=\"" + fileName + "\"");
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(new PutObjectRequest(bucketName, fileId, stream, meta));
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} catch (IOException e)
{
LOG.error("本地流处理出错,上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileId;
}
/**
* @Description 阿里云OSS附件流形式上传(alikey需要带附件后缀,否则预览时会报不支持的文件类型)
* @Param [stream, fileName, suffix]
* @return java.lang.String
**/
public static String uploadDocumentSuffix(InputStream stream, String fileName, String suffix)
{
//fileId便是阿里云的alikey
String fileId = CommonUtil.getUUID() + "." + suffix.toLowerCase();
OSSClient ossClient = null;
try
{
// 创建上传Object的Metadata
ObjectMetadata meta = new ObjectMetadata();
// 设置上传文件长度
meta.setContentLength(stream.available());
// 设置上传内容类型
//meta.setContentType("text/plain");
meta.setContentType(getcontentType(fileName.substring(fileName.lastIndexOf("."))));
meta.setContentDisposition("attachment;filename=\"" + fileName + "\"");
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(new PutObjectRequest(bucketName, fileId, stream, meta));
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} catch (IOException e)
{
LOG.error("本地流处理出错,上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileId;
}
/**
* 告警图片上传
* @param stream 文件流
* @param fileName 文件名
* @param suffix 文件后缀
* @return key
*/
public static String aiAlarmUploadDocumentSuffix(InputStream stream, String fileName, String suffix)
{
OSSClient ossClient = null;
try
{
// 创建上传Object的Metadata
ObjectMetadata meta = new ObjectMetadata();
// 设置上传文件长度
meta.setContentLength(stream.available());
// 设置上传内容类型
//meta.setContentType("text/plain");
meta.setContentType(getcontentType(fileName.substring(fileName.lastIndexOf("."))));
meta.setContentDisposition("attachment;filename=\"" + fileName + "\"");
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(new PutObjectRequest(bucketName, fileName, stream, meta));
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} catch (IOException e)
{
LOG.error("本地流处理出错,上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileName;
}
/**
* @Description 文件类型区分
* @Param filenameExtension
* @return java.lang.String
**/
public static String getcontentType(String filenameExtension)
{
if (filenameExtension.equalsIgnoreCase(".bmp"))
{
return "image/bmp";
}
if (filenameExtension.equalsIgnoreCase(".gif"))
{
return "image/gif";
}
if (filenameExtension.equalsIgnoreCase(".jpeg") || filenameExtension.equalsIgnoreCase(".jpg")
|| filenameExtension.equalsIgnoreCase(".png"))
{
return "image/jpg";
}
if (filenameExtension.equalsIgnoreCase(".html"))
{
return "text/html";
}
if (filenameExtension.equalsIgnoreCase(".txt"))
{
return "text/plain";
}
if (filenameExtension.equalsIgnoreCase(".vsd"))
{
return "application/vnd.visio";
}
return backContentType(filenameExtension);
}
private static String backContentType(String filenameExtension)
{
if (filenameExtension.equalsIgnoreCase(".pptx") || filenameExtension.equalsIgnoreCase(".ppt"))
{
return "application/vnd.ms-powerpoint";
}
if (filenameExtension.equalsIgnoreCase(".docx") || filenameExtension.equalsIgnoreCase(".doc"))
{
return "application/msword";
}
if (filenameExtension.equalsIgnoreCase(".xml"))
{
return "text/xml";
}
return "text/plain";
}
public static String uploadDocument(InputStream stream, String fileName, String fileId)
{
//fileId便是阿里云的alikey
//o String fileId = CommonUtil.getUUID();
OSSClient ossClient = null;
try
{
// 创建上传Object的Metadata
ObjectMetadata meta = new ObjectMetadata();
// 设置上传文件长度
meta.setContentLength(stream.available());
// 设置上传内容类型
meta.setContentType("text/plain");
meta.setContentDisposition("attachment;filename=\"" + fileName + "\"");
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(new PutObjectRequest(bucketName, fileId, stream, meta));
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} catch (IOException e)
{
LOG.error("本地流处理出错,上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileId;
}
/**
* 用于获取网络图片 上传OSS
* 图片先下载到本地,上传后删除
* @param url
* @param fileId
* @return
*/
public static String uploadDocumentByUrl(String url, String fileId)
{
//fileId便是阿里云的alikey
OSSClient ossClient = null;
String fileName = "";
try
{
URL imgUrl = new URL(url);
URLConnection openConnection = imgUrl.openConnection();
//获取图片的实际长度
long contentLength = openConnection.getContentLengthLong();
if (contentLength > 2097152)
{
return null;
}
InputStream inStream = imgUrl.openStream();
ObjectMetadata meta = new ObjectMetadata();
// 获取文件类型
String formate = getPictureFormate(inStream);
String contentType = "image/" + (formate.equals("jpg") ? "jpeg" : formate);
// 设置上传内容类型 image/jpeg image/png image/gif
meta.setContentType(contentType);
fileName = fileId + "." + formate;
meta.setContentDisposition("attachment;filename=\"" + fileName + "\"");
download(url, fileName, "/temp");
File newFile = new File("/temp/" + fileName);
// 设置上传文件长度
meta.setContentLength(newFile.length());
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(new PutObjectRequest(bucketName, fileName, newFile, meta));
// 删除临时文件
newFile.delete();
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} catch (IOException e)
{
LOG.error("本地流处理出错,上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileName;
}
public static String uploadDocumentForNewName(File file, String newName) throws Exception
{
String fileId = CommonUtil.getUUID();
// System.out.println("ID:" + fileId);
OSSClient ossClient = null;
try
{
// 创建上传Object的Metadata
ObjectMetadata meta = new ObjectMetadata();
// 设置上传文件长度
meta.setContentLength(file.length());
// 设置上传内容类型
meta.setContentType("text/plain");
meta.setContentDisposition("attachment;filename=\"" + newName + "\"");
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(bucketName, fileId, file, meta);
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileId;
}
/**
* 删除阿里云oss对应key的文件
*
* @param key
*/
public static void deleteDocument(String key)
{
OSSClient ossClient = null;
try
{
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
ossClient.deleteObject(bucketName, key);
} catch (OSSException e)
{
LOG.error("删除阿里云附件失败:" + key, e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
}
/**
* 获取阿里云oss的文件
* @param key
* @return
* @throws Exception
*/
public static InputStream getDocument(String key) throws Exception
{
OSSClient ossClient = null;
OSSObject object = null;
InputStream input = null;
try
{
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
object = ossClient.getObject(bucketName, key);
return new DataInputStream(object.getObjectContent());
} catch (OSSException oe)
{
System.out.println("Caught an OSSException, which means your request made it to OSS, "
+ "but was rejected with an error response for some reason.");
System.out.println("Error Message: " + oe.getErrorCode());
System.out.println("Error Code: " + oe.getErrorCode());
System.out.println("Request ID: " + oe.getRequestId());
System.out.println("Host ID: " + oe.getHostId());
} catch (ClientException ce)
{
System.out.println("Caught an ClientException, which means the client encountered "
+ "a serious internal problem while trying to communicate with OSS, "
+ "such as not being able to access the network.");
System.out.println("Error Message: " + ce);
} finally
{
if (object != null)
{
// object.close();
}
if (ossClient != null)
{
// ossClient.shutdown();
}
}
return input;
}
/**
* 获取阿里云oss的文件
* @param aliKey 阿里云key集合
* @return
* @throws Exception
*/
public static Map<String, InputStream> getDocument(List<String> aliKey) throws Exception
{
OSSClient ossClient = null;
Map<String, InputStream> map = Maps.newHashMap();
try
{
OSSObject obj = null;
ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
for (String alikey : aliKey)
{
try
{
obj = ossClient.getObject(bucketName, alikey);
map.put(alikey, new DataInputStream(obj.getObjectContent()));
} catch (OSSException oe)
{
System.out.println("Caught an OSSException, which means your request made it to OSS, "
+ "but was rejected with an error response for some reason.");
System.out.println("Error Message: " + oe.getErrorCode());
System.out.println("Error Code: " + oe.getErrorCode());
System.out.println("Request ID: " + oe.getRequestId());
System.out.println("Host ID: " + oe.getHostId());
} catch (ClientException ce)
{
System.out.println("Caught an ClientException, which means the client encountered "
+ "a serious internal problem while trying to communicate with OSS, "
+ "such as not being able to access the network.");
System.out.println("Error Message: " + ce);
}
}
} finally
{
if (ossClient != null)
{
// ossClient.shutdown();
}
}
return map;
}
/**
* 获取文件的MD5值
* @param fileKey 阿里云返回的文件key
* @return MD5
* @throws Exception
*/
public static String getFileMD5(String fileKey) throws Exception
{
String returnValue = null;
InputStream inputStream = null;
int i = 0;
do
{
// System.out.println(i + "============================>");
try
{
inputStream = AliyunSdkUtil.getDocument(fileKey);//文件流
returnValue = DigestUtils.md5Hex(inputStream);//文件MD5
break;
} catch (Exception e)
{
LOG.error("阿里云获取文件失败", e);
if (i > 50)
{
throw new Exception("阿里云获取文件失败", e);
}
i++;
Thread.sleep(200);
} finally
{
if (null != inputStream)
{
inputStream.close();
}
}
} while (true);
return returnValue;
}
/**TODO
* 删除无效的附件数据
* 只限删除前一天的所有无效数据
*/
public static void deleteInvalidAttachment()
{
}
private AliyunSdkUtil()
{
super();
}
public static String uploadDocument(String filePath, String fileName) throws IOException
{
/*Socket socket=new Socket();
SocketAddress remoteAddr=new InetSocketAddress("192.168.6.248",8081);
//等待建立连接的超时时间为1分钟
socket.connect(remoteAddr, 60000);*/
//fileId便是阿里云的alikey
String fileId = CommonUtil.getUUID() + fileName;
OSSClient ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
try
{
File file = new File(filePath);
ossClient.putObject(new PutObjectRequest(bucketName, fileId, file));
} catch (OSSException e)
{
LOG.error("上传数据至阿里云失败!", e);
} finally
{
if (ossClient != null)
{
ossClient.shutdown();
}
}
return fileId;
}
/**
* 从文件流中读出图片格式 仅支持3种格式
* JPEG
* PNG
* GIF
*
* @param inStream
* @return
*/
private static String getPictureFormate(InputStream inStream)
{
byte[] b = new byte[3];
try
{
inStream.read(b, 0, b.length);
} catch (IOException e)
{
LOG.error("读取流异常", e);
}
String head = bytesToHexString(b);
head = head.toUpperCase();
return pictureFromateMap.get(head);
}
/**
* 将文件头转换成16进制字符串
*
* @param
* @return 16进制字符串
*/
private static String bytesToHexString(byte[] src)
{
StringBuilder stringBuilder = new StringBuilder();
if (src == null || src.length <= 0)
{
return null;
}
for (int i = 0; i < src.length; i++)
{
int v = src[i] & 0xFF;
String hv = Integer.toHexString(v);
if (hv.length() < 2)
{
stringBuilder.append(0);
}
stringBuilder.append(hv);
}
return stringBuilder.toString();
}
/**
* 下载图片到本地
*
* @param urlString
* @param filename
* @param savePath
* @throws Exception
*/
public static void download(String urlString, String filename, String savePath) throws IOException
{
// 构造URL
URL url = new URL(urlString);
// 打开连接
URLConnection con = url.openConnection();
//设置请求超时为5s
con.setConnectTimeout(5 * 1000);
// 输入流
InputStream is = con.getInputStream();
// 1K的数据缓冲
byte[] bs = new byte[1024];
// 读取到的数据长度
int len;
// 输出的文件流
File sf = new File(savePath);
if (!sf.exists())
{
sf.mkdirs();
}
OutputStream os = new FileOutputStream(sf.getPath() + "/" + filename);
// 开始读取
while ((len = is.read(bs)) != -1)
{
os.write(bs, 0, len);
}
// 完毕,关闭所有链接
os.close();
is.close();
}
}
context constant
/**
* 上下文常量类
*/
@Component
public interface ContextApplicationConst
{
/** aliyun-oss相关配置 start*/
public static String ACCESSKEYID = SpringContextConfig.getProp("AccessKeyID");
public static String ACCESSKEYSECRET = SpringContextConfig.getProp("AccessKeySecret");
public static String ENDPOINT = SpringContextConfig.getProp("Endpoint");
public static String REGIONID = SpringContextConfig.getProp("RegionId");
public static String BUCKETNAME = SpringContextConfig.getProp("BucketName");
public static String ROLEARN = SpringContextConfig.getProp("RoleArn");
public static long TOKENEXPIRETIME = Long.valueOf(SpringContextConfig.getProp("TokenExpireTime"));
public static String POLICYFILE = SpringContextConfig.getProp("PolicyFile");
public static String PROJECTNAME = SpringContextConfig.getProp("ProjectName");
}
upload picture
@RequestMapping(value = "/aiInfo", method = RequestMethod.POST)
public void ai(@RequestBody JSONObject json) throws IOException
{
// 解析Json数据
String jsonStr = JSONObject.toJSONString(json);
AiInfo params = JSON.parseObject(jsonStr, AiInfo.class);
// 图片往OSS里面存
String fileId = AliyunSdkUtil.aiAlarmUploadDocumentSuffix(ImageUtils.baseToInputStream(params.getImgBase64()),
TokenUtil.getUUID() + ".jpg", "jpg");
}
take pictures
URL url = AliyunSdkUtil.getUrlListToList(aliKey);
There are two ways to take pictures:
Directly give the aliKey of the front-end OSS, and the front-end directly fetches the picture from the OSS for display by obtaining the front-end returned by the back-end. (pc side)
Give the front-end a url (absolute path) that can be accessed directly, and the front-end directly accesses the picture through this url.
Attached:
all_policy.txt
{
"Statement": [
{
"Action": [
"oss:*"
],
"Effect": "Allow",
"Resource": ["acs:oss:*:*:*"]
}
],
"Version": "1"
}
The content of the file can be modified according to the authority, the official document .